 |
|
C# WinApp datetimepicker ใน WinApp สามารถตั้งค่าให้เลือกได้ ทั้ง วันที่ และ เวลา ตรงไหน ครับ |
|
 |
|
|
 |
 |
|
แอบดูน้องเมียอาบน้ำ ยังยากกว่านี้เสียอีก
|
 |
 |
 |
 |
Date :
2021-01-26 15:17:42 |
By :
ผ่านมา |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
https://www.youtube.com/watch?v=rH_PywHKBCg
|
 |
 |
 |
 |
Date :
2021-01-26 16:19:39 |
By :
ผ่านมา |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ขอสอบถามต่อเลยนะครับ
มีใครเคยแงะ แกะ class DateTimePicker ของ .net ไม๊ ครับ
ไม่ทราบว่า บรรทัดไหน ที่บอกว่า มีการสร้าง ออกมาเป็น วันที่ หรือ สร้างเป็น เวลาครับ
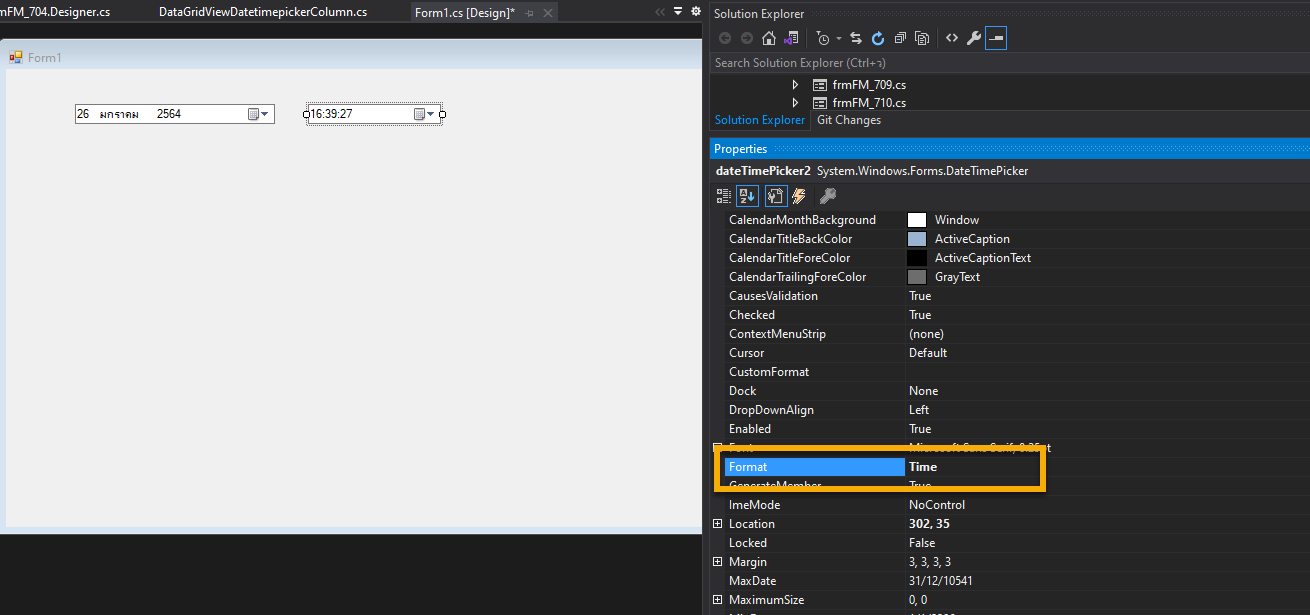
คือเบื้องต้น อยากลอง เขียนให้เวลาคลิก แล้วให้สร้างทั้ง วันที่ และ เวลา ดูก่อน ถ้าไม่ได้ก็จะเขียน control ใหม่ขึ้นมาใช้ครับ
Code (C#)
public class DateTimePicker : Control {
protected static readonly Color DefaultTitleBackColor = SystemColors.ActiveCaption;
protected static readonly Color DefaultTitleForeColor = SystemColors.ActiveCaptionText;
protected static readonly Color DefaultMonthBackColor = SystemColors.Window;
protected static readonly Color DefaultTrailingForeColor = SystemColors.GrayText;
private static readonly object EVENT_FORMATCHANGED = new object();
private const int TIMEFORMAT_NOUPDOWN = NativeMethods.DTS_TIMEFORMAT & (~NativeMethods.DTS_UPDOWN);
private EventHandler onCloseUp;
private EventHandler onDropDown;
private EventHandler onValueChanged;
private EventHandler onRightToLeftLayoutChanged;
[Browsable(false), EditorBrowsable(EditorBrowsableState.Never)]
public static readonly DateTime MinDateTime = new DateTime(1753, 1, 1);
[Browsable(false), EditorBrowsable(EditorBrowsableState.Never)]
public static readonly DateTime MaxDateTime = new DateTime(9998, 12, 31);
private int style;
private short prefHeightCache = -1;
private bool validTime = true;
private bool userHasSetValue = false;
private DateTime value = DateTime.Now;
private DateTime creationTime = DateTime.Now;
// VSWhidbey 400284: Reconcile out-of-range min/max values in the property getters.
private DateTime max = DateTime.MaxValue;
private DateTime min = DateTime.MinValue;
private Color calendarForeColor = DefaultForeColor;
private Color calendarTitleBackColor = DefaultTitleBackColor;
private Color calendarTitleForeColor = DefaultTitleForeColor;
private Color calendarMonthBackground = DefaultMonthBackColor;
private Color calendarTrailingText = DefaultTrailingForeColor;
private Font calendarFont = null;
private FontHandleWrapper calendarFontHandleWrapper = null;
private string customFormat;
private DateTimePickerFormat format;
private bool rightToLeftLayout = false;
public DateTimePicker()
: base() {
// this class overrides GetPreferredSizeCore, let Control automatically cache the result
SetState2(STATE2_USEPREFERREDSIZECACHE, true);
SetStyle(ControlStyles.FixedHeight, true);
SetStyle(ControlStyles.UserPaint |
ControlStyles.StandardClick, false);
format = DateTimePickerFormat.Long;
}
[Browsable(false), EditorBrowsable(EditorBrowsableState.Never)]
public override Color BackColor {
get {
if (ShouldSerializeBackColor()) {
return base.BackColor;
}
else {
return SystemColors.Window;
}
}
set {
base.BackColor = value;
}
}
[Browsable(false), EditorBrowsable(EditorBrowsableState.Never)]
new public event EventHandler BackColorChanged {
add {
base.BackColorChanged += value;
}
remove {
base.BackColorChanged -= value;
}
}
[Browsable(false), EditorBrowsable(EditorBrowsableState.Never)]
public override Image BackgroundImage {
get {
return base.BackgroundImage;
}
set {
base.BackgroundImage = value;
}
}
[Browsable(false), EditorBrowsable(EditorBrowsableState.Never)]
new public event EventHandler BackgroundImageChanged {
add {
base.BackgroundImageChanged += value;
}
remove {
base.BackgroundImageChanged -= value;
}
}
[Browsable(false), EditorBrowsable(EditorBrowsableState.Never)]
public override ImageLayout BackgroundImageLayout {
get {
return base.BackgroundImageLayout;
}
set {
base.BackgroundImageLayout = value;
}
}
[Browsable(false), EditorBrowsable(EditorBrowsableState.Never)]
new public event EventHandler BackgroundImageLayoutChanged {
add {
base.BackgroundImageLayoutChanged += value;
}
remove {
base.BackgroundImageLayoutChanged -= value;
}
}
[
SRCategory(SR.CatAppearance),
SRDescription(SR.DateTimePickerCalendarForeColorDescr)
]
public Color CalendarForeColor {
get {
return calendarForeColor;
}
set {
if (value.IsEmpty) {
throw new ArgumentException(SR.GetString(SR.InvalidNullArgument,
"value"));
}
if (!value.Equals(calendarForeColor)) {
calendarForeColor = value;
SetControlColor(NativeMethods.MCSC_TEXT, value);
}
}
}
[
SRCategory(SR.CatAppearance),
Localizable(true),
AmbientValue(null),
SRDescription(SR.DateTimePickerCalendarFontDescr)
]
public Font CalendarFont {
get {
if (calendarFont == null) {
return Font;
}
return calendarFont;
}
set {
if ((value == null && calendarFont != null) || (value != null && !value.Equals(calendarFont))) {
calendarFont = value;
calendarFontHandleWrapper = null;
SetControlCalendarFont();
}
}
}
private IntPtr CalendarFontHandle {
get {
if (calendarFont == null) {
Debug.Assert(calendarFontHandleWrapper == null, "font handle out of [....] with Font");
return FontHandle;
}
if (calendarFontHandleWrapper == null) {
calendarFontHandleWrapper = new FontHandleWrapper(CalendarFont);
}
return calendarFontHandleWrapper.Handle;
}
}
[
SRCategory(SR.CatAppearance),
SRDescription(SR.DateTimePickerCalendarTitleBackColorDescr)
]
public Color CalendarTitleBackColor {
get {
return calendarTitleBackColor;
}
set {
if (value.IsEmpty) {
throw new ArgumentException(SR.GetString(SR.InvalidNullArgument,
"value"));
}
if (!value.Equals(calendarTitleBackColor)) {
calendarTitleBackColor = value;
SetControlColor(NativeMethods.MCSC_TITLEBK, value);
}
}
}
[
SRCategory(SR.CatAppearance),
SRDescription(SR.DateTimePickerCalendarTitleForeColorDescr)
]
public Color CalendarTitleForeColor {
get {
return calendarTitleForeColor;
}
set {
if (value.IsEmpty) {
throw new ArgumentException(SR.GetString(SR.InvalidNullArgument,
"value"));
}
if (!value.Equals(calendarTitleForeColor)) {
calendarTitleForeColor = value;
SetControlColor(NativeMethods.MCSC_TITLETEXT, value);
}
}
}
[
SRCategory(SR.CatAppearance),
SRDescription(SR.DateTimePickerCalendarTrailingForeColorDescr)
]
public Color CalendarTrailingForeColor {
get {
return calendarTrailingText;
}
set {
if (value.IsEmpty) {
throw new ArgumentException(SR.GetString(SR.InvalidNullArgument,
"value"));
}
if (!value.Equals(calendarTrailingText)) {
calendarTrailingText = value;
SetControlColor(NativeMethods.MCSC_TRAILINGTEXT, value);
}
}
}
[
SRCategory(SR.CatAppearance),
SRDescription(SR.DateTimePickerCalendarMonthBackgroundDescr)
]
public Color CalendarMonthBackground {
get {
return calendarMonthBackground;
}
set {
if (value.IsEmpty) {
throw new ArgumentException(SR.GetString(SR.InvalidNullArgument,
"value"));
}
if (!value.Equals(calendarMonthBackground)) {
calendarMonthBackground = value;
SetControlColor(NativeMethods.MCSC_MONTHBK, value);
}
}
}
[
SRCategory(SR.CatBehavior),
DefaultValue(true),
Bindable(true),
SRDescription(SR.DateTimePickerCheckedDescr)
]
public bool Checked {
get {
// the information from win32 DateTimePicker is reliable only when ShowCheckBoxes is True
if (this.ShowCheckBox && IsHandleCreated) {
NativeMethods.SYSTEMTIME sys = new NativeMethods.SYSTEMTIME();
int gdt = (int)UnsafeNativeMethods.SendMessage(new HandleRef(this, Handle), NativeMethods.DTM_GETSYSTEMTIME, 0, sys);
return gdt == NativeMethods.GDT_VALID;
} else {
return validTime;
}
}
set {
if (this.Checked != value) {
// set the information into the win32 DateTimePicker only if ShowCheckBoxes is True
if (this.ShowCheckBox && IsHandleCreated) {
if (value) {
int gdt = NativeMethods.GDT_VALID;
NativeMethods.SYSTEMTIME sys = DateTimePicker.DateTimeToSysTime(Value);
UnsafeNativeMethods.SendMessage(new HandleRef(this, Handle), NativeMethods.DTM_SETSYSTEMTIME, gdt, sys);
}
else {
int gdt = NativeMethods.GDT_NONE;
NativeMethods.SYSTEMTIME sys = null;
UnsafeNativeMethods.SendMessage(new HandleRef(this, Handle), NativeMethods.DTM_SETSYSTEMTIME, gdt, sys);
}
}
// this.validTime is used when the DateTimePicker receives date time change notification
// from the Win32 control. this.validTime will be used to know when we transition from valid time to unvalid time
// also, validTime will be used when ShowCheckBox == false
this.validTime = value;
}
}
}
[
Browsable(false),
EditorBrowsable(EditorBrowsableState.Never)
]
new public event EventHandler Click {
add { base.Click += value; }
remove { base.Click -= value; }
}
protected override CreateParams CreateParams {
[SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.UnmanagedCode)]
get {
CreateParams cp = base.CreateParams;
cp.ClassName = NativeMethods.WC_DATETIMEPICK;
cp.Style |= style;
switch (format) {
case DateTimePickerFormat.Long:
cp.Style |= NativeMethods.DTS_LONGDATEFORMAT;
break;
case DateTimePickerFormat.Short:
break;
case DateTimePickerFormat.Time:
cp.Style |= TIMEFORMAT_NOUPDOWN;
break;
case DateTimePickerFormat.Custom:
break;
}
cp.ExStyle |= NativeMethods.WS_EX_CLIENTEDGE;
if (RightToLeft == RightToLeft.Yes && RightToLeftLayout == true) {
//We want to turn on mirroring for DateTimePicker explicitly.
