Example 7 : Windows Store App and Login Form (Web Services) - C# |
Example 7 : Windows Store App and Login Form (Web Services) - C# สำหรับ Example : 7 จะเป็น Workshop ตัวอย่างการเขียน Windows Store Apps เพื่อทำระบบ Login ตรวจสอบ Username และ Password จากระบบสมาชิกที่ข้อมูลถูกอยู่บน Web Server และเราจะใช้ช่องทางของ Web Services ในการตรวจสอบข้อมูลที่ Login นั้นถูกต้องกับฐานข้อมูลของสมาชิกหรือไม่ ซึ่งในตัวอย่างนี้จะประกอบด้วย App จำนวน 2 Page ใน Page แรกจะสร้าง Form สำหรับกรอก Username และ Password เพื่อไปตรวจสอบ และหลังจากที่ Login ผ่านหรือข้อมูลถูกต้อง ก็จะส่งข้อมูลไปยัง Page ที่สอง เพื่อแสดงข้อมูลในรูปแบบของ User Profile ของสมาชิกที่ทำการ Login เข้ามาในระบบ และเนื่องจากการทำงาจะเป็น 2 รูปแบบ ฉะนั้นบน Web Services ก็จะต้องมี Method อยู่ 2 ส่วนด้วยกันคือ สำหรับตรวจสอบการ Login และ แสดงรายการที่เลือก (User Profile) มาแสดง
Windows Store App and Web Services (C#)
Windows Store Apps / User Login Form
Windows Store Apps / Login Detail Profile
สิ่งที่จะได้จากตัวอย่างนี้คือ
- การสร้าง Web Services การทำระบบตรวจสอบ Username และ Password
- การใช้ Windows Store Apps เรียกใช้งาน Web Services การส่ง Parameters ของ User/Password ไปตรวจสอบ
- การนำ Result JSON จากการ Web Services มา แสดงผล บนหน้าจอ Windows Store Apps
- การสร้าง Page บน Windos Store App ที่มากกว่า 1 Page
- การส่งค่าข้าม Page ในรูปแบบของ Master-Detail เพื่อแสดง Profile
ฝั่ง Web Services (ASP.Net/C#/MySQL)
MySQL Table บน Web Server

CREATE TABLE `member` (
`MemberID` int(2) NOT NULL auto_increment,
`Username` varchar(50) NOT NULL,
`Password` varchar(50) NOT NULL,
`Name` varchar(50) NOT NULL,
`Tel` varchar(50) NOT NULL,
`Email` varchar(150) NOT NULL,
PRIMARY KEY (`MemberID`),
UNIQUE KEY `Username` (`Username`),
UNIQUE KEY `Email` (`Email`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8 AUTO_INCREMENT=4 ;
INSERT INTO `member` VALUES (1, 'weerachai', 'weerachai@1', 'Weerachai Nukitram', '0819876107', '[email protected]');
INSERT INTO `member` VALUES (2, 'adisorn', 'adisorn@2', 'Adisorn Bunsong', '021978032', '[email protected]');
INSERT INTO `member` VALUES (3, 'surachai', 'surachai@3', 'Surachai Sirisart', '0876543210', '[email protected]');
เรียกใช้ NameSpace ไว้จัดการกับ MySQL Database
using System.Data;
using Newtonsoft.Json;
using MySql.Data.MySqlClient;
Method CheckUsernamePassword() บน Web Services ทำหน้าที่ตรวจสอบ Username และ Password
[WebMethod]
public string CheckUsernamePassword(string sUsername,string sPassword)
{
MySqlConnection objConn = new MySqlConnection();
MySqlCommand objCmd = new MySqlCommand();
MySqlDataAdapter dtAdapter = new MySqlDataAdapter();
DataSet ds = new DataSet();
DataTable dt;
String strConnString, strSQL;
strConnString = "Server=localhost;User Id=root; Password=root; Database=mydatabase; Pooling=false";
strSQL = "SELECT * FROM member WHERE Username = '" + sUsername + "' AND Password = '" + sPassword + "' ";
objConn.ConnectionString = strConnString;
objCmd.Connection = objConn;
objCmd.CommandText = strSQL;
objCmd.CommandType = CommandType.Text;
dtAdapter.SelectCommand = objCmd;
dtAdapter.Fill(ds);
dt = ds.Tables[0];
dtAdapter = null;
objConn.Close();
objConn = null;
if (dt.Rows.Count == 0)
{
return "0"; // Login Failed
}
else
{
return "1"; // Login Complete
}
}
ตรวจสอบสถานะการ Login โดยถ้า 0 = Login ไม่ผ่าน และ 1 = Login ผ่าน
Method getMemberByUsername() บน Web Services ซึ่งจะเลือกข้อมูลจาก Username จาก MySQL แล้วส่งกลับเป็น JSON
[WebMethod]
public string getMemberByUsername(string sUsername)
{
MySqlConnection objConn = new MySqlConnection();
MySqlCommand objCmd = new MySqlCommand();
MySqlDataAdapter dtAdapter = new MySqlDataAdapter();
DataSet ds = new DataSet();
DataTable dt;
String strConnString, strSQL;
strConnString = "Server=localhost;User Id=root; Password=root; Database=mydatabase; Pooling=false";
strSQL = "SELECT * FROM member WHERE Username ='" + sUsername + "'";
objConn.ConnectionString = strConnString;
objCmd.Connection = objConn;
objCmd.CommandText = strSQL;
