 |
|

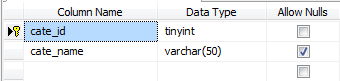
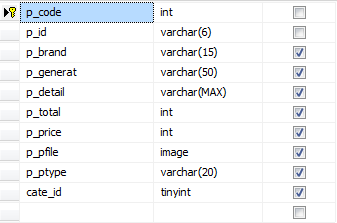
ต้องการแสดงชื่อประเภทใน RowDataBound ครับ
Code (ASP)
Imports System.Data
Imports System.Data.SqlClient
Partial Class Admin_Productlist
Inherits System.Web.UI.Page
Dim objConn As SqlConnection
Dim objCmd As SqlCommand
Protected Sub Page_Load(ByVal sender As Object, ByVal e As System.EventArgs) Handles Me.Load
Dim strConnString As String
strConnString = "Server=localhost;UID=sa;PASSWORD=30011991;database=db_JSPJ;Max Pool Size=400;Connect Timeout=600;"
objConn = New SqlConnection(strConnString)
objConn.Open()
BindData()
End Sub
Protected Sub BindData()
Dim strSQL As String
strSQL = "SELECT * FROM Product ORDER BY p_code "
Dim dtReader As SqlDataReader
objCmd = New SqlCommand(strSQL, objConn)
dtReader = objCmd.ExecuteReader()
'*** BindData to GridView ***'
dgvPro.DataSource = dtReader
dgvPro.DataBind()
dtReader.Close()
dtReader = Nothing
End Sub
Protected Sub Page_Unload(ByVal sender As Object, ByVal e As System.EventArgs) Handles Me.Unload
objConn.Close()
objConn = Nothing
End Sub
Protected Sub dgvPro_RowDataBound(ByVal sender As Object, ByVal e As System.Web.UI.WebControls.GridViewRowEventArgs) Handles dgvPro.RowDataBound
Dim lblid As Label = DirectCast(e.Row.FindControl("lblid"), Label)
If Not IsNothing(lblid) Then
lblid.Text = e.Row.DataItem("p_id")
End If
Dim lblbrand As Label = DirectCast(e.Row.FindControl("lblbrand"), Label)
If Not IsNothing(lblbrand) Then
lblbrand.Text = e.Row.DataItem("p_brand")
End If
Dim lblgen As Label = DirectCast(e.Row.FindControl("lblgen"), Label)
If Not IsNothing(lblgen) Then
lblgen.Text = e.Row.DataItem("p_generat")
End If
Dim lbldetail As Label = DirectCast(e.Row.FindControl("lbldetail"), Label)
If Not IsNothing(lbldetail) Then
lbldetail.Text = e.Row.DataItem("p_detail")
End If
Dim lblcate As Label = DirectCast(e.Row.FindControl("lblcate"), Label)
If Not IsNothing(lblcate) Then
lblcate.Text = e.Row.DataItem("cate_id")
End If
Dim lbltotal As Label = DirectCast(e.Row.FindControl("lbltotal"), Label)
If Not IsNothing(lbltotal) Then
lbltotal.Text = e.Row.DataItem("p_total")
End If
Dim lblprice As Label = DirectCast(e.Row.FindControl("lblprice"), Label)
If Not IsNothing(lblprice) Then
lblprice.Text = e.Row.DataItem("p_price")
End If
'*** Picture ***'
Dim ImgPic As Image = DirectCast(e.Row.FindControl("ImgPic"), Image)
If Not IsNothing(ImgPic) Then
ImgPic.ImageUrl = "ViewImg.aspx?p_code= " & e.Row.DataItem("p_code")
End If
'*** Hyperlink ***'
Dim hplEdit As HyperLink = DirectCast(e.Row.FindControl("hplEdit"), HyperLink)
If Not IsNothing(hplEdit) Then
hplEdit.Text = "Edit"
hplEdit.NavigateUrl = "EditPro.aspx?p_code=" & e.Row.DataItem("p_code")
End If
End Sub
End Class
Tag : ASP
|
|
 |
 |
 |
 |
Date :
2014-03-16 12:42:07 |
By :
Realiku |
View :
1254 |
Reply :
2 |
|
 |
 |
 |
 |
|
|
|
 |