cp.ExStyle |= NativeMethods.WS_EX_LAYOUTRTL;
//Don't need these styles when mirroring is turned on.
cp.ExStyle &= ~(NativeMethods.WS_EX_RTLREADING | NativeMethods.WS_EX_RIGHT | NativeMethods.WS_EX_LEFTSCROLLBAR);
}
return cp;
}
}
[
DefaultValue(null),
Localizable(true),
RefreshProperties(RefreshProperties.Repaint),
SRCategory(SR.CatBehavior),
SRDescription(SR.DateTimePickerCustomFormatDescr)
]
public string CustomFormat {
get {
return customFormat;
}
set {
if ((value != null && !value.Equals(customFormat)) ||
(value == null && customFormat != null)) {
customFormat = value;
if (IsHandleCreated) {
if (format == DateTimePickerFormat.Custom)
SendMessage(NativeMethods.DTM_SETFORMAT, 0, customFormat);
}
}
}
}
protected override Size DefaultSize {
get {
return new Size(200, PreferredHeight);
}
}
[EditorBrowsable(EditorBrowsableState.Never)]
protected override bool DoubleBuffered {
get {
return base.DoubleBuffered;
}
set {
base.DoubleBuffered = value;
}
}
[
Browsable(false),
EditorBrowsable(EditorBrowsableState.Never)
]
new public event EventHandler DoubleClick {
add { base.DoubleClick += value; }
remove { base.DoubleClick -= value; }
}
[
DefaultValue(LeftRightAlignment.Left),
SRCategory(SR.CatAppearance),
Localizable(true),
SRDescription(SR.DateTimePickerDropDownAlignDescr)
]
public LeftRightAlignment DropDownAlign {
get {
return((style & NativeMethods.DTS_RIGHTALIGN) != 0)
? LeftRightAlignment.Right
: LeftRightAlignment.Left;
}
set {
//valid values are 0x0 to 0x1
if (!ClientUtils.IsEnumValid(value, (int)value, (int)LeftRightAlignment.Left, (int)LeftRightAlignment.Right)){
throw new InvalidEnumArgumentException("value", (int)value, typeof(LeftRightAlignment));
}
SetStyleBit((value == LeftRightAlignment.Right), NativeMethods.DTS_RIGHTALIGN);
}
}
[Browsable(false), EditorBrowsable(EditorBrowsableState.Never)]
public override Color ForeColor {
get {
if (ShouldSerializeForeColor()) {
return base.ForeColor;
}
else {
return SystemColors.WindowText;
}
}
set {
base.ForeColor = value;
}
}
[Browsable(false), EditorBrowsable(EditorBrowsableState.Never)]
new public event EventHandler ForeColorChanged {
add {
base.ForeColorChanged += value;
}
remove {
base.ForeColorChanged -= value;
}
}
[
SRCategory(SR.CatAppearance),
RefreshProperties(RefreshProperties.Repaint),
SRDescription(SR.DateTimePickerFormatDescr)
]
public DateTimePickerFormat Format {
get {
return format;
}
set {
//valid values are 0x1, 0x2,0x4,0x8. max number of bits on at a time is 1
if (!ClientUtils.IsEnumValid(value, (int)value, (int)DateTimePickerFormat.Long, (int)DateTimePickerFormat.Custom, /*maxNumberOfBitsOn*/1))
{
throw new InvalidEnumArgumentException("value", (int)value, typeof(DateTimePickerFormat));
}
if (format != value) {
format = value;
RecreateHandle();
OnFormatChanged(EventArgs.Empty);
}
}
}
[SRCategory(SR.CatPropertyChanged), SRDescription(SR.DateTimePickerOnFormatChangedDescr)]
public event EventHandler FormatChanged {
add {
Events.AddHandler(EVENT_FORMATCHANGED, value);
}
remove {
Events.RemoveHandler(EVENT_FORMATCHANGED, value);
}
}
[Browsable(false), EditorBrowsable(EditorBrowsableState.Never)]
public new event PaintEventHandler Paint {
add {
base.Paint += value;
}
remove {
base.Paint -= value;
}
}
static internal DateTime EffectiveMinDate(DateTime minDate)
{
DateTime minSupportedDate = DateTimePicker.MinimumDateTime;
if (minDate < minSupportedDate)
{
return minSupportedDate;
}
return minDate;
}
static internal DateTime EffectiveMaxDate(DateTime maxDate)
{
DateTime maxSupportedDate = DateTimePicker.MaximumDateTime;
if (maxDate > maxSupportedDate)
{
return maxSupportedDate;
}
return maxDate;
}
[
SRCategory(SR.CatBehavior),
SRDescription(SR.DateTimePickerMaxDateDescr)
]
public DateTime MaxDate {
get {
return EffectiveMaxDate(max);
}
set {
if (value != max) {
if (value < EffectiveMinDate(min))
{
throw new ArgumentOutOfRangeException("MaxDate", SR.GetString(SR.InvalidLowBoundArgumentEx, "MaxDate", FormatDateTime(value), "MinDate"));
}
// If trying to set the maximum greater than MaxDateTime, throw.
if (value > MaximumDateTime) {
throw new ArgumentOutOfRangeException("MaxDate", SR.GetString(SR.DateTimePickerMaxDate, FormatDateTime(DateTimePicker.MaxDateTime)));
}
max = value;
SetRange();
//If Value (which was once valid) is suddenly greater than the max (since we just set it)
//then adjust this...
if (Value > max) {
Value = max;
}
}
}
}
public static DateTime MaximumDateTime {
get {
DateTime maxSupportedDateTime = CultureInfo.CurrentCulture.Calendar.MaxSupportedDateTime;
if (maxSupportedDateTime.Year > MaxDateTime.Year)
{
return MaxDateTime;
}
return maxSupportedDateTime;
}
}
[
SRCategory(SR.CatBehavior),
SRDescription(SR.DateTimePickerMinDateDescr)
]
public DateTime MinDate {
get {
return EffectiveMinDate(min);
}
set
{
if (value != min)
{
if (value > EffectiveMaxDate(max))
{
throw new ArgumentOutOfRangeException("MinDate", SR.GetString(SR.InvalidHighBoundArgument, "MinDate", FormatDateTime(value), "MaxDate"));
}
// If trying to set the minimum less than MinimumDateTime, throw.
if (value < MinimumDateTime)
{
throw new ArgumentOutOfRangeException("MinDate", SR.GetString(SR.DateTimePickerMinDate, FormatDateTime(DateTimePicker.MinimumDateTime)));
}
min = value;
SetRange();
//If Value (which was once valid) is suddenly less than the min (since we just set it)
//then adjust this...