objCmd.CommandType = CommandType.Text;
dtAdapter.SelectCommand = objCmd;
dtAdapter.Fill(ds);
dt = ds.Tables[0];
dtAdapter = null;
objConn.Close();
objConn = null;
string json = JsonConvert.SerializeObject(dt, Formatting.Indented);
return json;
}
สำหรับ method นี้ จะใช้สำหรับการแสดง Profile บนหน้าจอ Windows Store Apps
รูปแบบการส่ง Parameters และการอ่านค่า JSON ฝั่ง Windows Store Apps (C#)
สำหรับการตรวจสอบ Username และ Password
var client = new myWebServices.myWSVSoapClient();
var result = await client.CheckUsernamePasswordAsync(this.txtUsername.Text, this.txtPassword.Password.ToString());
string jsonData = result.Body.CheckUsernamePasswordResult;
if (jsonData == "0")
{
MessageDialog msgDialog = new MessageDialog("Username or Password Incorrect!", "Error");
await msgDialog.ShowAsync();
}
else
{
this.Frame.Navigate(typeof(InfoPage), this.txtUsername.Text);
}
การอ่านข้อมูลมาแสดงในหน้า Profile
var client = new myWebServices.myWSVSoapClient();
var result = await client.getMemberByUsernameAsync(sUsername);
string jsonData = result.Body.getMemberByUsernameResult;
MemoryStream ms = new MemoryStream(Encoding.UTF8.GetBytes(jsonData));
ObservableCollection<myMember> list = new ObservableCollection<myMember>();
DataContractJsonSerializer serializer = new DataContractJsonSerializer(typeof(ObservableCollection<myMember>));
list = (ObservableCollection<myMember>)serializer.ReadObject(ms);
myMember customer = list.First();
this.lblMemberID.Text = customer.MemberID;
this.lblUsername.Text = customer.Username;
this.lblName.Text = customer.Name;
this.lblTel.Text = customer.Tel;
this.lblEmail.Text = customer.Email;
Example การเขียน Windows Store Apps เพื่อทำรระบบ Login แบบตรวจสอบ Username/Password บน Web Services
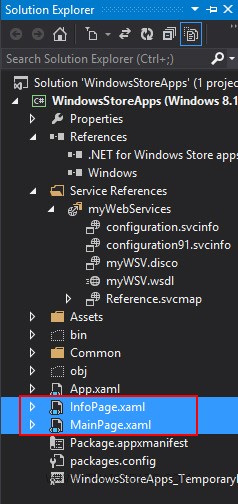
ใน Project ประกอบด้วย 2 Page คือ MainPage.xaml และ InfoPage.xaml
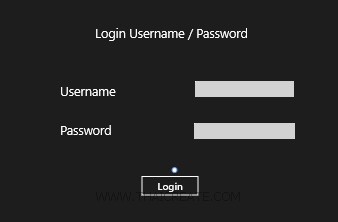
ใน MainPage.xaml ออกแบบหน้าจอประกอบด้วย TextBlock , TexBox และ Button สำหรับรับค่า Username/Password และส่งไปตรวจสอบที่ Web Services
MainPage.xaml
<Page
x:Class="WindowsStoreApps.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:WindowsStoreApps"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d">
<Grid Background="{ThemeResource ApplicationPageBackgroundThemeBrush}">
<TextBlock HorizontalAlignment="Left" Margin="524,98,0,0" TextWrapping="Wrap" Text="Login Username / Password" VerticalAlignment="Top" FontSize="25"/>
<TextBlock HorizontalAlignment="Left" Margin="454,214,0,0" TextWrapping="Wrap" Text="Username" VerticalAlignment="Top" FontSize="25"/>
<TextBox x:Name="txtUsername" HorizontalAlignment="Left" Margin="724,206,0,0" TextWrapping="Wrap" VerticalAlignment="Top" Width="198" FontSize="20" FontFamily="Global User Interface"/>
<TextBlock HorizontalAlignment="Left" Margin="454,292,0,0" TextWrapping="Wrap" Text="Password" VerticalAlignment="Top" FontSize="25"/>
<PasswordBox x:Name="txtPassword" HorizontalAlignment="Left" Margin="722,290,0,0" VerticalAlignment="Top" Width="202" FontFamily="Global User Interface" FontSize="20"/>
<Button x:Name="btnLogin" Content="Login" HorizontalAlignment="Left" Margin="614,393,0,0" VerticalAlignment="Top" FontSize="20" Width="120" FontFamily="Global User Interface" Click="btnLogin_Click"/>
</Grid>
</Page>
MainPage.xaml.cs
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using Windows.Devices.Geolocation;
using Windows.Foundation;
using Windows.Foundation.Collections;
using Windows.UI.Core;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml.Controls.Primitives;
using Windows.UI.Xaml.Data;
using Windows.UI.Xaml.Input;
using Windows.UI.Xaml.Media;
using Windows.UI.Xaml.Navigation;
using WindowsStoreApps.myWebServices;
using Windows.UI.Popups;
// The Blank Page item template is documented at http://go.microsoft.com/fwlink/?LinkId=234238
namespace WindowsStoreApps
{
/// <summary>
/// An empty page that can be used on its own or navigated to within a Frame.
