 |
|
พอดีเพลงผมเยอะเลยเขียนโค๊ดสำหรับจัดการชื่อเพลงไว้
โดย ลบ/เพิ่ม ชื่อนักร้อง หรือชื่อโฟลเดอร์ได้
ลบเจ้าลำดับ 001,002,003 ..... ออกได้
เอาเป็นว่าลบที่เราอยากลบ เพิ่มที่เราอยากเพิ่มได้ครับ
Code (C#)
public static void ReplaceFileName(string Path, string Cri, System.Collections.Generic.List<string> lst, bool chngformFolder = false, bool delformFolder = false, string strFnt = "", string strBck = "", bool msg = true)
{
new System.Threading.Thread(ReplaceFileName) { IsBackground = true }.Start(new object[] { Path, Cri, lst, chngformFolder, delformFolder, strFnt, strBck, msg });
}
public static void ReplaceFileName(object obj)
{
DateTime d1 = DateTime.Now;
string path = (string)((object[])obj)[0];
string Exp = (((string)((object[])obj)[1]).Trim().Length <= 0) ? "*.*" : "*" + (string)((object[])obj)[1] + "*";
System.Collections.Generic.List<string> strReplace = (System.Collections.Generic.List<string>)((object[])obj)[2];
bool chngformFolder= (bool)((object[])obj)[3];
bool delformFolder = (bool)((object[])obj)[4];
string strFnt = (string)((object[])obj)[5];
string strBck = (string)((object[])obj)[6];
bool msg = (bool)((object[])obj)[7];
System.Collections.Generic.Stack<string> stack = new System.Collections.Generic.Stack<string>();
stack.Push(path);
while ((stack.Count > 0))
{
string dir = stack.Pop();
foreach (string file in System.IO.Directory.GetFiles(dir, Exp))
{
string _file = System.IO.Path.GetFileNameWithoutExtension(file);
foreach(string str in strReplace)
{
_file = _file.Replace(str, "").Trim();
}
