 |
|
ใช้งานบางครั้งก็จะ ERROR ดังรูปด้านล่างครับ
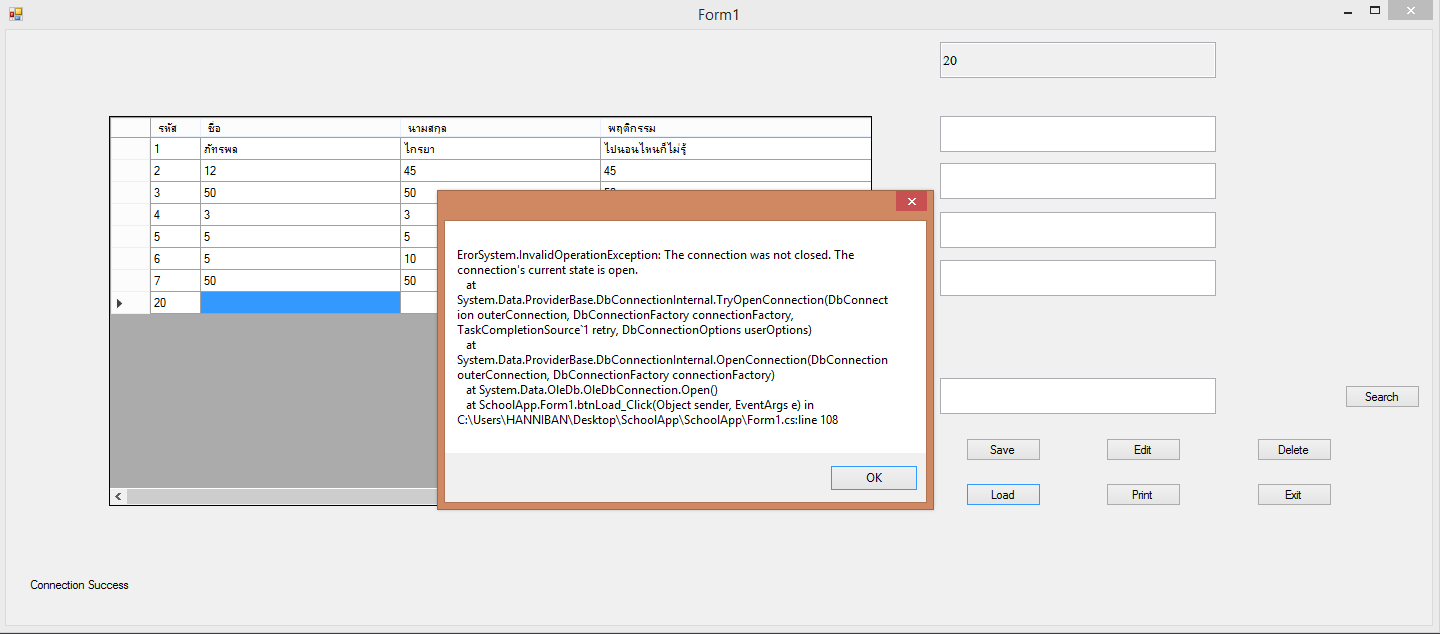
โค้ดครับ
Code (C#)
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Data.OleDb;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace SchoolApp
{
public partial class Form1 : Form
{
private OleDbConnection connection = new OleDbConnection();
public Form1()
{
InitializeComponent();
try {
connection.ConnectionString = @"Provider=Microsoft.Jet.OLEDB.4.0;Data Source=|datadirectory|\Database.mdb";
connection.Open();
label1.Text = "Connection Success";
connection.Close();
}
catch (Exception ex)
{
MessageBox.Show("Error" + ex);
}
}
private void btnSave_Click(object sender, EventArgs e)
{
try {
connection.Open();
OleDbCommand command = new OleDbCommand();
command.Connection = connection;
command.CommandText = "insert into Student (Title,Fname,Lname,Bev) values('"+textBox1.Text.Trim()+ "','" + textBox2.Text.Trim() + "','" + textBox3.Text.Trim() + "','" + textBox4.Text.Trim() + "') ";
command.ExecuteNonQuery();
MessageBox.Show("DATA SAVED");
connection.Close();
Cleardata();
Showdata();
}
catch(Exception ex)
{
MessageBox.Show("เกิดข้อผิดพลาดบางอย่าง"+ex);
}
}
private void btnEdit_Click(object sender, EventArgs e)
{
try
{
connection.Open();
OleDbCommand command = new OleDbCommand();
command.Connection = connection;
string query = "update Student set Title='" + textBox1.Text + "', Fname='" + textBox2.Text + "',Lname='" + textBox3.Text + "',Bev='" + textBox1.Text + "' where ID=" + textBox6.Text + "";
command.CommandText = query;
command.ExecuteNonQuery();
MessageBox.Show("DATA Edit Success");
connection.Close();
Cleardata();
Showdata();
}
catch
{
MessageBox.Show("กรุณากลับไปเลือกและแก้ไขข้อมูลก่อนกดปุ่มแก้ไข");
}
}
private void btnDelete_Click(object sender, EventArgs e)
{
try
{
connection.Open();
OleDbCommand command = new OleDbCommand();
command.Connection = connection;
string query = "delete from Student where ID=" + textBox6.Text + "";
command.CommandText = query;
command.ExecuteNonQuery();
MessageBox.Show("DATA Delete Success");
connection.Close();
Cleardata();
Showdata();
}
catch
{
MessageBox.Show("กรุณากลับไปเลือกข้อมูลก่อนกดปุ่มลบ" );
}
}
private void btnLoad_Click(object sender, EventArgs e)
{
try
{
connection.Open();
OleDbCommand command = new OleDbCommand();
command.Connection = connection;
string query = "select * from Student ";
command.CommandText = query;
OleDbDataAdapter da = new OleDbDataAdapter(command);
DataTable dt = new DataTable();
da.Fill(dt);
dataGridView1.DataSource = dt;
dataGridView1.Columns[0].HeaderText = "รหัส";
dataGridView1.Columns[0].Width = 50;
dataGridView1.Columns[1].HeaderText = "ชื่อ";
dataGridView1.Columns[1].Width = 200;
dataGridView1.Columns[2].HeaderText = "นามสกุล";
dataGridView1.Columns[2].Width = 200;
dataGridView1.Columns[3].HeaderText = "พฤติกรรม";
dataGridView1.Columns[3].Width = 300;
dataGridView1.Columns[4].HeaderText = "วันที่ ";
dataGridView1.Columns[4].Width = 100;
connection.Close();
}
catch (Exception ex)
{
MessageBox.Show("Eror" + ex);
}
}
private void dataGridView1_CellMouseClick(object sender, DataGridViewCellMouseEventArgs e)
{
if (e.RowIndex>=0)