/// </summary>
///
public sealed partial class MainPage : Page
{
public MainPage()
{
this.InitializeComponent();
}
private async void btnLogin_Click(object sender, RoutedEventArgs e)
{
var client = new myWebServices.myWSVSoapClient();
var result = await client.CheckUsernamePasswordAsync(this.txtUsername.Text, this.txtPassword.Password.ToString());
string jsonData = result.Body.CheckUsernamePasswordResult;
if (jsonData == "0")
{
MessageDialog msgDialog = new MessageDialog("Username or Password Incorrect!", "Error");
await msgDialog.ShowAsync();
}
else
{
this.Frame.Navigate(typeof(InfoPage), this.txtUsername.Text);
}
}
}
}
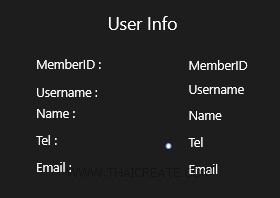
ใน InfoPage.xaml ออกแบบหน้าจอประกอบด้วย TextBlock สำหรับแสดงรายการข้อมูล Profile จาก Web Services
InfoPage.xaml
<Page
x:Class="WindowsStoreApps.InfoPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:WindowsStoreApps"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d">
<Grid Background="{ThemeResource ApplicationPageBackgroundThemeBrush}">
<Grid x:Name="ContentPanel" Margin="12,2,12,-2">
<TextBlock HorizontalAlignment="Left" Margin="549,121,0,0" TextWrapping="Wrap" Text="User Info" VerticalAlignment="Top" FontSize="35"/>
<TextBlock HorizontalAlignment="Left" Margin="406,207,0,0" TextWrapping="Wrap" Text="MemberID :" VerticalAlignment="Top" FontSize="25"/>
<TextBlock x:Name="lblMemberID" HorizontalAlignment="Left" Height="36" Margin="711,210,0,0" Text="MemberID" TextWrapping="Wrap" VerticalAlignment="Top" Width="222" FontSize="25"/>
<TextBlock HorizontalAlignment="Left" Margin="406,264,0,0" TextWrapping="Wrap" Text="Username :" VerticalAlignment="Top" FontSize="25"/>
<TextBlock x:Name="lblUsername" HorizontalAlignment="Left" Height="36" Margin="711,258,0,0" Text="Username" TextWrapping="Wrap" VerticalAlignment="Top" Width="319" FontSize="25"/>
<TextBlock HorizontalAlignment="Left" Margin="406,306,0,0" TextWrapping="Wrap" Text="Name :" VerticalAlignment="Top" FontSize="25"/>
<TextBlock x:Name="lblName" HorizontalAlignment="Left" Height="36" Margin="711,309,0,0" Text="Name" TextWrapping="Wrap" VerticalAlignment="Top" Width="326" FontSize="25"/>
<TextBlock HorizontalAlignment="Left" Margin="406,359,0,0" TextWrapping="Wrap" Text="Tel :" VerticalAlignment="Top" FontSize="25"/>
<TextBlock x:Name="lblTel" HorizontalAlignment="Left" Height="36" Margin="711,363,0,0" Text="Tel" TextWrapping="Wrap" VerticalAlignment="Top" Width="326" FontSize="25"/>
<TextBlock HorizontalAlignment="Left" Margin="406,413,0,0" TextWrapping="Wrap" Text="Email :" VerticalAlignment="Top" FontSize="25"/>
<TextBlock x:Name="lblEmail" HorizontalAlignment="Left" Height="36" Margin="711,417,0,0" Text="Email" TextWrapping="Wrap" VerticalAlignment="Top" Width="326" FontSize="25"/>
</Grid>
</Grid>
</Page>
InfoPage.xaml.cs
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using System.Runtime.InteropServices.WindowsRuntime;
using Windows.Foundation;
using Windows.Foundation.Collections;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml.Controls.Primitives;
using Windows.UI.Xaml.Data;
using Windows.UI.Xaml.Input;
using Windows.UI.Xaml.Media;
using Windows.UI.Xaml.Navigation;
using WindowsStoreApps.myWebServices;
using System.Xml.Linq;
using System.Runtime.Serialization;
using System.Runtime.Serialization.Json;
using System.Collections.ObjectModel;
using System.Text;
// The Blank Page item template is documented at http://go.microsoft.com/fwlink/?LinkId=234238
namespace WindowsStoreApps
{
/// <summary>
/// An empty page that can be used on its own or navigated to within a Frame.
