 |
|
จากภาพปัญหาที่เกิดคือ 1.ผมทำ Search จาก Code 2.พอผมเอา Code มา Search ก็ได้ผล Search ได้,เจอ 3.แต่พอกด Select |
|
 |
|
|
 |
 |
|
จากภาพปัญหาที่เกิดคือ
1.ผมทำ Search จาก Code
2.พอผมเอา Code มา Search ก็ได้ผล Search ได้,เจอ
3.แต่พอกด Select แล้วดันเลิก ค่าอื่น เช่นตามภาม Select ค่า CaseKey 1 แต่ดัน ออก 2
ปล.พอไม่ Search แล้วคลิก Select เลย มันเลือก ตามค่าที่คลิกไม่มีปัญหา อยากถามผู้รู้ว่าเป็นเพราะอะครับ บัค หรอครับ
หน้าที่ส่งค่าไป
Code (C#)
using System;
using System.Collections.Generic;
using System.Data;
using System.Data.SqlClient;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
namespace Test
{
public partial class ShowIT : System.Web.UI.Page
{
string connectiondb = @"Data Source=ARTEMIS\SQL2014;Initial Catalog=SvlITDB;User ID=it;Password=Tigers1234;";
protected void Page_Load(object sender, EventArgs e)
{
using (SqlConnection sqlcon = new SqlConnection(connectiondb))
{
sqlcon.Open();
SqlDataAdapter sqlda = new SqlDataAdapter("SELECT c.CaseKey, c.CaseCode, ct.Name, c.CaseName, c.CaseDetail, c.CaseDT, c.Requester, u.DeptName, u.Tel, cs.Name AS Expr1, ic.StaffName, ic.Solve, ic.Dates, ic.ExpectedDT FROM [Case] AS c LEFT OUTER JOIN CaseType AS ct ON c.CaseTypeKey = ct.CaseTypeKey LEFT JOIN Usr AS u ON c.UsrKey = u.UsrKey LEFT JOIN CaseStatus AS cs ON c.CaseStatusKey = cs.CaseStatusKey LEFT OUTER JOIN ItCase AS ic ON c.CaseKey = ic.CaseKey ORDER BY c.CaseKey DESC", sqlcon);
DataTable aaa = new DataTable();
sqlda.Fill(aaa);
GridView1.DataSource = aaa;
GridView1.DataBind();
}
}
protected void Button1_Click(object sender, EventArgs e)
{
if (DropDownList1.Text == "1") {
using (SqlConnection sqlcon = new SqlConnection(connectiondb))
{
sqlcon.Open();
SqlDataAdapter sqlda = new SqlDataAdapter("SELECT c.CaseKey, c.CaseCode, ct.Name, c.CaseName, c.CaseDetail, c.CaseDT, c.Requester, u.DeptName, u.Tel, cs.Name AS Expr1, ic.StaffName, ic.Solve, ic.Dates, ic.ExpectedDT FROM [Case] AS c LEFT OUTER JOIN CaseType AS ct ON c.CaseTypeKey = ct.CaseTypeKey LEFT JOIN Usr AS u ON c.UsrKey = u.UsrKey LEFT JOIN CaseStatus AS cs ON c.CaseStatusKey = cs.CaseStatusKey LEFT OUTER JOIN ItCase AS ic ON c.CaseKey = ic.CaseKey WHERE cs.Name = 'Process' ORDER BY c.CaseKey DESC", sqlcon);
DataTable aaa = new DataTable();
sqlda.Fill(aaa);
GridView1.DataSource = aaa;
GridView1.DataBind();
}
}
if (DropDownList1.Text=="2") {
using (SqlConnection sqlcon = new SqlConnection(connectiondb))
{
sqlcon.Open();
