 |
|
[vb.net] Error ขึ้น the sqldbtype enumeration value 128 is invalid อะครับ |
|
 |
|
|
 |
 |
|
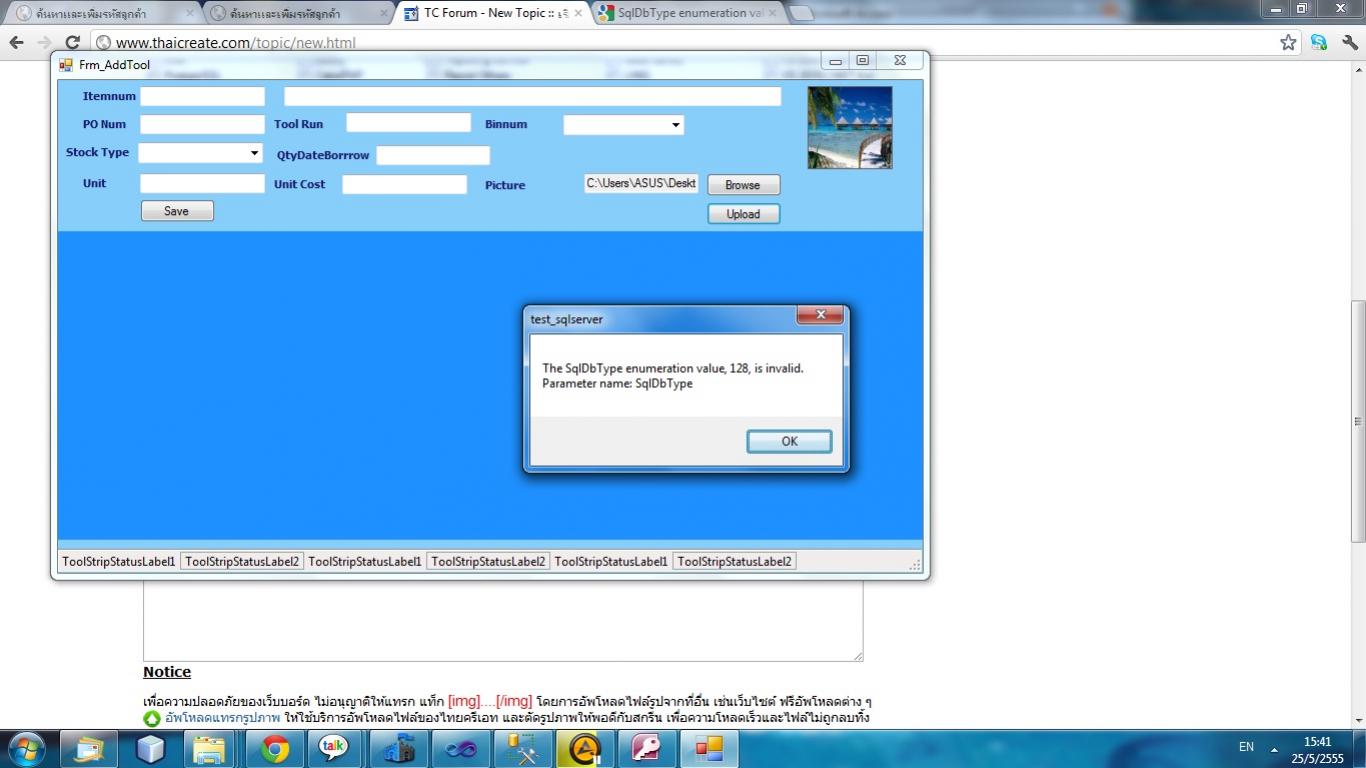
Erroe ดังภาพอะครับ คือผมจะเขียนไฟล์โดยเก็บไฟล์ภาพไว้อะครับ โดยฐานข้อมูล data type เป็น Binary(MAX)
คือตอนแรกใช้ฐานข้อมูล Access ใช้ Dataype เป็น OLE Object สามารถบันทึกได้นะครับ แต่พอเปลี่ยนฐานข้อมูลเป็น SQl SERVER มันขึ้น Error pังงี้อะครับ
ใน Class DBFile
Code (VB.NET)
Public Function SaveFile(ByVal clientFilePath As String, ByVal clientFileExt As String) As Integer
Dim targetFileName As String = "f_" & DateTime.Now.ToString("yyyyhMMdd_hmmss") & clientFileExt
Dim objReader As SqlDataReader
Dim objCmd As New SqlCommand
Dim resultDt As DataTable = New DataTable()
Dim new_id As Integer = 0
'Declare a file stream object
Dim o As FileStream
'Declare a stream reader object
Dim r As StreamReader
'--- เปิดไฟล์เพื่ออ่านข้อมูล ตาม path ที่เก็บไฟล์จากตัวแปร clientFilePath
o = New FileStream(clientFilePath, FileMode.Open, FileAccess.Read, FileShare.Read)
'--- อ่านข้อมูลไฟล์ลง stream
r = New StreamReader(o)
Try
'--- สร้างตัวแปรขึ้นมาตัวนึง เป็นประเภท Byte Array เพื่อเอาไว้เก็บข้อมูลรูปภาพ
Dim FileByteArray(o.Length - 1) As Byte
'--- อ่านข้อมูลจาก stream ลงใน byte array
o.Read(FileByteArray, 0, o.Length)
'--- insert ลงฐานข้อมูล
Dim strSQL As String = "INSERT INTO tbl_file ([file_name], [file_byte], [file_ext], [uploader], [upload_date]) VALUES ('" & targetFileName & "', @PictureL, '" & clientFileExt & "', '', '" & DateTime.Now.ToString("dd/MM/yyyy") & "')"
objCmd = New SqlCommand(strSQL, objConn)
'Add parameters to the OleDbCommand object
objCmd.Parameters.Add("@PictureL", System.Data.OleDb.OleDbType.Binary, o.Length).Value = FileByteArray
'Save the image into the database
Dim rowEffect As Integer = objCmd.ExecuteNonQuery()
objCmd.Dispose()
'--- เมื่อ insert แล้ว เราจะดึง fileID ล่าสุดที่เพิ่ง insert ไปขึ้นมา
If (rowEffect > 0) Then
strSQL = "SELECT @@identity AS NewID FROM tbl_file"
objCmd = New SqlCommand(strSQL, objConn)
objReader = objCmd.ExecuteReader()
resultDt.Load(objReader)
new_id = CInt(resultDt.Rows(0)("NewID"))
objCmd.Dispose()
End If
'Close the connection
objConn.Close()
Catch ex As Exception
ErrorMessage = ex.Message
End Try
'--- แล้วรีเทิร์นออกไป ถ้า fileID > 0 แสดงว่า insert สำเร็จ
Return new_id
End Function
