 |
|
C# WinApp สอบถามเรื่อง การสร้าง Usercontrol อยากทราบ Event ของ DataGridView ครับ |
|
 |
|
|
 |
 |
|
จากกระทู้นี้ครับ
https://www.thaicreate.com/dotnet/forum/124690.html
และรูปนี้
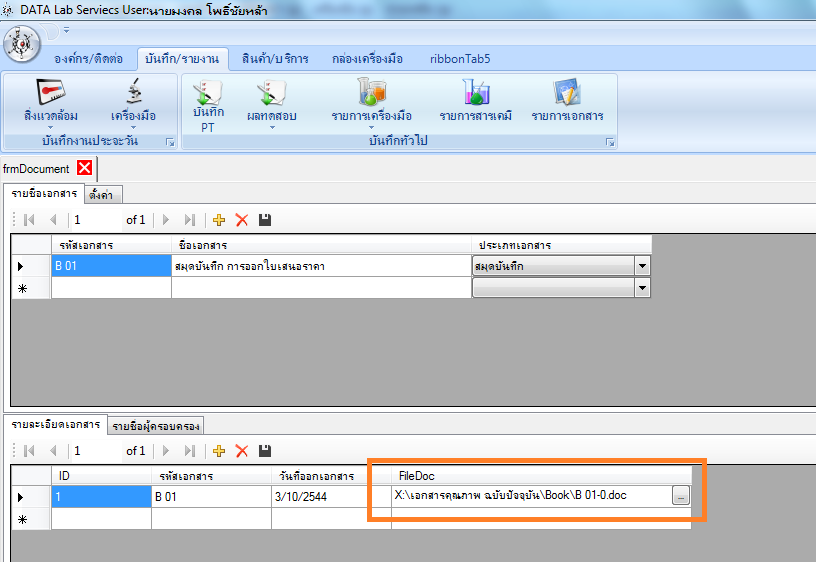
ผมไม่แน่ใจว่าจะสื่อปัญหาถูกไม๊(เพราะตอนนี้ผมก็งงกับปัญหาเช่นกัน)
คือถ้าจากโค้ด
Code (C#)
void browseButton_Click(object sender, EventArgs e)
{
..................
...........................
}
ผมอยากเอาไปใช้งานในรูปแบบ Event ของ DataGridView ครับ
คือปัญหาเกิดจาก เวลา user คลิกปุ่มเลือกไฟล์ แล้ว
ผมหา Event ของ DataGridView ที่ใช้งานไม่เจอ
Code (C#)
private void doncument_listDetailDataGridView_CellClick(object sender, DataGridViewCellEventArgs e)
{
if (e.ColumnIndex == 3)
MessageBox.Show("3 5555");
}
private void doncument_listDetailDataGridView_CellValueChanged(object sender, DataGridViewCellEventArgs e)
{
/* if (e.ColumnIndex == 3)
{
MessageBox.Show("3");
if (System.IO.Path.GetDirectoryName((string)doncument_listDetailDataGridView[1, e.RowIndex].Value) != TORServices.PathFile.PathData.Path_Document)
{
System.IO.File.Move((string)doncument_listDetailDataGridView[1, e.RowIndex].Value, TORServices.PathFile.PathData.Path_Document + "\\" + System.IO.Path.GetFileName((string)doncument_listDetailDataGridView[1, e.RowIndex].Value));
doncument_listDetailDataGridView[1, e.RowIndex].Value = TORServices.PathFile.PathData.Path_Document + "\\" + System.IO.Path.GetFileName((string)doncument_listDetailDataGridView[1, e.RowIndex].Value);
MessageBox.Show("" + doncument_listDetailDataGridView[1, e.RowIndex].Value);
}
}*/
}
แล้วใน CellEndEdit ก็ไม่ได้เช่นกัน
เท่าที่ผมเดา คือ browseButton_Click ซึ่งไม่ใช่ Event ของ DataGridView
พอคลิกเสร็จเรียบร้อย DataGridView จึงไม่มีอะไรตอบรับ
ผมอยากได้ Event ที่ DataGridView ตอบรับการคลิกของปุ่มครับ
ว่า ฉันคลิกแล้วนะ อะไรประมาณนี้ครับ
ปล.ผมยังไม่รู้ว่าผมมาถูกทางรึยังนะครับ 5555
Tag : .NET, Win (Windows App), C#, VS 2012 (.NET 4.x), Windows
|
|
 |
 |
 |
 |
Date :
2016-10-07 16:23:15 |
By :
lamaka.tor |
View :
2784 |
Reply :
35 |
|
 |
 |
 |
 |
|
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ต่อเรื่องการทำงานกับไฟล์ครับ
ไม่รู้ผมคิดไปเองหรือว่าท่านอื่นเจอปัญหาแบบผมไม๊
วันนี้มานั่งทำเกียวกับไฟล์เอกสารของ หน่วยควบคุมเลยมานั่งๆคิดๆว่า
1.กรณีเราเลือกไฟล์มาแล้วเช่น X:\xxx\xx.doc แต่เราก็อยากให้เอาไปไว้ใน โฟลเดอร์จัดเก็บ X:\1311
ส่วนใหญ่ ท่านๆจะ copy หรือ move แล้วควรจะทำเลยหรือรอทำตอน save data
2. จากข้อ 1. ถ้า X:\1311 มีไฟล์อยู่แล้ว เราควรจะตัดสินยังไงครับ รึปล่อยให้ user จัดการ rename,overwrite,not move ฯลฯ เอง
3. ข้อคิดในการจัดการกับไฟล์ซ้ำ แต่อยู่คนละโฟลเดอร์ ครับ ควรทำยังไง
คิดไปคิดมาสงสัยอาจจะต้องเพิ่ม คลาส เกี่ยวกับไฟล์ อีกรึป่าวก็ไม่รู้ครับ
ท่าทางช่วงนี้จะเล่นเหล้าขาวหนักไปหน่อย สมองเริ่มจะ ตีบตันตื้อตึงตุนโตงเตง ไปหมดแล้ว 555
|
 |
 |
 |
 |
Date :
2016-10-07 16:33:38 |
By :
lamaka.tor |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ใช้ตัวนี้ได้ไหมครับ ปกติผมใช้ตัวนี้เลย
Code (VB.NET)
private int pCase;
private void SelectorClick(object sender, EventArgs e)
{
switch (pCase) {
case 1:
//value from dataGridView1_CellEnter
if (true) {
MessageBox.Show("Show Dialog here!!");
break; // TODO: might not be correct. Was : Exit Select
}
break;
}
}
private void DataGridView1_CellEnter(object sender, System.Windows.Forms.DataGridViewCellEventArgs e)
{
if (DataGridView1.Columns(e.ColumnIndex).Name == "Column1") {
pCase = 1;
}
}
|
ประวัติการแก้ไข 2016-10-07 17:44:40
 |
 |
 |
 |
Date :
2016-10-07 17:42:48 |
By :
TheCom |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
เล่นหูเล่นตากับอีเวนต์ (Events)
User Control
Code (VB.NET)
Public Class yourUserControl
Protected Friend Event btnงามClick As EventHandler
Private Sub btnหอย_Click(sender As Object, e As EventArgs) Handles btnหอย.Click
RaiseEvent btnงามClick(sender, e) '*****
End Sub
End Class
Forms XXX
Code (VB.NET)
Dim รักจิ่ม As New yourUserControl
Me.Controls.Add(รักจิ่ม)
AddHandler รักจิ่ม.btnงามClick, Sub(หอย, งาม)
MsgBox(DirectCast(หอย, Button).Name)
End Sub
|
 |
 |
 |
 |
Date :
2016-10-07 21:45:54 |
By :
หน้าฮี |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
เล่าเรื่องจริงและตลกให้ฟังนะ
--- วันนี้ ผมเจอผู้หญิงอายุ 25 - 45 ปี หลายสิบคน
--- สิบในนั้นเรียกผมว่า คุณตา ผมนี่สะอึกเลย
--- สิบในนั้นเรียกผมว่า คุณลุง ผมนี่สะอึกเลย
--- สิบในนั้นเรียกผมว่า น้าฯ ผมนี่สะอึกเลย
...
