 |
|
C# WinApp สอบถามเรื่อง การสร้าง Usercontrol กำหนด property ให้ Usercontrol แต่มันไม่จำค่าที่ใส่ครับ |
|
 |
|
|
 |
 |
|
ผมการสร้าง Usercontrol ขึ้นมาแล้วกำหนด property ให้ Usercontrol แต่มันไม่จำค่าที่ใส่ครับ
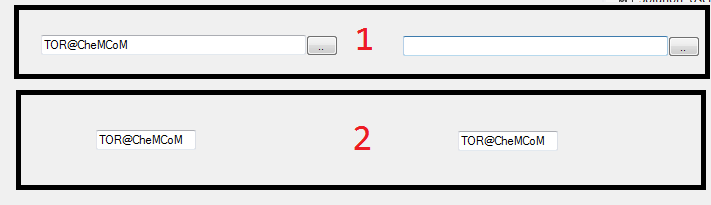
Code 1
Code (C#)
using System;
using System.Collections.Generic;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace UserControl_1
{
class TextBoxOpendialogControl : UserControl
{
public enum SelectedPathType { SelectFolder, SelectFile }
private TextBox txtCode;
private Button btnCode;
private string _path;
[System.ComponentModel.Browsable(true)]
[System.ComponentModel.DefaultValue(false)]
[System.ComponentModel.Category("Behavior")]
[System.ComponentModel.Description("OpenFileDialog is dispalyed and on success the contents of the Cell is replaced with the new file path.")]
public string Path
{
get { return _path; }
set
{
txtCode.Text = value;
}
}
public TextBoxOpendialogControl()
{
this.txtCode = new TextBox();
this.txtCode.TextChanged += new EventHandler(txtCode_TextChanged);
this.Controls.Add(this.txtCode);
this.btnCode = new Button();
this.btnCode.Text = "..";
this.btnCode.Click += new EventHandler(btnCode_Click);
this.Controls.Add(this.btnCode);
this.renderControl();
}
public void renderControl()
{
this.txtCode.Location = new Point(0, 0);
this.txtCode.Width = this.Width + 115;
this.txtCode.Height = this.Height;
this.btnCode.Location = new Point(this.Width + 115, 0);
this.btnCode.Width = 32;
this.btnCode.Height = 21;
}
private void InitializeComponent()
{
this.SuspendLayout();
this.Name = "TextBoxButtonControl";
this.Size = new System.Drawing.Size(267, 176);
this.Leave += new System.EventHandler(this.TextBoxButtonControl_Leave);
this.ResumeLayout(false);
}
private void txtCode_TextChanged(object sender, EventArgs e)
{
if (txtCode.Modified)
{
_path = txtCode.Text;
}
}
private void btnCode_Click(object sender, EventArgs e)
{
//Form2 form2 = new Form2();
//form2.ShowDialog();
OpenFileDialog dialog = new OpenFileDialog();
if (dialog.ShowDialog() == DialogResult.OK)
{
try
{
txtCode.Text = dialog.FileName.ToString();
}
catch (Exception)
{
}
}
}
private void TextBoxButtonControl_Leave(object sender, EventArgs e)
{
try
{
this.Visible = false;
this.txtCode.Text = "";
}
catch (Exception)
{
}
}
}
}
Code 2
Code (C#)
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Drawing;
using System.Data;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace UserControl_1
{
public partial class UserControl1 : UserControl
{
public UserControl1()
{
InitializeComponent();
}
private string _text;
[System.ComponentModel.Browsable(true)]
[System.ComponentModel.DefaultValue("")]
[System.ComponentModel.Category("Behavior")]
[System.ComponentModel.Description("Test User Control by TOR ")]
public string TextCaption
{
get { return _text; }
set
{
_text = value;
textBox1.Text = value;
}
}
}
}
Tag : .NET, Win (Windows App), C#, VS 2012 (.NET 4.x)
|
|
 |
 |
 |
 |
Date :
2016-09-21 10:03:50 |
By :
lamaka.tor |
View :
1097 |
Reply :
2 |
|
 |
 |
 |
 |
|
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
และแล้วก็สำเร็จ
เอามาให้เล่นกันครับ
TextBoxOpendialogControl แบบที่มีทั้ง colorDialog, folderBrowserDialog, fontDialog, openFileDialog, saveFileDialog
Code (C#)
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Drawing;
using System.Data;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace TORServices.Form
{
public partial class TextBoxOpendialogControl : UserControl
{
public enum SelectedPathType { colorDialog, folderBrowserDialog, fontDialog, openFileDialog, saveFileDialog }
private TextBox txtCode;
private Button btnCode;
public string Value
{
get { return txtCode.Text; }
}
[System.ComponentModel.Browsable(true)]
[System.ComponentModel.DefaultValue("..")]
