 |
|
C# WinApp ขอแนวทางในการ DragDrop ใน DataGridView หน่อยครับ |
|
 |
|
|
 |
 |
|
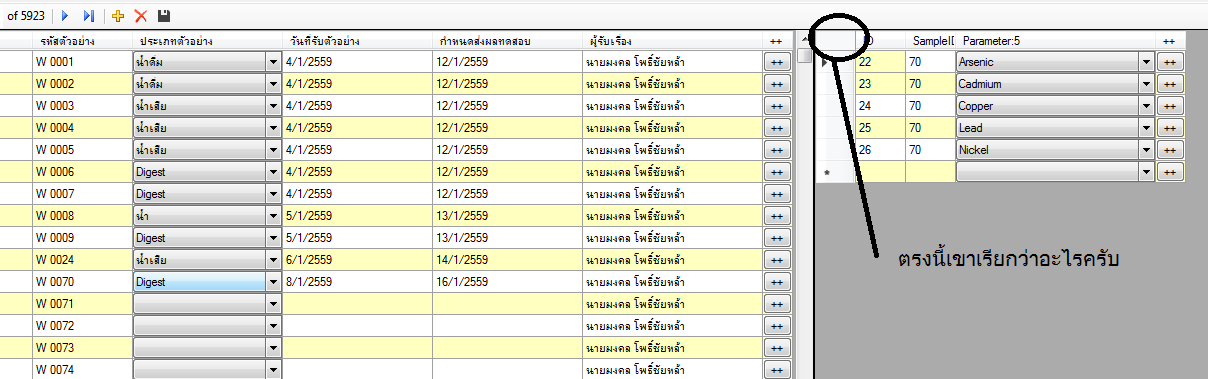
คือผมจะใส่ Event click ครับ
แต่ไม่รู้เขาเรียกว่าอะไร
RowHeaderMouseClick กับ ColumnHeaderMouseClick ก็ไม่ได้ครับ
|
 |
 |
 |
 |
Date :
2016-12-15 15:51:31 |
By :
lamaka.tor |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ติดปัญหาเรื่อง DragEventArgs to RowIndex ครับ(นึกว่าจะฉลุยแล้วซะอีก  )
คืออยากทราบ RowIndex ของตาราง sample ครับ
ว่าจะเพิ่มให้ sampleID ตัวไหน
Code (C#)
private void dataGridView2_DragDrop(object sender, DragEventArgs e)
{
DataGridViewSelectedRowCollection rows = (DataGridViewSelectedRowCollection)e.Data.GetData(typeof(DataGridViewSelectedRowCollection));
rowIndexFromMouseDown = dataGridView2.HitTest(e.X, e.Y).RowIndex;
MessageBox.Show(e.X + " "+ e.Y+ " " + rowIndexFromMouseDown);
for (int i = rows.Count-1; i >=0 ; i--)
{
if (!string.IsNullOrEmpty("" + rows[i].Cells[0].Value))
dataGridView2.Rows.Add(rows[i].Cells[0].Value, rows[i].Cells[1].Value, rows[i].Cells[2].Value);
}
/* foreach (DataGridViewRow row in rows)
{
if (!string.IsNullOrEmpty("" + row.Cells[0].Value))
dataGridView2.Rows.Add(row.Cells[0].Value, row.Cells[1].Value, row.Cells[2].Value);
//dataGridView1.Rows.Remove(row);
}*/
}
|
ประวัติการแก้ไข 2016-12-15 23:17:46
 |
 |
 |
 |
Date :
2016-12-15 22:44:22 |
By :
lamaka.tor |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
สงสัยวันนี้ท่าทางจะบ้า
ถามเองแล้วก็ตอบเอง
สรุป ได้แล้วนะครับ
//RowIndex
Code (C#)
public static int DragDropRowIndex(this System.Windows.Forms.DataGridView dgv, System.Windows.Forms.DragEventArgs e)
{
int r = 0;
System.Drawing.Point p = dgv.PointToClient(new System.Drawing.Point(e.X, e.Y));
r = dgv.HitTest(p.X, p.Y).RowIndex;
return r;
}
โค้ดบ้านๆ ประมาณนี้ครับครับ
Code (C#)
static class Program
{
/// <summary>
/// The main entry point for the application.