...
...
สาววัยมันส์ คิดอะไรของมันหว่า?
...
...
...
กลับถึงบ้าน ผมแอบคิดอยู่ในใจ (เมียตู... ผ่านวัย 18 ปีมาได้ไม่กี่วัน เรียกตูว่า ผัวจ๋า ทุกคำ)
ปล. ...
|
 |
 |
 |
 |
Date :
2016-10-07 22:20:47 |
By :
หน้าฮี |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
Code (C#)
public event EventHandler browseButtonClick;
void browseButton_Click(object sender, EventArgs e)
{
DataGridViewDialogColumn filePathColumn = (DataGridViewDialogColumn)this.DataGridView.Columns[ColumnIndex];
try
{
if (filePathColumn.SelectedMode == DataGridViewDialogColumn.SelectedPathType.OpenFileDialog)
{
OpenFileDialog dialog = new OpenFileDialog();
if (dialog.ShowDialog() == DialogResult.OK)
{
base.Value = dialog.FileName.ToString();
}
}
else if (filePathColumn.SelectedMode == DataGridViewDialogColumn.SelectedPathType.folderDialog)
{
FolderBrowserDialog dialog = new FolderBrowserDialog();
if (dialog.ShowDialog() == DialogResult.OK)
{
base.Value = dialog.SelectedPath;
}
}
else if (filePathColumn.SelectedMode == DataGridViewDialogColumn.SelectedPathType.colorDialog)
{
ColorDialog dialog = new ColorDialog();
if (dialog.ShowDialog() == DialogResult.OK)
{
base.Value = dialog.Color.ToString() + ":"
+ dialog.Color.Name.ToString()
+ ": A=" + dialog.Color.A + " R=" + dialog.Color.R + " G=" + dialog.Color.G + " B=" + dialog.Color.B;
}
}
else if (filePathColumn.SelectedMode == DataGridViewDialogColumn.SelectedPathType.fontDialog)
{
FontDialog dialog = new FontDialog();
if (dialog.ShowDialog() == DialogResult.OK)
{
base.Value = dialog.Font.ToString();
}
}
else if (filePathColumn.SelectedMode == DataGridViewDialogColumn.SelectedPathType.SaveFileDialog)
{
SaveFileDialog dialog = new SaveFileDialog();
if (dialog.ShowDialog() == DialogResult.OK)
{
base.Value = dialog.FileName;
}
}
}
catch (Exception)
{
}
EventHandler _browseButtonClick = this.browseButtonClick;
}
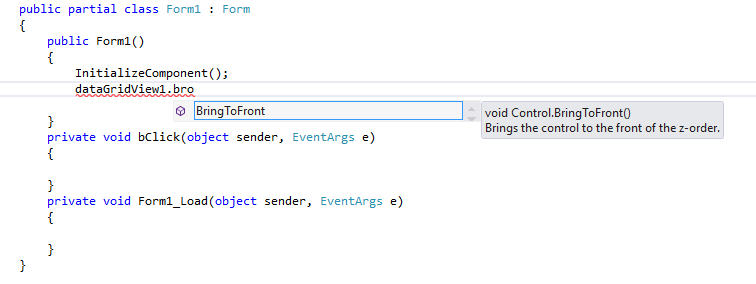
ทำไมใช้ browseButtonClick ไม่ได้รึครับ
|
 |
 |
 |
 |
Date :
2016-10-13 14:22:17 |
By :
lamaka.tor |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ผมมาเริ่มศึกษา event ใหม่ครับ
Code (C#)
namespace UserControl_1
{
public partial class UserControl2 : UserControl
{
public UserControl2()
{
InitializeComponent();
}
protected internal event EventHandler browseButtonClick;
private void button1_Click(object sender, EventArgs e)
{
if (browseButtonClick != null)
{
browseButtonClick(this, e);
}
}
}
}
Code (C#)
namespace UserControl_1
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void userControl21_browseButtonClick(object sender, EventArgs e)
{
MessageBox.Show("sfdsg");
}
}
}
เหมือนจะได้นะครับ แต่กับ datagrid ยังงงๆ อยู่เลย
|
 |
 |
 |
 |
Date :
2016-10-13 16:16:04 |
By :
lamaka.tor |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
จาก No. 12
เท่าที่เดา(ล้วนๆ)
ผมว่า
browseButton_Click มันอยู่ใน DataGridViewDialogCell ซึ่งจะอยู่ใน DataGridViewDialogColumn แล้วก็อยู่ใน
DataGridView อีกที(usercontrol ใน usercontrol ซ้อน usercontrol ซ้อน usercontrol )
ต่างจาก No. 12 ที่ button1_Click อยู่ใน UserControl2 เลยครับ
|
 |
 |
 |
 |
Date :
2016-10-13 16:31:48 |
By :
lamaka.tor |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
Code (C#)
protected override void OnClick(DataGridViewCellEventArgs e)
{
base.OnClick(e);
}
void browseButton_Click(object sender, EventArgs e)
{
// อยากใช้ base.OnClick(e); ได้มั่งครับ แต่ติด DataGridViewCellEventArgs ไม่รู้จะเอามาจากไหนครับ
}
|
 |
 |
 |
 |
Date :
2016-10-15 11:10:33 |
By :
lamaka.tor |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ตอนนี้สามารถให้ ฟอร์ม เรียกใช้ Event ได้แล้วครับ
Code (C#)
using System;
using System.Collections.Generic;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace TORServices.Forms.Datagridview
{
#region _DataGridViewDialog
/// <summary>
/// Hosts a collection of DataGridViewTextBoxCell cells.