SqlDataAdapter sqlda = new SqlDataAdapter("SELECT c.CaseKey, c.CaseCode, ct.Name, c.CaseName, c.CaseDetail, c.CaseDT, c.Requester, u.DeptName, u.Tel, cs.Name AS Expr1, ic.StaffName, ic.Solve, ic.Dates, ic.ExpectedDT FROM [Case] AS c LEFT OUTER JOIN CaseType AS ct ON c.CaseTypeKey = ct.CaseTypeKey LEFT JOIN Usr AS u ON c.UsrKey = u.UsrKey LEFT JOIN CaseStatus AS cs ON c.CaseStatusKey = cs.CaseStatusKey LEFT OUTER JOIN ItCase AS ic ON c.CaseKey = ic.CaseKey WHERE cs.Name = 'Success' ORDER BY c.CaseKey DESC", sqlcon);
DataTable aaa = new DataTable();
sqlda.Fill(aaa);
GridView1.DataSource = aaa;
GridView1.DataBind();
}
}
if (DropDownList1.Text == "3") {
using (SqlConnection sqlcon = new SqlConnection(connectiondb))
{
sqlcon.Open();
SqlDataAdapter sqlda = new SqlDataAdapter("SELECT c.CaseKey, c.CaseCode, ct.Name, c.CaseName, c.CaseDetail, c.CaseDT, c.Requester, u.DeptName, u.Tel, cs.Name AS Expr1, ic.StaffName, ic.Solve, ic.Dates, ic.ExpectedDT FROM [Case] AS c LEFT OUTER JOIN CaseType AS ct ON c.CaseTypeKey = ct.CaseTypeKey LEFT JOIN Usr AS u ON c.UsrKey = u.UsrKey LEFT JOIN CaseStatus AS cs ON c.CaseStatusKey = cs.CaseStatusKey LEFT OUTER JOIN ItCase AS ic ON c.CaseKey = ic.CaseKey ORDER BY c.CaseKey DESC", sqlcon);
DataTable aaa = new DataTable();
sqlda.Fill(aaa);
GridView1.DataSource = aaa;
GridView1.DataBind();
}
}
}
protected void Button2_Click(object sender, EventArgs e)
{
using (SqlConnection sqlcon = new SqlConnection(connectiondb))
{
sqlcon.Open();
SqlDataAdapter sqlda = new SqlDataAdapter("SELECT c.CaseKey, c.CaseCode, ct.Name, c.CaseName, c.CaseDetail, c.CaseDT, c.Requester, u.DeptName, u.Tel, cs.Name AS Expr1, ic.StaffName, ic.Solve, ic.Dates, ic.ExpectedDT FROM [Case] AS c LEFT OUTER JOIN CaseType AS ct ON c.CaseTypeKey = ct.CaseTypeKey LEFT JOIN Usr AS u ON c.UsrKey = u.UsrKey LEFT JOIN CaseStatus AS cs ON c.CaseStatusKey = cs.CaseStatusKey LEFT OUTER JOIN ItCase AS ic ON c.CaseKey = ic.CaseKey Where c.CaseCode LIKE '%' +'"+TextBox1.Text.Trim()+"'+'%' ", sqlcon);
DataTable aaa = new DataTable();
sqlda.Fill(aaa);
GridView1.DataSource = aaa;
GridView1.DataBind();
}
}
protected void GridView1_SelectedIndexChanged(object sender, EventArgs e)
{
GridViewRow gr = GridView1.SelectedRow;
Response.Redirect("TestEdit.aspx?CaseKey=" + gr.Cells[1].Text + "&CaseCode=" + gr.Cells[2].Text + "&ct.Name=" + gr.Cells[3].Text + "&CaseName=" + gr.Cells[4].Text + "&CaseDetail=" + gr.Cells[5].Text + "&CaseDT=" + gr.Cells[6].Text + "&Requester=" + gr.Cells[7].Text + "&DeptName=" + gr.Cells[8] + "cs.Tel" + gr.Cells[9] + "cs.Name" + gr.Cells[10] + "StaffName" + gr.Cells[11] + "Solve" + gr.Cells[12] + "Dates" + gr.Cells[13] + "ExpectedDT" + gr.Cells[14]);
}
}
}
หน้าที่รับค่ามา
Code (C#)