if (Value < min)
{
Value = min;
}
}
}
}
public static DateTime MinimumDateTime {
get {
DateTime minSupportedDateTime = CultureInfo.CurrentCulture.Calendar.MinSupportedDateTime;
if (minSupportedDateTime.Year < 1753)
{
return new DateTime(1753, 1, 1);
}
return minSupportedDateTime;
}
}
[
Browsable(false),
EditorBrowsable(EditorBrowsableState.Never)
]
new public event MouseEventHandler MouseClick {
add { base.MouseClick += value; }
remove { base.MouseClick -= value; }
}
[
Browsable(false),
EditorBrowsable(EditorBrowsableState.Never)
]
new public event MouseEventHandler MouseDoubleClick {
add { base.MouseDoubleClick += value; }
remove { base.MouseDoubleClick -= value; }
}
[
Browsable(false),
EditorBrowsable(EditorBrowsableState.Never),
DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden)
]
public new Padding Padding {
get { return base.Padding; }
set { base.Padding = value;}
}
[
Browsable(false),
EditorBrowsable(EditorBrowsableState.Never)
]
public new event EventHandler PaddingChanged {
add { base.PaddingChanged += value; }
remove { base.PaddingChanged -= value; }
}
[
Browsable(false),
DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden),
]
public int PreferredHeight {
get {
if (prefHeightCache > -1)
return(int)prefHeightCache;
// Base the preferred height on the current font
int height = FontHeight;
// Adjust for the border
height += SystemInformation.BorderSize.Height * 4 + 3;
prefHeightCache = (short)height;
return height;
}
}
[
SRCategory(SR.CatAppearance),
Localizable(true),
DefaultValue(false),
SRDescription(SR.ControlRightToLeftLayoutDescr)
]
public virtual bool RightToLeftLayout {
get {
return rightToLeftLayout;
}
set {
if (value != rightToLeftLayout) {
rightToLeftLayout = value;
using(new LayoutTransaction(this, this, PropertyNames.RightToLeftLayout)) {
OnRightToLeftLayoutChanged(EventArgs.Empty);
}
}
}
}
[
DefaultValue(false),
SRCategory(SR.CatAppearance),
SRDescription(SR.DateTimePickerShowNoneDescr)
]
public bool ShowCheckBox {
get {
return(style & NativeMethods.DTS_SHOWNONE) != 0;
}
set {
SetStyleBit(value, NativeMethods.DTS_SHOWNONE);
}
}
[
DefaultValue(false),
SRCategory(SR.CatAppearance),
SRDescription(SR.DateTimePickerShowUpDownDescr)
]
public bool ShowUpDown {
get {
return(style & NativeMethods.DTS_UPDOWN) != 0;
}
set {
if (ShowUpDown != value) {
SetStyleBit(value, NativeMethods.DTS_UPDOWN);
}
}
}
[Browsable(false), EditorBrowsable(EditorBrowsableState.Advanced), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden)]
public override string Text {
get {
return base.Text;
}
set {
// Clause to check length
//
if (value == null || value.Length == 0) {
ResetValue();
}
else {
Value = DateTime.Parse(value, CultureInfo.CurrentCulture);
}
}
}
[Browsable(false), EditorBrowsable(EditorBrowsableState.Advanced)]
new public event EventHandler TextChanged {
add {
base.TextChanged += value;
}
remove {
base.TextChanged -= value;
}
}
[
SRCategory(SR.CatBehavior),
Bindable(true),
RefreshProperties(RefreshProperties.All),
SRDescription(SR.DateTimePickerValueDescr)
]
public DateTime Value {
get {
//checkbox clicked, no value set - no value set state should never occur, but just in case
if (!userHasSetValue && validTime)
return creationTime;
else
return value;
}
set {
bool valueChanged = !DateTime.Equals(this.Value, value);
if (!userHasSetValue || valueChanged) {
if ((value < MinDate) || (value > MaxDate)) {
throw new ArgumentOutOfRangeException("Value", SR.GetString(SR.InvalidBoundArgument, "Value", FormatDateTime(value), "'MinDate'", "'MaxDate'"));
}
string oldText = this.Text;
this.value = value;
userHasSetValue = true;
if (IsHandleCreated) {
/*
* Make sure any changes to this code
* get propagated to createHandle
*/
int gdt = NativeMethods.GDT_VALID;
NativeMethods.SYSTEMTIME sys = DateTimePicker.DateTimeToSysTime(value);
UnsafeNativeMethods.SendMessage(new HandleRef(this, Handle), NativeMethods.DTM_SETSYSTEMTIME, gdt, sys);
}
if (valueChanged) {
OnValueChanged(EventArgs.Empty);
}
if (!oldText.Equals(this.Text)) {
OnTextChanged(EventArgs.Empty);
}
}
}
}
[SRCategory(SR.CatAction), SRDescription(SR.DateTimePickerOnCloseUpDescr)]
public event EventHandler CloseUp {
add {
onCloseUp += value;
}
remove {
onCloseUp -= value;
}
}
[SRCategory(SR.CatPropertyChanged), SRDescription(SR.ControlOnRightToLeftLayoutChangedDescr)]
public event EventHandler RightToLeftLayoutChanged {
add {
onRightToLeftLayoutChanged += value;
}
remove {
onRightToLeftLayoutChanged -= value;
}
}
[SRCategory(SR.CatAction), SRDescription(SR.valueChangedEventDescr)]
public event EventHandler ValueChanged {
add {
onValueChanged += value;
}
remove {
onValueChanged -= value;
}
}
[SRCategory(SR.CatAction), SRDescription(SR.DateTimePickerOnDropDownDescr)]
public event EventHandler DropDown {
add {
onDropDown += value;
}
remove {
onDropDown -= value;
}
}
protected override AccessibleObject CreateAccessibilityInstance() {
return new DateTimePickerAccessibleObject(this);
}
protected override void CreateHandle() {
if (!RecreatingHandle) {
IntPtr userCookie = UnsafeNativeMethods.ThemingScope.Activate();
try {
NativeMethods.INITCOMMONCONTROLSEX icc = new NativeMethods.INITCOMMONCONTROLSEX();
icc.dwICC = NativeMethods.ICC_DATE_CLASSES;
SafeNativeMethods.InitCommonControlsEx(icc);
}
finally {
UnsafeNativeMethods.ThemingScope.Deactivate(userCookie);
}
}
creationTime = DateTime.Now;
base.CreateHandle();
if (userHasSetValue && validTime) {
/*
* Make sure any changes to this code
* get propagated to setValue
*/
int gdt = NativeMethods.GDT_VALID;
NativeMethods.SYSTEMTIME sys = DateTimePicker.DateTimeToSysTime(Value);
UnsafeNativeMethods.SendMessage(new HandleRef(this, Handle), NativeMethods.DTM_SETSYSTEMTIME, gdt, sys);
}
else if (!validTime) {
int gdt = NativeMethods.GDT_NONE;
NativeMethods.SYSTEMTIME sys = null;
UnsafeNativeMethods.SendMessage(new HandleRef(this, Handle), NativeMethods.DTM_SETSYSTEMTIME, gdt, sys);
}
if (format == DateTimePickerFormat.Custom) {
SendMessage(NativeMethods.DTM_SETFORMAT, 0, customFormat);
}
UpdateUpDown();
SetAllControlColors();
SetControlCalendarFont();
SetRange();
}
[UIPermission(SecurityAction.LinkDemand, Window=UIPermissionWindow.AllWindows)]
protected override void DestroyHandle() {
value = Value;
base.DestroyHandle();
}
// Return a localized string representation of the given DateTime value.
// Used for throwing exceptions, etc.
//
private static string FormatDateTime(DateTime value) {
return value.ToString("G", CultureInfo.CurrentCulture);
}
// GetPreferredSize and SetBoundsCore call this method to allow controls to self impose
// constraints on their size.
internal override Rectangle ApplyBoundsConstraints(int suggestedX, int suggestedY, int proposedWidth, int proposedHeight) {
// Lock DateTimePicker to its preferred height.
return base.ApplyBoundsConstraints(suggestedX,suggestedY, proposedWidth, PreferredHeight);
}
internal override Size GetPreferredSizeCore(Size proposedConstraints) {
int height = PreferredHeight;
int width = CommonProperties.GetSpecifiedBounds(this).Width;
return new Size(width, height);
}
protected override bool IsInputKey(Keys keyData) {
if ((keyData & Keys.Alt) == Keys.Alt) return false;
switch (keyData & Keys.KeyCode) {
case Keys.PageUp:
case Keys.PageDown:
case Keys.Home:
case Keys.End:
return true;
}
return base.IsInputKey(keyData);
}
protected virtual void OnCloseUp(EventArgs eventargs) {
if (onCloseUp != null) onCloseUp(this, eventargs);
}
protected virtual void OnDropDown(EventArgs eventargs) {
if (onDropDown != null) {
onDropDown(this, eventargs);
}
}
protected virtual void OnFormatChanged(EventArgs e) {
EventHandler eh = Events[EVENT_FORMATCHANGED] as EventHandler;
if (eh != null) {
eh(this, e);
}
}
protected override void OnHandleCreated(EventArgs e)
{
base.OnHandleCreated(e);
SystemEvents.UserPreferenceChanged += new UserPreferenceChangedEventHandler(this.MarshaledUserPreferenceChanged);
}
protected override void OnHandleDestroyed(EventArgs e)
{
SystemEvents.UserPreferenceChanged -= new UserPreferenceChangedEventHandler(this.MarshaledUserPreferenceChanged);
base.OnHandleDestroyed(e);
}
protected virtual void OnValueChanged(EventArgs eventargs) {
if (onValueChanged != null) {
onValueChanged(this, eventargs);
}
}
[EditorBrowsable(EditorBrowsableState.Advanced)]
protected virtual void OnRightToLeftLayoutChanged(EventArgs e) {
if (GetAnyDisposingInHierarchy()) {
return;
}
if (RightToLeft == RightToLeft.Yes) {
RecreateHandle();
}
if (onRightToLeftLayoutChanged != null) {
onRightToLeftLayoutChanged(this, e);
}
}
protected override void OnFontChanged(EventArgs e) {
base.OnFontChanged(e);
//clear the pref height cache
prefHeightCache = -1;
Height = PreferredHeight;
if (calendarFont == null) {
calendarFontHandleWrapper = null;
SetControlCalendarFont();
}
}
private void ResetCalendarForeColor() {
CalendarForeColor = DefaultForeColor;
}
private void ResetCalendarFont() {
CalendarFont = null;
}
private void ResetCalendarMonthBackground() {
CalendarMonthBackground = DefaultMonthBackColor;
}
private void ResetCalendarTitleBackColor() {
CalendarTitleBackColor = DefaultTitleBackColor;
}
private void ResetCalendarTitleForeColor() {
CalendarTitleBackColor = DefaultForeColor;
}
private void ResetCalendarTrailingForeColor() {
CalendarTrailingForeColor = DefaultTrailingForeColor;
}
private void ResetFormat() {
Format = DateTimePickerFormat.Long;
}
private void ResetMaxDate() {
MaxDate = MaximumDateTime;
}
private void ResetMinDate() {
MinDate = MinimumDateTime;
}
private void ResetValue() {
// always update on reset with ShowNone = false -- as it'll take the current time.
this.value = DateTime.Now;
userHasSetValue = false;
// Update the text displayed in the DateTimePicker
if (IsHandleCreated) {
int gdt = NativeMethods.GDT_VALID;
NativeMethods.SYSTEMTIME sys = DateTimePicker.DateTimeToSysTime(value);
UnsafeNativeMethods.SendMessage(new HandleRef(this, Handle), NativeMethods.DTM_SETSYSTEMTIME, gdt, sys);
}
// Updating Checked to false will set the control to "no date",
// and clear its checkbox.
Checked = false;
OnValueChanged(EventArgs.Empty);
OnTextChanged(EventArgs.Empty);
}
private void SetControlColor(int colorIndex, Color value) {
if (IsHandleCreated) {
SendMessage(NativeMethods.DTM_SETMCCOLOR, colorIndex, ColorTranslator.ToWin32(value));
}
}
private void SetControlCalendarFont() {
if (IsHandleCreated) {
SendMessage(NativeMethods.DTM_SETMCFONT, CalendarFontHandle, NativeMethods.InvalidIntPtr);
}
}
private void SetAllControlColors() {
SetControlColor(NativeMethods.MCSC_MONTHBK, calendarMonthBackground);
SetControlColor(NativeMethods.MCSC_TEXT, calendarForeColor);
SetControlColor(NativeMethods.MCSC_TITLEBK, calendarTitleBackColor);
SetControlColor(NativeMethods.MCSC_TITLETEXT, calendarTitleForeColor);
SetControlColor(NativeMethods.MCSC_TRAILINGTEXT, calendarTrailingText);
}
private void SetRange() {
SetRange(EffectiveMinDate(min), EffectiveMaxDate(max));
}
private void SetRange(DateTime min, DateTime max) {
if (IsHandleCreated) {
int flags = 0;
NativeMethods.SYSTEMTIMEARRAY sa = new NativeMethods.SYSTEMTIMEARRAY();
flags |= NativeMethods.GDTR_MIN | NativeMethods.GDTR_MAX;
NativeMethods.SYSTEMTIME sys = DateTimePicker.DateTimeToSysTime(min);
sa.wYear1 = sys.wYear;
sa.wMonth1 = sys.wMonth;
sa.wDayOfWeek1 = sys.wDayOfWeek;
sa.wDay1 = sys.wDay;
sa.wHour1 = sys.wHour;
sa.wMinute1 = sys.wMinute;
sa.wSecond1 = sys.wSecond;
sa.wMilliseconds1 = sys.wMilliseconds;
sys = DateTimePicker.DateTimeToSysTime(max);
sa.wYear2 = sys.wYear;
sa.wMonth2 = sys.wMonth;
sa.wDayOfWeek2 = sys.wDayOfWeek;
sa.wDay2 = sys.wDay;
sa.wHour2 = sys.wHour;
sa.wMinute2 = sys.wMinute;
sa.wSecond2 = sys.wSecond;
sa.wMilliseconds2 = sys.wMilliseconds;
UnsafeNativeMethods.SendMessage(new HandleRef(this, Handle), NativeMethods.DTM_SETRANGE, flags, sa);
}
}
private void SetStyleBit(bool flag, int bit) {
if (((style & bit) != 0) == flag) return;
if (flag) {
style |= bit;
}
else {
style &= ~bit;
}
if (IsHandleCreated) {
RecreateHandle();
Invalidate();
Update();
}
}
private bool ShouldSerializeCalendarForeColor() {
return !CalendarForeColor.Equals(DefaultForeColor);
}
private bool ShouldSerializeCalendarFont() {
return calendarFont != null;
}
private bool ShouldSerializeCalendarTitleBackColor() {
return !calendarTitleBackColor.Equals(DefaultTitleBackColor);
}
private bool ShouldSerializeCalendarTitleForeColor() {
return !calendarTitleForeColor.Equals(DefaultTitleForeColor);
}
private bool ShouldSerializeCalendarTrailingForeColor() {
return !calendarTrailingText.Equals(DefaultTrailingForeColor);
}
private bool ShouldSerializeCalendarMonthBackground() {
return !calendarMonthBackground.Equals(DefaultMonthBackColor);
}
private bool ShouldSerializeMaxDate() {
return max != MaximumDateTime && max != DateTime.MaxValue;
}
private bool ShouldSerializeMinDate() {
return min != MinimumDateTime && min != DateTime.MinValue;
}
private bool ShouldSerializeValue() {
return userHasSetValue;
}
private bool ShouldSerializeFormat() {
return(Format != DateTimePickerFormat.Long);
}
public override string ToString() {
string s = base.ToString();
return s + ", Value: " + FormatDateTime(Value);
}
private void UpdateUpDown() {
// The upDown control doesn't repaint correctly.