/// </summary>
public class DataGridViewDialogColumn : System.Windows.Forms.DataGridViewColumn
{
private bool showBrowseButton;
[System.ComponentModel.Browsable(true)]
// [System.ComponentModel.EditorBrowsable(System.ComponentModel.EditorBrowsableState.Always)]
[System.ComponentModel.DefaultValue(false)]
[System.ComponentModel.Category("Appearance")]
[System.ComponentModel.Description("Show a button in each cell for browsing for files.")]
public enum SelectedPathType { colorDialog, folderDialog, fontDialog, OpenFileDialog, SaveFileDialog }
public bool ShowBrowseButton
{
get { return showBrowseButton; }
set
{
showBrowseButton = value;
}
}
protected internal event EventHandler ButtonColumnClick;
public virtual void browseColumnButton_Click(object sender, EventArgs e)
{
if(ButtonColumnClick != null)
{
ButtonColumnClick(this, e);
}
}
public override object Clone()
{
var clm = base.Clone() as DataGridViewDialogColumn;
DataGridViewDialogColumn xxx = base.Clone() as DataGridViewDialogColumn;
if (clm != null)
{
clm.SelectedMode = _selected;
clm.ShowBrowseButton = showBrowseButton;
}
return clm;
}
private bool useOpenFileDialogOnButtonClick;
[System.ComponentModel.Browsable(true)]
[System.ComponentModel.EditorBrowsable(System.ComponentModel.EditorBrowsableState.Always)]
[System.ComponentModel.DefaultValue(false)]
[System.ComponentModel.Category("Behavior")]
[System.ComponentModel.Description("Dialog is dispalyed and on success the contents of the Cell is replaced with the new file path.")]
public bool UseOpenFileDialogOnButtonClick
{
get { return useOpenFileDialogOnButtonClick; }
set
{
useOpenFileDialogOnButtonClick = value;
}
}
private SelectedPathType _selected;
[System.ComponentModel.Browsable(true)]
//[System.ComponentModel.EditorBrowsable(System.ComponentModel.EditorBrowsableState.Always)]
// [System.ComponentModel.DefaultValue(SelectedPathType.OpenFileDialog)]
[System.ComponentModel.Category("Behavior")]
[System.ComponentModel.Description("Open Dialog is dispalyed and on success the contents of the Cell is replaced with the new path.")]
public SelectedPathType SelectedMode
{
get { return _selected; }
set
{
_selected = value;
}
}
public DataGridViewDialogColumn()
: base(new DataGridViewDialogCell())
{
}
public override System.Windows.Forms.DataGridViewCell CellTemplate
{
get
{
return base.CellTemplate;
}
set
{
if (null != value &&
!value.GetType().IsAssignableFrom(typeof(DataGridViewDialogCell)))
{
throw new InvalidCastException("must be a DataGridViewDialogCell");
}
base.CellTemplate = value;
}
}
}
/// <summary>
/// Displays editable text information in a DataGridView control. Uses
/// PathEllipsis formatting if the column is smaller than the width of a
/// displayed filesystem path.
/// </summary>
public class DataGridViewDialogCell : DataGridViewLinkCell
{
public Button browseButton;
Dictionary<Color, SolidBrush> brushes = new Dictionary<Color, SolidBrush>();
protected virtual SolidBrush GetCachedBrush(Color color)
{
if (this.brushes.ContainsKey(color))
return this.brushes[color];
SolidBrush brush = new SolidBrush(color);
this.brushes.Add(color, brush);
return brush;
}
protected virtual bool RightToLeftInternal
{
get
{
return this.DataGridView.RightToLeft == RightToLeft.Yes;
}
}
protected override void OnClick(DataGridViewCellEventArgs e)
{
base.OnClick(e);
if (this.DataGridView.CurrentCell == this)
{
string _Value = Convert.ToString(base.Value);
DataGridViewDialogColumn filePathColumn = (DataGridViewDialogColumn)this.DataGridView.Columns[ColumnIndex];
if (filePathColumn.SelectedMode == DataGridViewDialogColumn.SelectedPathType.OpenFileDialog)
{
if (!System.IO.File.Exists(_Value))
{ MessageBox.Show("ไม่พบ " + _Value); }
else
{ System.Diagnostics.Process.Start(_Value); }
}
if (filePathColumn.SelectedMode == DataGridViewDialogColumn.SelectedPathType.folderDialog)
{
if (!System.IO.Directory.Exists(_Value))
{ MessageBox.Show("ไม่พบ " + _Value); }
else
{ System.Diagnostics.Process.Start(_Value); }
}
}
}
protected override void Paint(Graphics graphics, Rectangle clipBounds, Rectangle cellBounds, int rowIndex, DataGridViewElementStates cellState, object value, object formattedValue, string errorText, DataGridViewCellStyle cellStyle, DataGridViewAdvancedBorderStyle advancedBorderStyle, DataGridViewPaintParts paintParts)
{
if (cellStyle == null) { throw new ArgumentNullException("cellStyle"); }
this.PaintPrivate(graphics, clipBounds, cellBounds, rowIndex, cellState, formattedValue, errorText, cellStyle, advancedBorderStyle, paintParts);
}
