 |
|
รบกวนช่วยดูโค้ด insert แบบ DropDownlist ให้ทีค่ะ เปลี่ยนมาหลายแบบแล้วก็ยังไม่ได้ |
|
 |
|
|
 |
 |
|
คือต้องการ insert แบบ DropDownlist จากหลาย table ค่ะ
แต่ขึ้น error Fill: SelectCommand.Connection property ค่ะ ที่ dtAdapter2.Fill(dt) ของ Page_Load ช่วง strSQL2 ค่ะ
ขอความกรุณา ช่วยแนะนำที่นะคะ ขอบคุณค่ะ
code.vb
Code (VB.NET)
Imports Microsoft.VisualBasic
Imports System
Imports System.Data
Imports System.Data.SqlClient
Imports System.Web.Configuration
Imports System.Web.UI.WebControls
Partial Class HRInsertEmployee
Inherits System.Web.UI.Page
Dim objConn As SqlConnection
Dim objCmd As SqlCommand
Dim dtAdapter As SqlDataAdapter
Dim query, query1, strSQL As String
Dim strSQL1, strSQL2, strSQL3, strSQL4, strSQL5 As String
Dim dtAdapter2, dtAdapter3, dtAdapter4, dtAdapter5 As SqlDataAdapter
Protected Sub Page_Load(ByVal sender As Object, ByVal e As System.EventArgs) Handles Me.Load
Dim dt As New DataTable
Dim strConnString As String
strConnString = ConfigurationManager.ConnectionStrings.Item("ConnectionString").ConnectionString
objConn = New SqlConnection(strConnString)
objConn.Open()
If Not Page.IsPostBack() Then
strSQL = "SELECT head_id, head_name FROM head"
dtAdapter = New SqlDataAdapter(strSQL, objConn)
dtAdapter.Fill(dt)
dtAdapter = Nothing
objConn.Close()
objConn = Nothing
With Me.ddlHead
.DataSource = dt
.DataTextField = "head_name"
.DataValueField = "head_id"
.DataBind()
End With
strSQL2 = "SELECT department_id, department_name FROM department"
dtAdapter2 = New SqlDataAdapter(strSQL2, objConn)
dtAdapter2.Fill(dt)
dtAdapter2 = Nothing
objConn.Close()
objConn = Nothing
With Me.ddldepartment
.DataSource = dt
.DataTextField = "department_name"
.DataValueField = "department_id"
.DataBind()
End With
strSQL3 = "SELECT authentication_id, authentication_name FROM authentication"
dtAdapter = New SqlDataAdapter(strSQL3, objConn)
dtAdapter.Fill(dt)
dtAdapter = Nothing
objConn.Close()
objConn = Nothing
With Me.ddlauthentication
.DataSource = dt
.DataTextField = "authentication_name"
.DataValueField = "authentication_id"
.DataBind()
End With
strSQL4 = "SELECT role_id, role_name FROM roces_role"
dtAdapter = New SqlDataAdapter(strSQL4, objConn)
dtAdapter.Fill(dt)
dtAdapter = Nothing
objConn.Close()
objConn = Nothing
With Me.ddlrole
.DataSource = dt
.DataTextField = "role_name"
.DataValueField = "role_id"
.DataBind()
End With
strSQL5 = "SELECT term_id, term_name FROM term"
dtAdapter = New SqlDataAdapter(strSQL5, objConn)
dtAdapter.Fill(dt)
dtAdapter = Nothing
objConn.Close()
objConn = Nothing
With Me.ddlterm
.DataSource = dt
.DataTextField = "term_name"
.DataValueField = "term_id"
.DataBind()
End With
ddlHead.SelectedIndex = ddlHead.Items.IndexOf(ddlHead.Items.FindByValue("head_id"))
ddldepartment.SelectedIndex = ddldepartment.Items.IndexOf(ddldepartment.Items.FindByValue("department_id"))
ddlauthentication.SelectedIndex = ddlauthentication.Items.IndexOf(ddlauthentication.Items.FindByValue("authentication_id"))
ddlrole.SelectedIndex = ddlrole.Items.IndexOf(ddlrole.Items.FindByValue("role_id"))
ddlterm.SelectedIndex = ddlterm.Items.IndexOf(ddlterm.Items.FindByValue("term_id"))
End If
End Sub
