Android Shared Preferences : Login Username/Password (PHP/MySQL) |
Android Shared Preferences : Login Username/Password (PHP/MySQL) บทความนี้จะเป็นการเขียน Android App ประยุกต์การใช้งาน Shared Preferences กับการ Login ผ่านระบบ Database ของ MySQL ซึ่งจะใช้ PHP ทำงานในฝั่ง Web Server โดยใน Application ของ Android จะส่งค่า Username/Password ไปตรวจสอบที่ Web Server เมื่อข้อมูลถูกต้องทางฝั่ง Web Server จะส่งสถานะกลับมายัง Android App พร้อมด้วย UserID ที่ถูกต้อง ซึ่งบน Android App จะนำค่า UserID หรือ MemberID นี้จัดเก็บลงใน Shared Preferences เพื่อไปใช้งานในส่วนอื่น ๆ ของ App การทำงานนี้จะคล้าย ๆ กับระบบสมาชิกที่ทำงานบน Web Application ในรูปแบบของ Session ทั่ว ๆ ไป
Android Shared Preferences : Login Username/Password (PHP/MySQL)
AndroidManifest.xml
<uses-permission android:name="android.permission.INTERNET" />
ในการเขียน Android เพื่อติดต่อกับ Internet จะต้องกำหนด Permission ในส่วนนี้ด้วยทุกครั้ง
Web Server (PHP and MySQL)
member
CREATE TABLE `member` (
`MemberID` int(2) NOT NULL auto_increment,
`Username` varchar(50) NOT NULL,
`Password` varchar(50) NOT NULL,
`Name` varchar(50) NOT NULL,
`Tel` varchar(50) NOT NULL,
`Email` varchar(150) NOT NULL,
PRIMARY KEY (`MemberID`),
UNIQUE KEY `Username` (`Username`),
UNIQUE KEY `Email` (`Email`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8 AUTO_INCREMENT=4 ;
--
-- Dumping data for table `member`
--
INSERT INTO `member` VALUES (1, 'weerachai', 'weerachai@1', 'Weerachai Nukitram', '0819876107', '[email protected]');
INSERT INTO `member` VALUES (2, 'adisorn', 'adisorn@2', 'Adisorn Bunsong', '021978032', '[email protected]');
INSERT INTO `member` VALUES (3, 'surachai', 'surachai@3', 'Surachai Sirisart', '0876543210', '[email protected]');

โครงสร้างของข้อมูล MySQL Database
checkLogin.php (ไฟล์ PHP ใช้สำหรับตรวจสอบ Username และ Password)
<?php
$objConnect = mysql_connect("localhost","root","root");
$objDB = mysql_select_db("mydatabase");
//$_POST["strUser"] = "weerachai"; // for Sample
//$_POST["strUser"] = "weerachai@1"; // for Sample
$strUsername = $_POST["strUser"];
$strPassword = $_POST["strPass"];
$strSQL = "SELECT * FROM member WHERE 1
AND Username = '".$strUsername."'
AND Password = '".$strPassword."'