_file = (chngformFolder != true) ? _file :System.IO.Path.GetFileName(System.IO.Path.GetDirectoryName(file)) + "_" + _file;
_file = (strFnt.Trim().Length <= 0) ? _file : strFnt.Trim() + _file;
_file = (strBck.Trim().Length <= 0) ? _file : _file + strBck.Trim();
_file = (delformFolder != true) ? _file : _file.Replace(System.IO.Path.GetFileName(System.IO.Path.GetDirectoryName(file)), "");
_file = System.IO.Path.GetDirectoryName(file) + "\\" + _file + System.IO.Path.GetExtension(file);
if (System.IO.File.Exists(_file))
{
string stg;
int i = 1;
do
{
stg = System.IO.Path.GetDirectoryName(_file) + "\\" + System.IO.Path.GetFileNameWithoutExtension(_file) + "_" + i + System.IO.Path.GetExtension(_file);
i++;
} while (System.IO.File.Exists(stg));
_file = stg;
}
try
{System.IO.File.Move(file, _file); }
catch {}
System.Threading.Thread.Sleep(10);
}
string directoryName = null;
foreach (string directoryName_loopVariable in System.IO.Directory.GetDirectories(dir))
{
directoryName = directoryName_loopVariable;
stack.Push(directoryName);
}
System.Threading.Thread.Sleep(10);
}
DateTime d2 = DateTime.Now;
TimeSpan dd = (d2-d1);
if (msg == true) { MessageBox.Show("Complete...\n Time: " + dd.TotalSeconds.ToString("0.000") + " s" ); }
}
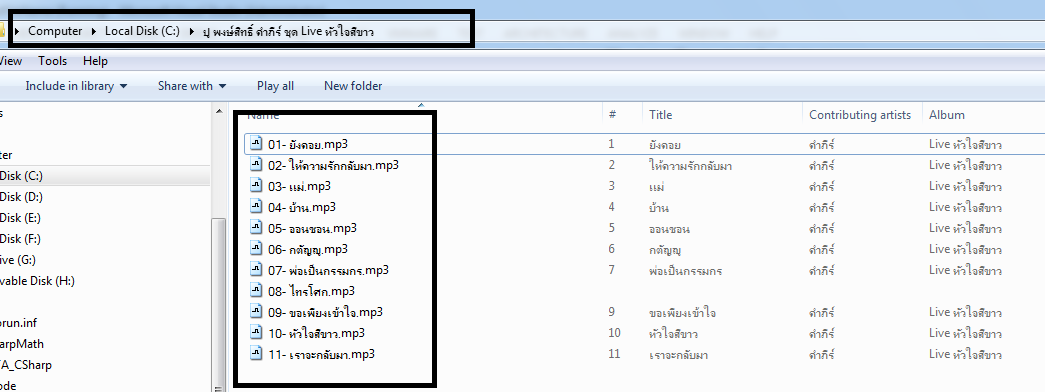
หลังเปลี่ยน
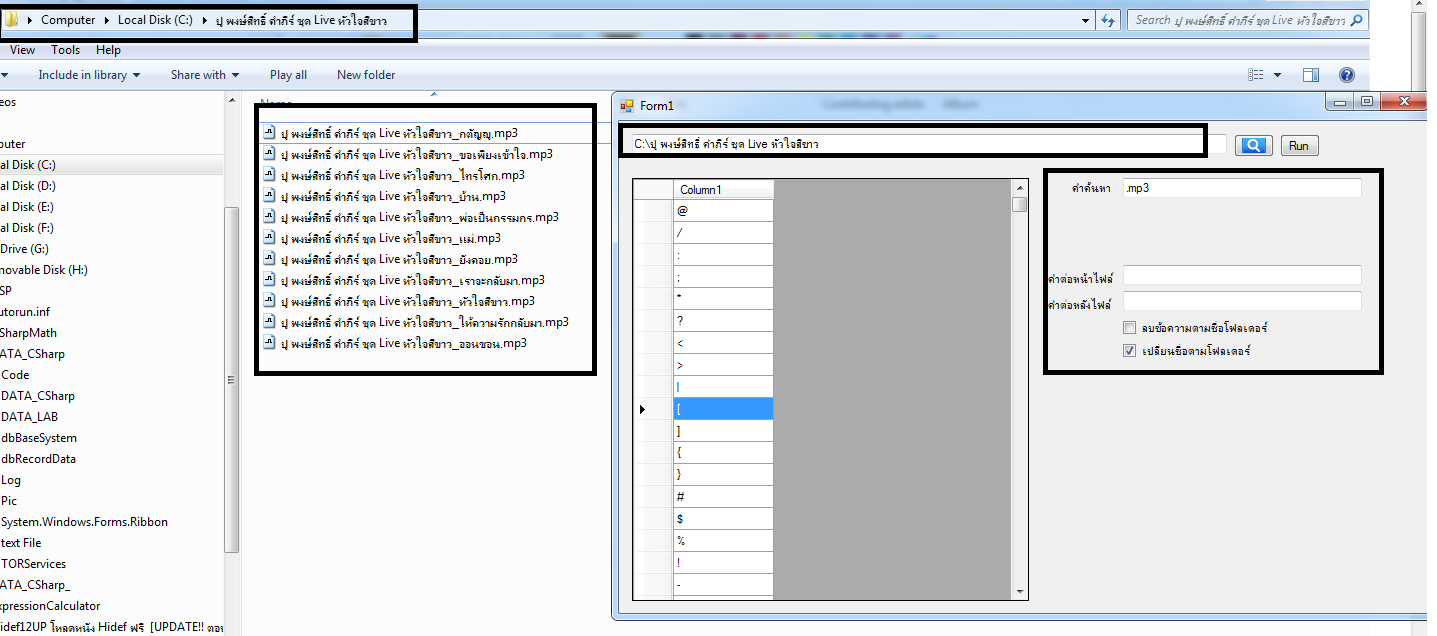
Tag : .NET, Win (Windows App), C#, VS 2012 (.NET 4.x), Windows
|
ประวัติการแก้ไข 2015-07-21 11:21:20
|
 |
 |
 |
 |
Date :
2015-07-21 10:53:38 |
By :
lamaka.tor |
View :
971 |
Reply :
1 |
|
 |
 |
 |
 |
|
|
|
 |