 |
|
มาดู SqlDatabaseManager New Version ก่อนเอาไปลงไว้ใน Community |
|
 |
|
|
 |
 |
|
Basic Query แบบบ้านๆ
Code (C#)
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="TestSqlConnection.aspx.cs" Inherits="TestSqlConnection" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
</head>
<body>
<form id="form1" runat="server">
<div>
<asp:Label ID="Label1" runat="server" Text=""></asp:Label>
<br />
<br />
<asp:GridView ID="GridView1" runat="server">
</asp:GridView>
</div>
</form>
</body>
</html>
Code (C#)
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Data;
public partial class TestSqlConnection : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
SqlDatabaseManager SqlDatabaseManager1 = new SqlDatabaseManager();
SqlDatabaseManager1.CommandString = "Select [DayID], [DayName] From [DayTable]";
DataTable Dt = new DataTable();
Dt = SqlDatabaseManager1.ExecuteQuery();
GridView1.DataSource = Dt;
GridView1.DataBind();
Label1.Text = SqlDatabaseManager1.Message;
}
}
ผลลัพธ์
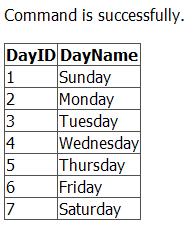
|
 |
 |
 |
 |
Date :
2010-04-07 11:59:21 |
By :
tungman |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
Query แบบมีเงื่อนไขนิดๆ
Code (C#)
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="TestSqlConnection.aspx.cs" Inherits="TestSqlConnection" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
</head>
<body>
<form id="form1" runat="server">
<div>
<asp:Label ID="Label1" runat="server" Text=""></asp:Label>
<br />
<br />
<asp:GridView ID="GridView1" runat="server">
</asp:GridView>
</div>
</form>
</body>
</html>
Code (C#)
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Data;
public partial class TestSqlConnection : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
SqlDatabaseManager SqlDatabaseManager1 = new SqlDatabaseManager();
SqlDatabaseManager1.CommandString = "Select [DayID], [DayName] From [DayTable] Where [DayName] Like @DayName Or [DayID]=@DayID";
SqlDatabaseManager1.AddParameter("@DayName", "Wed%");
SqlDatabaseManager1.AddParameter("@DayID", 2);
DataTable Dt = new DataTable();
Dt = SqlDatabaseManager1.ExecuteQuery();
GridView1.DataSource = Dt;
GridView1.DataBind();
Label1.Text = SqlDatabaseManager1.Message;
}
}
ผลลัพธ์
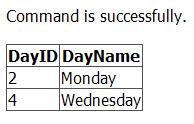
|
 |
 |
 |
 |
Date :
2010-04-07 11:59:50 |
By :
tungman |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
Query โดยใช้ Datetime แบบ String (ใช้ native command 'SmallDateTime()' หรือ 'DateTime()' ช่วย แต่ได้แค่ format เดียวคือ 'd/M/yyyy')
Code (C#)
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="TestSqlConnection.aspx.cs" Inherits="TestSqlConnection" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
</head>
<body>
<form id="form1" runat="server">
<div>
<asp:Label ID="Label1" runat="server" Text=""></asp:Label>
<br />
<br />
<asp:GridView ID="GridView1" runat="server">
</asp:GridView>
</div>
</form>
</body>
</html>
Code (C#)
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Data;
public partial class TestSqlConnection : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
SqlDatabaseManager SqlDatabaseManager1 = new SqlDatabaseManager();
SqlDatabaseManager1.CommandString = "Select [HolidayTable].[HolidayID], [DayTable].[DayName], [HolidayTable].[Holiday], [HolidayTable].[HolidayThaiDetail], [HolidayTable].[HolidayDetail] From [HolidayTable] Inner Join [DayTable] On ([HolidayTable].[DayID] = [DayTable].[DayID]) Where [HolidayTable].[Holiday]=SmallDateTime(@MyDate)";
SqlDatabaseManager1.AddParameter("@MyDate", "13/4/2010");
DataTable Dt = new DataTable();
Dt = SqlDatabaseManager1.ExecuteQuery();
GridView1.DataSource = Dt;
GridView1.DataBind();
Label1.Text = SqlDatabaseManager1.Message;
}
}
ผลลัพธ์

|
 |
 |
 |
 |
Date :
2010-04-07 12:00:14 |
By :
tungman |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
Query โดยใช้ Datetime แบบ DateTime Type (แนะนำ)
Code (C#)
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="TestSqlConnection.aspx.cs" Inherits="TestSqlConnection" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