/// </summary>
public sealed partial class InfoPage : Page
{
public InfoPage()
{
this.InitializeComponent();
}
public class myMember
{
public string MemberID { get; set; }
public string Username { get; set; }
public string Name { get; set; }
public string Tel { get; set; }
public string Email { get; set; }
}
string sUsername = string.Empty;
protected async override void OnNavigatedTo(NavigationEventArgs e)
{
sUsername = e.Parameter as string;
var client = new myWebServices.myWSVSoapClient();
var result = await client.getMemberByUsernameAsync(sUsername);
string jsonData = result.Body.getMemberByUsernameResult;
MemoryStream ms = new MemoryStream(Encoding.UTF8.GetBytes(jsonData));
ObservableCollection<myMember> list = new ObservableCollection<myMember>();
DataContractJsonSerializer serializer = new DataContractJsonSerializer(typeof(ObservableCollection<myMember>));
list = (ObservableCollection<myMember>)serializer.ReadObject(ms);
myMember customer = list.First();
this.lblMemberID.Text = customer.MemberID;
this.lblUsername.Text = customer.Username;
this.lblName.Text = customer.Name;
this.lblTel.Text = customer.Tel;
this.lblEmail.Text = customer.Email;
}
}
}
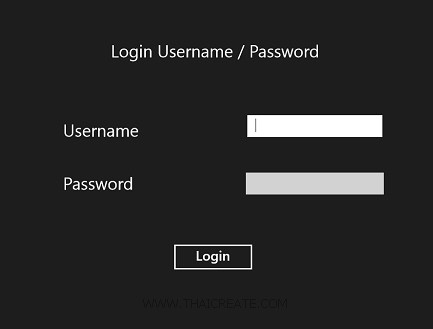
แสดงหน้าจอ Login ตรวจสอบ Username และ Password
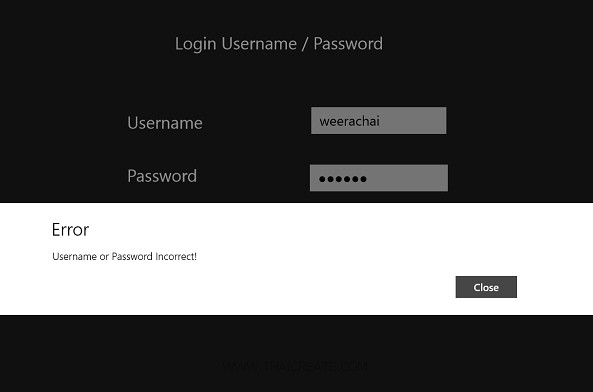
ในกรณีที่ Login ไม่ผ่านหรือไม่ถูกต้องจะแสดง Error ดังรูป
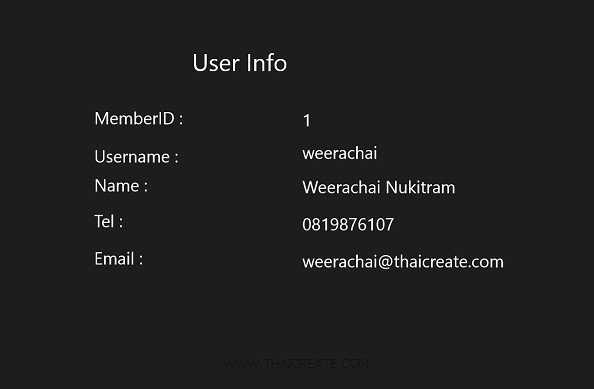
ในกรณีที่ Login ถูกต้อง จะแสดงหน้า User Profile
.
|