 |
|
[Web,C#] ต้องการ Pass Value ไปหน้าที่ 1 แล้วกดปุ่มในหน้าที่ 1 ส่งต่อไปหน้าที่ 2 ค่ะ |
|
 |
|
|
 |
 |
|
ต้องการ Pass Value ไปหน้าที่ 1 แล้วกดปุ่มในหน้าที่ 1 ส่งต่อไปหน้าที่ 2 ค่ะ
สามารถ Pass Value ไปหน้าที่ 1 ได้แล้วดังนี้ค่ะ
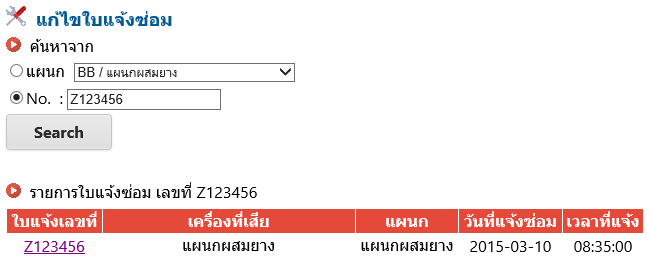
กดลิ้งเพื่อส่งไปหน้า 1 ได้แล้ว
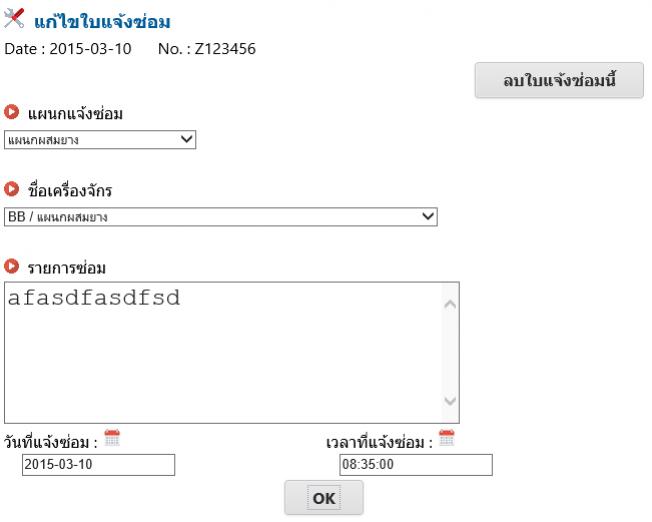
แต่ถ้ากดปุ่ม OK ในหน้า 1 อยากให้ Pass Value ไปหน้า 2 แต่ใน Textbox และ Dropdownlist ไม่มีข้อมูลขึ้นเลยค่ะ
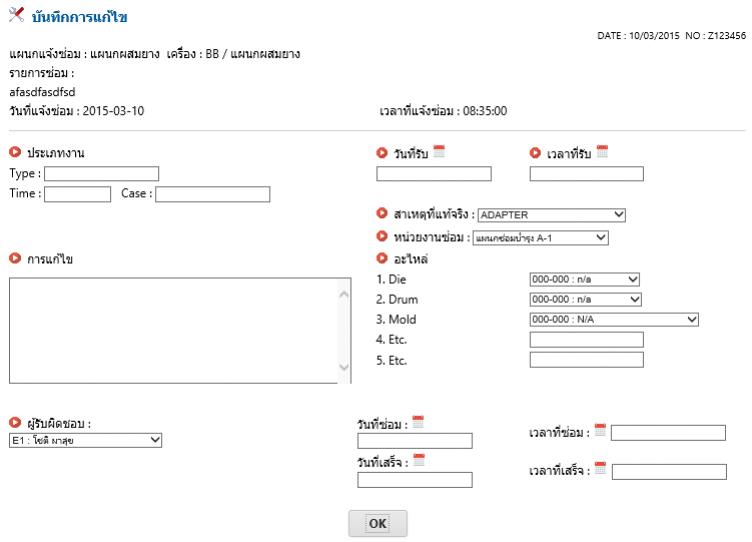
โค้ดในหน้า 1 คืออันนี้ค่ะ
Code (C#)
<script runat="server">
String strConnString = WebConfigurationManager.ConnectionStrings["MDSConnectionString"].ConnectionString;
protected void Page_Load(object sender, EventArgs e)
{
if (Session["strUser"] == null)
{
Response.Redirect("~/index.aspx");
Response.End();
}
else if (!IsPostBack)
{
lblIDser.Text = Request.QueryString["id_nob"];
BindContrydropdown1();
BindContrydropdown2();
BindData();
this.btnOK = btnOK;
}
}
protected void BindContrydropdown1()
{
using (SqlConnection con = new SqlConnection(strConnString))
{
con.Open();
SqlCommand cmd = new SqlCommand("Select id_itemtype,itemtype FROM dbo.tbl_itemtype WHERE archive_date IS NULL", con);
SqlDataAdapter da = new SqlDataAdapter(cmd);
DataSet ds = new DataSet();
da.Fill(ds);
ddlDep.DataSource = ds;
ddlDep.DataTextField = "itemtype";
ddlDep.DataValueField = "id_itemtype";
ddlDep.DataBind();
con.Close();
}
}
protected void BindContrydropdown2()
{
using (SqlConnection con = new SqlConnection(strConnString))
{
con.Open();
string sqlStatement = "SELECT id_item + ' / ' + item AS item, id_item FROM dbo.tbl_item WHERE archive_date IS NULL";
SqlCommand cmd = new SqlCommand(sqlStatement, con);
SqlDataAdapter da = new SqlDataAdapter(cmd);
DataSet ds = new DataSet();
da.Fill(ds);
ddlMachine.DataSource = ds;
ddlMachine.DataTextField = "item";
ddlMachine.DataValueField = "id_item";
ddlMachine.DataBind();
con.Close();
}
}
protected void BindData()
{
DataTable dt = new DataTable();
SqlConnection con = new SqlConnection(strConnString);
con.Open();
SqlCommand cmd = new SqlCommand("SELECT dt_to,id_cu,id_item,txt,dt_sa,ti_sa from dbo.tbl_blue WHERE id_nob = '" + lblIDser.Text + "'", con);
SqlDataAdapter da = new SqlDataAdapter(cmd);
da.Fill(dt);
if (dt.Rows.Count > 0)
{
lblDate.Text = dt.Rows[0]["dt_to"].ToString();
lblDate.Text = dt.Rows[0].Field<DateTime>(0).ToString("yyyy-MM-dd");
txtSerList.Text = dt.Rows[0]["txt"].ToString();
ddlDep.SelectedValue = dt.Rows[0]["id_cu"].ToString();
ddlMachine.SelectedValue = dt.Rows[0]["id_item"].ToString();
txtDtsa.Text = dt.Rows[0]["dt_sa"].ToString();
txtDtsa.Text = dt.Rows[0].Field<DateTime>(4).ToString("yyyy-MM-dd");
txtTisa.Text = dt.Rows[0]["ti_sa"].ToString();