Protected Sub btnSave_Click(ByVal sender As Object, ByVal e As System.EventArgs) Handles btnSave.Click
Dim strConnString As String
strConnString = ConfigurationManager.ConnectionStrings.Item("ConnectionString").ConnectionString
strSQL = "INSERT INTO employee(employee_id, username, password, first_name, last_name, email)" & _
" VALUES " & _
" ('" & Me.txtemployee_id.Text & "','" & Me.txtusername.Text & "','" & Me.txtpassword.Text & "', " & _
" '" & Me.txtfirst_name.Text & "','" & Me.txtlast_name.Text & "','" & Me.txtemail.Text & "')"
objConn = New SqlConnection(strConnString)
objConn.Open()
objCmd = New SqlCommand
With objCmd
.Connection = objConn
.CommandText = strSQL
.CommandType = CommandType.Text
End With
strSQL1 = "INSERT INTO head(head_id)" & _
" VALUES " & _
" ('" & Me.ddlHead.SelectedItem.Value & "')"
objConn = New SqlConnection(strConnString)
objConn.Open()
objCmd = New SqlCommand
With objCmd
.Connection = objConn
.CommandText = strSQL
.CommandType = CommandType.Text
''*** DropDownlist ***'
'With Me.ddlHead
' .DataTextField = "head_name"
' .DataValueField = "head_id"
'End With
End With
strSQL2 = "INSERT INTO department(department_id, department_name)" & _
" VALUES " & _
" ('" & Me.ddldepartment.Text & "')"
objConn = New SqlConnection(strConnString)
objConn.Open()
objCmd = New SqlCommand
With objCmd
.Connection = objConn
.CommandText = strSQL2
.CommandType = CommandType.Text
''*** DropDownlist ***'
'With Me.ddldepartment
' .DataTextField = "department_name"
' .DataValueField = "department_id"
'End With
End With
strSQL3 = "INSERT INTO authentication(authentication_id, authentication_name)" & _
" VALUES " & _
" ('" & Me.ddlauthentication.Text & "')"
objConn = New SqlConnection(strConnString)
objConn.Open()
objCmd = New SqlCommand
With objCmd
.Connection = objConn
.CommandText = strSQL3
.CommandType = CommandType.Text
''*** DropDownlist ***'
'With Me.ddlauthentication
' .DataTextField = "authentication_name"
' .DataValueField = "authentication_id"
'End With
End With
strSQL4 = "INSERT INTO roces_role(role_id, role_name)" & _
" VALUES " & _
" ('" & Me.ddlrole.Text & "')"
objConn = New SqlConnection(strConnString)
objConn.Open()
objCmd = New SqlCommand
With objCmd
.Connection = objConn
.CommandText = strSQL4
.CommandType = CommandType.Text
''*** DropDownlist ***'
'With Me.ddlrole
' .DataTextField = "role_name"
' .DataValueField = "role_id"
'End With
End With
strSQL5 = "INSERT INTO term(term_id, term_name)" & _
" VALUES " & _
" ('" & Me.ddlterm.Text & "')"
objConn = New SqlConnection(strConnString)
objConn.Open()
objCmd = New SqlCommand
With objCmd
.Connection = objConn
.CommandText = strSQL5
.CommandType = CommandType.Text
''*** DropDownlist ***'
'With Me.ddlterm
' .DataTextField = "term_name"
' .DataValueField = "term_id"
' .DataBind()
'End With
End With
Me.pnlAdd.Visible = False
Try
objCmd.ExecuteNonQuery()
Me.lblStatus.Text = "Record Inserted"
Me.lblStatus.Visible = True
Catch ex As Exception
Me.lblStatus.Visible = True
Me.lblStatus.Text = "Record can not insert Error (" & ex.Message & ")"
End Try
objConn.Close()
objConn = Nothing
Response.Redirect("HRManagerEmployee.aspx")