/// </summary>
[STAThread]
static void Main()
{
Application.EnableVisualStyles();
Application.SetCompatibleTextRenderingDefault(false);
Application.Run(new frmDragNDrop_2() );
}
public static int DragDropRowIndex(this System.Windows.Forms.DataGridView dgv, System.Windows.Forms.DragEventArgs e)
{
int r = 0;
System.Drawing.Point p = dgv.PointToClient(new System.Drawing.Point(e.X, e.Y));
r = dgv.HitTest(p.X, p.Y).RowIndex;
return r;
}
}
Code (C#)
public partial class frmDragNDrop_2 : Form
{
public frmDragNDrop_2()
{
InitializeComponent();
this.dataGridView1.AllowDrop = true;
this.dataGridView2.AllowDrop = true;
}
private int rowIndexFromMouseDown;
private void frmDragNDrop_2_Load(object sender, EventArgs e)
{
for (int i = 0; i < 10; i++)
dataGridView1.Rows.Add("Test " + i, "Tor " + i);
}
private void dataGridView1_MouseDown(object sender, MouseEventArgs e)
{
dataGridView1.DoDragDrop(dataGridView1.SelectedRows, DragDropEffects.Copy);
}
private void listBox2_DragDrop(object sender, System.Windows.Forms.DragEventArgs e)
{
DataGridViewSelectedRowCollection rows = (DataGridViewSelectedRowCollection)e.Data.GetData(typeof(DataGridViewSelectedRowCollection));
foreach (DataGridViewRow row in rows)
{
try
{
listBox2.Items.Add(row.Cells[1].Value);
}
catch { }
}
}
private void listBox2_DragEnter(object sender, DragEventArgs e)
{
if (e.Data.GetDataPresent(typeof(DataGridViewSelectedRowCollection)))
e.Effect = DragDropEffects.Copy;
}
private void dataGridView2_DragDrop(object sender, DragEventArgs e)
{
DataGridViewSelectedRowCollection rows = (DataGridViewSelectedRowCollection)e.Data.GetData(typeof(DataGridViewSelectedRowCollection));
rowIndexFromMouseDown = dataGridView2.DragDropRowIndex(e);
for (int i = rows.Count-1; i >=0 ; i--)
{
if (!string.IsNullOrEmpty("" + rows[i].Cells[0].Value))
dataGridView2.Rows.Add(rows[i].Cells[0].Value, rows[i].Cells[1].Value, rows[i].Cells[2].Value);
}
}
private void dataGridView2_DragEnter(object sender, DragEventArgs e)
{
Text = "dataGridView2_DragEnter";
if (e.Data.GetDataPresent(typeof(DataGridViewSelectedRowCollection))) e.Effect = DragDropEffects.Copy;
}
private void dataGridView2_DragOver(object sender, DragEventArgs e)
{
Text = "dataGridView2_DragOver";
if (e.Data.GetDataPresent(typeof(DataGridViewSelectedRowCollection))) e.Effect = DragDropEffects.Copy;
}
}
ต่อไปคือ
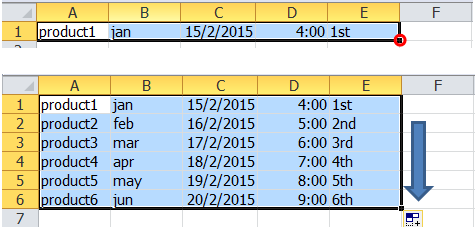
คลิก ลาก เพื่อเพิ่ม rows แบบ excel ครับ
ประมาณว่า W 0001 เป็นน้ำดื่ม รายการวิเคราะห์ Cadmium Iron Lead