protected Rectangle PaintPrivate(Graphics graphics, Rectangle clipBounds, Rectangle cellBounds, int rowIndex, DataGridViewElementStates cellState, object formattedValue, string errorText, DataGridViewCellStyle cellStyle, DataGridViewAdvancedBorderStyle advancedBorderStyle, DataGridViewPaintParts paintParts)
{
System.Diagnostics.Debug.WriteLine(string.Format("Painting Cell row {0} for rowindex {2} with rectangle {1}", this.RowIndex, cellBounds, rowIndex));
SolidBrush cachedBrush;
Rectangle empty = Rectangle.Empty;
if (((paintParts & DataGridViewPaintParts.Border) != DataGridViewPaintParts.None)) { this.PaintBorder(graphics, clipBounds, cellBounds, cellStyle, advancedBorderStyle); }
Rectangle rectangle2 = this.BorderWidths(advancedBorderStyle);
Rectangle borderedCellRectangle = cellBounds;
borderedCellRectangle.Offset(rectangle2.X, rectangle2.Y);
borderedCellRectangle.Width -= rectangle2.Right;
borderedCellRectangle.Height -= rectangle2.Bottom;
Point currentCellAddress = base.DataGridView.CurrentCellAddress;
bool isFirstCell = (currentCellAddress.X == base.ColumnIndex) && (currentCellAddress.Y == rowIndex);
bool flagisFirstCellAndNotEditing = isFirstCell && (base.DataGridView.EditingControl != null);
bool thisCellIsSelected = (cellState & DataGridViewElementStates.Selected) != DataGridViewElementStates.None;
cachedBrush = ((((paintParts & DataGridViewPaintParts.SelectionBackground) != DataGridViewPaintParts.None) && thisCellIsSelected) && !flagisFirstCellAndNotEditing) ? GetCachedBrush(cellStyle.SelectionBackColor) : GetCachedBrush(cellStyle.BackColor);
if (((((paintParts & DataGridViewPaintParts.Background) != DataGridViewPaintParts.None)) && ((cachedBrush.Color.A == 0xff) && (borderedCellRectangle.Width > 0))) && (borderedCellRectangle.Height > 0))
{
graphics.FillRectangle(cachedBrush, borderedCellRectangle);
}
if (cellStyle.Padding != Padding.Empty)
{
if (RightToLeftInternal)
{
borderedCellRectangle.Offset(cellStyle.Padding.Right, cellStyle.Padding.Top);
}
else
{
borderedCellRectangle.Offset(cellStyle.Padding.Left, cellStyle.Padding.Top);
}
borderedCellRectangle.Width -= cellStyle.Padding.Horizontal;
borderedCellRectangle.Height -= cellStyle.Padding.Vertical;
}
if (((isFirstCell) && (!flagisFirstCellAndNotEditing && ((paintParts & DataGridViewPaintParts.Focus) != DataGridViewPaintParts.None))) && ((ShowFocusCues && base.DataGridView.Focused) && ((borderedCellRectangle.Width > 0) && (borderedCellRectangle.Height > 0))))
{
ControlPaint.DrawFocusRectangle(graphics, borderedCellRectangle, Color.Empty, cachedBrush.Color);
}
Rectangle cellValueBounds = borderedCellRectangle;
string text = formattedValue as string;
if ((text != null) && (!flagisFirstCellAndNotEditing))
{
int y = (cellStyle.WrapMode == DataGridViewTriState.True) ? 1 : 2;
borderedCellRectangle.Offset(0, y);
borderedCellRectangle.Width = borderedCellRectangle.Width;
borderedCellRectangle.Height -= y + 1;
if ((borderedCellRectangle.Width > 0) && (borderedCellRectangle.Height > 0))
{
TextFormatFlags flags = TextFormatFlags.PathEllipsis;
if (((paintParts & DataGridViewPaintParts.ContentForeground) != DataGridViewPaintParts.None))
{
if ((flags & TextFormatFlags.SingleLine) != TextFormatFlags.GlyphOverhangPadding) { flags |= TextFormatFlags.EndEllipsis; }
DataGridViewDialogColumn filePathColumn = (DataGridViewDialogColumn)this.DataGridView.Columns[ColumnIndex];
if (this.RowIndex >= 0)
{
bool changed = false;
if ((browseButton.Width != System.Math.Max(10, borderedCellRectangle.Width / 4)) && (browseButton.Width != 20))
{
System.Diagnostics.Trace.WriteLine(string.Format("browseButton Width was incorrect:{0} for given rectangle:{1}", browseButton.Width, borderedCellRectangle));
browseButton.Width = System.Math.Max(10, borderedCellRectangle.Width / 4);
browseButton.Width = System.Math.Min(browseButton.Width, 20);
changed = true;
}
if (browseButton.Height != (borderedCellRectangle.Height + 4))
{
System.Diagnostics.Trace.WriteLine(string.Format("browseButton Height was incorrect:{0} for given rectangle:{1}", browseButton.Height, borderedCellRectangle));
browseButton.Height = borderedCellRectangle.Height + 4;
changed = true;
}
Point loc = new Point();
loc.X = borderedCellRectangle.X + borderedCellRectangle.Width - browseButton.Width;
loc.Y = borderedCellRectangle.Y - 4;
if (browseButton.Location != loc)
{
System.Diagnostics.Trace.WriteLine(string.Format("browseButton location was incorrect:{0} for given rectangle:{1} with loc: {2}", browseButton.Location, borderedCellRectangle, loc));
browseButton.Location = loc;
changed = true;
}
if (changed)
browseButton.Invalidate();
if (!this.DataGridView.Controls.Contains(browseButton))
this.DataGridView.Controls.Add(browseButton);
borderedCellRectangle.Width -= browseButton.Width;
}
}