//
if (ShowUpDown) {
EnumChildren c = new EnumChildren();
NativeMethods.EnumChildrenCallback cb = new NativeMethods.EnumChildrenCallback(c.enumChildren);
UnsafeNativeMethods.EnumChildWindows(new HandleRef(this, Handle), cb, NativeMethods.NullHandleRef);
if (c.hwndFound != IntPtr.Zero) {
SafeNativeMethods.InvalidateRect(new HandleRef(c, c.hwndFound), null, true);
SafeNativeMethods.UpdateWindow(new HandleRef(c, c.hwndFound));
}
}
}
private void MarshaledUserPreferenceChanged(object sender, UserPreferenceChangedEventArgs pref) {
try {
//use begininvoke instead of invoke in case the destination thread is not processing messages.
BeginInvoke(new UserPreferenceChangedEventHandler(this.UserPreferenceChanged), new object[] { sender, pref });
}
catch (InvalidOperationException) { } //if the destination thread does not exist, don't send.
}
private void UserPreferenceChanged(object sender, UserPreferenceChangedEventArgs pref) {
if (pref.Category == UserPreferenceCategory.Locale) {
// We need to recreate the monthcalendar handle when the locale changes, because
// the day names etc. are only updated on a handle recreate (comctl32 limitation).
//
RecreateHandle();
}
}
private void WmCloseUp(ref Message m) {
OnCloseUp(EventArgs.Empty);
}
private void WmDateTimeChange(ref Message m) {
NativeMethods.NMDATETIMECHANGE nmdtc = (NativeMethods.NMDATETIMECHANGE)m.GetLParam(typeof(NativeMethods.NMDATETIMECHANGE));
DateTime temp = value;
bool oldvalid = validTime;
if (nmdtc.dwFlags != NativeMethods.GDT_NONE) {
validTime = true;
value = DateTimePicker.SysTimeToDateTime(nmdtc.st);
userHasSetValue = true;
}
else {
validTime = false;
}
if (value!=temp || oldvalid != validTime) {
OnValueChanged(EventArgs.Empty);
OnTextChanged(EventArgs.Empty);
}
}
private void WmDropDown(ref Message m) {
if (this.RightToLeftLayout == true && this.RightToLeft == RightToLeft.Yes) {
IntPtr handle = SendMessage(NativeMethods.DTM_GETMONTHCAL, 0,0);
if (handle != IntPtr.Zero) {
int style = unchecked((int)((long)UnsafeNativeMethods.GetWindowLong(new HandleRef(this, handle), NativeMethods.GWL_EXSTYLE)));
style |= NativeMethods.WS_EX_LAYOUTRTL | NativeMethods.WS_EX_NOINHERITLAYOUT;
style &= ~(NativeMethods.WS_EX_RIGHT | NativeMethods.WS_EX_RTLREADING);
UnsafeNativeMethods.SetWindowLong(new HandleRef(this, handle), NativeMethods.GWL_EXSTYLE, new HandleRef(this, (IntPtr)style));
}
}
OnDropDown(EventArgs.Empty);
}
protected override void OnSystemColorsChanged(EventArgs e) {
SetAllControlColors();
base.OnSystemColorsChanged(e);
}
private void WmReflectCommand(ref Message m) {
if (m.HWnd == Handle) {
NativeMethods.NMHDR nmhdr = (NativeMethods.NMHDR)m.GetLParam(typeof(NativeMethods.NMHDR));
switch (nmhdr.code) {
case NativeMethods.DTN_CLOSEUP:
WmCloseUp(ref m);
break;
case NativeMethods.DTN_DATETIMECHANGE:
WmDateTimeChange(ref m);
break;
case NativeMethods.DTN_DROPDOWN:
WmDropDown(ref m);
break;
}
}
}
[SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.UnmanagedCode)]
protected override void WndProc(ref Message m) {
switch (m.Msg) {
case NativeMethods.WM_LBUTTONDOWN:
FocusInternal();
if (!ValidationCancelled) {
base.WndProc(ref m);
}
break;
case NativeMethods.WM_REFLECT + NativeMethods.WM_NOTIFY:
WmReflectCommand(ref m);
base.WndProc(ref m);
break;
case NativeMethods.WM_WINDOWPOSCHANGED:
base.WndProc(ref m);
UpdateUpDown();
break;
default:
base.WndProc(ref m);
break;
}
}
internal static NativeMethods.SYSTEMTIME DateTimeToSysTime(DateTime time) {
NativeMethods.SYSTEMTIME sys = new NativeMethods.SYSTEMTIME();
sys.wYear = (short)time.Year;
sys.wMonth = (short)time.Month;
sys.wDayOfWeek = (short)time.DayOfWeek;
sys.wDay = (short)time.Day;
sys.wHour = (short)time.Hour;
sys.wMinute = (short)time.Minute;
sys.wSecond = (short)time.Second;
sys.wMilliseconds = 0;
return sys;
}
internal static DateTime SysTimeToDateTime(NativeMethods.SYSTEMTIME s) {
return new DateTime(s.wYear, s.wMonth, s.wDay, s.wHour, s.wMinute, s.wSecond);
}
/// <devdoc>
/// </devdoc>
private sealed class EnumChildren {
public IntPtr hwndFound = IntPtr.Zero;
public bool enumChildren(IntPtr hwnd, IntPtr lparam) {
hwndFound = hwnd;
return true;
}
}
[ComVisible(true)]
public class DateTimePickerAccessibleObject : ControlAccessibleObject {
public DateTimePickerAccessibleObject(DateTimePicker owner) : base(owner) {
}
public override string KeyboardShortcut {
get {
Label previousLabel = this.PreviousLabel;
if (previousLabel != null) {
char previousLabelMnemonic = WindowsFormsUtils.GetMnemonic(previousLabel.Text, false /*convertToUpperCase*/);
if (previousLabelMnemonic != (char) 0) {
return "Alt+" + previousLabelMnemonic;
}
}
string baseShortcut = base.KeyboardShortcut;
if ((baseShortcut == null || baseShortcut.Length == 0)) {
char ownerTextMnemonic = WindowsFormsUtils.GetMnemonic(this.Owner.Text, false /*convertToUpperCase*/);
if (ownerTextMnemonic != (char) 0) {
return "Alt+" + ownerTextMnemonic;
}
}
return baseShortcut;
}
}
public override string Value {
[SecurityPermission(SecurityAction.Demand, Flags = SecurityPermissionFlag.UnmanagedCode)]
get {
string baseValue = base.Value;
if (baseValue == null || baseValue.Length == 0) {
return Owner.Text;
}
return baseValue;
}
}
public override AccessibleStates State {
get {
AccessibleStates state = base.State;
if(((DateTimePicker)Owner).ShowCheckBox &&
((DateTimePicker)Owner).Checked) {
state |= AccessibleStates.Checked;
}
return state;
}
}
public override AccessibleRole Role {
get {
AccessibleRole role = Owner.AccessibleRole;
if (role != AccessibleRole.Default) {
return role;
}
return AccessibleRole.DropList;
}
}
}
}
|
 |
 |
 |
 |
Date :
2021-01-26 16:33:52 |
By :
lamaka.tor |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
usb drive 2TB 555555 อยากเก็บอะไรก็เก็บเข้าไป
|
 |
 |
 |
 |
Date :
2021-01-26 16:50:52 |
By :
Chaidhanan |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
DataGridViewDatetimepickerColumn ตอนเรียกใข้นำไปใช้ยังไงครับ
|
 |
 |
 |
 |
Date :
2021-01-26 17:34:19 |
By :
lakornworld |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
จากที่ลองสร้างโปรเจค แล้ว ก๊อบไฟล์มาลองแก้ดู
สรุปได้ว่าเป็นที่โค้ดใน DataGridViewDatetimepickerColumn ครับ
โดย ตัว Datetimepicker เวลาคลิก สามารถ แก้ เวลาได้ปกติ
แต่ Datetimepicker ใน DataGridViewDatetimepickerColumn เวลาคลิกจะเป็นรูปแบบวันที่
ตามนี้ครับ
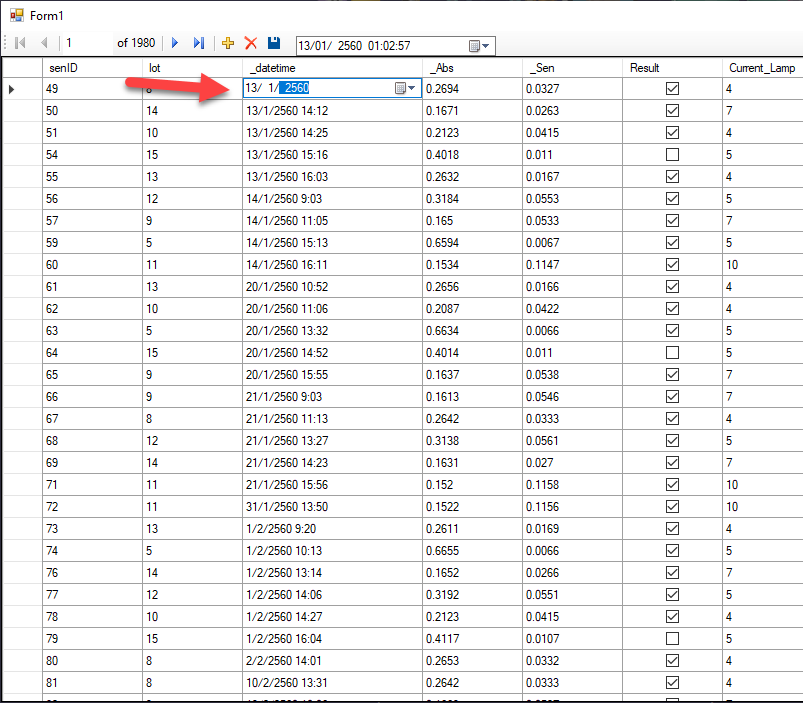
น่าจะอยู่ใน event click หรือ อะไรซักอย่างของ DataGridViewDatetimepickerColumn
จะลองเช็คดูอีกทีครับ
|
 |
 |
 |
 |
Date :
2021-01-26 18:08:07 |
By :
lamaka.tor |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
มีปัญหาใน cell
เอา constructor จากบรรพบุรุษมาด้วย
Code (C#)
public DataGridViewDatetimepickerCell()
: base()
{
this.Style.Format = "d"; // ให้แสดงข้อมูลแบบไหนก็จัดไป
}
|
ประวัติการแก้ไข 2021-01-26 21:13:26
 |
 |
 |
 |
Date :
2021-01-26 21:12:04 |
By :
lakornworld |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ผมคนคนเดียวทำทุกอย่าง บางทีเมียบอกว่า มาถอนขนจั๊กแร้ให้หน่อยสิ
--- รอแป๊บหนึ่งนะ ล้างจานไกล้เสร็จแล้ว
มันก็มีความสุขเหมือนกันนะ (บ่อยครั้งก็ถามเมียว่า วันนี้ไม่ถอนเหรอ เป็นงั้นไปเสียอีก)
มันกลายเป็นความเคยชิน ถ้าไม่ได้ทำมันเหมือนขาดอะไรไป
งานโปรแกรมมิ่งก็เหมือนกัน ผมก็พูดตรงฯ ไม่ได้สนใจว่า คนที่อยู่ข้างหน้าเป็นใคร
--- อยากได้แบบนี้ ผมย้อนแย้งถ้าทำแบบนี้แล้ว ผัวจะรักผัวจะหลง ใช่มั้ย ผมยิงคำถามไปตรงฯแบบนี้
--- etc...
|
 |
 |
 |
 |
Date :
2021-01-27 09:41:04 |
By :
ผ่านมา |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
เป็นข้อมูลทดสอบ (จริงฯต้องเป็นข้อมูลจริง มากหรือน้อยก็ดีทั้งนั้นแหละ)
--- เพื่อเห็นภาพได้ชัดเจน (ผมไม่มีเวลาทำ)
ละเอียดรอบคอบ
--- จัดวางข้อความ อะไรควรอยู่ชิดซ้าย/ชิดขวา/กึ่งกลาง
--- มีหน้าเดียว แสดง Paging ทำไม?