</head>
<body>
<form id="form1" runat="server">
<div>
<asp:Label ID="Label1" runat="server" Text=""></asp:Label>
<br />
<br />
<asp:GridView ID="GridView1" runat="server">
</asp:GridView>
</div>
</form>
</body>
</html>
Code (C#)
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Data;
public partial class TestSqlConnection : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
SqlDatabaseManager SqlDatabaseManager1 = new SqlDatabaseManager();
SqlDatabaseManager1.CommandString = "Select [HolidayTable].[HolidayID], [DayTable].[DayName], [HolidayTable].[Holiday], [HolidayTable].[HolidayThaiDetail], [HolidayTable].[HolidayDetail] From [HolidayTable] Inner Join [DayTable] On ([HolidayTable].[DayID] = [DayTable].[DayID]) Where [HolidayTable].[Holiday]=@MyDate";
SqlDatabaseManager1.AddParameter("@MyDate", SqlConvert.ToDateTime("13/4/2010"));
DataTable Dt = new DataTable();
Dt = SqlDatabaseManager1.ExecuteQuery();
GridView1.DataSource = Dt;
GridView1.DataBind();
Label1.Text = SqlDatabaseManager1.Message;
}
}
ผลลัพธ์

|
 |
 |
 |
 |
Date :
2010-04-07 12:00:42 |
By :
tungman |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
การใช้ ExecuteScalar()
Code (C#)
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="TestSqlConnection.aspx.cs" Inherits="TestSqlConnection" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
</head>
<body>
<form id="form1" runat="server">
<div>
<asp:Label ID="Label1" runat="server" Text=""></asp:Label>
<br />
<br />
<asp:Label ID="Label2" runat="server" Text="Label"></asp:Label>
</div>
</form>
</body>
</html>
Code (C#)
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
public partial class TestSqlConnection : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
SqlDatabaseManager SqlDatabaseManager1 = new SqlDatabaseManager();
SqlDatabaseManager1.CommandString = "Select Count(*) From [HolidayTable]";
int AllHoliday = (int)SqlDatabaseManager1.ExecuteScalar();
Label1.Text = SqlDatabaseManager1.Message;
Label2.Text = "All Holiday in 2010: " + AllHoliday + " Days";
}
}
ผลลัพธ์
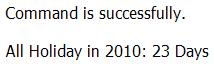
|
 |
 |
 |
 |
Date :
2010-04-07 12:01:10 |
By :
tungman |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
การใช้งาน ExecuteNonQuery() เพื่อ upload ไฟล์ไปเก็บไว้ใน Sql Server
Code (C#)
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="UploadFile.aspx.cs" Inherits="UploadFile" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
</head>
<body>
<form id="form1" runat="server">
<div>
<asp:FileUpload ID="FileUpload1" runat="server" />
<asp:RequiredFieldValidator ID="RequiredFieldValidator1" runat="server" ErrorMessage="*" ControlToValidate="FileUpload1"></asp:RequiredFieldValidator>
<asp:Button ID="Button1" runat="server" Text="Button" />
<br />
<asp:Label ID="Label1" runat="server" Text="Label"></asp:Label>
<br />
<asp:Label ID="Label2" runat="server" Text="Label"></asp:Label>
</div>
</form>
</body>
</html>
Code (C#)
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
public partial class UploadFile : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
Button1.Click += new EventHandler(Button1_Click);
}
protected void Button1_Click(object sender, EventArgs e)
{
SqlDatabaseManager SqlDatabaseManager1 = new SqlDatabaseManager();
SqlDatabaseManager1.CommandString = "Insert Into [UploadFile] ([FileName], [ContentType], [BinaryStream]) Values (@FileName, @ContentType, @BinaryStream)";
SqlDatabaseManager1.AddParameter("@FileName", FileUpload1.PostedFile.FileName);
SqlDatabaseManager1.AddParameter("@ContentType", FileUpload1.PostedFile.ContentType);
SqlDatabaseManager1.AddParameter("@BinaryStream", SqlConvert.ToVarBinary(FileUpload1.PostedFile.InputStream, FileUpload1.PostedFile.ContentLength));
SqlDatabaseManager1.ExecuteNonQuery();
Label1.Text = SqlDatabaseManager1.Message;
Label2.Text = "Insert " + SqlDatabaseManager1.RowsAffected + " Row(s).";
}
}
ผลลัพธ์

|
 |
 |
 |
 |
Date :
2010-04-07 12:01:36 |
By :
tungman |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