using System;
using System.Collections.Generic;
using System.Data;
using System.Data.SqlClient;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
namespace Test
{
public partial class TestEdit : System.Web.UI.Page
{
string connectiondb = @"Data Source=ARTEMIS\SQL2014;Initial Catalog=SvlITDB;User ID=it;Password=Tigers1234;";
protected void Page_Load(object sender, EventArgs e)
{
DateTime daValues = DateTime.Now;
string MyDt = daValues.ToString("dd-MM-yyyy HH:mm");
TextBox3.Text = DateTime.Now.ToString(MyDt);
TextBox1.Text = Request.QueryString["CaseKey"];
TextBox2.Text = Request.QueryString["CaseCode"];
using (SqlConnection sqlcon = new SqlConnection(connectiondb))
{
sqlcon.Open();
SqlDataAdapter sqlda = new SqlDataAdapter("SELECT LFET.CaseKey, LFET.CaseCode, ItCase.StaffName, ItCase.Solve, ItCase.Dates, ItCase.ExpectedDT, ItCase.DateHope FROM [Case] AS LFET INNER JOIN ItCase ON LFET.CaseKey = ItCase.CaseKey Order By ItCase.CaseKey DESC", sqlcon);
DataTable aaa = new DataTable();
sqlda.Fill(aaa);
GridView1.DataSource = aaa;
GridView1.DataBind();
}
}
#region Button1_Click
protected void Button1_Click(object sender, EventArgs e)
{
if (DropDownList4.Text == "0")
{
ScriptManager.RegisterStartupScript(this.Page, this.GetType(), "Myscript", "alert('กรุณากรอกข้อมุลให้ครบ')", true);
}
else if (DropDownList1.Text == "กำลังดำเนินการ")
{
ScriptManager.RegisterStartupScript(this.Page, this.GetType(), "Myscript", "alert('กรุณากรอกข้อมุลให้ครบ')", true);
}
else if (DropDownList5.Text == "0")
{
ScriptManager.RegisterStartupScript(this.Page, this.GetType(), "Myscript", "alert('กรุณากรอกข้อมุลให้ครบ')", true);
}
else if (Request.Form["Message"] == null)
{
ScriptManager.RegisterStartupScript(this.Page, this.GetType(), "Myscript", "alert('กรุณากรอกข้อมุลให้ครบ')", true);
}
else
{
SqlConnection conn = new SqlConnection("Data Source=ARTEMIS\\SQL2014;Initial Catalog=SvlITDB;User ID=it;Password=Tigers1234");
conn.Open();
SqlCommand sqlcom = conn.CreateCommand();
SqlCommand sqlcom2 = conn.CreateCommand();
sqlcom.CommandText = "INSERT INTO [dbo].[ItCase] ([CaseKey], [Solve], [StaffName], [ExpectedDT], [CaseCode],[Dates],[DateHope]) VALUES ('" + TextBox1.Text + "','" + Request.Form["Message"].ToString() + "', '" + DropDownList1.Text + "', '"+TextBox8.Text+"','"+TextBox2.Text+"','"+TextBox3.Text+"','"+DropDownList5.Text+"')";
sqlcom2.CommandText = "Update [Case] set CaseStatusKey = '" + DropDownList4.Text + "' where CaseKey = '" + TextBox1.Text + "'";
//sqlcom.CommandText = "INSERT INTO[dbo].[CaseType] ( [CodeType], [NameType]) VALUES" + "('" + this.TextBox2.Text + "','" + TextBox3.Text + "')";