[System.ComponentModel.Category("Behavior")]
[System.ComponentModel.Description("OpenFileDialog is dispalyed and on success the contents of the Cell is replaced with the new file path.")]
public string ButtonCaption
{
get { return btnCode.Text; }
set
{
btnCode.Text = value;
}
}
private bool _selectFolder;
[System.ComponentModel.Browsable(true)]
[System.ComponentModel.DefaultValue(false)]
[System.ComponentModel.Category("Behavior")]
public bool selectFolder
{
get { return _selectFolder; }
set { _selectFolder = value; }
}
private SelectedPathType _selected;
[System.ComponentModel.Browsable(true)]
//[System.ComponentModel.DefaultValue(SelectedPathType.SelectFile)]
[System.ComponentModel.Category("Behavior")]
public SelectedPathType SelectedMode
{
get { return _selected; }
set { _selected = value; }
}
[System.ComponentModel.Browsable(true)]
[System.ComponentModel.DefaultValue(null)]
[System.ComponentModel.Category("Behavior")]
public Image Image { get { return btnCode.Image; } set { btnCode.Image = value; } }
public TextBoxOpendialogControl()
{
InitializeComponent();
this.txtCode = new TextBox();
this.Controls.Add(this.txtCode);
this.btnCode = new Button();
this.btnCode.Text = "..";
btnCode.Dock = DockStyle.Right;
txtCode.Dock = DockStyle.Fill;
txtCode.ReadOnly = true;
this.btnCode.Click += new EventHandler(btnCode_Click);
this.Controls.Add(this.btnCode);
this.renderControl();
}
public void renderControl()
{
this.btnCode.Width = 32;
this.btnCode.Height = 21;
}
private void btnCode_Click(object sender, EventArgs e)
{
try
{
if (_selected == SelectedPathType.folderBrowserDialog)
{
OpenFileDialog dialog = new OpenFileDialog();
if (dialog.ShowDialog() == DialogResult.OK)
{
txtCode.Text = dialog.FileName.ToString();
}
}
else if (_selected == SelectedPathType.openFileDialog)
{
FolderBrowserDialog dialog = new FolderBrowserDialog();
if (dialog.ShowDialog() == DialogResult.OK)
{
txtCode.Text = dialog.SelectedPath;
}
}
else if (_selected == SelectedPathType.colorDialog)
{
ColorDialog dialog = new ColorDialog();
if (dialog.ShowDialog() == DialogResult.OK)
{
txtCode.Text = dialog.Color.ToString() + ":"
+ dialog.Color.Name.ToString()
+ ": A=" + dialog.Color.A + " R=" + dialog.Color.R + " G=" + dialog.Color.G + " B=" + dialog.Color.B;
}
}
else if (_selected == SelectedPathType.fontDialog)
{
FontDialog dialog = new FontDialog();
if (dialog.ShowDialog() == DialogResult.OK)
{
txtCode.Text = dialog.Font.ToString();
}
}
else if (_selected == SelectedPathType.saveFileDialog)
{
SaveFileDialog dialog = new SaveFileDialog();
if (dialog.ShowDialog() == DialogResult.OK)
{
txtCode.Text = dialog.FileName;
}
}
}
catch (Exception)
{
}
}
private void TextBoxButtonControl_Leave(object sender, EventArgs e)
{
try
{
this.Visible = false;
this.txtCode.Text = "";
}
catch (Exception)
{
}
}
}
}
|
 |
 |
 |
 |
Date :
2016-09-21 11:37:34 |
By :
lamaka.tor |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
เพิ่มเติมอีกนิด
txtCode.BackColor = dialog.Color;//จะได้ดูสีที่เลือก
Code (C#)
else if (_selected == SelectedPathType.colorDialog)
{
ColorDialog dialog = new ColorDialog();
if (dialog.ShowDialog() == DialogResult.OK)
{
txtCode.Text = dialog.Color.ToString() + ":"
+ dialog.Color.Name.ToString()
+ ": A=" + dialog.Color.A + " R=" + dialog.Color.R + " G=" + dialog.Color.G + " B=" + dialog.Color.B;
txtCode.BackColor = dialog.Color;
}
}
แต่ตอนนี้ก็มาติดอีกว่า
จะหาวิธีให้ txtCode.ForeColor เป็นสีตัดกับ txtCode.BackColor ได้ยังไง
ปัญหามีกันต่อไปเรื่อย 555
|
 |
 |
 |
 |
Date :
2016-09-21 11:49:59 |
By :
lamaka.tor |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
|
|