 |
|
select ข้อมูล reportList แสดงใน datagrid ครับ................... |
|
 |
|
|
 |
 |
|
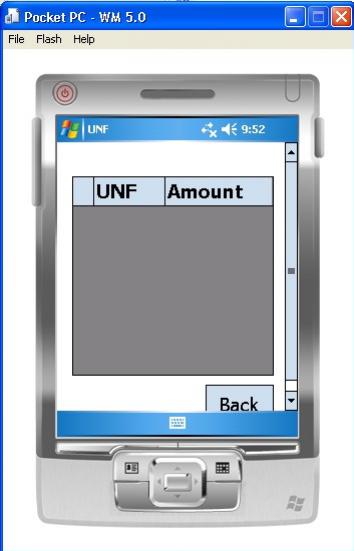
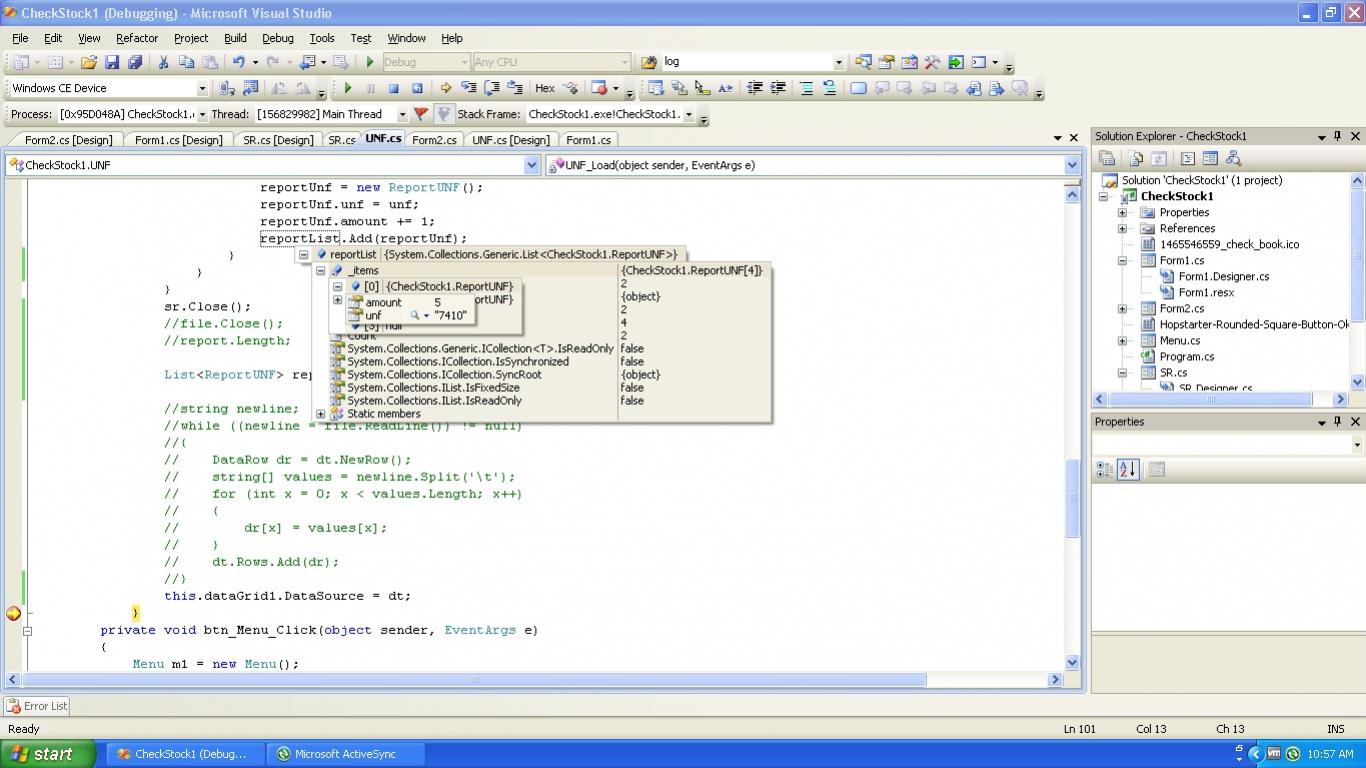
Code (C#)
using System;
using System.Linq;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Text;
using System.Windows.Forms;
using System.IO;
using System.Configuration;
using System.Text.RegularExpressions;
namespace CheckStock1
{
public partial class UNF : Form
{
public string A { get; set; }
public string B { get; set; }
public UNF(string data, string data2)
{
InitializeComponent();
A = data;
B = data2;
}
private void UNF_Load(object sender, EventArgs e)
{
DataGridTableStyle tabStyle = new DataGridTableStyle();
tabStyle.MappingName = "UNF";
DataGridTextBoxColumn col = new DataGridTextBoxColumn();
col.MappingName = "U";
col.HeaderText = "UNF";
col.Width = 70;
DataGridTextBoxColumn col1 = new DataGridTextBoxColumn();
col1.MappingName = "A";
col1.HeaderText = "Amount";
col1.Width = 107;
tabStyle.GridColumnStyles.Add(col);
tabStyle.GridColumnStyles.Add(col1);
this.dataGrid1.TableStyles.Clear();
this.dataGrid1.TableStyles.Add(tabStyle);
string log = "CheckStock.txt";
StreamReader sr = new StreamReader(log);
string[] columnnames = sr.ReadLine().Split('\t');
DataTable dt = new DataTable("UNF");
dt.Columns.Add("U");
dt.Columns.Add("A");
foreach (string c in columnnames)
{
dt.Columns.Add(c);
}
if (File.Exists(log) == false)
{
return;
}
sr = new StreamReader(log);
string line;
List<ReportUNF> reportList = new List<ReportUNF>();
ReportUNF reportUnf;
string unf;
string orderNo;
string containerNo;
while ((line = sr.ReadLine()) != null)
{
int tab_target = line.Split('\t').Length - 2;
int indOfUnf = line.IndexOf(line.Substring(45,4));
unf = line.Substring(indOfUnf,4);
int indOfOrder = line.IndexOf(line.Substring(11, 10));
orderNo = line.Substring(indOfOrder, 10);
int indOfContainer = line.IndexOf(line.Substring(22, 11));
containerNo = line.Substring(indOfContainer, 11);
if (orderNo == A && containerNo == B)
{
if (reportList.Where(v => v.unf == unf).FirstOrDefault() != null)