--- หาที่ ติเตียน ได้ไม่มีวันหมด
ผมไม่ได้สนใจ วันที่-เวลา มากจนเกินไป (ถ้าจะทำ เอาหอไอเฟลมาใส่ยังได้เลย)
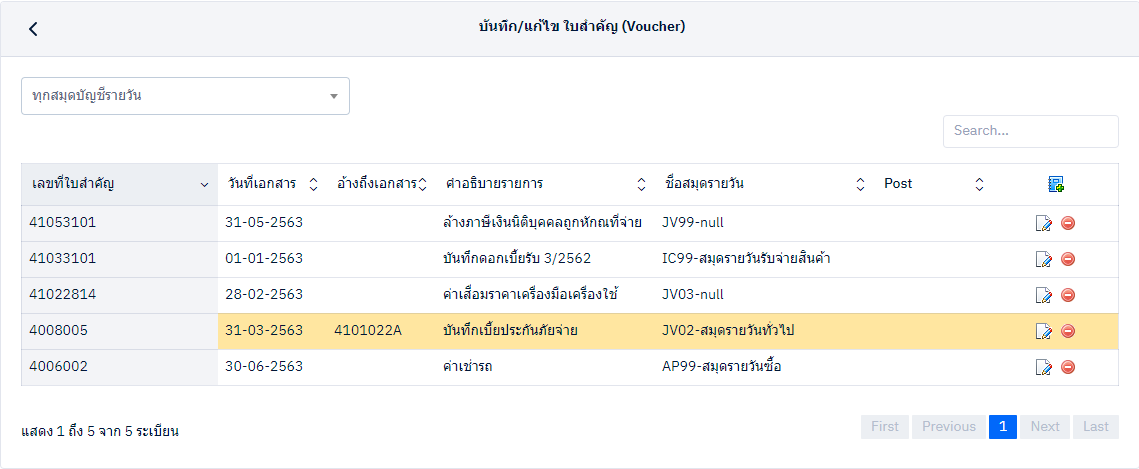
|
 |
 |
 |
 |
Date :
2021-01-28 10:18:29 |
By :
ผ่านมา |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
อยากถอดออกอีกเยอะเลย (ไม่ใช่ว่าไม่ดี แต่บางอย่างผมใช้นิดเดียวเอง) เช่น
1. Boostrap 4.3.x มันเยี่ยมยอด
--- แต่ผมเห็นคุณ Chaidhanan บอกว่าใช้ CSS-Grid ดีกว่า (ผมเห็นด้วยเป็นอย่างยิ่งยวด)
2. moment.js
--- มันยอดเยี่ยม แต่ผมก็ใช้นิดเดียว อาจเปลี่ยนไปใช้ Day.js แทน
คิดเอาไว้นะ ชาตินี้ไม่รู้จะได้ทำหรือเปล่ายังไม่แน่ใจเหมือนกัน
คิดเอาไว้อีกเยอะเลย คิดเยอะมาก บางทีก็ไม่ได้ทำในสิ่งที่คิดเลยสักเรื่องเดียวก็มีอยู่บ่อยฯ
|
 |
 |
 |
 |
Date :
2021-01-28 10:28:34 |
By :
ผ่านมา |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
Eric Clapton - "Wonderful Tonight" [Live Video Version]
Quote:https://www.youtube.com/watch?v=UprwkbzUX6g
วัน-เวลา เขียนเอง (เอาโน่นมาแปะเอานี่มาแปะ คล้ายฯผงผสมพระสมเด็จ)
ใช้ได้ระดับหนึ่งและเริ่มนิ่งแล้ว (นานฯเจอปัญหา ก็ปรับปรุงแก้ไขโดยไม่บ่นสักคำ)
siteCore.js
Code (JavaScript)
wls.Date.ValidateOnblur = function () {
$(".ZeroDate").each(function (index, value) {
$(this).on("blur", function () {
if (!wls.Date.isDate($(this).val()) && $(this).val().trim() != '') {
$(this).val('');
wls.HTML.focus($(this).attr("id")); // Fixed bug sometime can't focus.
}
});
});
}
wls.Date.isDate = function (strDate) { //function (strDate, datePattern = "d, m, Y") {
if (!strDate)
return false;
let spStr = wls.String.split(strDate, ["/", "-", ".", " "]); // ตัวคั่นวันที่ นอกเหนือจากนี้ก็ไม่ใช่คนแล้ว
//var yearValue = spStr.reduce((r, s) => r.length > s.length ? r : s, 0); //หาปีโดยการเปรียบเทียบความยาว
let spPattern = DATE_PATTERN.split(","); //datePattern.split(",");
let yPos = spPattern.indexOf("Y"); // บังคับให้ใช้ Y
let mPos = spPattern.indexOf("m"); // บังคับให้ใช้ m
let dPos = spPattern.indexOf("d"); // บังคับให้ใช้ d
if (spStr.length != 3 || (spStr[yPos].length != 4)) {
return false;
}
let yVal = parseInt(spStr[yPos]); //ปี
if (wls.globalVar.curLang() == "th") {
yVal -= 543;
}
let mVal = parseInt(spStr[mPos]); //เดือน
let dVal = parseInt(spStr[dPos]); //วัน
let tmpDate = new Date(yVal, mVal - 1, dVal); // Month is zero base slow as fuck javascript
let x_date = "" + tmpDate.getFullYear() + (tmpDate.getMonth() + 1) + tmpDate.getDate();
let y_date = "" + yVal + mVal + dVal;
return (x_date == y_date) ? true : false;
}
/**@description แปลงวันที่เพื่อจัดเก็บลงฐานข้อมูล
* @param {string} strDate วันที่ ไทย/อังกฤษ
*/
wls.Date.StringDate2DB = function (strDate) {
let spStr = wls.String.split(strDate, ["/", "-", ".", " "]); // ตัวคั่นวันที่ นอกเหนือจากนี้ก็ไม่ใช่คนแล้ว
//var yearValue = spStr.reduce((r, s) => r.length > s.length ? r : s, 0); //หาปีโดยการเปรียบเทียบความยาว
let spPattern = DATE_PATTERN.split(",");
let yPos = spPattern.indexOf("Y"); // บังคับให้ใช้ Y
let mPos = spPattern.indexOf("m"); // บังคับให้ใช้ m
let dPos = spPattern.indexOf("d"); // บังคับให้ใช้ d
if (spStr.length != 3 || (spStr[yPos].length != 4)) {
//return false;
return null; // fixed bug 2020-12-31
}
let yVal = parseInt(spStr[yPos]); //ปี
if (wls.globalVar.curLang() == "th") {
yVal -= 543;
}
let mVal = parseInt(spStr[mPos]); //เดือน
let dVal = parseInt(spStr[dPos]); //วัน
//เต็มศูนย์ให้ครบ 2 หลัก (เดือน/วัน)
return "" + yVal + "-" + ("0" + mVal).slice(-2) + "-" + ("0" + dVal).slice(-2); // yyyy-mm-dd วันที่ก็คือวันที่ ปี ค.ศ-เดือน-วัน
}
/**@description แปลงวันที่ให้แสดงตามความเป็นจริง ตามที่เลือกภาษาใช้งาน
* @param {string} strJsonDate วันที่ Json มาเต็มยศ 2019-05-19T00:00:00
*/
wls.Date.JsonDate2String = function (strJsonDate) { //function (strJsonDate, dp = "d, m, Y", dl = "-") {
if (!strJsonDate || strJsonDate == "0001-01-01T00:00:00") { // แก้ไข 30/12/2563
return "";
}
let srcDate = moment(strJsonDate, "YYYY-MM-DDTHH:mm:ss"); // ตรงนี้รับรู้ Time zone แล้ว
let curLang = wls.globalVar.curLang();
if (curLang == "th") {
srcDate = moment(srcDate).add(543, 'year'); //ถ้าเป็น พ.ศ. +543 ปี
}
let dps = DATE_PATTERN.split(",");
let dls = DATE_DELIMITER;
let ret = "";
for (var i = 0; i < dps.length; i++) {
switch (dps[i]) {
case "d":
ret += ("0" + srcDate.date()).slice(-2) + dls; //เติมศูนย์ให้ครบ สองหลัก ในกรณีวันที่ 1-9
break;
case "m":
ret += ("0" + (srcDate.month() + 1)).slice(-2) + dls; // เติมศูนย์ให้ครบสองหลักของเดือน 1-9 (Month start with 0 fuck JavaScript)
break;
case "y":
ret += ("" + srcDate.year()).substring(2, 2) + dls; //ปี 2 หลัก
break;
case "Y":
ret += "" + srcDate.year() + dls; //ปี 4 หลัก
break;
default:
break;
}
}
return (ret.endsWith(dls)) ? ret.substring(0, ret.length - 1) : ret;
// วัน-เดือน-ปี ตามจริง ไทยก็ไทย อังกฤษก็อังกฤษ ตามรูปแบบที่ตั้งเอาไว้ใน ค่าเริ่มต้นระบบ
}
|
 |
 |
 |
 |
Date :
2021-01-28 11:02:19 |
By :
ผ่านมา |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
Code (SQL)
if object_id('tempdb..#temp') is not null drop table #temp;
declare @recordstotal int;
declare @recordsfiltered int;
set @recordsfiltered = (select count(1) from jvh);
select * into #temp
from
(
select a.*, b.BDesc, b.BDesc2 from jvh a
left join book b on a.jvbook = b.bcode
--left join VIP_Class c on b.VIP_Class = c.VIP_Class
where a.pflag like '%%' or a.pflag is null
) as temp;
set @recordstotal = (select count(1) from #temp);
select * from #temp tempdata order by jvno desc offset 0 rows fetch next 10 rows only;
select @recordstotal as recordstotal, @recordsfiltered as recordsfiltered;
if object_id('tempdb..#temp') is not null drop table #temp;
|
 |
 |
 |
 |
Date :
2021-01-28 13:05:46 |
By :
ผ่านมา |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
มาอัพเดทการแก้ปัญหานี้ให้ทราบครับ
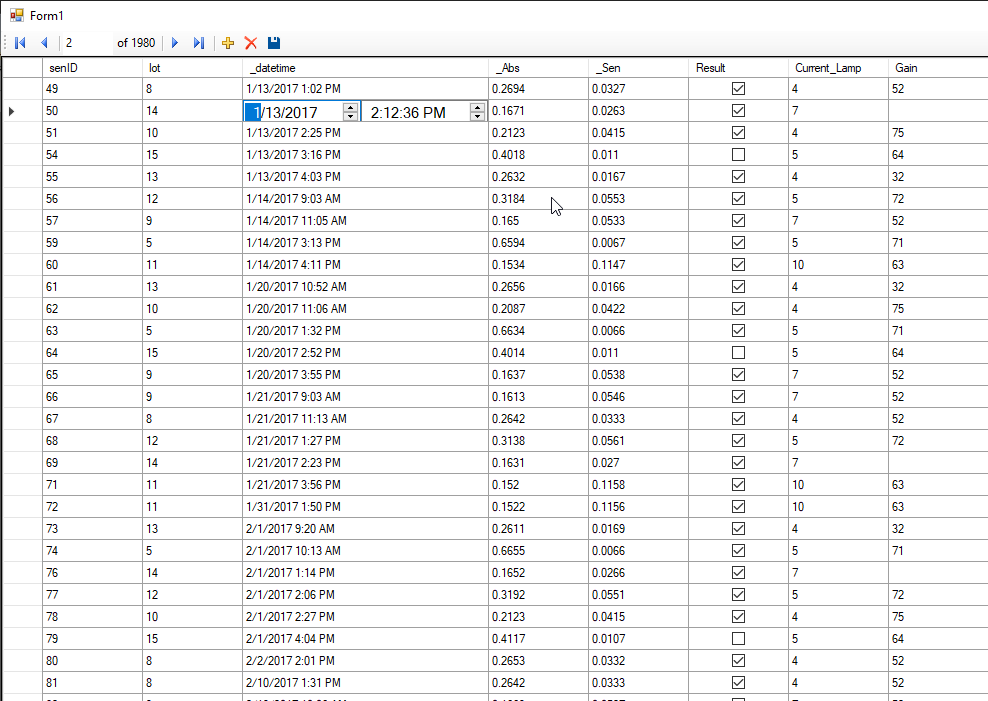
ในส่วนการใช้งาน ผมว่าน่าจะตอบโจทย์ คือ ตัววันที่ สามารถ เลือกวันที่ได้(หรือเลือกเลื่อนขึ้นลงได้)
และปรับค่าเวลาได้ แบบเลื่อนขึ้นลง
|
 |
 |
 |
 |
Date :
2021-01-29 22:03:23 |
By :
lamaka.tor |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ตอบความคิดเห็นที่ : 30 เขียนโดย : lakornworld เมื่อวันที่ 2021-01-29 22:09:46
รายละเอียดของการตอบ ::
ตอนนี้ก็กำลังหาโค้ดที่จะให้ตั้งค่า จาก DataGridViewDatetimeSelectColumn ให้แก้ไปถึง DataGridViewDatetimeSelectEdit ครับ
คือใจจริงจะปรับให้ตั้งค่า ShowUpDown ได้ด้วยครับ จะได้ เลือกได้
โค้ด DatetimeSelect
Code (C#)
public partial class DatetimeSelect : UserControl
{
private System.Windows.Forms.DateTimePicker dpkDate;
private System.Windows.Forms.DateTimePicker dpkTime;
/// <summary>
/// Required designer variable.