การใช้งาน transaction ทำการ upload ไฟล์ไปเก็บไว้ใน Sql Server และเก็บวันที่ upload ไว้อีก table นึง
Code (C#)
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="UploadFile.aspx.cs" Inherits="UploadFile" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
</head>
<body>
<form id="form1" runat="server">
<div>
<asp:FileUpload ID="FileUpload1" runat="server" />
<asp:RequiredFieldValidator ID="RequiredFieldValidator1" runat="server" ErrorMessage="*" ControlToValidate="FileUpload1"></asp:RequiredFieldValidator>
<asp:Button ID="Button1" runat="server" Text="Button" />
<br />
<asp:Label ID="Label1" runat="server" Text="Label"></asp:Label>
<br />
<asp:Label ID="Label2" runat="server" Text="Label"></asp:Label>
<br />
<asp:Label ID="Label3" runat="server" Text="Label"></asp:Label>
<br />
<asp:Label ID="Label4" runat="server" Text="Label"></asp:Label>
</div>
</form>
</body>
</html>
Code (C#)
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Web.Configuration;
using System.Data.SqlClient;
public partial class UploadFile : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
Button1.Click += new EventHandler(Button1_Click);
}
protected void Button1_Click(object sender, EventArgs e)
{
SqlDatabaseManager SqlDatabaseManager1 = new SqlDatabaseManager();
SqlDatabaseManager1.TransactionStart();
//ตรวจสอบก่อน execute
Label1.Text = SqlDatabaseManager1.Message;
SqlDatabaseManager1.CommandString = "Insert Into [UploadFile] ([FileName], [ContentType], [BinaryStream]) Values (@FileName, @ContentType, @BinaryStream)";
SqlDatabaseManager1.AddParameter("@FileName", FileUpload1.PostedFile.FileName);
SqlDatabaseManager1.AddParameter("@ContentType", FileUpload1.PostedFile.ContentType);
SqlDatabaseManager1.AddParameter("@BinaryStream", SqlConvert.ToVarBinary(FileUpload1.PostedFile.InputStream, FileUpload1.PostedFile.ContentLength));
SqlDatabaseManager1.ExecuteNonQuery();
//ตรวจสอบตอนรัน command แรก
Label2.Text = SqlDatabaseManager1.Message;
//สามารถ query ที่ insert ภายใน transaction ได้
SqlDatabaseManager1.CommandString = "Select Max(ID) From [UploadFile]";
int MaxID = (int)SqlDatabaseManager1.ExecuteScalar();
SqlDatabaseManager1.CommandString = "Insert Into [UploadDetail] ([FileID], [UploadDate]) Value (@FileID, @UploadDate)";
SqlDatabaseManager1.AddParameter("@FileID", MaxID);
SqlDatabaseManager1.AddParameter("@UploadDate", DateTime.Today);
SqlDatabaseManager1.ExecuteNonQuery();
//ตรวจสอบตอนรัน command ที่สาม
Label3.Text = SqlDatabaseManager1.Message;
SqlDatabaseManager1.ExecuteTransaction();
//ผลของ transaction
Label4.Text = SqlDatabaseManager1.Message;
}
}
ผลลัพธ์แบบ error เพราะอยากให้ดูแบบ error เพราะ value ไม่ได้เติม s
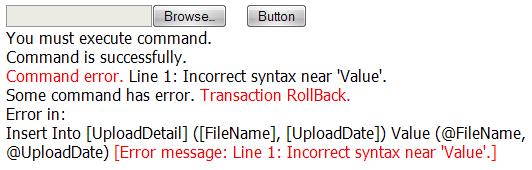
ผลลัพธ์แบบ success แก้ไข values แล้ว
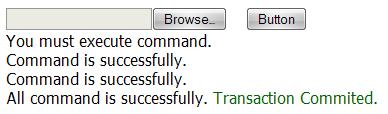
|
 |
 |
 |
 |
Date :
2010-04-07 12:02:11 |
By :
tungman |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ส่วน SqlDatabaseManager ให้ไป download ใน community นะครับ
ในบทความเดิมเลยครับ ที่ SqlDatabaseManager
หรือก็อบ source code ด้านล่างไปก็ได้
SqlDatabaseManager.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Data;
using System.Data.SqlClient;
using System.Globalization;
using System.IO;
using System.Text.RegularExpressions;
using System.Web.Configuration;
#region ========== Class SqlDatabaseManager ==========
/// <summary>
/// Summary description for SqlDatabase
/// </summary>
public class SqlDatabaseManager
{
#region ========== Global Variables ==========
private SqlConnection sqlConnection;
private SqlCommand sqlCommand;
private SqlTransaction sqlTransaction;
private List<string> errorCommand;
private string sqlConnectionString = string.Empty;
private string sqlConnectionKeyName = string.Empty;
private string sqlCommandString = string.Empty;
private string commandMessage = "You must execute command.";
private bool commandSuccess = false;
private bool transaction = false;
private int rowsAffected = 0;
#endregion
#region ========= Constructor ==========
/// <summary>
/// Use sql connection string from web.config configulation.
