 |
|
WinApp C# เขียนโค้ด ButtonCollections แล้ว แต่อยากเลือกแก้ไข ปุ่มทีละปุ่มได้ เหมือนปุ่มปกติครับ |
|
 |
|
|
 |
 |
|
โค้ดครับ
Code (C#)
public class ButtonCollections : UserControl
{
public ButtonCollections()
{
RefreshGrid();
}
int sizeX = 10; int sizeY = 10;
int rowCount = 2;
int ColumnCount = 2;
Size _ButtonSize = new Size(300, 140);
Color _ButtonColor = System.Drawing.Color.FromArgb(((int)(((byte)(255)))), ((int)(((byte)(224)))), ((int)(((byte)(192)))));
Color _ButtonForeColor = System.Drawing.Color.Blue;
public void RefreshGrid()
{
if (rowCount <= 0 || ColumnCount <= 0) return;
this.Controls.Clear();
int X = 20, Y = 20;
int row = 0, column = 0;
for (int i = 1; i <= rowCount * ColumnCount; i++)
{
Button btn = new Button();
btn.BackColor = _ButtonColor;
btn.Font = this.Font;
btn.ForeColor = _ButtonForeColor;
btn.Location = new System.Drawing.Point(X, Y);
btn.Name = "button" + column + "_" + row;
btn.Size = _ButtonSize;
btn.Text = btn.Name;
btn.UseVisualStyleBackColor = false;
btn.Click += new EventHandler(buttonChoie_Click);
X += _ButtonSize.Width + sizeX;
this.Controls.Add(btn);
column++;
if (i % ColumnCount == 0)
{
Y += _ButtonSize.Height + sizeY; X = 20;
column = 0;
row++;
}
}
}
private void buttonChoie_Click(object sender, EventArgs e)
{
ButtonSelect = sender as Button;
}
#region _Property
[System.ComponentModel.Browsable(true)]
[System.ComponentModel.EditorBrowsable(System.ComponentModel.EditorBrowsableState.Always)]
[System.ComponentModel.Category("TOR Setting")]
public Font ButtonFont
{
get { return this.Font; }
set
{
this.Font = value;
RefreshGrid();
}
}
[System.ComponentModel.Browsable(true)]
[System.ComponentModel.EditorBrowsable(System.ComponentModel.EditorBrowsableState.Always)]