TextRenderer.DrawText(graphics, text, cellStyle.Font, borderedCellRectangle, thisCellIsSelected ? cellStyle.SelectionForeColor : cellStyle.ForeColor, flags);
}
}
if ((base.DataGridView.ShowCellErrors) && ((paintParts & DataGridViewPaintParts.ErrorIcon) != DataGridViewPaintParts.None))
{
if ((!string.IsNullOrEmpty(errorText) && (cellValueBounds.Width >= 20)) && (cellValueBounds.Height >= 0x13))
{
Rectangle iconBounds = this.GetErrorIconBounds(graphics, cellStyle, rowIndex);
if ((iconBounds.Width >= 4) && (iconBounds.Height >= 11))
{
iconBounds.X += cellBounds.X;
iconBounds.Y += cellBounds.Y;
Bitmap errorBitmap = new Bitmap(typeof(DataGridViewCell), "DataGridViewRow.error.bmp");
errorBitmap.MakeTransparent();
if (errorBitmap != null)
{
lock (errorBitmap)
{
graphics.DrawImage(errorBitmap, iconBounds, 0, 0, 12, 11, GraphicsUnit.Pixel);
}
}
}
}
}
return empty;
}
public bool ShowFocusCues
{
get { return true; }
}
protected bool ApplyVisualStylesToHeaders
{
get
{
if (Application.RenderWithVisualStyles)
{
return this.DataGridView.EnableHeadersVisualStyles;
}
return false;
}
}
/* private void DataGridView_CellClick(object sender, DataGridViewCellEventArgs e)
{
if (base.Value.ToString().Length > 0)
{
System.Diagnostics.Process.Start(base.Value.ToString());
}
}*/
// protected internal event EventHandler ButtonCellClick;
void browseButton_Click(object sender, EventArgs e)
{
DataGridViewDialogColumn filePathColumn = (DataGridViewDialogColumn)this.DataGridView.Columns[ColumnIndex];
try
{
if (filePathColumn.SelectedMode == DataGridViewDialogColumn.SelectedPathType.OpenFileDialog)
{
OpenFileDialog dialog = new OpenFileDialog();
if (dialog.ShowDialog() == DialogResult.OK)
{
base.Value = dialog.FileName.ToString();
}
}
else if (filePathColumn.SelectedMode == DataGridViewDialogColumn.SelectedPathType.folderDialog)
{
FolderBrowserDialog dialog = new FolderBrowserDialog();
if (dialog.ShowDialog() == DialogResult.OK)
{
base.Value = dialog.SelectedPath;
}
}
else if (filePathColumn.SelectedMode == DataGridViewDialogColumn.SelectedPathType.colorDialog)
{
ColorDialog dialog = new ColorDialog();
if (dialog.ShowDialog() == DialogResult.OK)
{
base.Value = dialog.Color.ToString() + ":"
+ dialog.Color.Name.ToString()
+ ": A=" + dialog.Color.A + " R=" + dialog.Color.R + " G=" + dialog.Color.G + " B=" + dialog.Color.B;
}
}
else if (filePathColumn.SelectedMode == DataGridViewDialogColumn.SelectedPathType.fontDialog)
{
FontDialog dialog = new FontDialog();
if (dialog.ShowDialog() == DialogResult.OK)
{
base.Value = dialog.Font.ToString();
}
}
else if (filePathColumn.SelectedMode == DataGridViewDialogColumn.SelectedPathType.SaveFileDialog)
{
SaveFileDialog dialog = new SaveFileDialog();
if (dialog.ShowDialog() == DialogResult.OK)
{
base.Value = dialog.FileName;
}
}
}
catch (Exception)
{
}
filePathColumn.browseColumnButton_Click(this, e);
/* if (ButtonCellClick != null)
{
ButtonCellClick(this, e);
}*/
}
public DataGridViewDialogCell()
: base()
{
browseButton = new Button();
browseButton.Text = "…";
browseButton.Click += new EventHandler(browseButton_Click);
}
}
#endregion
}
Code (C#)
private void Form1_Load(object sender, EventArgs e)
{
userControl21.browseButtonClick+=new EventHandler(this.userControl21_browseButtonClick);
Column1.ButtonColumnClick += new EventHandler(this.button1_Click);
}
private void button1_Click(object sender, EventArgs e)
{
MessageBox.Show("55555");
}
แต่ที่ไม่ชอบใจคือ ต้องเรียกใช้จาก Column1 อยากทราบว่าเราจะให้เรียกใช้ผ่าน dataGridView1 ยังไงครับ
และอีกอย่างครับ
ผมต้องการให้โชว์ event ใน event ของ dataGridView1 ต้องทำยังไงครับ
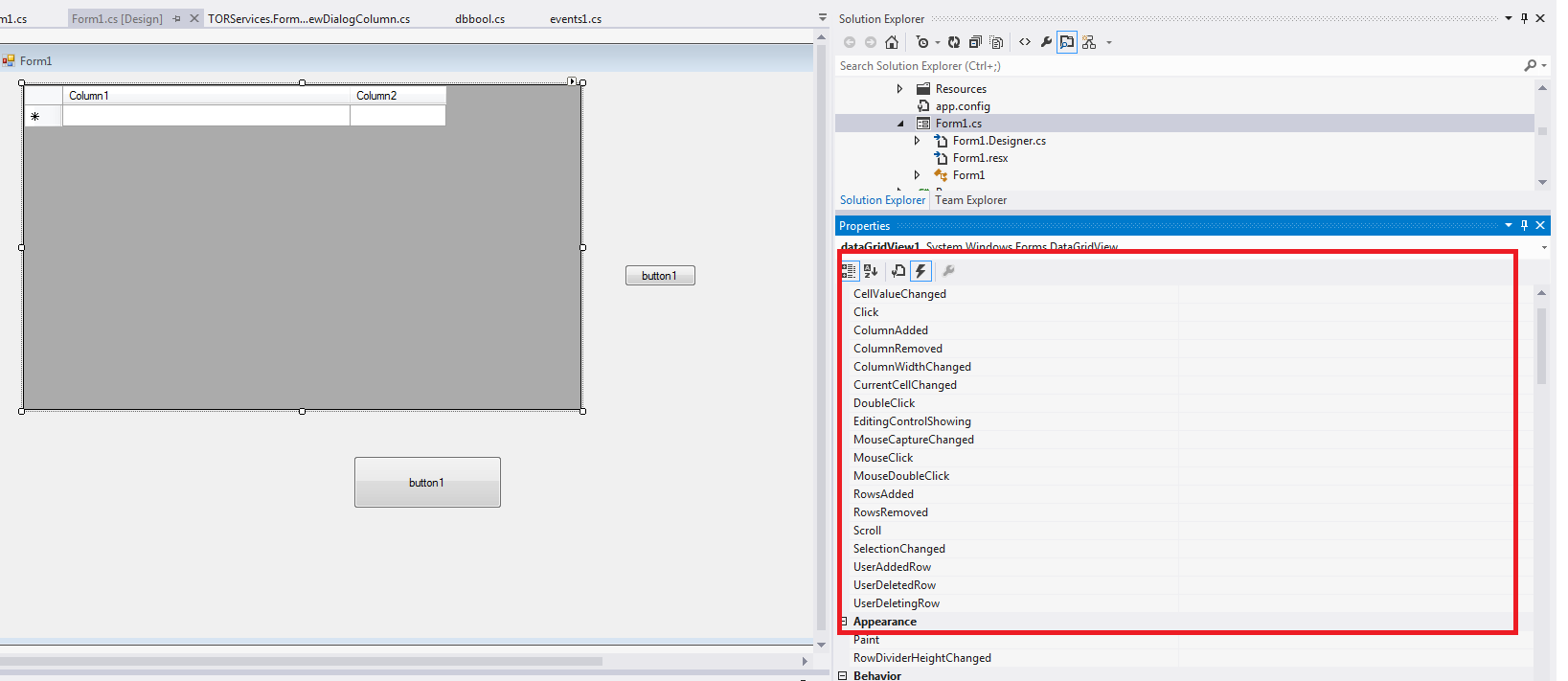
|
 |
 |
 |
 |
Date :
2016-10-17 00:26:51 |
By :
lamaka.tor |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ถ้าเข้าใจไม่ผิด
จะทำปุ่ม Browse เล็ก ๆ ใน Textbox เพื่อกดเลือกไฟล์ใช่ไหมครับ
|
 |
 |
 |
 |
Date :
2016-10-17 08:16:57 |
By :
fonfire |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
Browse เล็ก ๆ ใน Datagrid ครับ
แต่มันต้องทำใน Column และ cell ครับ
|
 |
 |
 |
 |
Date :
2016-10-17 08:58:00 |
By :
lamaka.tor |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ผมลองสร้าง event ใน textbox ก็ไม่ขึ้นมาเหมือนกันครับ
Code (C#)
using System;
using System.Collections.Generic;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace TORServices.Forms
{
class TextboxDialog:TextBox
{
Button browseButton;
public enum SelectedPathType { colorDialog, folderDialog, fontDialog, OpenFileDialog, SaveFileDialog }
private SelectedPathType _selected;
[System.ComponentModel.Browsable(true)]
[System.ComponentModel.Category("Behavior")]
[System.ComponentModel.Description("Open Dialog is dispalyed and on success the contents of the Cell is replaced with the new path.")]