/// </summary>
public SqlDatabaseManager()
{
if (WebConfigurationManager.ConnectionStrings["SqlConnectionString"] != null)
{
//สำหรับ web.config ที่มีการ add connection string ชื่อ SqlConnectionString เอาไว้
//
//ตัวอย่างใน web.config
//<connectionStrings>
// <add name="SqlConnectionString" connectionString="Data Source=.\SQLEXPRESS;Initial Catalog=SqlDatabase;Integrated Security=True;" providerName="System.Data.SqlClient"/>
//</connectionStrings>
sqlConnectionKeyName = "SqlConnectionString";
sqlConnectionString = WebConfigurationManager.ConnectionStrings["SqlConnectionString"].ToString();
sqlConnection = new SqlConnection(sqlConnectionString);
}
else
{
sqlConnection = new SqlConnection();
}
}
/// <summary>
/// Use sql connection string from web.config configulation by key name.
/// </summary>
public SqlDatabaseManager(string SqlConnectionKeyName)
{
sqlConnectionKeyName = SqlConnectionKeyName;
sqlConnectionString = WebConfigurationManager.ConnectionStrings[sqlConnectionKeyName].ToString();
sqlConnection = new SqlConnection(sqlConnectionString);
}
/// <summary>
/// Use sql connection string by user define.
/// </summary>
public SqlDatabaseManager(string SqlConnectionKeyName, string SqlConnectionString)
{
sqlConnectionKeyName = SqlConnectionKeyName;
sqlConnectionString = SqlConnectionString;
sqlConnection = new SqlConnection(sqlConnectionString);
}
#endregion
#region ========== Property ==========
/// <summary>
/// Gets or sets Sql connection key name from web.config.
/// </summary>
public virtual string ConnectionKeyName
{
get { return sqlConnectionKeyName; }
set
{
sqlConnectionKeyName = value;
sqlConnectionString = WebConfigurationManager.ConnectionStrings[sqlConnectionKeyName].ToString();
sqlConnection = new SqlConnection(sqlConnectionString);
}
}
/// <summary>
/// Gets or sets Sql connection.
/// </summary>
public virtual string ConnectionString
{
get { return sqlConnectionString; }
set
{
sqlConnectionString = value;
sqlConnection = new SqlConnection(sqlConnectionString);
}
}
/// <summary>
/// Gets or sets Sql command.
/// </summary>
public virtual string CommandString
{
get { return sqlCommandString; }
set
{
sqlCommandString = ConvertDateCommand(value);
sqlCommand = new SqlCommand(sqlCommandString, sqlConnection);
commandMessage = "You must execute command.";
commandSuccess = false;
rowsAffected = 0;
if (transaction)
sqlCommand.Transaction = sqlTransaction;
}
}
/// <summary>
/// Check for Sql command.
/// </summary>
public virtual bool IsSuccess
{
get { return commandSuccess; }
}
/// <summary>
/// Gets message from Sql command.
/// </summary>
public virtual string Message
{
get { return commandMessage; }
}
/// <summary>
/// Gets Number of rows affected.
/// </summary>
public virtual int RowsAffected
{
get { return rowsAffected; }
}
#endregion
#region ========== Method ==========
/// <summary>
/// Add the parameter value to the sql command.