[System.ComponentModel.Category("TOR Setting")]
public Color ButtonColor
{
get { return _ButtonColor; }
set
{
_ButtonColor = value;
RefreshGrid();
}
}
[System.ComponentModel.Browsable(true)]
[System.ComponentModel.EditorBrowsable(System.ComponentModel.EditorBrowsableState.Always)]
[System.ComponentModel.Category("TOR Setting")]
public Color ButtonForeColor
{
get { return _ButtonForeColor; }
set
{
_ButtonForeColor = value;
RefreshGrid();
}
}
[System.ComponentModel.Browsable(true)]
[System.ComponentModel.EditorBrowsable(System.ComponentModel.EditorBrowsableState.Always)]
[System.ComponentModel.DefaultValue(2)]
[System.ComponentModel.Category("TOR Setting")]
public int rowCounts
{
get { return rowCount; }
set
{
rowCount = value;
RefreshGrid();
}
}
[System.ComponentModel.Browsable(true)]
[System.ComponentModel.EditorBrowsable(System.ComponentModel.EditorBrowsableState.Always)]
[System.ComponentModel.Category("TOR Setting")]
public Size ButtonSize
{
get { return _ButtonSize; }
set
{
_ButtonSize = value;
RefreshGrid();
}
}
[System.ComponentModel.Browsable(true)]
[System.ComponentModel.EditorBrowsable(System.ComponentModel.EditorBrowsableState.Always)]
[System.ComponentModel.DefaultValue(2)]
[System.ComponentModel.Category("TOR Setting")]
public int ColumnCounts
{
get { return ColumnCount; }
set
{
ColumnCount = value;
RefreshGrid();
}
}
public Button Item(int row,int column)
{
return this.Controls.Find("button" + column + "_" + row,true).FirstOrDefault() as Button;
}
public virtual void Clear()
{
this.Controls.Clear();
}
#endregion
public Button ButtonSelect;
public event EventHandler ButtonClick
{
add
{
for (int r = 0; r < rowCount; r++)
{
for (int c = 0; c < ColumnCount; c++)
{