}
con.Close();
}
void btnOK_Click(Object sender, EventArgs e)
{
SqlConnection con = new SqlConnection(strConnString);
SqlCommand cmd = new SqlCommand();
String strSQL;
strSQL = "UPDATE dbo.tbl_blue SET " +
" id_cu = '" + ddlDep.SelectedItem.Value + "'" +
" ,id_item = '" + ddlMachine.SelectedItem.Value + "'" +
" ,txt = '" + txtSerList.Text + "'" +
" ,dt_sa = '" + txtDtsa.Text + "'" +
" ,ti_sa = '" + txtTisa.Text + "'" +
" WHERE id_nob = '" + lblIDser.Text + "'";
Session["strIDser"] = lblIDser.Text;
Session["strDep"] = ddlDep.SelectedItem.Text;
Session["strMachine"] = ddlMachine.SelectedItem.Text;
Session["strSerlist"] = txtSerList.Text;
Session["strDtsa"] = txtDtsa.Text;
Session["strTisa"] = txtTisa.Text;
con.ConnectionString = strConnString;
con.Open();
cmd.Connection = con;
cmd.CommandText = strSQL;
cmd.CommandType = CommandType.Text;
try
{
cmd.ExecuteNonQuery();
Response.Redirect("~/service/fr_eservice2.aspx");
//this.lblStatus.Visible = true;
//this.lblStatus.Text = "บันทึกการแก้ไขใบแจ้งซ่อมสำเร็จ";
}
catch (Exception ex)
{
this.lblStatus.Visible = true;
this.lblStatus.Text = "Record can not insert Error (" + ex.Message + ")";
}
con.Close();
con = null;
}
protected void btnDel_Click(object sender, EventArgs e)
{
String strSQL;
strSQL = "SELECT b.id_nob INTO #RecordsToDelete FROM dbo.tbl_blue b JOIN dbo.tbl_yellow y ON b.id_nob = y.id_noy WHERE b.id_nob = '" + lblIDser.Text + "' DELETE FROM dbo.tbl_blue WHERE id_nob IN (SELECT id_nob FROM #RecordsToDelete) DELETE FROM dbo.tbl_yellow WHERE id_noy IN (SELECT id_nob FROM #RecordsToDelete)";
SqlConnection con = new SqlConnection(strConnString);
SqlCommand cmd = new SqlCommand(strSQL, con);
try
{
con.Open();
cmd.ExecuteNonQuery();
this.lblStatus.Visible = true;
this.lblStatus.Text = "Record deleted.";
}
catch (Exception ex)
{
this.lblStatus.Visible = true;
this.lblStatus.Text = "Record can not delete Error (" + ex.Message + ")";
}
con.Close();
con = null;
}
</script>
Tag : .NET, Ms SQL Server 2008, Web (ASP.NET), VS 2012 (.NET 4.x)
|
|
 |
 |
 |
 |
Date :
2015-03-10 21:55:15 |
By :
MercBenz |
View :
1037 |
Reply :
2 |
|
 |
 |
 |
 |
|
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
พี่คับผมเคยใช้วิธีเนี้ยได้อ่ะ ส่งข้อมูล ตัวอย่างแรก อ่าคับ
http://www.nfex.ru/index.php/ASP.Net/Page/Cross_page_posting
|
 |
 |
 |
 |
Date :
2015-03-11 10:54:02 |
By :
tongiggabite |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ปกติใน ASP.Net จะนิยมทำทุกอย่างให้อยู่ในหน้าเดียวกันครับ เพราะจะสามารถจัดการกับคาตัวแปรต่าง ๆ ผ่านการ PostBack ได้ครับ ลองใช้พวก Tab หรือ Panel ก็ได้ครับ 
|
 |
 |
 |
 |
Date :
2015-03-11 20:43:49 |
By :
mr.win |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
|
|