 |
|
Code (VB.NET)
<%@ Import Namespace="System.Data"%>
<%@ Import Namespace="System.Data.OleDb"%>
<%@ Page Language="VB" %>
<script runat="server">
Sub Page_Load(sender As Object, e As EventArgs)
IF Not Page.IsPostBack() Then
CheckBoxListDataTable()
CheckBoxListDataTableRows()
CheckBoxListSortedList()
CheckBoxListAddInsertItem()
End IF
End Sub
'*** CheckBoxList & DataTable ***'
Function CheckBoxListDataTable()
Dim objConn As OleDbConnection
Dim dtAdapter As OleDbDataAdapter
Dim dt As New DataTable
Dim strConnString As String
strConnString = "Provider=Microsoft.Jet.OLEDB.4.0;Data Source="& _
Server.MapPath("database/mydatabase.mdb")&";"
objConn = New OleDbConnection(strConnString)
objConn.Open()
Dim strSQL As String
strSQL = "SELECT * FROM country"
dtAdapter = New OleDbDataAdapter(strSQL, objConn)
dtAdapter.Fill(dt)
dtAdapter = Nothing
objConn.Close()
objConn = Nothing
'*** CheckBoxList ***'
With Me.myCBoxList1
.DataSource = dt
.DataTextField = "CountryName"
.DataValueField = "CountryCode"
.DataBind()
End With
'*** Default Value ***'
myCBoxList1.SelectedIndex = myCBoxList1.Items.IndexOf(myCBoxList1.Items.FindByValue("TH")) '*** By DataValueField ***'
'myCBoxList1.SelectedIndex = myCBoxList1.Items.IndexOf(myCBoxList1.Items.FindByText("Thailand")) '*** By DataTextField ***'
End Function
'*** CheckBoxList & TableRows ***'
Sub CheckBoxListDataTableRows()
Dim dt As New DataTable
Dim dr As DataRow
'*** Column ***'
dt.Columns.Add("Sex")
dt.Columns.Add("SexDesc")
'*** Rows ***'
dr = dt.NewRow
dr("Sex") = "M"
dr("SexDesc") = "Man"
dt.Rows.Add(dr)
'*** Rows ***'
dr = dt.NewRow
dr("Sex") = "W"
dr("SexDesc") = "Woman"
dt.Rows.Add(dr)
'*** CheckBoxList ***'
With Me.myCBoxList2
.DataSource = dt
.DataTextField = "SexDesc"
.DataValueField = "Sex"
.DataBind()
End With
'*** Default Value ***'
myCBoxList2.SelectedIndex = myCBoxList2.Items.IndexOf(myCBoxList2.Items.FindByValue("W")) '*** By DataValueField ***'
'myCBoxList2.SelectedIndex = myCBoxList2.Items.IndexOf(myCBoxList2.Items.FindByText("Woman")) '*** By DataTextField ***'
End Sub
'*** CheckBoxList & SortedList ***'
Sub CheckBoxListSortedList()
Dim mySortedList AS New SortedList
mySortedList.Add("M","Man")
mySortedList.Add("W","Woman")
'*** CheckBoxList ***'
With Me.myCBoxList3
.DataSource = mySortedList
.DataTextField = "Value"
.DataValueField = "Key"
.DataBind()
End With
'*** Default Value ***'
myCBoxList3.SelectedIndex = myCBoxList3.Items.IndexOf(myCBoxList3.Items.FindByValue("W")) '*** By DataValueField ***'
'myCBoxList3.SelectedIndex = myCBoxList3.Items.IndexOf(myCBoxList3.Items.FindByText("Woman")) '*** By DataTextField ***'
End Sub
'*** Add/Insert Items ***'
Sub CheckBoxListAddInsertItem()
Dim mySortedList AS New SortedList
mySortedList.Add("M","Man")