W 0002 เป็นน้ำดื่ม รายการวิเคราะห์ Cadmium Iron Lead
อยากจะให้ User กรอกข้อมูล W 0001 แล้วก็ลากลงมา เป็น W 0002 ได้เลยครับ
ยังหาวิธีใช้เม้ายังไม่ได้ครับ
|
 |
 |
 |
 |
Date :
2016-12-15 23:39:36 |
By :
lamaka.tor |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
เดี๋ยวซักหน่อยจะมีคนเข้ามาตอบ เห็นเล่นโพสคุณตลอด 
|
 |
 |
 |
 |
Date :
2016-12-16 00:08:54 |
By :
Luz |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
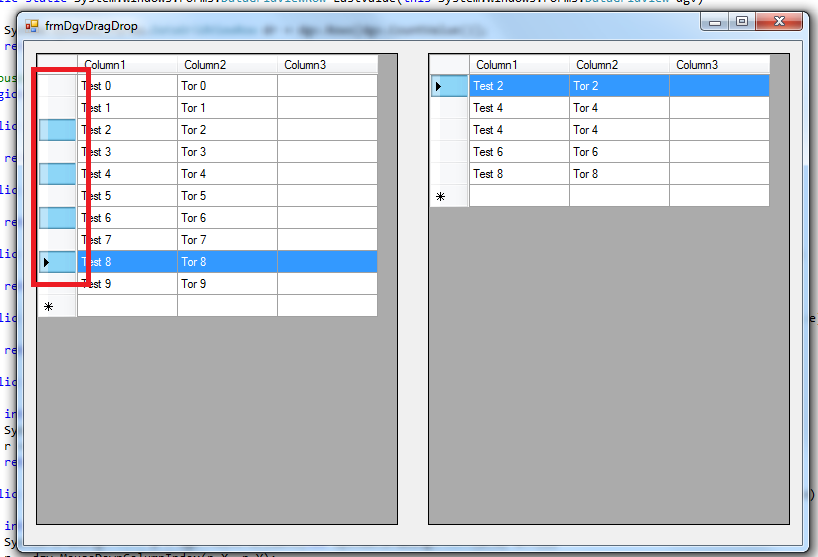
สงสัยทำไมพอลากไปแล้ว dataGridView2 ก็เพิ่มข้อมูลไปแล้ว
แต่ทำไม dataGridView1 ยังมีร่องรอยอารยธรรม การ คลิกเลือกอยู่ละครับ
ต้อง เอามันออกตรงไหนครับ
Code (C#)
public partial class frmDgvDragDrop : Form
{
public frmDgvDragDrop()
{
InitializeComponent();
this.dataGridView1.AllowDrop = true;
this.dataGridView2.AllowDrop = true;
for (int i = 0; i < 10; i++)
dataGridView1.Rows.Add("Test " + i, "Tor " + i);
dataGridView1.MouseDown+=dataGridView1_MouseDown;
dataGridView2.DragDrop += dataGridView2_DragDrop;
dataGridView2.DragEnter+=dataGridView2_DragEnter;
}
private int rowIndexFromMouseDown;
private void frmDgvDragDrop_Load(object sender, EventArgs e)
{
}
private void dataGridView1_MouseDown(object sender, MouseEventArgs e)
{
dataGridView1.DoDragDrop(dataGridView1.SelectedRows, DragDropEffects.Copy);
}
private void dataGridView2_DragDrop(object sender, DragEventArgs e)
{
DataGridViewSelectedRowCollection rows = (DataGridViewSelectedRowCollection)e.Data.GetData(typeof(DataGridViewSelectedRowCollection));
rowIndexFromMouseDown = dataGridView2.MouseDownRowIndex(e);
MessageBox.Show("" + rowIndexFromMouseDown);
for (int i = rows.Count - 1; i >= 0; i--)
{
if (!string.IsNullOrEmpty("" + rows[i].Cells[0].Value))
dataGridView2.Rows.Add(rows[i].Cells[0].Value, rows[i].Cells[1].Value, rows[i].Cells[2].Value);
}
e = null;
}
private void dataGridView2_DragEnter(object sender, DragEventArgs e)
{
if (e.Data.GetDataPresent(typeof(DataGridViewSelectedRowCollection))) e.Effect = DragDropEffects.Copy;
}
}
|
 |
 |
 |
 |
Date :
2016-12-16 11:46:35 |
By :
lamaka.tor |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ขยันมาก ๆ ครับ
นับถือเลย
ตัวด้านหน้าของแถว (row header) ปิดทิ้งไหมครับ
อาจจะสวยขึ้น
|
 |
 |
 |
 |
Date :
2016-12-16 13:16:08 |
By :
fonfire |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
.RowHeadersVisible = false
|
 |
 |
 |
 |
Date :
2016-12-16 13:30:20 |
By :
fonfire |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
|
|