End Sub
'Protected Sub ddlHead_SelectedIndexChanged(ByVal sender As Object, ByVal e As System.EventArgs) Handles ddlHead.SelectedIndexChanged
' Dim objConn As New SqlConnection
' Dim objCmd As New SqlCommand
' Dim strConnString, strSQL As String
' strConnString = ConfigurationManager.ConnectionStrings.Item("ConnectionString").ConnectionString
' strSQL = "SELECT head_id, head_name FROM head"
' objConn = New SqlConnection(strConnString)
' objConn.Open()
' '*** DropDownlist ***'
' With Me.ddlHead
' .DataTextField = "head_name"
' .DataValueField = "head_id"
' End With
'End Sub
End Class
Tag : .NET, Ms SQL Server 2005, VS 2008 (.NET 3.x)
|
|
 |
 |
 |
 |
Date :
2011-03-31 10:32:03 |
By :
myASP |
View :
1133 |
Reply :
4 |
|
 |
 |
 |
 |
|
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
รบกวนกรุณาผู้ใจบุญ ชี้ทางสว่างให้ทีค่ะ
|
 |
 |
 |
 |
Date :
2011-03-31 21:03:50 |
By :
myASP |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
Code (VB.NET)
Imports Microsoft.VisualBasic
Imports System
Imports System.Data
Imports System.Data.SqlClient
Imports System.Web.Configuration
Imports System.Web.UI.WebControls
Partial Class HRInsertEmployee
Inherits System.Web.UI.Page
Dim objConn As SqlConnection
Dim objCmd As SqlCommand
Dim dtAdapter As SqlDataAdapter
Dim query, query1, strSQL As String
Dim strSQL1, strSQL2, strSQL3, strSQL4, strSQL5 As String
Dim dtAdapter2, dtAdapter3, dtAdapter4, dtAdapter5 As SqlDataAdapter
Protected Sub Page_Load(ByVal sender As Object, ByVal e As System.EventArgs) Handles Me.Load
If Not Page.IsPostBack() Then
Dim dt As New DataTable
Dim strConnString As String
strConnString = ConfigurationManager.ConnectionStrings.Item("ConnectionString").ConnectionString
objConn = New SqlConnection(strConnString)
objConn.Open()
strSQL1 = "SELECT head_id, head_name FROM head"
dtAdapter = New SqlDataAdapter(strSQL1, objConn)
dtAdapter.Fill(dt)
dtAdapter = Nothing
objConn.Close()
objConn = Nothing
With Me.ddlHead
.DataSource = dt
.DataTextField = "head_name"
.DataValueField = "head_id"
.DataBind()
End With
ddlHead.SelectedIndex = ddlHead.Items.IndexOf(ddlHead.Items.FindByValue("head_id"))
End If
If Not Page.IsPostBack() Then
Dim dt As New DataTable
Dim strConnString As String
strConnString = ConfigurationManager.ConnectionStrings.Item("ConnectionString").ConnectionString
objConn = New SqlConnection(strConnString)
objConn.Open()
strSQL = "SELECT department_id, department_name FROM department"
dtAdapter = New SqlDataAdapter(strSQL, objConn)
dtAdapter.Fill(dt)
dtAdapter = Nothing
objConn.Close()
objConn = Nothing
With Me.ddldepartment
.DataSource = dt
.DataTextField = "department_name"
.DataValueField = "department_id"
.DataBind()
End With
ddldepartment.SelectedIndex = ddldepartment.Items.IndexOf(ddldepartment.Items.FindByValue("department_id"))
End If
If Not Page.IsPostBack() Then
Dim dt As New DataTable
Dim strConnString As String
strConnString = ConfigurationManager.ConnectionStrings.Item("ConnectionString").ConnectionString
objConn = New SqlConnection(strConnString)
objConn.Open()
strSQL = "SELECT authentication_id, authentication_name FROM authentication"
dtAdapter = New SqlDataAdapter(strSQL, objConn)
