 |
|
ผมมีปัญหาเรื่องการปิดฟอร์มครับ พอดี ผมทำ datagrid เชื่อม database กับ sql
มีการ เพิ่มข้อมูล แก้ไขข้อมูล ลบข้อมูล ซึ่งผ่านไปได้ด้วยดี ตรงนี้ไม่ใช่ปญหาครับ
ปัญหาที่ผมเจอคือตามนี้ครับ ผมขออธิบายตามรูปนะ
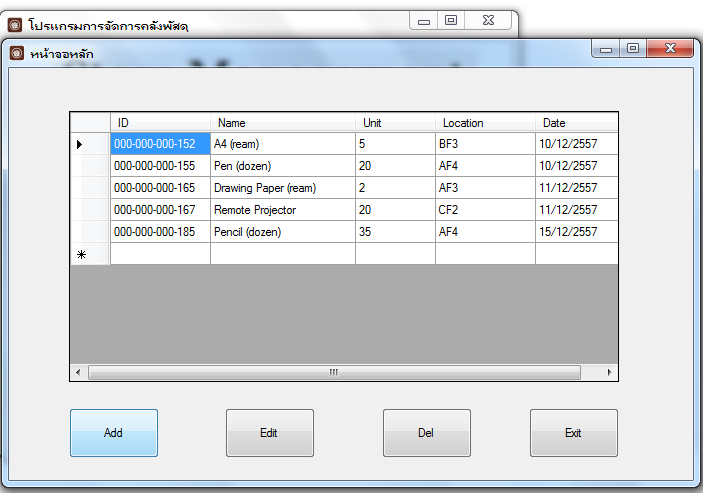
1. รูปนี้เป็นหน้าหลักโปรแกรมครับผม (ผมกำลังจะเข้าไปที่ปุ่มฟังก์ชั่น ADD)
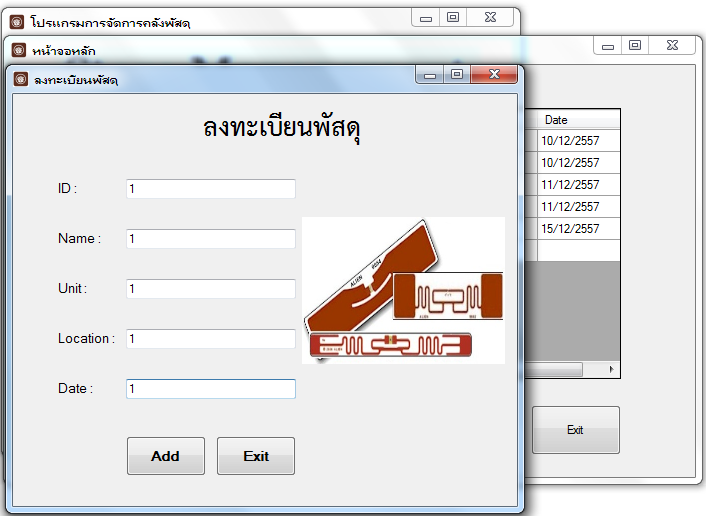
2. รูปนี้เป็นรูปในฟังก์ชั่น ADD มีหน้าตา form ดังนี้
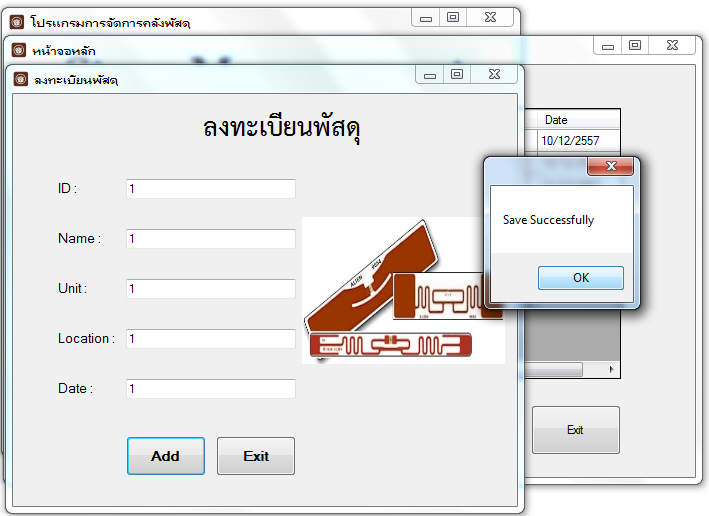
3. รูปนี้เป็นรูปตอนกรอกข้อมูลทั้งหมดเรียบร้อยแล้วกด ADD แล้วครับ (Success เรียบร้อย)
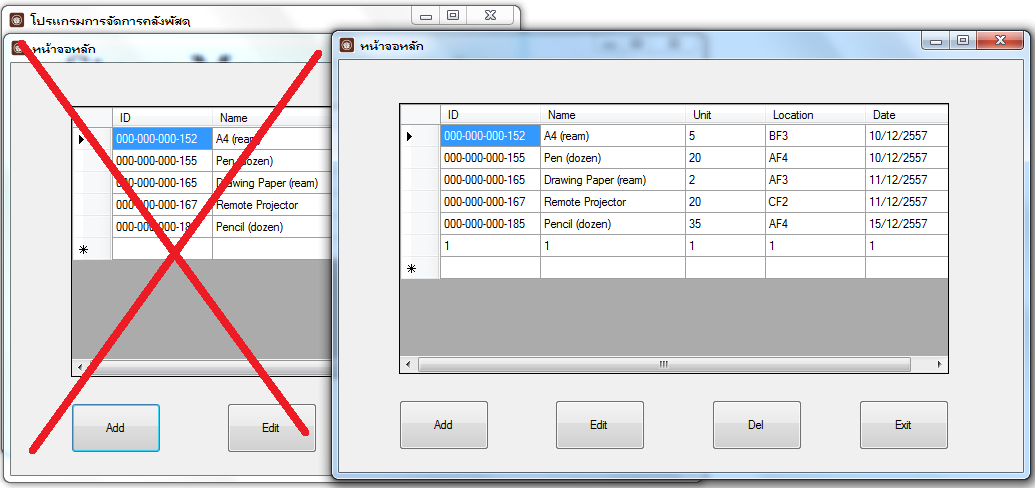
4. รูปนี้เป็นจุดที่ผมปวดใจมากที่สุดเลยครับ พอกรอกเสร็จมันขึ้น Form ใหม่ที่อัพเดทล่าสุด โดยที่คาฟอร์มเก่าที่ไม่อัพเดทไว้ข้างหลัง
ผมลองแก้หลายวิธีแล้วคับ แต่ยังไม่ถูกจุดซักที ผมรบกวนพี่ๆเพื่อนๆน้องๆทุกคนหน่อยนะครับ
ผมอยากได้ประมาณ่า ให้ form เก่ามันหายไปโดยที่โชว์ฟอร์มใหม่แทน หรือ ให้ฟอร์มเก่ามันอัพเดทโดยที่ไม่ต้องโชว์ฟอร์มใหม่อะครับ
ส่วนนี้เป็นโค้ด form ADD นะครับ ช่วยทีนะครับ
Code (C#)
using System;
using System.Linq;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Text;
using System.Windows.Forms;
using System.Data.SqlServerCe;
using System.Data.SqlTypes;
namespace WindowsFormsApplication
{
public partial class frmAdd : Form
{
public frmAdd()
{
InitializeComponent();
}
// อินพุทค่าลงใน textbox แต่ละช่องก่อนทำการส่งไปยัง database
private void btnAdd_Click(object sender, EventArgs e)
{
if (string.IsNullOrEmpty(this.txtID.Text))
{
MessageBox.Show("Please input (ID)");
this.txtID.Focus();
return;
}
if (string.IsNullOrEmpty(this.txtName.Text))
{
MessageBox.Show("Please input (Name)");
this.txtName.Focus();
return;
}