";
$objQuery = mysql_query($strSQL);
$objResult = mysql_fetch_array($objQuery);
$intNumRows = mysql_num_rows($objQuery);
if($intNumRows==0)
{
$arr['StatusID'] = "0";
$arr['MemberID'] = "0";
$arr['Error'] = "Incorrect Username and Password";
}
else
{
$arr['StatusID'] = "1";
$arr['MemberID'] = $objResult["MemberID"];
$arr['Error'] = "";
}
/**
$arr['StatusID'] // (0=Failed , 1=Complete)
$arr['MemberID'] // MemberID
$arr['Error' // Error Message
*/
mysql_close($objConnect);
echo json_encode($arr);
?>
getByMemberID.php (ไฟล์ PHP ใช้สำหรับอ่านรายละเอียดของ User)
<?php
$objConnect = mysql_connect("localhost","root","root");
$objDB = mysql_select_db("mydatabase");
//$_POST["sMemberID"] = "1"; // for Sample
$strMemberID = $_POST["sMemberID"];
$strSQL = "SELECT * FROM member WHERE 1 AND MemberID = '".$strMemberID."' ";
$objQuery = mysql_query($strSQL);
$obResult = mysql_fetch_array($objQuery);
if($obResult)
{
$arr["MemberID"] = $obResult["MemberID"];
$arr["Username"] = $obResult["Username"];
$arr["Password"] = $obResult["Password"];
$arr["Name"] = $obResult["Name"];
$arr["Email"] = $obResult["Email"];
$arr["Tel"] = $obResult["Tel"];
}
mysql_close($objConnect);
/*** return JSON by MemberID ***/
/* Eg :
{"MemberID":"2",
"Username":"adisorn",
"Password":"adisorn@2",
"Name":"Adisorn Bunsong",
"Tel":"021978032",
"Email":"[email protected]"}
*/
echo json_encode($arr);
?>
Example การสร้าง Login กับ PHP/MySQL เพื่อเก็บค่า UserID หรือ MemberID นำไปใช้งานใน Activity อื่น ๆ
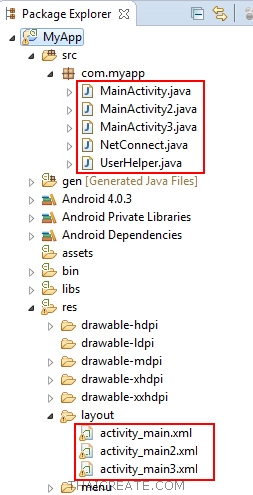
โครงสร้างของไฟล์ที่จะใช้สามารถใช้ได้ทั้งบน Eclipse และ Android Studio
UserHelper.java
package com.myapp;
import android.content.Context;
import android.content.SharedPreferences;
import android.content.SharedPreferences.Editor;
public class UserHelper {
Context context;
SharedPreferences sharedPerfs;
Editor editor;
// Prefs Keys
static String perfsName = "UserHelper";
static int perfsMode = 0;
public UserHelper(Context context) {
this.context = context;
this.sharedPerfs = this.context.getSharedPreferences(perfsName, perfsMode);
this.editor = sharedPerfs.edit();
}
public void createSession(String sMemberID) {
editor.putBoolean("LoginStatus", true);
editor.putString("MemberID", sMemberID);
editor.commit();
}
public void deleteSession() {
editor.clear();
editor.commit();
}
public boolean getLoginStatus() {
return sharedPerfs.getBoolean("LoginStatus", false);
}
public String getMemberID() {
return sharedPerfs.getString("MemberID", null);
}
}
NetConnect.java
package com.myapp;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.util.List;
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.NameValuePair;
import org.apache.http.StatusLine;
import org.apache.http.client.ClientProtocolException;
import org.apache.http.client.HttpClient;
import org.apache.http.client.entity.UrlEncodedFormEntity;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.impl.client.DefaultHttpClient;
import android.util.Log;
public class NetConnect {
public static String getHttpPost(String url,List<NameValuePair> params) {
StringBuilder str = new StringBuilder();
HttpClient client = new DefaultHttpClient();
HttpPost httpPost = new HttpPost(url);
try {
httpPost.setEntity(new UrlEncodedFormEntity(params,"UTF-8"));
HttpResponse response = client.execute(httpPost);
StatusLine statusLine = response.getStatusLine();
int statusCode = statusLine.getStatusCode();
if (statusCode == 200) { // Status OK
HttpEntity entity = response.getEntity();
InputStream content = entity.getContent();
BufferedReader reader = new BufferedReader(new InputStreamReader(content));
String line;
while ((line = reader.readLine()) != null) {
str.append(line);
}
} else {
Log.e("Log", "Failed to download result..");
}