dtAdapter.Fill(dt)
dtAdapter = Nothing
objConn.Close()
objConn = Nothing
With Me.ddlauthentication
.DataSource = dt
.DataTextField = "authentication_name"
.DataValueField = "authentication_id"
.DataBind()
End With
ddlauthentication.SelectedIndex = ddlauthentication.Items.IndexOf(ddlauthentication.Items.FindByValue("authentication_id"))
End If
If Not Page.IsPostBack() Then
Dim dt As New DataTable
Dim strConnString As String
strConnString = ConfigurationManager.ConnectionStrings.Item("ConnectionString").ConnectionString
objConn = New SqlConnection(strConnString)
objConn.Open()
strSQL = "SELECT role_id, role_name FROM roces_role"
dtAdapter = New SqlDataAdapter(strSQL, objConn)
dtAdapter.Fill(dt)
dtAdapter = Nothing
objConn.Close()
objConn = Nothing
With Me.ddlrole
.DataSource = dt
.DataTextField = "role_name"
.DataValueField = "role_id"
.DataBind()
End With
ddlrole.SelectedIndex = ddlrole.Items.IndexOf(ddlrole.Items.FindByValue("role_id"))
End If
If Not Page.IsPostBack() Then
Dim dt As New DataTable
Dim strConnString As String
strConnString = ConfigurationManager.ConnectionStrings.Item("ConnectionString").ConnectionString
objConn = New SqlConnection(strConnString)
objConn.Open()
strSQL = "SELECT term_id, term_name FROM term"
dtAdapter = New SqlDataAdapter(strSQL, objConn)
dtAdapter.Fill(dt)
dtAdapter = Nothing
objConn.Close()
objConn = Nothing
With Me.ddlterm
.DataSource = dt
.DataTextField = "term_name"
.DataValueField = "term_id"
.DataBind()
End With
ddlterm.SelectedIndex = ddlterm.Items.IndexOf(ddlterm.Items.FindByValue("term_id"))
End If
End Sub
Protected Sub btnSave_Click(ByVal sender As Object, ByVal e As System.EventArgs) Handles btnSave.Click
Dim strConnString As String
strConnString = ConfigurationManager.ConnectionStrings.Item("ConnectionString").ConnectionString
strSQL = "INSERT INTO employee(employee_id, username, password, first_name, last_name, email)" & _
" VALUES " & _
" ('" & Me.txtemployee_id.Text & "','" & Me.txtusername.Text & "','" & Me.txtpassword.Text & "', " & _
" '" & Me.txtfirst_name.Text & "','" & Me.txtlast_name.Text & "','" & Me.txtemail.Text & "')"
objConn = New SqlConnection(strConnString)
objConn.Open()
objCmd = New SqlCommand
With objCmd
.Connection = objConn
.CommandText = strSQL
.CommandType = CommandType.Text
End With
strSQL1 = "INSERT INTO head(head_id)" & _
" VALUES " & _
" ('" & Me.ddlHead.SelectedItem.Value & "')"
objConn = New SqlConnection(strConnString)
objConn.Open()
objCmd = New SqlCommand
With objCmd
.Connection = objConn
.CommandText = strSQL
.CommandType = CommandType.Text
''*** DropDownlist ***'
'With Me.ddlHead
' .DataTextField = "head_name"
' .DataValueField = "head_id"
'End With
End With
strSQL2 = "INSERT INTO department(department_id, department_name)" & _
" VALUES " & _
" ('" & Me.ddldepartment.Text & "')"
objConn = New SqlConnection(strConnString)
objConn.Open()
objCmd = New SqlCommand
With objCmd
.Connection = objConn
.CommandText = strSQL2
.CommandType = CommandType.Text
''*** DropDownlist ***'
'With Me.ddldepartment
' .DataTextField = "department_name"
' .DataValueField = "department_id"
'End With
End With