sqlcom.CommandType = CommandType.Text;
sqlcom2.CommandType = CommandType.Text;
sqlcom2.ExecuteNonQuery();
sqlcom.ExecuteNonQuery();
conn.Close();
string scriptText = "alert('แก้ไขการแจ้งปัญหาสำเร็จ'); window.location='" + Request.ApplicationPath + "TestEdit.aspx'";
ScriptManager.RegisterStartupScript(this, this.GetType(), "alertMessage", scriptText, true);
}
}
#endregion
protected void Button2_Click(object sender, EventArgs e)
{
if (DropDownList4.Text == "0")
{
ScriptManager.RegisterStartupScript(this.Page, this.GetType(), "Myscript", "alert('กรุณากรอกข้อมุลให้ครบ')", true);
}
else if (DropDownList1.Text == "กำลังดำเนินการ")
{
ScriptManager.RegisterStartupScript(this.Page, this.GetType(), "Myscript", "alert('กรุณากรอกข้อมุลให้ครบ')", true);
}
else if (DropDownList5.Text == "0")
{
ScriptManager.RegisterStartupScript(this.Page, this.GetType(), "Myscript", "alert('กรุณากรอกข้อมุลให้ครบ')", true);
}
else if (Request.Form["Message"] == null)
{
ScriptManager.RegisterStartupScript(this.Page, this.GetType(), "Myscript", "alert('กรุณากรอกข้อมุลให้ครบ')", true);
}
else if (TextBox1.Text != "")
{
SqlConnection conn = new SqlConnection("Data Source=ARTEMIS\\SQL2014;Initial Catalog=SvlITDB;User ID=it;Password=Tigers1234");
conn.Open();
SqlCommand sqlcom = conn.CreateCommand();
SqlCommand sqlcom2 = conn.CreateCommand();
sqlcom.CommandText = "Update [dbo].[ItCase] set Solve = '" + Request.Form["Message"] + "', StaffName = '" + DropDownList1.Text + "', ExpectedDT = '" + TextBox8.Text + "', DateHope = '" + DropDownList5.Text + "' WHERE CaseKey='" + TextBox1.Text + "'";
sqlcom2.CommandText = "Update [Case] set CaseStatusKey = '" + DropDownList4.Text + "' where CaseKey = '" + TextBox1.Text + "'";
sqlcom.CommandType = CommandType.Text;
sqlcom.ExecuteNonQuery();
sqlcom2.CommandType = CommandType.Text;
sqlcom2.ExecuteNonQuery();
conn.Close();
string scriptText = "alert('แก้ไขการแจ้งปัญหาสำเร็จ'); window.location='" + Request.ApplicationPath + "TestEdit.aspx'";
ScriptManager.RegisterStartupScript(this, this.GetType(), "alertMessage", scriptText, true);
}
}
protected void GridView1_SelectedIndexChanged1(object sender, EventArgs e)
{
Response.RedirectPermanent("~/ShowIT.aspx");
}
}
}
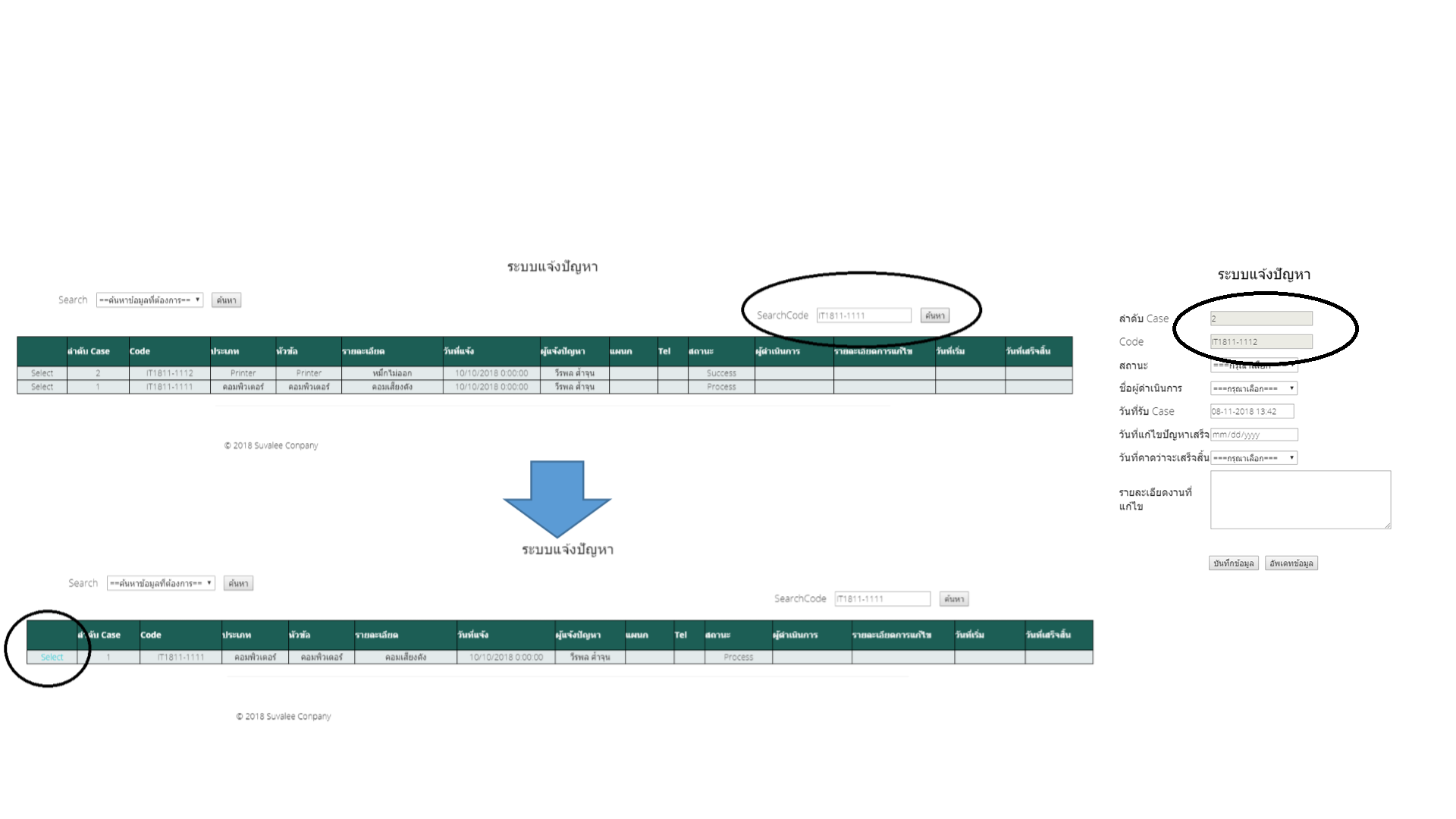
Tag : .NET, Web (ASP.NET), C#
|
ประวัติการแก้ไข 2018-11-08 13:55:42 2018-11-08 14:56:14 2018-11-08 14:56:50
|
 |
 |
 |
 |
Date :
2018-11-08 13:54:16 |
By :
wiraphon |
View :
848 |
Reply :
10 |
|
 |
 |
 |
 |
|
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