} catch (ClientProtocolException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
return str.toString();
}
public static String getHttpGet(String url) {
StringBuilder str = new StringBuilder();
HttpClient client = new DefaultHttpClient();
HttpGet httpGet = new HttpGet(url);
try {
HttpResponse response = client.execute(httpGet);
StatusLine statusLine = response.getStatusLine();
int statusCode = statusLine.getStatusCode();
if (statusCode == 200) { // Download OK
HttpEntity entity = response.getEntity();
InputStream content = entity.getContent();
BufferedReader reader = new BufferedReader(new InputStreamReader(content));
String line;
while ((line = reader.readLine()) != null) {
str.append(line);
}
} else {
Log.e("Log", "Failed to download result..");
}
} catch (ClientProtocolException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
return str.toString();
}
}
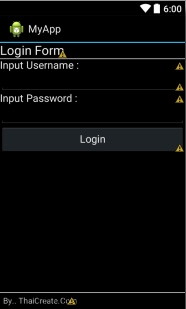
activity_main.xml
<TableLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/tableLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<TableRow
android:id="@+id/tableRow1"
android:layout_width="wrap_content"
android:layout_height="wrap_content" >
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:gravity="center"
android:text="Login Form "
android:layout_span="1"
android:textAppearance="?android:attr/textAppearanceLarge" />
</TableRow>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<TableLayout
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_weight="0.1"
android:orientation="horizontal">
<TextView
android:id="@+id/textView3"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Input Username :"
android:textAppearance="?android:attr/textAppearanceMedium" />
<EditText
android:id="@+id/txtUsername"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:ems="10" >
</EditText>
<TextView
android:id="@+id/textView4"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Input Password :"
android:textAppearance="?android:attr/textAppearanceMedium" />
<EditText
android:id="@+id/txtPassword"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:ems="10"
android:inputType="textPassword" >
</EditText>
<Button
android:id="@+id/btnLogin"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Login" />
</TableLayout >
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<LinearLayout
android:id="@+id/LinearLayout1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="5dip" >
<TextView
android:id="@+id/textView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="By.. ThaiCreate.Com" />
</LinearLayout>
</TableLayout>
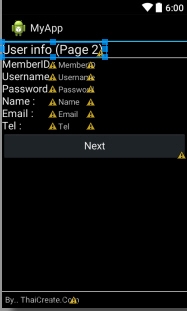
activity_main2.xml
<TableLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/tableLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<TableRow
android:id="@+id/tableRow1"
android:layout_width="wrap_content"
android:layout_height="wrap_content" >
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:gravity="center"
android:text="User info (Page 2) "
android:layout_span="1"
android:textAppearance="?android:attr/textAppearanceLarge" />
</TableRow>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<TableLayout
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_weight="0.1"
android:orientation="horizontal">
<TableRow
android:layout_width="fill_parent"
android:layout_height="wrap_content">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="MemberID : "
android:textAppearance="?android:attr/textAppearanceMedium" />
<TextView
android:id="@+id/txtMemberID"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="MemberID" />
</TableRow>
<TableRow
android:layout_width="fill_parent"
android:layout_height="wrap_content">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Username : "
android:textAppearance="?android:attr/textAppearanceMedium" />