/// </summary>
private System.ComponentModel.IContainer components = null;
/// <summary>
/// Clean up any resources being used.
/// </summary>
/// <param name="disposing">true if managed resources should be disposed; otherwise, false.</param>
protected override void Dispose(bool disposing)
{
if (disposing && (components != null))
{
components.Dispose();
}
base.Dispose(disposing);
}
#region Component Designer generated code
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
private void InitializeComponent()
{
this.dpkDate = new System.Windows.Forms.DateTimePicker();
this.dpkTime = new System.Windows.Forms.DateTimePicker();
this.SuspendLayout();
//
// dpkDate
//
this.dpkDate.Dock = System.Windows.Forms.DockStyle.Fill;
this.dpkDate.Format = System.Windows.Forms.DateTimePickerFormat.Short;
this.dpkDate.Location = new System.Drawing.Point(0, 0);
this.dpkDate.Margin = new System.Windows.Forms.Padding(4);
this.dpkDate.Name = "dpkDate";
this.dpkDate.Size = new System.Drawing.Size(118, 24);
this.dpkDate.TabIndex = 0;
//
// dpkTime
//
this.dpkTime.Dock = System.Windows.Forms.DockStyle.Right;
this.dpkTime.Format = System.Windows.Forms.DateTimePickerFormat.Time;
this.dpkTime.Location = new System.Drawing.Point(118, 0);
this.dpkTime.Margin = new System.Windows.Forms.Padding(4);
this.dpkTime.Name = "dpkTime";
this.dpkTime.ShowUpDown = true;
this.dpkTime.Size = new System.Drawing.Size(119, 24);
this.dpkTime.TabIndex = 1;
//
// DatetimeSelect
//
this.AutoScaleDimensions = new System.Drawing.SizeF(9F, 18F);
this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font;
this.Controls.Add(this.dpkDate);
this.Controls.Add(this.dpkTime);
this.Font = new System.Drawing.Font("Microsoft Sans Serif", 11.25F, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, ((byte)(222)));
this.Margin = new System.Windows.Forms.Padding(4);
this.Name = "DatetimeSelect";
this.Size = new System.Drawing.Size(237, 29);
this.ResumeLayout(false);
}
#endregion
public DatetimeSelect()
{
InitializeComponent();
}
#region _Properties
private DateTime value = DateTime.Now;
[
Bindable(true),
RefreshProperties(RefreshProperties.All)
]
public DateTime Value
{
get
{
return value;
}
set
{
this.value = value;
dpkDate.Value = value;
dpkTime.Value = value;
}
}
[
DefaultValue(false)
]
public bool ShowUpDownDate
{
get
{
return dpkDate.ShowUpDown;
}
set
{
dpkDate.ShowUpDown = value;
}
}
#endregion
#region _Event
private EventHandler onValueChanged;
protected virtual void OnValueChanged(EventArgs eventargs)
{
if (onValueChanged != null)
{
onValueChanged(this, eventargs);
}
}
#endregion
}
Code (C#)
namespace TORServices.FormsTor
{
#region _DataGridViewDatetimeSelectColumn
public class DataGridViewDatetimeSelectColumn : System.Windows.Forms.DataGridViewColumn
{
public DataGridViewDatetimeSelectColumn()
: base(new DataGridViewDatetimeSelectCell())
{
}
}
public class DataGridViewDatetimeSelectCell : System.Windows.Forms.DataGridViewTextBoxCell
{
public DataGridViewDatetimeSelectCell()
:base()
{
}
public override void InitializeEditingControl(int rowIndex, object initialFormattedValue, System.Windows.Forms.DataGridViewCellStyle dataGridViewCellStyle)
{
// Set the value of the editing control to the current cell value.
base.InitializeEditingControl(rowIndex, initialFormattedValue, dataGridViewCellStyle);
DataGridViewDatetimeSelectEdit ctl = (DataGridViewDatetimeSelectEdit)DataGridView.EditingControl;
if ((!object.ReferenceEquals(this.Value, DBNull.Value)))
{
if ((this.Value != null))
{
ctl.Value = (DateTime)this.Value;
}
}
DataGridViewDatetimeSelectColumn fileColumn = (DataGridViewDatetimeSelectColumn)this.DataGridView.Columns[ColumnIndex];
}
public override Type EditType
{
// Return the type of the editing contol that DataGridViewDatetimeSelectCell uses.
get { return typeof(DataGridViewDatetimeSelectEdit); }
}
}
class DataGridViewDatetimeSelectEdit : DatetimeSelect, System.Windows.Forms.IDataGridViewEditingControl
{
private System.Windows.Forms.DataGridView dataGridViewControl;
private bool valueIsChanged = false;
private int rowIndexNum;
public DataGridViewDatetimeSelectEdit()
{
}
public object EditingControlFormattedValue
{
get { return this.Value; }
set
{
if (value is String)
{
this.Value = DateTime.Parse(Convert.ToString(value));
}
}
}
public object GetEditingControlFormattedValue(System.Windows.Forms.DataGridViewDataErrorContexts context)
{
return this.Value;
}
public void ApplyCellStyleToEditingControl(System.Windows.Forms.DataGridViewCellStyle dataGridViewCellStyle)
{
}
public int EditingControlRowIndex
{
get { return rowIndexNum; }
set { rowIndexNum = value; }
}
public bool EditingControlWantsInputKey(System.Windows.Forms.Keys key, bool dataGridViewWantsInputKey)
{
// Let the DateTimePicker handle the keys listed.
switch (key & System.Windows.Forms.Keys.KeyCode)
{
case System.Windows.Forms.Keys.Left:
case System.Windows.Forms.Keys.Up:
case System.Windows.Forms.Keys.Down:
case System.Windows.Forms.Keys.Right:
case System.Windows.Forms.Keys.Home:
case System.Windows.Forms.Keys.End:
case System.Windows.Forms.Keys.PageDown:
case System.Windows.Forms.Keys.PageUp:
return true;
default:
return false;
}
}
public void PrepareEditingControlForEdit(bool selectAll)
{
// No preparation needs to be done.
}
public bool RepositionEditingControlOnValueChange
{
get { return false; }
}
public System.Windows.Forms.DataGridView EditingControlDataGridView
{
get { return dataGridViewControl; }
set { dataGridViewControl = value; }
}
public bool EditingControlValueChanged
{
get { return valueIsChanged; }
set { valueIsChanged = value; }
}
public System.Windows.Forms.Cursor EditingControlCursor
{
get { return base.Cursor; }
}
System.Windows.Forms.Cursor System.Windows.Forms.IDataGridViewEditingControl.EditingPanelCursor
{
get { return EditingControlCursor; }
}
protected override void OnValueChanged(EventArgs eventargs)
{
// Notify the DataGridView that the contents of the cell have changed.
valueIsChanged = true;
this.EditingControlDataGridView.NotifyCurrentCellDirty(true);
base.OnValueChanged(eventargs);
}
}
#endregion
}
|
 |
 |
 |
 |
Date :
2021-01-29 22:25:08 |
By :
lamaka.tor |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
มาแก้โค้ดให้แหล่มขึ้นครับ
โค้ด DatetimeSelect ใช้โค้ดเดิม
แก้เฉพาะ DataGridViewDatetimeSelectColumn
โดยการสร้าง properties ShowUpDownDate ขึ้นมารองรับ
แต่ก็ต้องไม่ลืม Clone เพราะมันจะดึงค่าที่ เราตั้งไว้
Code (C#)
public class DataGridViewDatetimeSelectColumn : System.Windows.Forms.DataGridViewColumn
{
public DataGridViewDatetimeSelectColumn()
: base(new DataGridViewDatetimeSelectCell())
{
}
private bool _ShowUpDownDate = false;
[
DefaultValue(false), System.ComponentModel.Category("TORSetting")
]
public bool ShowUpDownDate
{
get
{
return _ShowUpDownDate;
}
set
{
_ShowUpDownDate = value;
}
}
public override object Clone()
{
var clm = base.Clone() as DataGridViewDatetimeSelectColumn;
DataGridViewDatetimeSelectColumn xxx = base.Clone() as DataGridViewDatetimeSelectColumn;
if (clm != null)
{
clm.ShowUpDownDate = _ShowUpDownDate;
}
return clm;
}
}
และ DataGridViewDatetimeSelectCell
แค่ เพิ่ม
Code (C#)
DataGridViewDatetimeSelectColumn fileColumn = (DataGridViewDatetimeSelectColumn)this.DataGridView.Columns[ColumnIndex];
ctl.ShowUpDownDate = fileColumn.ShowUpDownDate;
เพื่อให้ DataGridViewDatetimeSelectEdit มันรับค่า DataGridViewDatetimeSelectColumn มาได้ ครับ
Code (C#)
public class DataGridViewDatetimeSelectCell : System.Windows.Forms.DataGridViewTextBoxCell
{
public DataGridViewDatetimeSelectCell()
:base()
{
}
public override void InitializeEditingControl(int rowIndex, object initialFormattedValue, System.Windows.Forms.DataGridViewCellStyle dataGridViewCellStyle)
{
// Set the value of the editing control to the current cell value.