/// </summary>
/// <param name="ParameterName">The name of Parameter.</param>
/// <param name="ParameterValue">The value to be added.</param>
public virtual void AddParameter(string ParameterName, object ParameterValue)
{
sqlCommand.Parameters.AddWithValue(ParameterName, ParameterValue);
}
/// <summary>
/// Start Sql Transaction.
/// </summary>
public virtual void TransactionStart()
{
transaction = true;
errorCommand = new List<string>();
if (sqlConnection.State != ConnectionState.Open)
sqlConnection.Open();
sqlTransaction = sqlConnection.BeginTransaction(IsolationLevel.ReadCommitted);
}
/// <summary>
/// Execute Sql Transaction.
/// </summary>
/// <returns>Result of transaction.</returns>
public virtual bool ExecuteTransaction()
{
transaction = false;
if (errorCommand.Count == 0)
{
sqlTransaction.Commit();
commandMessage = "All command is successfully. <font color=\"darkgreen\">Transaction Commited.</font>";
commandSuccess = true;
}
else
{
sqlTransaction.Rollback();
string ErrorText = "Some command has error. <font color=\"red\">Transaction RollBack.</font><br />Error in: <br />";
foreach (string aErrorSqlCommand in errorCommand)
{
ErrorText += aErrorSqlCommand + "<br />";
}
commandMessage = ErrorText;
commandSuccess = false;
}
errorCommand.Clear();
if (sqlConnection.State == ConnectionState.Open)
sqlConnection.Close();
sqlTransaction.Dispose();
sqlCommand.Dispose();
sqlConnection.Dispose();
return commandSuccess;
}
/// <summary>
/// Execute Query Sql command.
/// </summary>
/// <returns>Query data in DataTable.</returns>
public virtual DataTable ExecuteQuery()
{
DataTable dataTable = new DataTable();
try
{
SqlDataAdapter sqlDataAdapter = new SqlDataAdapter(sqlCommand);
sqlDataAdapter.Fill(dataTable);
sqlDataAdapter.Dispose();
if (!transaction)
{
sqlCommand.Dispose();
sqlConnection.Dispose();
}
commandMessage = "Command is successfully.";
commandSuccess = true;
}
catch (Exception ex)
{
commandMessage = ErrorMessage(ex.Message);
commandSuccess = false;
}
rowsAffected = dataTable.Rows.Count;
return dataTable;
}
/// <summary>
/// Execute Scalar Sql command.
/// </summary>
/// <returns>Object of value.</returns>
public virtual object ExecuteScalar()
{
object Result = 0;
try
{
if (transaction)
{
Result = sqlCommand.ExecuteScalar();
}
else
{
if (sqlConnection.State != ConnectionState.Open)
sqlConnection.Open();
Result = sqlCommand.ExecuteScalar();
sqlConnection.Close();
sqlCommand.Dispose();
sqlConnection.Dispose();
}
commandMessage = "Command is successfully.";
commandSuccess = true;
}
catch (Exception ex)
{
commandMessage = ErrorMessage(ex.Message);
commandSuccess = false;
AddErrorCommand(sqlCommandString, ex.Message);
}
return Result;
}
/// <summary>
/// Execute Non Query Sql command.
/// </summary>
/// <returns>Result of execute command.</returns>
public virtual bool ExecuteNonQuery()
{
rowsAffected = 0;
try
{
if (transaction)
{
rowsAffected = sqlCommand.ExecuteNonQuery();
}
else
{
if (sqlConnection.State != ConnectionState.Open)
sqlConnection.Open();
rowsAffected = sqlCommand.ExecuteNonQuery();
sqlConnection.Close();
sqlCommand.Dispose();
sqlConnection.Dispose();
}
commandMessage = "Command is successfully.";
commandSuccess = true;
}
catch (Exception ex)
{
commandMessage = ErrorMessage(ex.Message);
commandSuccess = false;
AddErrorCommand(sqlCommandString, ex.Message);
}
return commandSuccess;
}
/// <summary>
/// Build error message.
/// </summary>
/// <param name="Message">Message string.</param>
/// <returns>Error message string.</returns>
protected virtual string ErrorMessage(string MessageString)
{
return "<font color=\"red\">Command error.</font> " + MessageString;
}
/// <summary>
/// Add error sql command to string collections.
