 |
|
ช่วยหน่อยคะ Update Database ด่วนๆๆๆๆๆๆๆๆ ขอความช่วยเหลือหน่อยเหลืออีกนิดเดียวแล้ว |
|
 |
|
|
 |
 |
|
ขอความช่วยเหลือหน่อย
เหลืออีกนิดเดียวแล้ว คือ ในส่วนที่ว่าจะให้อัพเดตรหัสผ่านได้เฉพาะแถวของไอดีที่เราป้อนไปเท่านั้น
ขอบคุณล่วงหน้าค๊า
Code (C#)
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using System.Data.SqlClient;
namespace NewProjectClient
{
public partial class ChangePassword : Form
{
public ChangePassword()
{
InitializeComponent();
}
private void button2_Click(object sender, EventArgs e)
{
Showdata f = new Showdata();
f.Show();
this.Hide();
}
private void ClearPassword()
{
txtID.Text = "";
txtPasswd.Text = "";
txtPasswd1.Text = "";
txtPasswd2.Text = "";
txtID.Focus();
}
private void button1_Click(object sender, EventArgs e)
{
string StrConn = "Data Source=.\\SQLExpress;Initial Catalog=Server;Integrated Security=True";
if (txtID.Text.Trim() == "" || txtPasswd.Text.Trim() == "" || txtPasswd1.Text.Trim() == "" || txtPasswd2.Text.Trim() == "")
{
MessageBox.Show("กรุณาป้อนข้อมูลให้ครบด้วยคะ", "ผลการตรวจสอบ", MessageBoxButtons.OK, MessageBoxIcon.Exclamation);
ClearPassword();
return;
}
if (txtPasswd1.Text.Length < 4 || txtPasswd2.Text.Length < 4)
{
MessageBox.Show("รหัสผ่านต้องมีจำนวนตัวอักษรระหว่าง 4-16 ตัวอักษร !!!", "ผลการตรวจสอบ", MessageBoxButtons.OK, MessageBoxIcon.Exclamation);
ClearPassword();
return;
}
if (txtPasswd.Text.Trim() == txtPasswd1.Text.Trim())
{
MessageBox.Show("รหัสผ่านเก่ากับใหม่ ห้ามเหมือนกัน !!!", "ผลการตรวจสอบ", MessageBoxButtons.OK, MessageBoxIcon.Exclamation);
ClearPassword();
return;
}
if (txtPasswd1.Text.Trim() != txtPasswd2.Text.Trim())
{
MessageBox.Show("คุณป้อนรหัสรหัสผ่านไม่เหมือนกัน กรุณาป้อนใหม่ !!!", "ผลการตรวจสอบ", MessageBoxButtons.OK, MessageBoxIcon.Exclamation);
ClearPassword();
return;
}
if (MessageBox.Show("คุณต้องการแก้ไขรหัสผ่านใช่หรือไม่?", "คำยืนยัน", MessageBoxButtons.YesNo, MessageBoxIcon.Question) == System.Windows.Forms.DialogResult.Yes)
{
string qry = @"select * from user1 ";
string upd = @"update user1 set Password = @Password where IDUser = @IDUser";
SqlConnection Conn = new SqlConnection(StrConn);
Conn.Open();
try
{
SqlDataAdapter da = new SqlDataAdapter();
da.SelectCommand = new SqlCommand(qry, Conn);
DataSet ds = new DataSet();
da.Fill(ds, "user1");
DataTable dt = ds.Tables["user1"];
int countrows = ds.Tables[0].Rows.Count; //นับจำนวน Rows ทั้งหมดมีกี่ แถว
int countcolumns = ds.Tables[0].Columns.Count;
for (int y = 0; y < countrows; y++)
{
string id = ds.Tables[0].Rows[y].ToString(); // ค้นหาแต่ล่ะ Row
txtID.Text = id.ToString();
dt.Rows[y]["Password"] = txtPasswd1.Text.Trim();
}
// dt.Rows[y]["Password"] = txtPasswd1.Text.Trim();
foreach (DataRow row in dt.Rows)
{
Console.WriteLine(
"{0}",
row["Password"].ToString().PadRight(15));
}
// Update user1
SqlCommand cmd = new SqlCommand(upd, Conn);
cmd.Parameters.Add("@Password", SqlDbType.NVarChar, 15, "Password").Value = txtPasswd.Text.Trim();
SqlParameter parm = cmd.Parameters.Add("@IDUser", SqlDbType.NVarChar, 15, "IDUser");
parm.SourceVersion = DataRowVersion.Original;
da.UpdateCommand = cmd;
da.Update(ds, "user1");
Showdata f = new Showdata();
f.Show();
this.Hide();
}
catch (Exception ex)
{
MessageBox.Show("ไม่สามารถแก้ไขรหัสผ่านได้เนื่องจาก " + ex.Message, "ผลการตรวจสอบ", MessageBoxButtons.OK, MessageBoxIcon.Exclamation);
// tr.Rollback();
}
finally
{
Conn.Close();
}
}
}
}
}
Tag : - - - -
|
|
 |
 |
 |
 |
Date :
2009-04-18 14:54:31 |
By :
Database |
View :
1707 |
Reply :
5 |
|
 |
 |
 |
 |
|
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
Capture หน้า Screen มาให้ดูหน่อยก็ดีครับ 
|
 |
 |
 |
 |
Date :
2009-04-18 15:39:38 |
By :
webmaster |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
รูปฟอร์ม
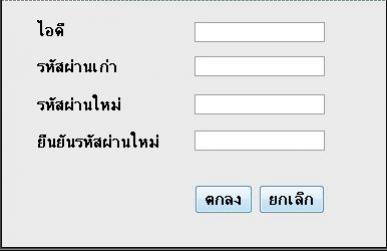
สมมุตข้อมูลไอดีที่ป้อนเป็น 10000001
รหัสผ่านเดิม 10000001
รหัสผ่านใหม่เป็น 1000000
ยืนยันรหัสผ่านใหม่เป็น 10000000
ฐานข้อมูลแรก ตอนที่ยังไม่เปลี่ยนรหัสผ่าน
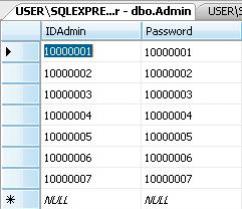
ฐานข้อมูลตอนเปลี่ยนรหัสผ่านแล้ว
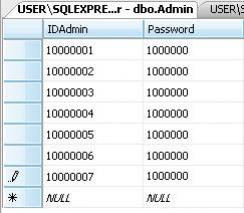
capture มาให้แล้วค๊า....ช่วยหน่อยน๊าคะ..ขอร้องด่วนจิงๆๆๆๆๆๆๆๆๆๆๆ]
|
 |
 |
 |
 |
Date :
2009-04-18 15:58:20 |
By :
Database |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ลองแล้วคะถ้ากำหนดค่าแล้วมัน error คะ
รูปภาพ
|
 |
 |
 |
 |
Date :
2009-04-18 18:52:24 |
By :
Database |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ถ้าคุณใช้ Stored Proceduer คุณไม่ได้ประกาศ@IDUser ใน Stored Proceduer
เช่น @IDuser int; เป็นต้น
|
 |
 |
 |
 |
Date :
2009-04-20 09:28:56 |
By :
sspt |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
|
|