<TextView
android:id="@+id/txtUsername"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Username" />
</TableRow>
<TableRow
android:layout_width="fill_parent"
android:layout_height="wrap_content">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Password : "
android:textAppearance="?android:attr/textAppearanceMedium" />
<TextView
android:id="@+id/txtPassword"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Password" />
</TableRow>
<TableRow
android:layout_width="fill_parent"
android:layout_height="wrap_content">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Name : "
android:textAppearance="?android:attr/textAppearanceMedium" />
<TextView
android:id="@+id/txtName"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Name" />
</TableRow>
<TableRow
android:layout_width="fill_parent"
android:layout_height="wrap_content">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Email : "
android:textAppearance="?android:attr/textAppearanceMedium" />
<TextView
android:id="@+id/txtEmail"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Email" />
</TableRow>
<TableRow
android:layout_width="fill_parent"
android:layout_height="wrap_content">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Tel : "
android:textAppearance="?android:attr/textAppearanceMedium" />
<TextView
android:id="@+id/txtTel"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Tel" />
</TableRow>
<Button
android:id="@+id/btnNext"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Next" />
</TableLayout >
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<LinearLayout
android:id="@+id/LinearLayout1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="5dip" >
<TextView
android:id="@+id/textView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="By.. ThaiCreate.Com" />
</LinearLayout>
</TableLayout>
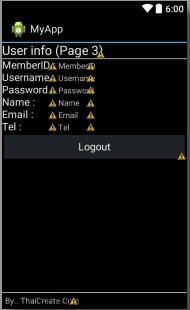
activity_main3.xml
<TableLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/tableLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<TableRow
android:id="@+id/tableRow1"
android:layout_width="wrap_content"
android:layout_height="wrap_content" >
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:gravity="center"
android:text="User info (Page 3) "
android:layout_span="1"
android:textAppearance="?android:attr/textAppearanceLarge" />
</TableRow>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<TableLayout
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_weight="0.1"
android:orientation="horizontal">
<TableRow
android:layout_width="fill_parent"
android:layout_height="wrap_content">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="MemberID : "
android:textAppearance="?android:attr/textAppearanceMedium" />
<TextView
android:id="@+id/txtMemberID"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="MemberID" />
</TableRow>
<TableRow
android:layout_width="fill_parent"
android:layout_height="wrap_content">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Username : "
android:textAppearance="?android:attr/textAppearanceMedium" />
<TextView
android:id="@+id/txtUsername"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Username" />
</TableRow>
<TableRow
android:layout_width="fill_parent"
android:layout_height="wrap_content">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Password : "
android:textAppearance="?android:attr/textAppearanceMedium" />
<TextView
android:id="@+id/txtPassword"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Password" />
</TableRow>
<TableRow
android:layout_width="fill_parent"
android:layout_height="wrap_content">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Name : "
android:textAppearance="?android:attr/textAppearanceMedium" />
<TextView