if (value != null && Item(r, c) != null)
Item(r, c).Click += value;
}
}
}
remove
{
for (int r = 0; r < rowCount; r++)
{
for (int c = 0; c < ColumnCount; c++)
{
if (value != null && Item(r, c) != null)
Item(r, c).Click -= value;
}
}
}
}
}
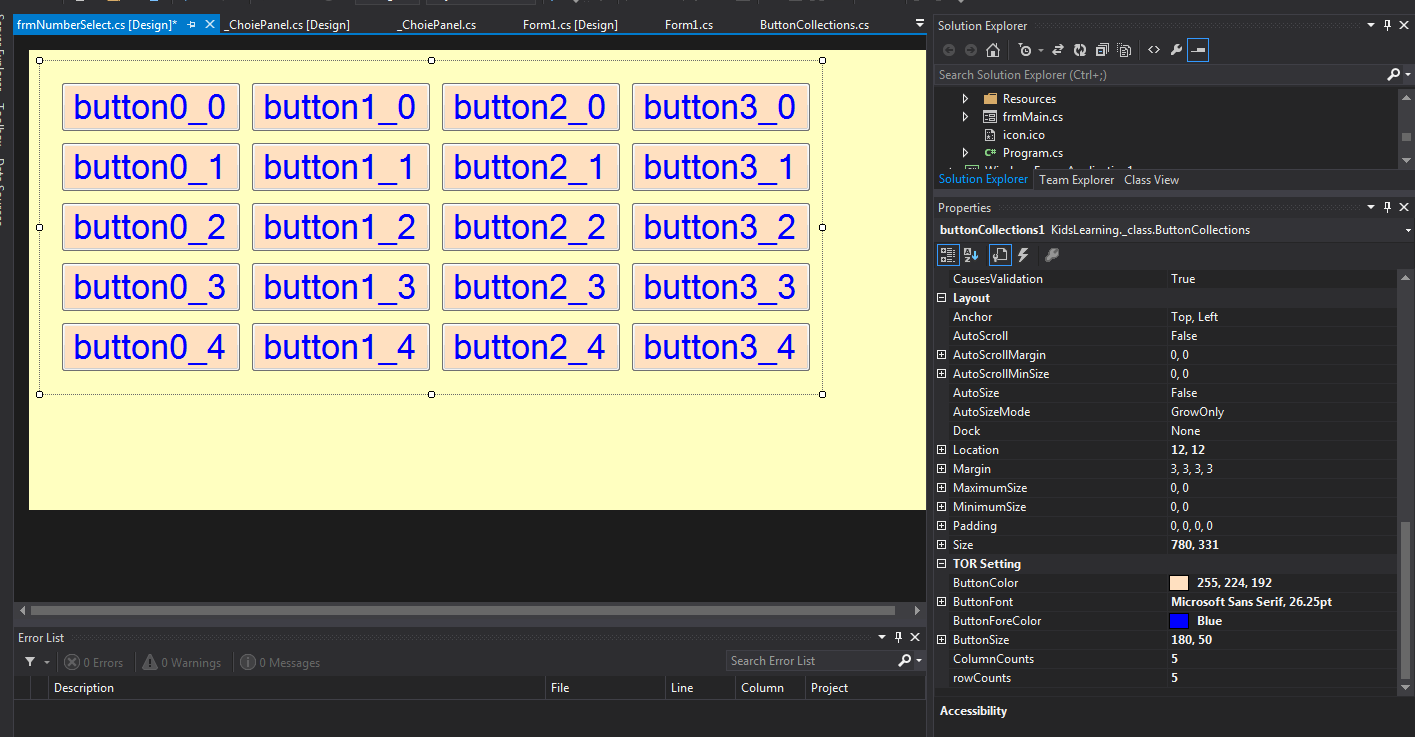
คืออยากจะแก้ไขทีละปุ่มได้ ตรงหน้า Design เหมือนกับที่เราแก้ใน DataGridView อ่าครับ
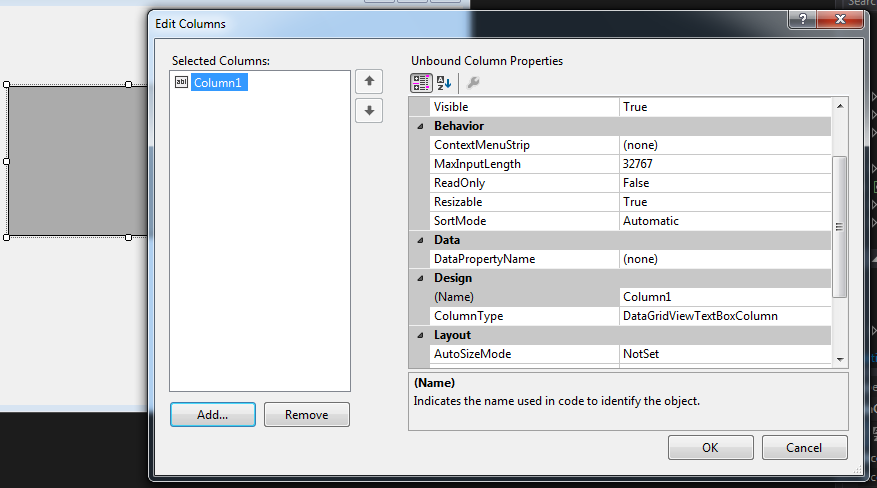
ปล.อยากทำแบบ OutoCopy ด้วยครับ
อย่างเช่น ใส่ test แล้วให้ รัน test_1,test_2,test_3...... จนกว่าจะครบ
Tag : .NET, C#, VS 2013 (.NET 4.x)
|
ประวัติการแก้ไข 2018-08-03 10:46:52
|
 |
 |
 |
 |
Date :
2018-08-03 10:41:03 |
By :
lamaka.tor |
View :
967 |
Reply :
3 |
|
 |
 |
 |
 |
|
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
แต่อยากเลือกแก้ไข ปุ่มทีละปุ่มได้ เหมือนปุ่มปกติครับ
ต้องการแก้ไข property ของปุ่ม หรือแก้ไข ข้อมูลที่ปุ่มอ้างอิงถีง ครับ
ถ้าต้องการเซท property แบบนี้น่าจะได้
Code (C#)
public Font getButtonFont(int idx)
{
return (this.Controls[idx] as Button).Font;
}
public void setButtonFont(int idx, Font value)
{
(this.Controls[idx] as Button).Font = value;
}
แต่ถ้าต้องการ แก้ไขข้อมูล ลองใช้ property tag เก็บข้อมูลของ object ที่ต้องการแก้ไขครับ
tag property เป็น ตัวแปรชนิด object เอาไว้ อ้างอิง อะไรต่างๆ ได้เลยครับ
จะเป็น reference หรือ constant ก็ได้ทั้งนั้นครับ
ปล. ผิดวัตถุประสงค์ ต้องการแก้ไข หน้า design time
|
ประวัติการแก้ไข 2018-08-03 14:15:22
 |
 |
 |
 |
Date :
2018-08-03 14:11:56 |
By :
Chaidhanan |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
น่าจะลองแกะ ของ tab panel นะครับ เพราะมันมีการ add control ให้เห็นอย่างชัดเจน
เราสามารถ ลาก object ต่างๆ ลงไปและแก้ไข property ต่างๆ ได้
|
 |
 |
 |
 |
Date :
2018-08-04 05:35:11 |
By :
Chaidhanan |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
|
|