 |
|
ใช้ String.Format ค้นหาจาก TextBox สามารถเพิ่มเงื่อนไขได้ไหมครับ |
|
 |
|
|
 |
 |
|
โลกโปรแกรมมิ่งเปลี่ยนไปและเดินไปข้างหน้าตลอดเวลา
แต่ใจคนไม่เปลี่ยนแปลงและยังเหมือนเดิม อยู่กัมพูชาหันหลังกลับไปมอง
เออยังดีว่ะยังอยู่รัฐยะไข่
Dynamic SQL Query น่าจะตอบใจทย์ได้ทุกยุคทุกสมัย
ยุคห้าจีมันไม่ใช่ยุคห้าศูนย์
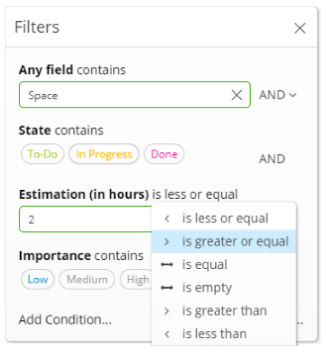
ปล. หน้าจอแบบนี้ผมเกาหำเล่นยังยากเสียยิ่งกว่า
|
 |
 |
 |
 |
Date :
2019-03-29 19:48:14 |
By :
หน้าฮี |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
จากข้อสงสัยของคุณและอีกคนหนึ่ง ณ. ที่นี้ (เขียน PHP และจะอัพขึ้น .NET Core โดยไม่ได้ใช้ EF)
จากข้อสงสัยนั้นผมแนะนำว่า ใช้ Dapper
จากข้อสงสัยของคุณผมใช้ Update แทนข้อสงสัยนั้น (หลักการเดียวกัน)
ตัวอย่างการเรียกใช้งาน
Code (C#)
clsMyFilter.Update("yourTable", new { PK1 = 1, PK2=2 }, new { Value1 = "Update1", Value2="Update2" });
Code (C#)
public static class clsMyFilter
{
private static string CommaSeparated(IEnumerable<string> list) => string.Join(",", list);
private static string SeparatedByAndStatement(IEnumerable<string> list) => string.Join(" AND ", list);
public static async Task Update(this IDbConnection con, string tableName, object identityParameters, object parametersObject)
{
IEnumerable<PropertyInfo> propertyInfo = parametersObject.GetType().GetProperties();
IEnumerable<PropertyInfo> identityPropertyInfo = identityParameters.GetType().GetProperties();
IEnumerable<string> columnsEqualToParameter = propertyInfo.Select(p => $"[{p.Name}] = @{p.Name}");