android:id="@+id/txtName"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Name" />
</TableRow>
<TableRow
android:layout_width="fill_parent"
android:layout_height="wrap_content">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Email : "
android:textAppearance="?android:attr/textAppearanceMedium" />
<TextView
android:id="@+id/txtEmail"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Email" />
</TableRow>
<TableRow
android:layout_width="fill_parent"
android:layout_height="wrap_content">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Tel : "
android:textAppearance="?android:attr/textAppearanceMedium" />
<TextView
android:id="@+id/txtTel"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Tel" />
</TableRow>
<Button
android:id="@+id/btnLogout"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Logout" />
</TableLayout >
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<LinearLayout
android:id="@+id/LinearLayout1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="5dip" >
<TextView
android:id="@+id/textView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="By.. ThaiCreate.Com" />
</LinearLayout>
</TableLayout>
ไฟล์ Java
MainActivity.java
package com.myapp;
import java.util.ArrayList;
import java.util.List;
import org.apache.http.NameValuePair;
import org.apache.http.message.BasicNameValuePair;
import org.json.JSONException;
import org.json.JSONObject;
import android.app.Activity;
import android.app.AlertDialog;
import android.content.Intent;
import android.os.Bundle;
import android.os.StrictMode;
import android.view.Menu;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.Toast;
public class MainActivity extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
//*** Permission StrictMode
if (android.os.Build.VERSION.SDK_INT > 9) {
StrictMode.ThreadPolicy policy = new StrictMode.ThreadPolicy.Builder().permitAll().build();
StrictMode.setThreadPolicy(policy);
}
//*** Session Login
final UserHelper usrHelper = new UserHelper(this);
//*** txtUsername & txtPassword
final EditText txtUser = (EditText)findViewById(R.id.txtUsername);
final EditText txtPass = (EditText)findViewById(R.id.txtPassword);
//*** Alert Dialog
final AlertDialog.Builder ad = new AlertDialog.Builder(this);
//*** Login Button
final Button btnLogin = (Button) findViewById(R.id.btnLogin);
btnLogin.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
String url = "https://www.thaicreate.com/android/checkLogin.php";
List<NameValuePair> params = new ArrayList<NameValuePair>();
params.add(new BasicNameValuePair("strUser", txtUser.getText().toString()));
params.add(new BasicNameValuePair("strPass", txtPass.getText().toString()));
/** Get result from Server (Return the JSON Code)
* StatusID = ? [0=Failed,1=Complete]
* MemberID = ? [Eg : 1]
* Error = ? [On case error return custom error message]
*
* Eg Login Failed = {"StatusID":"0","MemberID":"0","Error":"Incorrect Username and Password"}
* Eg Login Complete = {"StatusID":"1","MemberID":"2","Error":""}
*/
String resultServer = NetConnect.getHttpPost(url,params);
/*** Default Value ***/
String strStatusID = "0";
String strMemberID = "0";
String strError = "Unknow Status!";
JSONObject c;
try {
c = new JSONObject(resultServer);
strStatusID = c.getString("StatusID");
strMemberID = c.getString("MemberID");
strError = c.getString("Error");
} catch (JSONException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
// Prepare Login
if(strStatusID.equals("0"))
{
// Dialog
ad.setTitle("Error! ");
ad.setIcon(android.R.drawable.btn_star_big_on);
ad.setPositiveButton("Close", null);
ad.setMessage(strError);
ad.show();
txtUser.setText("");
txtPass.setText("");
}
else
{
Toast.makeText(MainActivity.this, "Login OK", Toast.LENGTH_SHORT).show();
// Create Session