{
foreach (var r in reportList.Where(v => v.unf == unf))
{
r.amount += 1;
}
}
else
{
reportUnf = new ReportUNF();
reportUnf.unf = unf;
reportUnf.amount += 1;
reportList.Add(reportUnf);
}
}
}
sr.Close();
List<ReportUNF> reportAll = new List<ReportUNF>();
this.dataGrid1.DataSource = dt;
}
private void btn_Menu_Click(object sender, EventArgs e)
{
Menu m1 = new Menu();
m1.Show();
this.Hide();
}
}
public class ReportUNF
{
public virtual string unf { get; set; }
public virtual int amount { get; set; }
}
}
ต้องการให้ข้อมูล reportList แสดงใน datagrid ครับ
Tag : .NET, C#, VS 2008 (.NET 3.x), Mobile
|
|
 |
 |
 |
 |
Date :
2016-07-12 11:10:01 |
By :
bankzbox1 |
View :
933 |
Reply :
7 |
|
 |
 |
 |
 |
|
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ข้อมูลที่ต้องการอยู่บรรทัดที่ 94ครับ
|
 |
 |
 |
 |
Date :
2016-07-12 11:11:31 |
By :
bankzbox1 |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
dt.Columns.Add(c);
หมายความว่า ให้ dt Add Columns เรื่อย
แน่ใจว่าต้องการ Add Columns ไม่ใช่ Add Rows นะครับ
แน่นอนโค้ดที่ใช้ต้องต่างไป
|
 |
 |
 |
 |
Date :
2016-07-12 11:19:34 |
By :
lamaka.tor |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ผมก็ไม่เก่ง นะครับ แต่เท่าที่ดู code นายเอาข้อมูลจาก .txt ยัดเข้าไปใน reportUnf แล้วยังไม่ได้เอา ใส่ใน dt เลย
|
 |
 |
 |
 |
Date :
2016-07-12 13:21:43 |
By :
gaowteen |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
Code (C#)
using System;
using System.Linq;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Text;
using System.Windows.Forms;
using System.IO;
using System.Configuration;
using System.Text.RegularExpressions;
namespace CheckStock1
{
public partial class UNF : Form
{
public string A { get; set; }
public string B { get; set; }
public UNF(string data, string data2)
{
InitializeComponent();
A = data;
B = data2;
}
private void UNF_Load(object sender, EventArgs e)
{
List<ReportUNF> reportList = new List<ReportUNF>();
DataGridTableStyle tableS = new DataGridTableStyle();
tableS.MappingName = reportList.GetType().Name;
DataGridTextBoxColumn tbcName = new DataGridTextBoxColumn();