public SelectedPathType SelectedMode
{
get { return _selected; }
set
{
_selected = value;
}
}
protected override void OnDoubleClick(EventArgs e)
{
base.OnDoubleClick(e);
if (_selected == SelectedPathType.OpenFileDialog)
{
if (!System.IO.File.Exists(this.Text))
{ MessageBox.Show("ไม่พบ " + this.Text); }
else
{ System.Diagnostics.Process.Start(this.Text); }
}
if (_selected == SelectedPathType.folderDialog)
{
if (!System.IO.Directory.Exists(this.Text))
{ MessageBox.Show("ไม่พบ " + this.Text); }
else
{ System.Diagnostics.Process.Start(this.Text); }
}
}
protected override void OnResize(EventArgs e)
{
base.OnResize(e);
browseButton.Size = new Size(28, this.ClientSize.Height + 2);
browseButton.Location = new Point(this.ClientSize.Width - browseButton.Width, -1);
}
protected internal event EventHandler ButtonCellClick;
void browseButton_Click(object sender, EventArgs e)
{
if (_selected == SelectedPathType.OpenFileDialog)
{
using (OpenFileDialog dialog = new OpenFileDialog())
{
if (dialog.ShowDialog() == DialogResult.OK)
base.Text = dialog.FileName.ToString();
}
}
else if (_selected == SelectedPathType.folderDialog)
{
using ( FolderBrowserDialog dialog = new FolderBrowserDialog())
{ if (dialog.ShowDialog() == DialogResult.OK)
base.Text = dialog.SelectedPath;
}
}
else if (_selected == SelectedPathType.colorDialog)
{
using(ColorDialog dialog = new ColorDialog())
{ if (dialog.ShowDialog() == DialogResult.OK)
base.Text = dialog.Color.ToString() + ":"
+ dialog.Color.Name.ToString()
+ ": A=" + dialog.Color.A + " R=" + dialog.Color.R + " G=" + dialog.Color.G + " B=" + dialog.Color.B;
}
}
else if (_selected == SelectedPathType.fontDialog)
{
using( FontDialog dialog = new FontDialog())
{ if (dialog.ShowDialog() == DialogResult.OK)
base.Text = dialog.Font.ToString();
}
}
else if (_selected == SelectedPathType.OpenFileDialog)
{
using( SaveFileDialog dialog = new SaveFileDialog())
{ if (dialog.ShowDialog() == DialogResult.OK)
base.Text = dialog.FileName;
}
}
ButtonCellClick(sender, e);
}
public TextboxDialog()
{
browseButton = new Button();
browseButton.Text = "...";
browseButton.SizeChanged += (o, e) => { OnResize(e); };
browseButton.Click += new EventHandler(this.browseButton_Click);
browseButton.Size = new Size(28, this.ClientSize.Height + 2);
browseButton.Location = new Point(this.ClientSize.Width - browseButton.Width, -1);
this.Controls.Add(browseButton);
}
}
}
Code (C#)
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e)
{
textboxDialog1.ButtonCellClick += new EventHandler(this.ButtonCellClick);
}
private void ButtonCellClick(object sender, EventArgs e)
{
MessageBox.Show("Test 5555");
}
}
ต้องไปเพิ่มใน โค๊ด เหมือนกัน รบกวนท่านผู้รู้มั่งครับ
ทำยังไง Event ถึงใช้งานในหน้า design ได้เหมือน Event อื่นๆครับ
_/\_
|
 |
 |
 |
 |
Date :
2016-10-18 11:32:58 |
By :
lamaka.tor |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
อยากให้ เลาเลือก OpenFileDialog, SaveFileDialog
ให้เพิ่มอีก property ขึ้นมาคือ path folder ครับ
ผมอยากผูก column ไว้เลย
เวลาคลิกที่ปุ่มจะให้เอาไฟล์ไปเก็บใน path folder นั้นๆ ได้เลย
ใน datagrid ก็จะโชว์แค่ ชื่อไฟล์
เวลาคลิกที่ cell ก็จะรวม path folder+ "\\" ชื่อไฟล์ ประมาณนี้ครับ
ถ้าเลือกตัวอื่นๆเช่น colorDialog, fontDialog property path folder ก็จะหายไป แบบนี้อ่าครับ
ต้องทำยังไงรึ
|
 |
 |
 |
 |
Date :
2016-10-20 11:31:01 |
By :
lamaka.tor |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
  