mySortedList.Add("W","Woman")
'*** CheckBoxList ***'
With Me.myCBoxList4
.DataSource = mySortedList
.DataTextField = "Value"
.DataValueField = "Key"
.DataBind()
End With
'*** Add & Insert New Item ***'
Dim strText,strValue As String
'*** Insert Item ***'
strText = ""
strValue = ""
Dim InsertItem As New ListItem(strText, strValue)
myCBoxList4.Items.Insert(0, InsertItem)
'*** Add Items ***'
strText = "Guy"
strValue = "G"
Dim AddItem As New ListItem(strText, strValue)
myCBoxList4.Items.Add(AddItem)
'*** Default Value ***'
myCBoxList4.SelectedIndex = myCBoxList4.Items.IndexOf(myCBoxList4.Items.FindByValue("W")) '*** By DataValueField ***'
'myCBoxList4.SelectedIndex = myCBoxList4.Items.IndexOf(myCBoxList4.Items.FindByText("Woman")) '*** By DataTextField ***'
End Sub
Sub Button1_OnClick(sender as Object, e As EventArgs)
Dim i As Integer
Me.lblText1.Text = ""
For i = 0 To Me.myCBoxList1.Items.Count - 1
If Me.myCBoxList1.Items(i).Selected Then
Me.lblText1.Text = Me.lblText1.Text & ","&Me.myCBoxList1.Items(i).Value '*** Or Me.myCBoxList1.Items(i).Text ***'
End If
Next
Me.lblText2.Text = ""
For i = 0 To Me.myCBoxList2.Items.Count - 1
If Me.myCBoxList2.Items(i).Selected Then
Me.lblText2.Text = Me.lblText2.Text & ","&Me.myCBoxList2.Items(i).Value '*** Or Me.myCBoxList2.Items(i).Text ***'
End If
Next
Me.lblText3.Text = ""
For i = 0 To Me.myCBoxList3.Items.Count - 1
If Me.myCBoxList3.Items(i).Selected Then
Me.lblText3.Text = Me.lblText3.Text & ","&Me.myCBoxList3.Items(i).Value '*** Or Me.myCBoxList3.Items(i).Text ***'
End If
Next
Me.lblText4.Text = ""
For i = 0 To Me.myCBoxList4.Items.Count - 1
If Me.myCBoxList4.Items(i).Selected Then
Me.lblText4.Text = Me.lblText4.Text & ","&Me.myCBoxList4.Items(i).Value '*** Or Me.myCBoxList4.Items(i).Text ***'
End If
Next
End Sub
</script>
<html>
<head>
<title>ThaiCreate.Com ASP.NET - CheckBoxList & DataBind</title>
</head>
<body>
<form id="form1" runat="server">
<asp:CheckBoxList id="myCBoxList1" runat="server"></asp:CheckBoxList><hr />
<asp:CheckBoxList id="myCBoxList2" runat="server"></asp:CheckBoxList><hr />
<asp:CheckBoxList id="myCBoxList3" runat="server"></asp:CheckBoxList><hr />
<asp:CheckBoxList id="myCBoxList4" runat="server"></asp:CheckBoxList>
<asp:Button id="Button1" onclick="Button1_OnClick" runat="server" Text="Button"></asp:Button>
<hr />
<asp:Label id="lblText1" runat="server"></asp:Label><br /><br />
<asp:Label id="lblText2" runat="server"></asp:Label><br /><br />
<asp:Label id="lblText3" runat="server"></asp:Label><br /><br />
<asp:Label id="lblText4" runat="server"></asp:Label><br /><br />
</form>
</body>
</html>
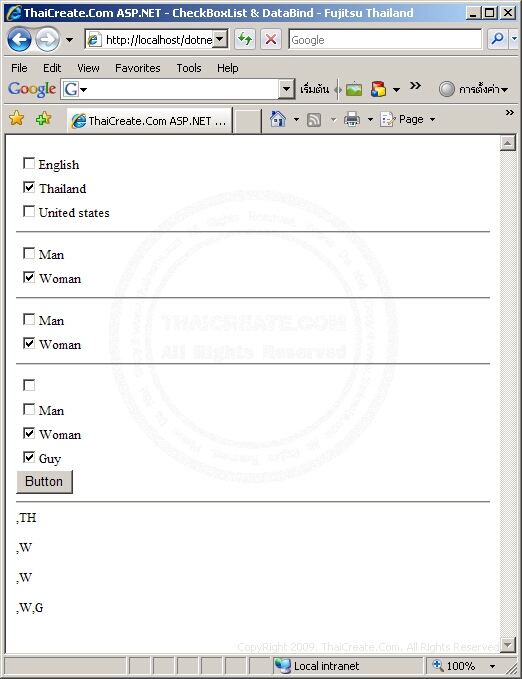
เลือกได้ตามสบายเลยครับ ได้หลายรูปแบบ
|
 |
 |
 |
 |
Date :
2010-01-25 15:22:54 |
By :
webmaster |
|
 |
 |
 |
 |
|
|
 |