if (string.IsNullOrEmpty(this.txtUnit.Text))
{
MessageBox.Show("Please input (Unit)");
this.txtUnit.Focus();
return;
}
if (string.IsNullOrEmpty(this.txtLocation.Text))
{
MessageBox.Show("Please input (Location)");
this.txtLocation.Focus();
return;
}
if (string.IsNullOrEmpty(this.txtDate.Text))
{
MessageBox.Show("Please input (Date)");
this.txtDate.Focus();
return;
}
SqlCeConnection myConnection = default(SqlCeConnection);
//myConnection = new SqlCeConnection("Data Source =" + (System.IO.Path.GetDirectoryName(System.Reflection.Assembly.GetExecutingAssembly().GetName().CodeBase) + "\\AppDatabase1.sdf;"));
myConnection = new SqlCeConnection("Data Source =C:\\Users\\Nick\\Documents\\Visual Studio 2010\\Projects\\Present\\KIKI\\WindowsFormsApplication\\Database1.sdf;");
myConnection.Open();
SqlCeCommand myCommand = myConnection.CreateCommand();
myCommand.CommandText = "INSERT INTO [mytable] ([ID], [Name], [Unit], [Location], [Date]) VALUES " + " ('" + this.txtID.Text + "','" + this.txtName.Text + "','" + this.txtUnit.Text + "','" + this.txtLocation.Text + "','" + this.txtDate.Text + "') ";
myCommand.CommandType = CommandType.Text;
myCommand.ExecuteNonQuery();
myConnection.Close();
MessageBox.Show("Save Successfully");
this.Hide();
frmHome f = new frmHome();
f.Show();
}
private void btnExit_Click(object sender, EventArgs e)
{
this.Close();
}
private void frmAdd_Load(object sender, EventArgs e)
{
}
}
}
รบกวนทีนะครับ พอดีมือใหม่มากๆ ขอบคุณล่วงหน้าทุกๆคนนะครับ ที่ช่วยเสียเวลา
Tag : .NET, MySQL, C#
|
|
 |
 |
 |
 |
Date :
2014-12-23 17:00:12 |
By :
oakioaki |
View :
1634 |
Reply :
2 |
|
 |
 |
 |
 |
|
|
|
 |