อันนี้หน้าฟอร์มนะครับ
Code (VB.NET)
Private Sub btnUploadClick(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnUpload.Click
Dim filePath As String = ""
Dim fileExt As String = ""
filePath = oFileDialog.FileName
fileExt = System.IO.Path.GetExtension(filePath)
Dim objFile As DBFile = New DBFile()
Dim new_id = objFile.SaveFile(filePath, fileExt)
If (new_id > 0) Then
MsgBox("อัพโหลดเรียบร้อยแล้ว")
lbReturnFileID.Text = new_id.ToString()
'--- เมื่ออัพโหลดสำเร็จ จะ return ค่า new_id ซึ่งตรงกับ file_id ในฐานข้อมูล เมื่อ new_id > 0 แสดงว่าอัพโหลดสำเร็จ
'--- ถ้าเป็น 0 แสดงว่า error เราสามารถเอา new_id ตัวนี้ไปใช้ insert ลง table tool ได้
Else
MsgBox(objFile.ErrorMessage)
End If
End Sub
Tag : .NET, Ms SQL Server 2008
|
|
 |
 |
 |
 |
Date :
2012-05-25 15:48:23 |
By :
tavada_b |
View :
2031 |
Reply :
1 |
|
 |
 |
 |
 |
|
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ลองดูนี่ครับการใช้ .NET กับ SQL Server BLOB
Code (VB.NET)
Dim imbByte(fUpload.PostedFile.InputStream.Length) As Byte
fUpload.PostedFile.InputStream.Read(imbByte, 0, imbByte.Length)
'*** MimeType ***'
Dim ExtType As String = System.IO.Path.GetExtension(fUpload.PostedFile.FileName).ToLower()
Dim strMIME As String = Nothing
Select Case ExtType
Case ".gif"
strMIME = "image/gif"
Case ".jpg", ".jpeg", ".jpe"
strMIME = "image/jpeg"
Case ".png"
strMIME = "image/png"
Case Else
Me.lblStatus.Text = "Invalid file type."
Exit Sub
End Select
'*** Insert to Database ***'
Dim objConn As New SqlConnection
Dim strConnString, strSQL As String
strConnString = "Server=localhost;UID=sa;PASSWORD=;database=mydatabase;Max Pool Size=400;Connect Timeout=600;"
strSQL = "INSERT INTO files (Name,FilesName,FilesType) " & _
" VALUES " & _
" (@sName,@sFilesName,@sFilesType)"
objConn.ConnectionString = strConnString
objConn.Open()
Dim objCmd As New SqlCommand(strSQL, objConn)
objCmd.Parameters.Add("@sName", SqlDbType.VarChar).Value = Me.txtName.Text
objCmd.Parameters.Add("@sFilesName", SqlDbType.Binary).Value = imbByte
objCmd.Parameters.Add("@sFilesType", SqlDbType.VarChar).Value = strMIME
objCmd.ExecuteNonQuery()
objConn.Close()
objConn = Nothing
Go to : ASP.NET SQL Server BLOB Binary Data and Parameterized Query
|
 |
 |
 |
 |
Date :
2012-05-25 17:52:39 |
By :
mr.win |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
|
|