strSQL3 = "INSERT INTO authentication(authentication_id, authentication_name)" & _
" VALUES " & _
" ('" & Me.ddlauthentication.Text & "')"
objConn = New SqlConnection(strConnString)
objConn.Open()
objCmd = New SqlCommand
With objCmd
.Connection = objConn
.CommandText = strSQL3
.CommandType = CommandType.Text
''*** DropDownlist ***'
'With Me.ddlauthentication
' .DataTextField = "authentication_name"
' .DataValueField = "authentication_id"
'End With
End With
strSQL4 = "INSERT INTO roces_role(role_id, role_name)" & _
" VALUES " & _
" ('" & Me.ddlrole.Text & "')"
objConn = New SqlConnection(strConnString)
objConn.Open()
objCmd = New SqlCommand
With objCmd
.Connection = objConn
.CommandText = strSQL4
.CommandType = CommandType.Text
''*** DropDownlist ***'
'With Me.ddlrole
' .DataTextField = "role_name"
' .DataValueField = "role_id"
'End With
End With
strSQL5 = "INSERT INTO term(term_id, term_name)" & _
" VALUES " & _
" ('" & Me.ddlterm.Text & "')"
objConn = New SqlConnection(strConnString)
objConn.Open()
objCmd = New SqlCommand
With objCmd
.Connection = objConn
.CommandText = strSQL5
.CommandType = CommandType.Text
''*** DropDownlist ***'
'With Me.ddlterm
' .DataTextField = "term_name"
' .DataValueField = "term_id"
' .DataBind()
'End With
End With
Me.pnlAdd.Visible = False
Try
objCmd.ExecuteNonQuery()
Me.lblStatus.Text = "Record Inserted"
Me.lblStatus.Visible = True
Catch ex As Exception
Me.lblStatus.Visible = True
Me.lblStatus.Text = "Record can not insert Error (" & ex.Message & ")"
End Try
objConn.Close()
objConn = Nothing
Response.Redirect("HRManagerEmployee.aspx")
End Sub
'Protected Sub ddlHead_SelectedIndexChanged(ByVal sender As Object, ByVal e As System.EventArgs) Handles ddlHead.SelectedIndexChanged
' Dim objConn As New SqlConnection
' Dim objCmd As New SqlCommand
' Dim strConnString, strSQL As String
' strConnString = ConfigurationManager.ConnectionStrings.Item("ConnectionString").ConnectionString
' strSQL = "SELECT head_id, head_name FROM head"
' objConn = New SqlConnection(strConnString)
' objConn.Open()
' '*** DropDownlist ***'
' With Me.ddlHead
' .DataTextField = "head_name"
' .DataValueField = "head_id"
' End With
'End Sub
End Class
แล้วมันขึ้น
Server Error in '/Core' Application.
--------------------------------------------------------------------------------
The resource cannot be found.
Description: HTTP 404. The resource you are looking for (or one of its dependencies) could have been removed, had its name changed, or is temporarily unavailable. Please review the following URL and make sure that it is spelled correctly.
Requested URL: /Core/HRManagerEmployee.aspx
--------------------------------------------------------------------------------
Version Information: Microsoft .NET Framework Version:2.0.50727.4952; ASP.NET Version:2.0.50727.4955
ค่ะ
ปัญหาขึ้น หนูใช้ SqlDataSource เป็นตัวขึ้นมาจาก DropDownList ค่ะ
|
 |
 |
 |
 |
Date :
2011-04-01 13:53:52 |
By :
myASP |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
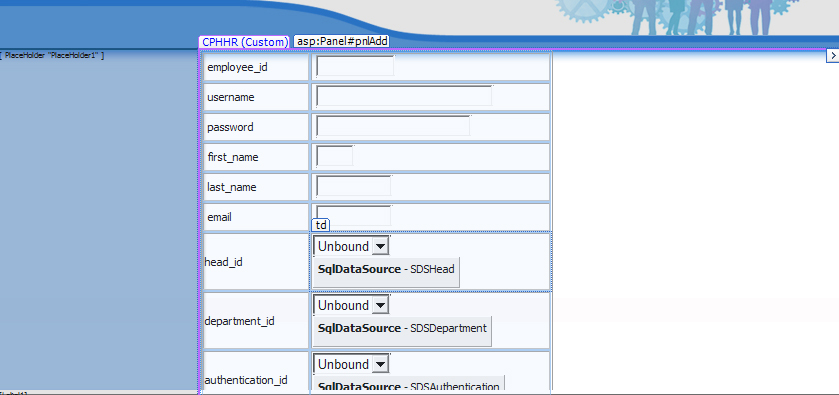
|
 |
 |
 |
 |
Date :
2011-04-01 14:00:29 |
By :
myASP |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
|
|