base.InitializeEditingControl(rowIndex, initialFormattedValue, dataGridViewCellStyle);
DataGridViewDatetimeSelectEdit ctl = (DataGridViewDatetimeSelectEdit)DataGridView.EditingControl;
if ((!object.ReferenceEquals(this.Value, DBNull.Value)))
{
if ((this.Value != null))
{
ctl.Value = (DateTime)this.Value;
}
}
DataGridViewDatetimeSelectColumn fileColumn = (DataGridViewDatetimeSelectColumn)this.DataGridView.Columns[ColumnIndex];
ctl.ShowUpDownDate = fileColumn.ShowUpDownDate;
}
public override Type EditType
{
// Return the type of the editing contol that DataGridViewDatetimeSelectCell uses.
get { return typeof(DataGridViewDatetimeSelectEdit); }
}
}
ผลคือ
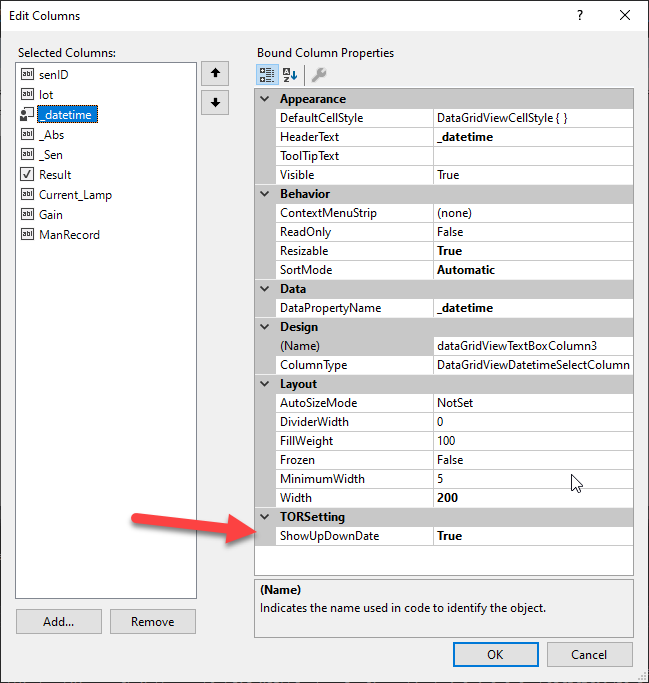

และ
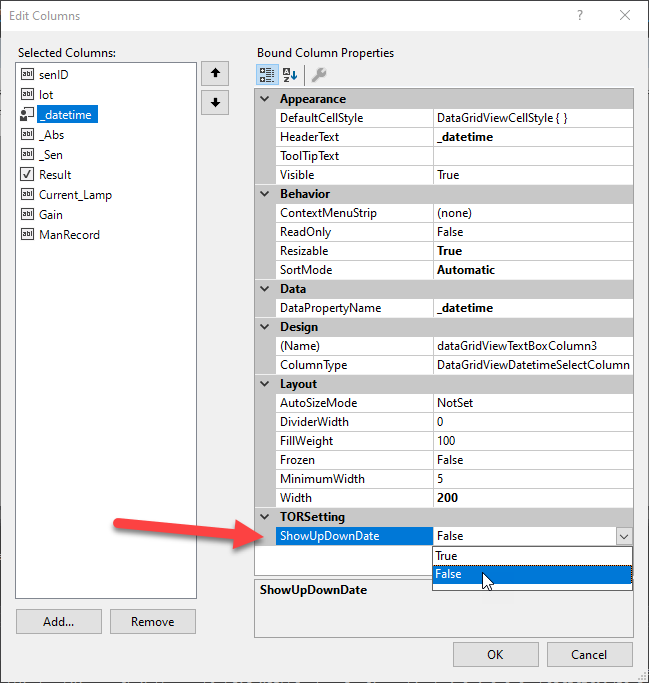
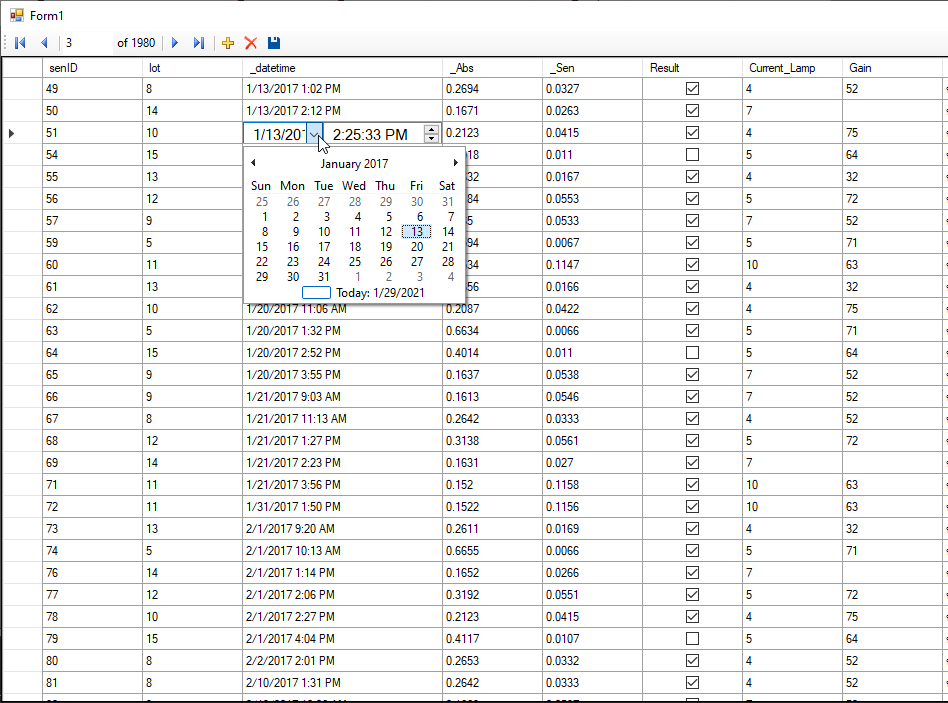
|
 |
 |
 |
 |
Date :
2021-01-29 22:46:43 |
By :
lamaka.tor |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ใส่ attribute browsable เพื่อให้สามารถกำหนดใน property ได้เลยครับ
|
 |
 |
 |
 |
Date :
2021-01-29 22:48:40 |
By :
LakornWorld |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
อ่าว ได้แล้ว ตอบช้าไป ในมือถือไม่ค่อยสะดวก
ขอบคุณอีกครั้งที่แจ้งข่าวครับ 
|
 |
 |
 |
 |
Date :
2021-01-29 22:52:59 |
By :
LakornWorld |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
คุณ LakornWorld กับ คุณ ผ่านมา
อย่าสลับ login กันสิครับ
ผม งง
|
 |
 |
 |
 |
Date :
2021-01-30 09:40:30 |
By :
...... |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
อ้าวววว
ทำไม comment โดยที่ไม่ได้ log in แล้วกลายเป็น user นี้ไปแทน
Стремись не к тому, чтобы добиться успеха, а к тому, чтобы твоя жизнь имела смысл. https://helloworl
งั้นเป็นที่ web หรือปล่าวครับ
|
 |
 |
 |
 |
Date :
2021-01-30 09:42:20 |
By :
..... |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
มีอะไรต้องทำอีกเยอะเลย อทิเช่น แก้ไขคำ
--- แก้ไขใบสำคัญ --> แก้ไขรายการบัญชี (อาจจะ)
--- เพิ่มผลแตกต่าง Dr/Cr จัดตำแหน่ง-แสดงผล ตามหลักการบัญชี
------ ชิดซ้าย/ชิดขวา และกรณีที่เป็น 0.00 ให้แสดง (-)
--- รายการบัญชี (Detail) สามารถ ระบุโครงการ/หน่วยงาน รวมถึง หมายเหตุด้วย
------ กำลังมองว่าจะ ยัดมันเข้าไป อย่างไร?