|
 |
 |
 |
 |
Date :
2016-10-28 09:03:23 |
By :
lamaka.tor |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
จาก #NO 22 ปัจจุบันนี้หายากมากฯ ที่ตนฯหนึ่งจะเป็น
--- โปรแกรมเมอร์จริงฯ และเป็น SA ในคนฯเดียวกัน (รอบรู้ทุกอย่าง)
ส่วนใหญ่ก็ของปลอมทั้งนั้น อาศัยคำว่า "ทำงานเป็นทีม" เพื่อหากิน
|
 |
 |
 |
 |
Date :
2016-10-28 14:45:19 |
By :
หน้าฮี |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ผมมาบ่นเล่าให้ฟัง
ผมจุดธูป ไหว้ และขอพรสิ่งศักดิ์สิทธิ์ (แอบขอและแอบคิดอยู่ในใจกับเทวดา)
อันนี้ผมกำลังพูดถึง Windows Applications และผมจะเอาไปใช้กับ DataGridView
กรองข้อมูลใน DataGridView โดยใช้ Column ComboBox
(Cascading Comboboxes in DataGridView Windows Application)
ตอนนี้ผมต้องการแค่ ประเทศ ---> จังหวัด --> อำเภอ
Code (VB.NET)
Private Sub Form1_Load(sender As Object, e As EventArgs) Handles MyBase.Load
Dim ประเทศ As New Country() With {.CountryCode = "TH",
.CountryName = "ประเทศไทย",
.Provinces = New List(Of Province)() From {New Province() With {.ProvinceCode = "01",
.ProvinceName = "กรุงเทพฯ",
.Amphoes = New List(Of Amphoe)() From {New Amphoe() With {.AmphoeCode = "11",
.AmphoeName = "เขตพระโขนง"
},
New Amphoe() With {.AmphoeCode = "77",
.AmphoeName = "เขตบางหำ"
}
}
}
}
}
End Sub
Code (VB.NET)
'Model ประเทส
Public Class Country
Public Property CountryCode As String = "TH"
Public Property CountryName As String = "ประเทศไทย"
Public Property Provinces As List(Of Province) = Nothing
End Class
'Model จังหวัด
Code (VB.NET)
[vb]Public Class Province
Public Property ProvinceCode As String = String.Empty
Public Property ProvinceName As String = String.Empty
Public Property Amphoes As List(Of Amphoe) = Nothing
End Class
'Model อำเภอ
Code (VB.NET)
Public Class Amphoe
Public Property AmphoeCode As String = String.Empty
Public Property AmphoeName As String = String.Empty
End Class
|
 |
 |
 |
 |
Date :
2016-10-29 22:34:37 |
By :
หน้าฮี |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
จาก #NO 24 จริงฯแล้วผมเขียน C#.NET ได้คล่องแคล่วกว่า VB.NET
(ผมดักเด็กฯ ผู้เชี่ยวชาญ C# [พวกละเมอในภาษา]) +55555
ผมมักเลือกใช้ VB.NET ในงานหลักฯของผมเสมอ
|
 |
 |
 |
 |
Date :
2016-10-29 22:49:07 |
By :
หน้าฮี |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
@lamaka.tor
และคนอื่นฯ พวกคุณคิดว่าผมรู้จักคำว่า MVC หรือไม่ล่ะ?
+55555
|
 |
 |
 |
 |
Date :
2016-10-29 22:55:10 |
By :
หน้าฮี |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ช่วงนี้รันเครื่องครับ
น้ำใต้ดิน 300 เครื่องสำอางค์ 60
งอมจนไม่มีตรวจโค้ด DatePicker ที่ให้มาเลยครับ
เด๋วอาทิตย์หน้าก็โดน Audit อีก กรรม แบบบ้านๆ ครับ
ว่าแต่ ยากเอาการนะครับ 55555
|
 |
 |
 |
 |
Date :
2016-10-30 10:52:19 |
By :
lamaka.tor |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
@ ALL
The life must be learning all time and not finish.
We never know that what will we met when we will have learning.
Even though, we perhaps meet good and bad person in the same time.
Maybe this situation will be awaked us for continued learning by never ending.
ปล.
someone love one.
someone love two.
but I love one.
that one is ...
|
 |
 |
 |
 |
Date :
2016-10-31 14:41:27 |
By :
หน้าฮี |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
@lamaka.tor, QC_C0M
ผมเห็นว่าพวกคุณมีความถนัด DataGridView (Windows Application)
--- สมมุติว่าอยู่ในห้วงความต้องการ ลึกสุดฯ "เอาอยู่หรือไม่?"
--- จากรูปภาพด้านล่าง (ถ้าพอมีเวลาว่าง (ไม่ว่างก็ไม่เป็นไร) ลองเดาความต้องการของผมเล่นฯดู)