/// </summary>
/// <param name="commandString">The sql command.</param>
/// <param name="errorMessage">The error message.</param>
protected virtual void AddErrorCommand(string commandString, string errorMessage)
{
errorCommand.Add(commandString + " <font color=\"red\">[Error message: " + errorMessage + "]</font>");
}
/// <summary>
/// Convert native command to sql command.
/// </summary>
/// <param name="commandString">The native sql command.</param>
/// <returns>The standard sql command.</returns>
protected virtual string ConvertDateCommand(string commandString)
{
string SmallDateTimePattern = "[sS][mM][aA][lL][lL][dD][aA][tT][eE][tT][iI][mM][eE]\\([@][0-9a-zA-Z\\s]{1,}\\)";
Regex SmallDateTimeRgx = new Regex(SmallDateTimePattern);
foreach (Match SmallDateTimeMatchCase in SmallDateTimeRgx.Matches(commandString))
{
string MatchCasePattern = "^[sS][mM][aA][lL][lL][dD][aA][tT][eE][tT][iI][mM][eE]";
Regex MatchCaseRgx = new Regex(MatchCasePattern);
Match RemoveMatch = MatchCaseRgx.Match(SmallDateTimeMatchCase.Value);
string TempMatchCase = SmallDateTimeMatchCase.Value.Replace(RemoveMatch.Value, "");
commandString = commandString.Replace(SmallDateTimeMatchCase.Value, TempMatchCase.Replace("(", "Convert(SmallDateTime, ").Replace(")", ", 103)"));
}
string DateTimePattern = "[dD][aA][tT][eE][tT][iI][mM][eE]\\([@][0-9a-zA-Z\\s]{1,}\\)";
Regex DateTimeRgx = new Regex(DateTimePattern);
foreach (Match DateTimeMatchCase in DateTimeRgx.Matches(commandString))
{
string MatchCasePattern = "^[dD][aA][tT][eE][tT][iI][mM][eE]";
Regex MatchCaseRgx = new Regex(MatchCasePattern);
Match RemoveMatch = MatchCaseRgx.Match(DateTimeMatchCase.Value);
string TempMatchCase = DateTimeMatchCase.Value.Replace(RemoveMatch.Value, "");
commandString = commandString.Replace(DateTimeMatchCase.Value, TempMatchCase.Replace("(", "Convert(DateTime, ").Replace(")", ", 103)"));
}
return commandString;
}
#endregion
}
#endregion
#region ========== Class SqlConvert ==========
/// <summary>
/// Summary description for SqlConvert
/// </summary>
public sealed class SqlConvert
{
/// <summary>
/// Convert to byte[].
/// </summary>
/// <param name="BinaryStream">File upload binary stream.</param>
/// <param name="StreamLength">Lenght of File upload binary stream.</param>
/// <returns>Byte[] of binary stream.</returns>
public static byte[] ToVarBinary(Stream BinaryStream, int StreamLength)
{
BinaryReader BinaryRead = new BinaryReader(BinaryStream);
byte[] binaryData = BinaryRead.ReadBytes(StreamLength);
return binaryData;
}
/// <summary>
/// Convert to DataTime DataType with d/M/yyyy format.
/// </summary>
/// <param name="DateString">DateTime sring.</param>
/// <returns>Datetime Type.</returns>
public static DateTime ToDateTime(string DateString)
{
//ควรกำหนด culture ใน web.config เป็น th
//
//ตัวอย่างใน web.config
//<globalization requestEncoding="utf-8" responseEncoding="utf-8" culture="th-TH" uiCulture="th-TH"/>
return DateTime.ParseExact(DateString, "d/M/yyyy", CultureInfo.InvariantCulture);
}
/// <summary>
/// Convert to DataTime DataType with user define format.
/// </summary>
/// <param name="DateString">DateTime sring.</param>
/// <param name="DateFormat">DateTime Format</param>
/// <returns>Datetime Type.</returns>
public static DateTime ToDateTime(string DateString, string DateFormat)
{
//ควรกำหนด culture ใน web.config เป็น th
//
//ตัวอย่างใน web.config
//<globalization requestEncoding="utf-8" responseEncoding="utf-8" culture="th-TH" uiCulture="th-TH"/>
return DateTime.ParseExact(DateString, DateFormat, CultureInfo.InvariantCulture);
}
}
#endregion
|
 |
 |
 |
 |
Date :
2010-04-07 12:05:39 |
By :
tungman |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
|
|