{
DataGridViewRow row = dataGridView1.Rows[e.RowIndex];
textBox1.Text = row.Cells[1].Value.ToString();
textBox2.Text = row.Cells[2].Value.ToString();
textBox3.Text = row.Cells[3].Value.ToString();
textBox4.Text = row.Cells[4].Value.ToString();
textBox6.Text = row.Cells[0].Value.ToString();
}
}
private void btnSearch_Click(object sender, EventArgs e)
{
try
{
connection.Open();
OleDbCommand command = new OleDbCommand();
command.Connection = connection;
string query = "SELECT * FROM Student WHERE Title LIKE '%" + txtSearch.Text + "%'";
command.CommandText = query;
DataTable dt = new DataTable();
OleDbDataAdapter da = new OleDbDataAdapter(command);
da.Fill(dt);
dataGridView1.DataSource = dt;
dataGridView1.Columns[0].HeaderText = "รหัส";
dataGridView1.Columns[0].Width = 50;
dataGridView1.Columns[1].HeaderText = "ชื่อ";
dataGridView1.Columns[1].Width = 200;
dataGridView1.Columns[2].HeaderText = "นามสกุล";
dataGridView1.Columns[2].Width = 200;
dataGridView1.Columns[3].HeaderText = "พฤติกรรม";
dataGridView1.Columns[3].Width = 300;
dataGridView1.Columns[4].HeaderText = "วันที่ ";
dataGridView1.Columns[4].Width = 100;
connection.Close();
}
catch (Exception ex)
{
MessageBox.Show("Eror" + ex);
}
}
private void printDocument1_PrintPage(object sender, System.Drawing.Printing.PrintPageEventArgs e)
{
Bitmap bm = new Bitmap(this.dataGridView1.Width, this.dataGridView1.Height);
dataGridView1.DrawToBitmap(bm, new Rectangle(0, 0, this.dataGridView1.Width, this.dataGridView1.Height));
e.Graphics.DrawImage(bm, 0, 0);
}
private void btnPrint_Click(object sender, EventArgs e)
{
PrintDialog printDialog = new PrintDialog();
printDialog.Document = printDocument1;
printDialog.UseEXDialog = true;
//Get the document
if (DialogResult.OK == printDialog.ShowDialog())
{
printDocument1.DocumentName = "Test Page Print";
printDocument1.Print();
}
}
private void btnExit_Click(object sender, EventArgs e)
{
if (MessageBox.Show("ต้องการปิดโปรแกรม ?", "", MessageBoxButtons.YesNo) == DialogResult.Yes)
{
Application.Exit();
}
}
private void Cleardata()
{
textBox1.Clear(); textBox2.Clear(); textBox3.Clear(); textBox4.Clear(); txtSearch.Clear(); textBox6.Clear();
}
private void Showdata()
{
try
{
connection.Open();
OleDbCommand command = new OleDbCommand();
command.Connection = connection;
string query = "select * from Student ";
command.CommandText = query;
OleDbDataAdapter da = new OleDbDataAdapter(command);
DataTable dt = new DataTable();
da.Fill(dt);
dataGridView1.DataSource = dt;
dataGridView1.Columns[0].HeaderText = "รหัส";
dataGridView1.Columns[0].Width = 50;
dataGridView1.Columns[1].HeaderText = "ชื่อ";
dataGridView1.Columns[1].Width = 200;
dataGridView1.Columns[2].HeaderText = "นามสกุล";
dataGridView1.Columns[2].Width = 200;
dataGridView1.Columns[3].HeaderText = "พฤติกรรม";
dataGridView1.Columns[3].Width = 300;
dataGridView1.Columns[4].HeaderText = "วันที่ ";
dataGridView1.Columns[4].Width = 100;
connection.Close();
}
catch (Exception ex)
{
MessageBox.Show("Eror" + ex);
}
}
}
}
Tag : .NET, Ms Access, C#
|
|
 |
 |
 |
 |
Date :
2015-10-26 19:44:50 |
By :
pkraiya |
View :
984 |
Reply :
2 |
|
 |
 |
 |
 |
|
|
|
 |