usrHelper.createSession(strMemberID);
// Goto Activity2
Intent newActivity = new Intent(MainActivity.this,MainActivity2.class);
startActivity(newActivity);
}
}
});
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.main, menu);
return true;
}
}
MainActivity2.java
package com.myapp;
import java.util.ArrayList;
import java.util.List;
import org.apache.http.NameValuePair;
import org.apache.http.message.BasicNameValuePair;
import org.json.JSONException;
import org.json.JSONObject;
import android.app.Activity;
import android.content.Intent;
import android.os.Bundle;
import android.os.StrictMode;
import android.view.Menu;
import android.view.View;
import android.widget.Button;
import android.widget.TextView;
public class MainActivity2 extends Activity {
String strMemberID = "";
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main2);
//*** Permission StrictMode
if (android.os.Build.VERSION.SDK_INT > 9) {
StrictMode.ThreadPolicy policy = new StrictMode.ThreadPolicy.Builder().permitAll().build();
StrictMode.setThreadPolicy(policy);
}
//*** Get Session Login
final UserHelper usrHelper = new UserHelper(this);
//*** Get Login Status
if(!usrHelper.getLoginStatus())
{
Intent newActivity = new Intent(MainActivity2.this, MainActivity.class);
startActivity(newActivity);
}
//*** Get Member ID from Session
strMemberID = usrHelper.getMemberID();
//*** Show User Info
showInfo();
//*** Button Next
final Button btnNext = (Button) findViewById(R.id.btnNext);
btnNext.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
// Goto Activity 2
Intent newActivity = new Intent(MainActivity2.this,MainActivity3.class);
startActivity(newActivity);
}
});
}
public void showInfo()
{
// txtMemberID,txtMemberID,txtUsername,txtPassword,txtName,txtEmail,txtTel
final TextView tMemberID = (TextView)findViewById(R.id.txtMemberID);
final TextView tUsername = (TextView)findViewById(R.id.txtUsername);
final TextView tPassword = (TextView)findViewById(R.id.txtPassword);
final TextView tName = (TextView)findViewById(R.id.txtName);
final TextView tEmail = (TextView)findViewById(R.id.txtEmail);
final TextView tTel = (TextView)findViewById(R.id.txtTel);
String url = "https://www.thaicreate.com/android/getByMemberID.php";
List<NameValuePair> params = new ArrayList<NameValuePair>();
params.add(new BasicNameValuePair("sMemberID", strMemberID));
/** Get result from Server (Return the JSON Code)
*
* {"MemberID":"2","Username":"adisorn","Password":"adisorn@2","Name":"Adisorn Bunsong","Tel":"021978032","Email":"[email protected]"}
*/
String resultServer = NetConnect.getHttpPost(url,params);
String strMemberID = "";
String strUsername = "";
String strPassword = "";
String strName = "";
String strEmail = "";
String strTel = "";
JSONObject c;
try {
c = new JSONObject(resultServer);
strMemberID = c.getString("MemberID");
strUsername = c.getString("Username");
strPassword = c.getString("Password");
strName = c.getString("Name");
strEmail = c.getString("Email");
strTel = c.getString("Tel");
if(!strMemberID.equals(""))
{
tMemberID.setText(strMemberID);
tUsername.setText(strUsername);
tPassword.setText(strPassword);
tName.setText(strName);
tEmail.setText(strEmail);
tTel.setText(strTel);
}
else
{
tMemberID.setText("-");
tUsername.setText("-");
tPassword.setText("-");
tName.setText("-");
tEmail.setText("-");
tTel.setText("-");
}
} catch (JSONException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.main, menu);
return true;
}
}
MainActivity3.java
package com.myapp;
import java.util.ArrayList;
import java.util.List;
import org.apache.http.NameValuePair;
import org.apache.http.message.BasicNameValuePair;
import org.json.JSONException;
import org.json.JSONObject;
import android.app.Activity;
import android.content.Intent;