เอารูปมาให้ดูไม่มีใครช่วยแก้ได้ครับ ถ้าเป็น ASP.net ให้ใช้วิธีการ Debug ดูค่าตัวแปรครับ
|
 |
 |
 |
 |
Date :
2018-11-08 14:48:32 |
By :
mr.win |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
เครื่องมือที่ใข้ แสดงข้อมูล เขียนขึ้นเอง หรือใช้ เครื่องมืออะไรบ้าง เอาแค่ภาพมาแสดง ไม่มีใครตอบได้ครับ
ตามที่ admin บอก อยากให้ตอบ ก็ต้องมีรายละเอียดของโค๊ดมาแสดงครับ
|
 |
 |
 |
 |
Date :
2018-11-08 14:58:58 |
By :
Chaidhanan |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ใน function page_load ไม่เห็นเอาตัวแปร request ไปคิวรี่เลยอะครับ
ใช้ อะไรค้นหา เหรอครับ ใช้ javascript ช่วย หรือเปล่า
Code (C#)
protected void Page_Load(object sender, EventArgs e)
{
DateTime daValues = DateTime.Now;
string MyDt = daValues.ToString("dd-MM-yyyy HH:mm");
TextBox3.Text = DateTime.Now.ToString(MyDt);
TextBox1.Text = Request.QueryString["CaseKey"]; // 2 บันทัดนี้ไม่เห็น นำไปใช้ในการ คิวรี่ข้างล่าง
TextBox2.Text = Request.QueryString["CaseCode"];
using (SqlConnection sqlcon = new SqlConnection(connectiondb))
{
sqlcon.Open();
SqlDataAdapter sqlda = new SqlDataAdapter("SELECT LFET.CaseKey, LFET.CaseCode, ItCase.StaffName, ItCase.Solve, ItCase.Dates, ItCase.ExpectedDT, ItCase.DateHope FROM [Case] AS LFET INNER JOIN ItCase ON LFET.CaseKey = ItCase.CaseKey
## เพิ่ม where clause ตรงนี้ เอาตัวแปร ข้างบนมา generate การค้นหา
Order By ItCase.CaseKey DESC", sqlcon);
DataTable aaa = new DataTable();
sqlda.Fill(aaa);
GridView1.DataSource = aaa;
GridView1.DataBind();
}
}
|
 |
 |
 |
 |
Date :
2018-11-08 16:52:08 |
By :
Chaidhanan |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
page life cycle => Page.IsPostBack
|
 |
 |
 |
 |
Date :
2018-11-08 17:54:20 |
By :
mr.win |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
Code
protected void Page_Load(object sender, EventArgs e)
{
if(!Page.IsPostBack)
{
using (SqlConnection sqlcon = new SqlConnection(connectiondb))
{
sqlcon.Open();
SqlDataAdapter sqlda = new SqlDataAdapter("SELECT c.CaseKey, c.CaseCode, ct.Name, c.CaseName, c.CaseDetail, c.CaseDT, c.Requester, u.DeptName, u.Tel, cs.Name AS Expr1, ic.StaffName, ic.Solve, ic.Dates, ic.ExpectedDT FROM [Case] AS c LEFT OUTER JOIN CaseType AS ct ON c.CaseTypeKey = ct.CaseTypeKey LEFT JOIN Usr AS u ON c.UsrKey = u.UsrKey LEFT JOIN CaseStatus AS cs ON c.CaseStatusKey = cs.CaseStatusKey LEFT OUTER JOIN ItCase AS ic ON c.CaseKey = ic.CaseKey ORDER BY c.CaseKey DESC", sqlcon);
DataTable aaa = new DataTable();
sqlda.Fill(aaa);
GridView1.DataSource = aaa;
GridView1.DataBind();
}
}
}
|
 |
 |
 |
 |
Date :
2018-11-08 17:57:21 |
By :
mr.win |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
|
|