IEnumerable<string> identityColumnsEqualToParameter = identityPropertyInfo.Select(p => $"[{p.Name}] = @{p.Name}");
string sql = new StringBuilder()
.Append("UPDATE " + tableName + " SET ")
.Append(CommaSeparated(columnsEqualToParameter))
.Append($" WHERE ")
.Append(SeparatedByAndStatement(identityColumnsEqualToParameter))
.Append(";")
.ToString();
IDictionary<string, object> allParameters = new ExpandoObject();
foreach (PropertyInfo info in propertyInfo)
allParameters.Add(info.Name, parametersObject.GetType().GetProperty(info.Name).GetValue(parametersObject));
foreach (PropertyInfo info in identityPropertyInfo)
allParameters.Add(info.Name, identityParameters.GetType().GetProperty(info.Name).GetValue(identityParameters));
await con.ExecuteAsync(sql, allParameters);
}
}
|
 |
 |
 |
 |
Date :
2019-03-30 08:52:21 |
By :
หน้าฮี |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
จาก #NO4
PK1, PK2 มันก็คือชื่อฟิวด์ (Field บนดาด้าเบสนั่นแหละ) อทิเช่น ProductID, ProductCode เป็นต้น
Value1, Value2 มันก็คือชื่อฟิวด์ (Field บนดาด้าเบสนั่นแหละ) อทิเช่น ProductName, ProductType เป็นต้น
เรียกง่ายฯว่า การแมพปิ้ง(ตรูจับปิ้งเอง) ระหว่าง App <---> EF (Entity Framework)
จากรูปภาพด้านล่าง ผมจำเป็นต้องไล่โปรแกรมเมอร์ออกอีกหลายคน
(อยู่ไปก็หายใจทิ้งไปวันวัน)
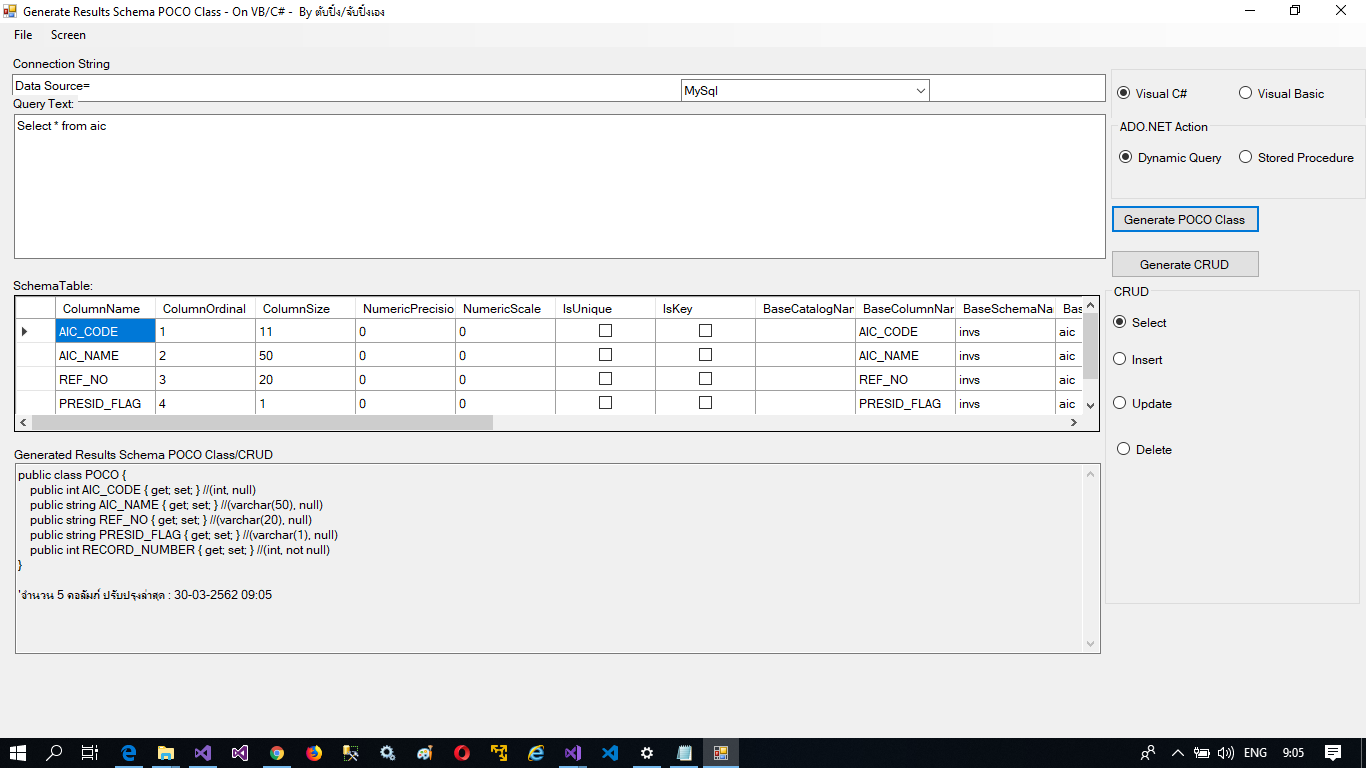
|
 |
 |
 |
 |
Date :
2019-03-30 09:03:59 |
By :
หน้าฮี |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
|
|