tbcName.MappingName = "UNF";
tbcName.HeaderText = "UNF";
tbcName.Width = 74;
tableS.GridColumnStyles.Add(tbcName);
DataGridTableStyle tableS1 = new DataGridTableStyle();
tableS.MappingName = reportList.GetType().Name;
DataGridTextBoxColumn tbcName1 = new DataGridTextBoxColumn();
tbcName1.MappingName = "AMOUNT";
tbcName1.HeaderText = "AMOUNT";
tbcName1.Width = 104;
tableS.GridColumnStyles.Add(tbcName1);
dataGrid1.TableStyles.Clear();
dataGrid1.TableStyles.Add(tableS);
dataGrid1.TableStyles.Add(tableS1);
string log = "CheckStock.txt";
StreamReader sr = new StreamReader(log);
string[] row = sr.ReadLine().Split('\t');
if (File.Exists(log) == false)
{
return;
}
sr = new StreamReader(log);
string line;
ReportUNF reportUnf;
string unf;
string orderNo;
string containerNo;
while ((line = sr.ReadLine()) != null)
{
int tab_target = line.Split('\t').Length - 2;
int indOfUnf = line.IndexOf(line.Substring(44,4));
unf = line.Substring(indOfUnf,4);
int indOfOrder = line.IndexOf(line.Substring(11, 10));
orderNo = line.Substring(indOfOrder, 10);
int indOfContainer = line.IndexOf(line.Substring(22, 11));
containerNo = line.Substring(indOfContainer, 11);
if (orderNo == A && containerNo == B)
{
if (reportList.Where(v => v.UNF == unf).FirstOrDefault() != null)
{
foreach (var r in reportList.Where(v => v.UNF == unf))
{
r.AMOUNT += 1;
}
}
else
{
reportUnf = new ReportUNF();
reportUnf.UNF = unf;
reportUnf.AMOUNT += 1;
reportList.Add(reportUnf);
}
}
}
sr.Close();
this.dataGrid1.DataSource = reportList;
}
private void btn_Menu_Click(object sender, EventArgs e)
{
Menu m1 = new Menu();
m1.Show();
this.Hide();
}
}
public class ReportUNF
{
public virtual string UNF { get; set; }
public virtual int AMOUNT { get; set; }
}
}
เสร็จเรียบร้อยครับ ขอบคุนพี่ๆที่ไห้แนวทาง จบไปอีก 1 เดี๋ยวโปรเจคหน้าไห้พี่ๆช่วยใหม่ครับ
|
 |
 |
 |
 |
Date :
2016-07-13 01:50:45 |
By :
bankzbox1 |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
แหล่มครับ
|
 |
 |
 |
 |
Date :
2016-07-13 09:01:45 |
By :
lamaka.tor |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
|
|