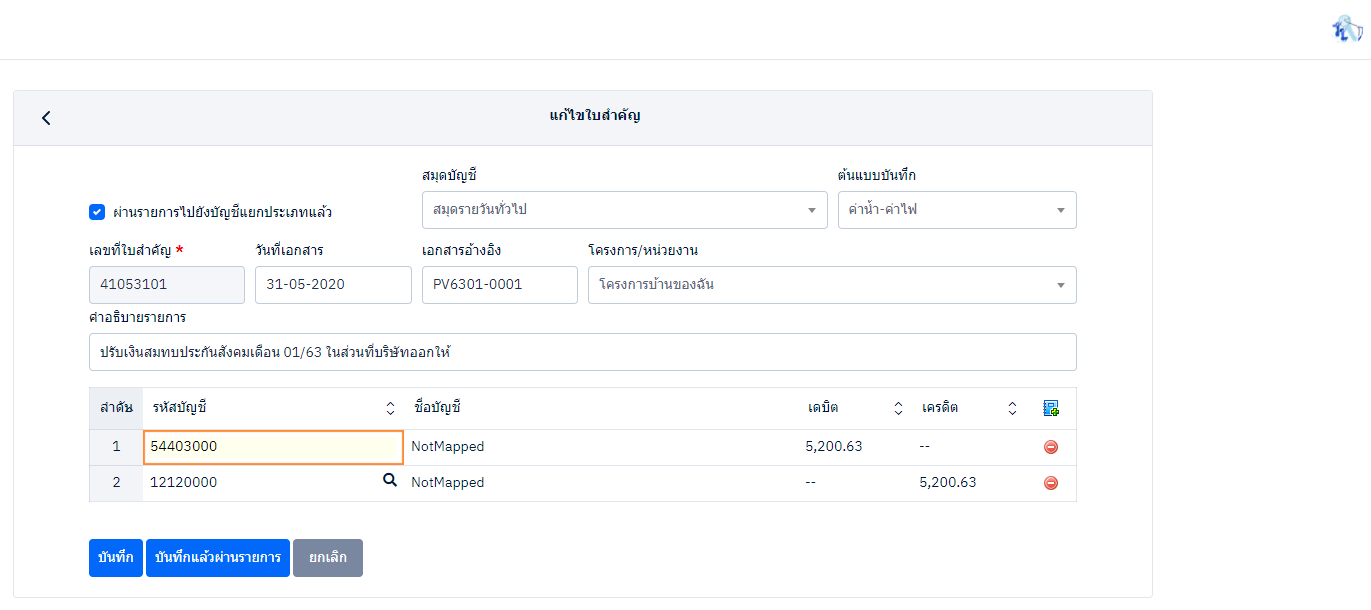
อันนี้ Source Code ของจริงเลยนะ
ดึงข้อมูลของส่วน เดบิต/เครดิต
Code (JavaScript)
function displayData(data, index, act) {
objJDTGl_JVD.clear().draw();
if (act == "E") { // แก้ไข ข้อมูล
txtJVNo.val(data.JVNo).prop("disabled", true);
txtJVDate.val(wls.Date.JsonDate2String(data.JVDate));
txtDocNo.val(data.DocNo);
txtTRemark.val(data.TRemark);
cboBook.val(data.JVBook);
cboProject.val(data.Project);
wlsAjaxHandler.execute("GET", "/Fucker/GeneralLedger/GetJVD?JVNo=" + data.JVNo + "&OrgCode=ETA", null, function (res) {
if (res) {
res.forEach((r, i) => {
let dr = new JVD();
dr.DT_RowId = "row_" + i; // Tricks
dr.JVSeq = r.JVSeq ? r.JVSeq : 0;
dr.ACCode = r.ACCode ? r.ACCode : "";
dr.ACTitle = r.ACTitle ? r.ACTitle : "NotMapped";
dr.Amount = r.Amount ? r.Amount : 0.00;
dr.Dr = r.Amount > 0 ? r.Amount : 0.00;
dr.Cr = r.Amount < 0 ? r.Amount * -1 : 0.00;
objJDTGl_JVD.row.add(dr).draw().node();
});
}
}, () => { });
} else { //เพิ่มข้อมูล
txtJVNo.val("").prop("disabled", false);
for (var i = 0; i < 3; i++) {
let dr = new JVD();
dr.DT_RowId = "row_" + i; // Tricks
dr.JVSeq = i;
dr.ACCode = "";
dr.ACTitle = "";
dr.Amount = 0.00;
dr.Dr = 0.00;
dr.Cr = 0.00;
objJDTGl_JVD.row.add(dr).draw().node();
}
wls.HTML.focus("txtJVNo");
}
}
|
 |
 |
 |
 |
Date :
2021-02-01 05:59:26 |
By :
ผ่านมา |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
นังคิดไปเรื่อยฯ เล่าอะไรให้ฟังไปเรื่อยเปื่อย
ระบบหลายภาษา ใช้ AskMeThat ไม่ได้ใช้ i18n
ใส่ Vue3 และ Blazor เข้าไปในหน้า Master file (ด้วยเหตุผลบางอย่าง)
ที่สำคัญ ตั้งใจเปิดเป็น Open Source ระบบบัญชี
เพิ่มช่องภาษาอังกฤษ ในส่วน คำอธิบายรายการ (Heading) (ใช้ไม่ใช้ ค่อยว่ากันทีหลัง) เก็บเอาไว้ก่อน
เพิ่มช่อง คำอธิบายบัญชีกำหนดเอง (ใช้ไม่ใช้ ค่อยว่ากันทีหลัง) เก็บเอาไว้ก่อน
เพิ่มช่องภาษาอังกฤษ ในส่วน คำอธิบายบัญชี (Detail) (ใช้ไม่ใช้ ค่อยว่ากันทีหลัง) เก็บเอาไว้ก่อน)
แก้ไข Source Code
Code (JavaScript)
wlsAjaxHandler.execute("GET", "/Fucker/GeneralLedger/GetJVD?JVNo=" + data.JVNo + "&OrgCode=ETA", null, function (res) {
if (res) {
res.forEach((r, i) => {
let dr = new JVD();
let amt = r.Amount ? r.Amount : 0.00;
dr.DT_RowId = "row_" + i; // Tricks
dr.JVSeq = r.JVSeq ? r.JVSeq : 0;
dr.ACCode = r.ACCode ? r.ACCode : "";
dr.ACTitle = r.ACTitle ? r.ACTitle : "NotMapped";
dr.Amount = amt; //r.Amount ? r.Amount : 0.00;
dr.Dr = amt > 0 ? amt : 0.00; //r.Amount > 0 ? r.Amount : 0.00;
dr.Cr = amt < 0 ? amt * -1 : 0.00; //r.Amount < 0 ? r.Amount * -1 : 0.00;
objJDTGl_JVD.row.add(dr).draw().node();
});
}
}, (error) => { alert(error); });
|
 |
 |
 |
 |
Date :
2021-02-01 07:54:01 |
By :
ผ่านมา |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
มาบ่นเล่าให้ฟังต่อ โดยโจทย์มีอยู่ว่า ปีนี้คือปี 2021 (2564) มันมีอะไรใหม่ฯบ้าง
คิดและวิเคราะห์แบบ ลึกสุดใจ ลึกสุดสุด มองไกลไปถึงโลกหน้า (Undocument)
1. Vue3 JavaScript Framework
--- OK ใส่เข้าไปแล้ว หนึ่งหน้า (ข้อมูลแผนก) +55555
2. Blazor and .NET 5
--- คิดเอาไว้ อย่างน้อยฯเยอะมาก อธิบายไม่ไหว ดูรูปเอาเองก็แล้วกัน
------ ถ้าจัดการ JavaScript/Jquery อยู่หมัด ก็น่าจะทำเป็น Production ได้
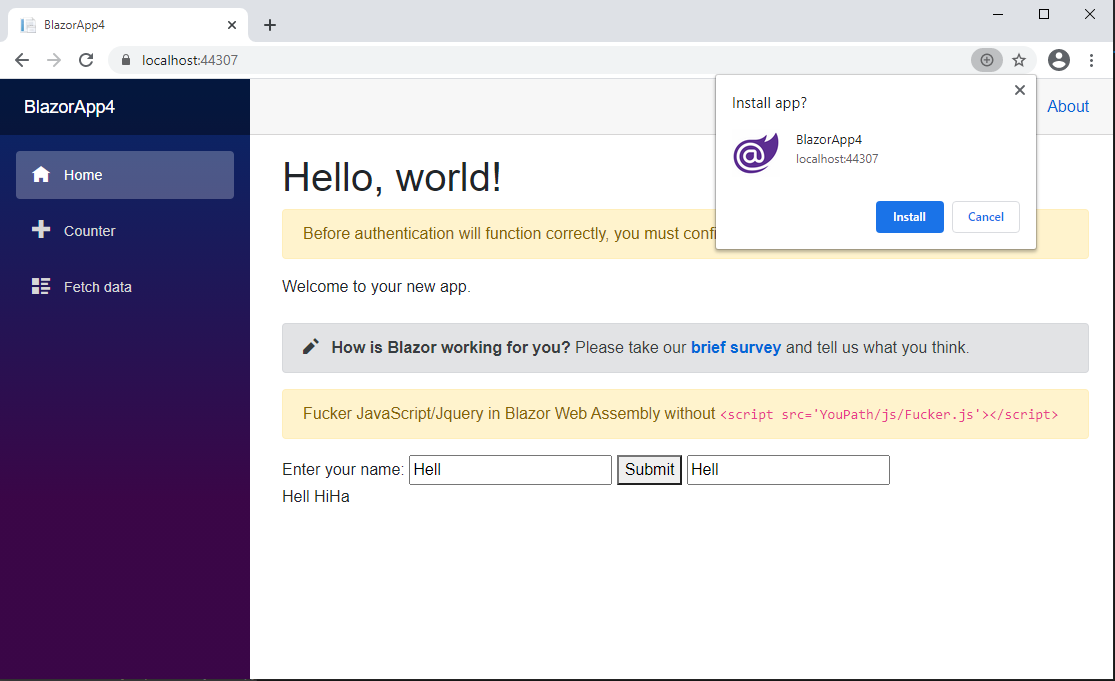
File Index.rasor
Code (C#)
@page "/"
@inject IJSRuntime JSRuntime
@implements IAsyncDisposable
<h1>Hello, world!</h1>
<div class="alert alert-warning" role="alert">
Before authentication will function correctly, you must configure your provider details in <code>Program.cs</code>
</div>
Welcome to your new app.
<SurveyPrompt Title="How is Blazor working for you?" />
<div class="alert alert-warning" role="alert">
Fucker JavaScript/Jquery in Blazor Web Assembly without <code>@("<script src='YouPath/js/Fucker.js'></script>")</code>
</div>
Enter your name:
<input @bind="name" />
<button @onclick="Submit">Submit</button>
<input @bind="name" />
@retValue
@code {
private string name;
private string retValue;
// Load the module and keep a reference to it
// You need to use .AsTask() to convert the ValueTask to Task as it may be awaited multiple times
private Task<IJSObjectReference> _module;
private Task<IJSObjectReference> Module => _module ??= JSRuntime.InvokeAsync<IJSObjectReference>("import", "./js/demo.js").AsTask();
async Task Submit() {
var module = await Module;
await module.InvokeVoidAsync("sayHi", name);
retValue = await module.InvokeAsync<string>("Fucker", name);
}
public async ValueTask DisposeAsync() {
if (_module != null) {
var module = await _module;
await module.DisposeAsync();
}
}
}
File demo.js
Code (JavaScript)
// Use the module syntax to export the function
export function sayHi(name) {
alert(`Hello ${name}!`);
}
export function Fucker(name) {
return name + " HiHa";
}
|
 |
 |
 |
 |
Date :
2021-02-03 08:19:34 |
By :
ผ่านมา |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
โครงสร้าง Project Template
1. Create Project Blazor
2. Choice Blazor Web Assembly (Not Blazor Server)
3. choice .NET 5 (Not .NET Core 3.1)
Enjoy with Fucker
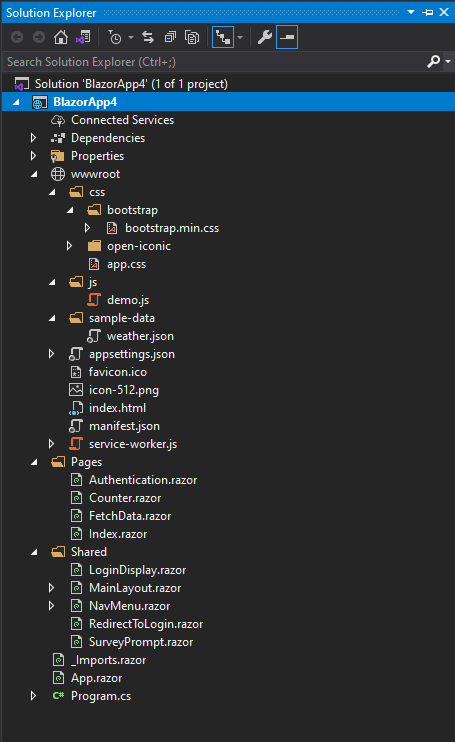
|
 |
 |
 |
 |
Date :
2021-02-03 08:48:08 |
By :
ผ่านมา |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
บ่นเล่าให้ฟัง ไปเรื่อยฯ
จริงฯแล้วเจ้าตัว Blazor มันออกแบบมาสำหรับคนที่เขียน VB/C#/etc...
และเขียน เวปไม่เป็น หรือ ไอ้พวก ดีแต่พูด
(เราคิดแบบนี้ อาจจะผิดก็ได้) และมีรายละเอียดอีกเยอะมากฯฯฯฯฯ ที่ต้องเก็บ
สองวันแล้วนั่ง แงะ (Hacks) มัน ผลลัพธ์ที่ได้ก็คุ้มนะ ทลายข้อจำกัดของ Blazor Web Assembly ได้ระดับหนึ่ง
นั่นคือ ทำตัว FuckerScript ขึ้นมา
--- Normal JavaScript (FuckerScript)
--- JavaScript Module เช่น
SayHi.js
Code (JavaScript)
export function sayHi(yourMother) {
alert("wow wow wow " + yourMother);
}
Code (C#)
<FuckerScript>
<script>
var JiMi = "wow wow wow";
$(function () {
$("#btnHacks").on("click", function () {
alert("sayHi aHa " + JiMi);
});
});
////If you want to include a JS file in a JS you can use jQuery.getScript() for that
//$.getScript('test1.js', function () {
// //script is loaded and executed put your dependent JS here
// test1();
//});
////And if you cannot use jQuery
//var imported = document.createElement('script');
//imported.src = '/path/to/imported/script';
//document.head.appendChild(imported);
////document.getElementsByTagName('head')[0].appendChild(...)
//document.getElementsByTagName("head")[0].insertAdjacentHTML("beforeend", "<link rel=\"stylesheet\" href=\"path/to/style.css\" />");
</script>
</FuckerScript>
|
 |
 |
 |
 |
Date :
2021-02-04 13:33:46 |
By :
ผ่านมา |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
|
|