จากรูปภาพด้านล่าง มีสิทธิ์ไม่เกิน 3 ระเบียน แล้ววิธีการคิดมันต้องอธิบายออกมาอย่างไร?
--- อันนี้บนเงื่อนไขที่ไม่ซับซ้อน (ของจริงโหดร้ายยิ่งกว่านี้)
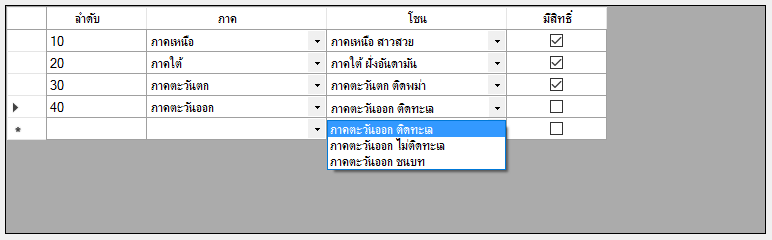
งานโปรแกรมมิ่ง ผมพูดได้ว่า "เด็กฯ"
--- บางคนเขียนโปรแกรมเก่งแต่เขียนเอกสารไม่เป็น (ไม่เป็นเลย) อันนี้แย่มากฯ
--- บางคนเขียนโปรแกรมไม่เก่งแต่เขียนเอกสารเป็น (พอใช้ได้) อันนี้พอใช้ได้
--- บางคนก็กินบุญเก่า (อันนี้แย่มากฯฯฯฯ)
ปล. จากรูปภาพด้านบน จริงฯแล้วผมยังมีคำถามกับตัวเองอีกมากมาย? (ผมไม่เคยง้อใครเหมือนกัน)
|
 |
 |
 |
 |
Date :
2016-11-03 15:55:03 |
By :
หน้าฮี |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
จาก #NO 29 อันนี้เป็น Source Code ที่กรองจำนวนผู้มีสิทธิ์
(ในเบื้องต้น เพื่อประกอบความเข้าใจ)
Code (VB.NET)
Private Function CalculateRight() As Short
Dim row = From r As DataGridViewRow In DataGridView1.Rows
Where (Not r.IsNewRow And r.Cells(3).Value IsNot DBNull.Value) AndAlso r.Cells(3).Value = 1S
Return If(row Is Nothing, 0, row.Count)
End Function
ปล. จะเรียก Function นี้ที่ไหนก็ตามสะดวก? (Events ใน Windows Application DataGridView มันพันกันอิรุงตุงนัง)
(ถ้าเป็นบนเวป หลับตาทำก็ยังได้เลย)
|
 |
 |
 |
 |
Date :
2016-11-03 16:05:14 |
By :
หน้าฮี |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
จาก #NO 29 - 30 เดี๋ยวจะหาว่า เขียนเวปไซต์ไม่เป็น +55555
อันนี้เล่นบน Tag HTML <table></table>
ถ้าจำไม่ผิดคือระบบ IC และไม่มี ViewState ปะปนด้วย
Code (VB.NET)
Private Function listCopy() As List(Of WL_Model.ICD)
Return (From r In rtABC.Items.Cast(Of RepeaterItem)() Select New WL_Model.ICD() With {.Part_NO = DirectCast(r.FindControl("txtPart_NO"), WL_Controls.WL_TextBox).Text,
.xTitleTH = DirectCast(r.FindControl("lblPart_Desc"), WL_Controls.WL_TextBox).Text,
.Tran_Qty = DirectCast(r.FindControl("txtTran_Qty"), WL_Controls.WL_TextBox).Text,
.UM = DirectCast(r.FindControl("cboUM"), HtmlSelect).Value,
.From_Bal = DirectCast(r.FindControl("cboBalType"), HtmlSelect).Value,
.Tran_Amt = DirectCast(r.FindControl("txtTran_Amt"), WL_Controls.WL_TextBox).Text,
.xID = CInt(DirectCast(r.FindControl("cbRemoveAttachment"), CheckBox).Checked)
}).ToList()
End Function
|
 |
 |
 |
 |
Date :
2016-11-03 16:26:03 |
By :
หน้าฮี |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
จาก #NO 29 -31 อันนี้ผมพูดติดตลก
--- ให้โปรแกรมเมอร์เด็กฯ 10 - 20 หรือ 100 คน เขียน ให้เวลาไปเลย 10 ปี
--- เขียนเสร็จหรือเปล่า? +55555
ปล. เก่งอยู่อย่างเดียวนั่นคือ "คำว่าขี้เกียจ"
|
 |
 |
 |
 |
Date :
2016-11-03 16:33:04 |
By :
หน้าฮี |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
พักซักหน่อยก่อนครับ
ช่วงนี้หัดเขียน Autocad ต่อ
หัดทำนาฬิกาไม้ครับ
https://www.youtube.com/watch?v=tAKCV5RBnFQ
จนถึงทุกวันนี้ยังไม่เข้าใจว่าตกลง ผมเป็นนักวิทย์ จริงๆ หรือ เขาจ้างมาให้เป็นช่างก็ไม่รู้
ซ่อมดะไปเรื่อย 5555
|
 |
 |
 |
 |
Date :
2016-11-04 11:13:42 |
By :
lamaka.tor |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ต้องเร่งทำ Uncer,Calibration,Validate กะ PT ให้เสร็จก่อน 20
เลยไม่ค่อยมีเวลาเขียนโปรแกรมต่อซักที
เย็นๆหน่อยก็เหล้าขาวอีก ไม่ว๊างไม่ว่าง ตามประสาคนบ้านๆ ครับ
|
 |
 |
 |
 |
Date :
2016-11-04 11:20:17 |
By :
lamaka.tor |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
GUI แบบข้างบนสุดของโพสต์นี้คุณทำอย่างไรอ่ะครับ
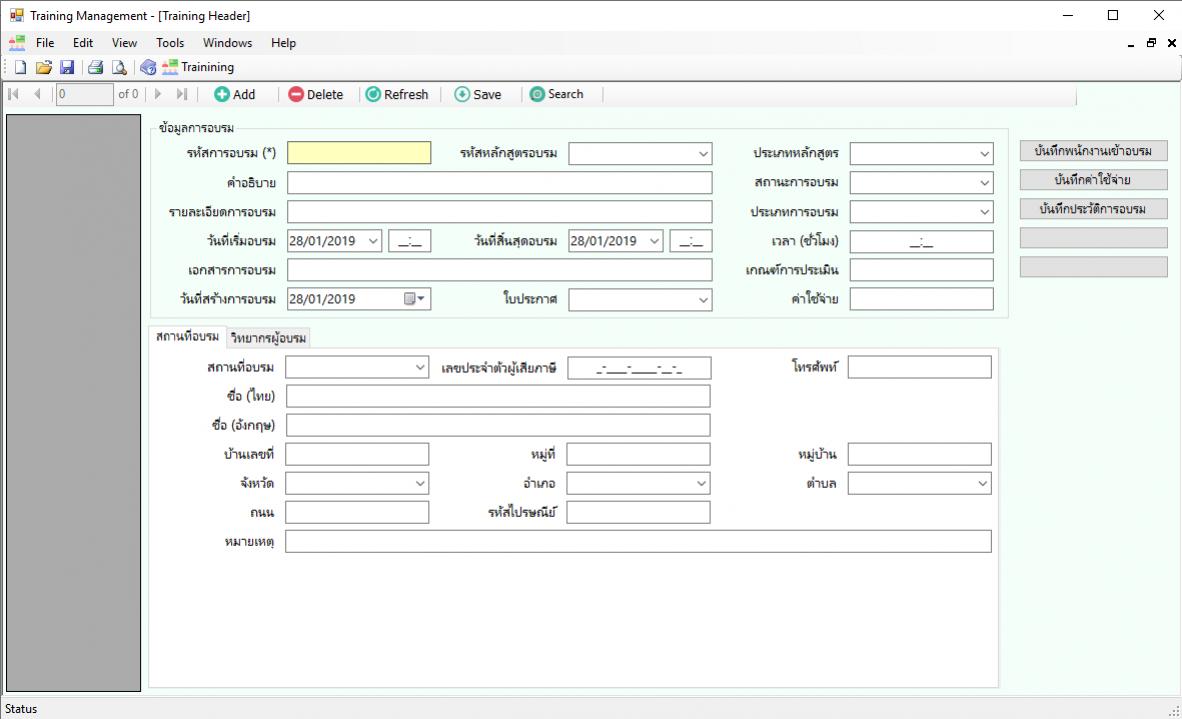
ผมอยากทำคล้ายๆ แบบคุณ อยากขอไอเดียหน่อยครับ
|
ประวัติการแก้ไข 2019-05-13 11:35:05
 |
 |
 |
 |
Date :
2019-05-13 11:30:47 |
By :
jaypang |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
|
|