import android.os.Bundle;
import android.os.StrictMode;
import android.view.Menu;
import android.view.View;
import android.widget.Button;
import android.widget.TextView;
public class MainActivity3 extends Activity {
String strMemberID = "";
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main3);
//*** Permission StrictMode
if (android.os.Build.VERSION.SDK_INT > 9) {
StrictMode.ThreadPolicy policy = new StrictMode.ThreadPolicy.Builder().permitAll().build();
StrictMode.setThreadPolicy(policy);
}
//*** Get Session Login
final UserHelper usrHelper = new UserHelper(this);
//*** Get Login Status
if(!usrHelper.getLoginStatus())
{
Intent newActivity = new Intent(MainActivity3.this, MainActivity.class);
startActivity(newActivity);
}
//*** Get Member ID from Session
strMemberID = usrHelper.getMemberID();
//*** Show User Info
showInfo();
//*** Button Logout
final Button btnLogout = (Button) findViewById(R.id.btnLogout);
btnLogout.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
// Clear Session
usrHelper.deleteSession();
// Goto Acitity2
Intent newActivity = new Intent(MainActivity3.this,MainActivity.class);
startActivity(newActivity);
}
});
}
public void showInfo()
{
// txtMemberID,txtMemberID,txtUsername,txtPassword,txtName,txtEmail,txtTel
final TextView tMemberID = (TextView)findViewById(R.id.txtMemberID);
final TextView tUsername = (TextView)findViewById(R.id.txtUsername);
final TextView tPassword = (TextView)findViewById(R.id.txtPassword);
final TextView tName = (TextView)findViewById(R.id.txtName);
final TextView tEmail = (TextView)findViewById(R.id.txtEmail);
final TextView tTel = (TextView)findViewById(R.id.txtTel);
String url = "https://www.thaicreate.com/android/getByMemberID.php";
List<NameValuePair> params = new ArrayList<NameValuePair>();
params.add(new BasicNameValuePair("sMemberID", strMemberID));
/** Get result from Server (Return the JSON Code)
*
* {"MemberID":"2","Username":"adisorn","Password":"adisorn@2","Name":"Adisorn Bunsong","Tel":"021978032","Email":"[email protected]"}
*/
String resultServer = NetConnect.getHttpPost(url,params);
String strMemberID = "";
String strUsername = "";
String strPassword = "";
String strName = "";
String strEmail = "";
String strTel = "";
JSONObject c;
try {
c = new JSONObject(resultServer);
strMemberID = c.getString("MemberID");
strUsername = c.getString("Username");
strPassword = c.getString("Password");
strName = c.getString("Name");
strEmail = c.getString("Email");
strTel = c.getString("Tel");
if(!strMemberID.equals(""))
{
tMemberID.setText(strMemberID);
tUsername.setText(strUsername);
tPassword.setText(strPassword);
tName.setText(strName);
tEmail.setText(strEmail);
tTel.setText(strTel);
}
else
{
tMemberID.setText("-");
tUsername.setText("-");
tPassword.setText("-");
tName.setText("-");
tEmail.setText("-");
tTel.setText("-");
}
} catch (JSONException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.main, menu);
return true;
}
}
AndroidManifest.xml
<activity
android:name=".MainActivity2"
android:label="@string/app_name" />
<activity
android:name=".MainActivity3"
android:label="@string/app_name" />
Screenshot
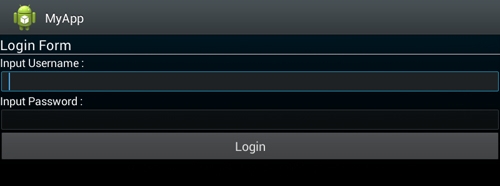
หน้า Login กรอก Username และ Password เพื่อทำการ Login
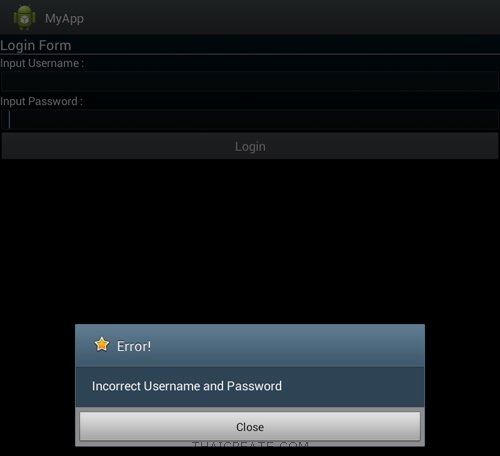
กรณีที่ Login ไม่ถูกต้องจะแจ้ง Error Message
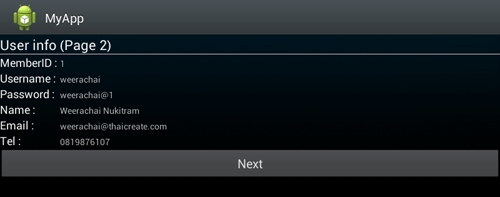
กรณีที่ Login ถูกต้องจะมีการจัดเก็บค่า UserID หรือ MemberID เพื่อไปใช้งานยัง Activity อื่น ๆ
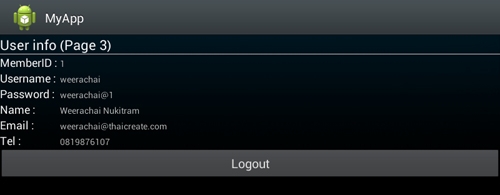
นำค่า UserID หรือ MemberID เพื่อไปใช้งานยัง Activity อื่น ๆ
.
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
   |
|
|
Create/Update Date : |
2015-11-16 15:53:54 /
2017-03-26 21:06:08 |
|
Download : |
No files |
|
Sponsored Links / Related |
|
|
|
|
|
|