 |
|
สอบถามเรื่อง format date ค่ะ กำหนดรูปแบบแสดง แต่เวลารันไม่แสดงตามที่กำหนด |
|
 |
|
|
 |
 |
|
dim dateteend as string = dateviewbegin.tostring("yyyy-MM-dd")
|
 |
 |
 |
 |
Date :
2019-06-10 15:57:19 |
By :
adminliver |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ลองดูของจริงนะ
Code (VB.NET)
Imports System.Globalization
'ชั่วโมงที่ผ่านมา ทำทำอะไรไปบ้าง ยิกยิกยิก
Dim StringDate = DateTime.Now.AddHours(-1).ToString("yyyy-MM-ddTHH:mm:ssZ", CultureInfo.CreateSpecificCulture("en-US"))
Code (VB.NET)
''' <summary>
''' Usage: https://localhost:6969/api/Sura/GetGoogleMapLocations
''' </summary>
''' <returns>List of (Id, Text, Remark[warehouseCN/provinceN]) From id_wherehouse/id_province (ตาราง โรงกลั่นน้ำมัน+จังหวัด)</returns>
<Route("GetGoogleMapLocations")>
<HttpGet>
Public Async Function GetGoogleMapLocations() As Task(Of IActionResult)
Dim lstLocation As New List(Of GoogleMapLocations)
Dim sb As New System.Text.StringBuilder()
sb.Append("select sum(rain_mm) as rain_mm from tran_node_data where time >= '" & DateTime.Now.AddMinutes(-5).ToString("yyyy-MM-ddTHH:mm:ssZ", CultureInfo.CreateSpecificCulture("en-US")) & "' and time <= '" & DateTime.Now.ToString("yyyy-MM-ddTHH:mm:ssZ", CultureInfo.CreateSpecificCulture("en-US")) & "';")
sb.Append("select sum(rain_mm) as rain_mm from tran_node_data where time >= '" & DateTime.Now.AddMinutes(-15).ToString("yyyy-MM-ddTHH:mm:ssZ", CultureInfo.CreateSpecificCulture("en-US")) & "' and time <= '" & DateTime.Now.ToString("yyyy-MM-ddTHH:mm:ssZ", CultureInfo.CreateSpecificCulture("en-US")) & "';")
sb.Append("select sum(rain_mm) as rain_mm from tran_node_data where time >= '" & DateTime.Now.AddMinutes(-30).ToString("yyyy-MM-ddTHH:mm:ssZ", CultureInfo.CreateSpecificCulture("en-US")) & "' and time <= '" & DateTime.Now.ToString("yyyy-MM-ddTHH:mm:ssZ", CultureInfo.CreateSpecificCulture("en-US")) & "';")
sb.Append("select sum(rain_mm) as rain_mm from tran_node_data where time >= '" & DateTime.Now.AddMinutes(-45).ToString("yyyy-MM-ddTHH:mm:ssZ", CultureInfo.CreateSpecificCulture("en-US")) & "' and time <= '" & DateTime.Now.ToString("yyyy-MM-ddTHH:mm:ssZ", CultureInfo.CreateSpecificCulture("en-US")) & "';")
sb.Append("select sum(rain_mm) as rain_mm from tran_node_data where time >= '" & DateTime.Now.AddHours(-1).ToString("yyyy-MM-ddTHH:mm:ssZ", CultureInfo.CreateSpecificCulture("en-US")) & "' and time <= '" & DateTime.Now.ToString("yyyy-MM-ddTHH:mm:ssZ", CultureInfo.CreateSpecificCulture("en-US")) & "';")
sb.Append("select sum(rain_mm) as rain_mm from tran_node_data where time >= '" & DateTime.Now.AddHours(-3).ToString("yyyy-MM-ddTHH:mm:ssZ", CultureInfo.CreateSpecificCulture("en-US")) & "' and time <= '" & DateTime.Now.ToString("yyyy-MM-ddTHH:mm:ssZ", CultureInfo.CreateSpecificCulture("en-US")) & "';")
sb.Append("select sum(rain_mm) as rain_mm from tran_node_data where time >= '" & DateTime.Now.AddHours(-6).ToString("yyyy-MM-ddTHH:mm:ssZ", CultureInfo.CreateSpecificCulture("en-US")) & "' and time <= '" & DateTime.Now.ToString("yyyy-MM-ddTHH:mm:ssZ", CultureInfo.CreateSpecificCulture("en-US")) & "';")
sb.Append("select sum(rain_mm) as rain_mm from tran_node_data where time >= '" & DateTime.Now.AddHours(-12).ToString("yyyy-MM-ddTHH:mm:ssZ", CultureInfo.CreateSpecificCulture("en-US")) & "' and time <= '" & DateTime.Now.ToString("yyyy-MM-ddTHH:mm:ssZ", CultureInfo.CreateSpecificCulture("en-US")) & "';")
sb.Append("select sum(rain_mm) as rain_mm from tran_node_data where time >= '" & DateTime.Now.AddHours(-18).ToString("yyyy-MM-ddTHH:mm:ssZ", CultureInfo.CreateSpecificCulture("en-US")) & "' and time <= '" & DateTime.Now.ToString("yyyy-MM-ddTHH:mm:ssZ", CultureInfo.CreateSpecificCulture("en-US")) & "';")
sb.Append("select sum(rain_mm) as rain_mm from tran_node_data where time >= '" & DateTime.Now.AddHours(-24).ToString("yyyy-MM-ddTHH:mm:ssZ", CultureInfo.CreateSpecificCulture("en-US")) & "' and time <= '" & DateTime.Now.ToString("yyyy-MM-ddTHH:mm:ssZ", CultureInfo.CreateSpecificCulture("en-US")) & "'")
lstLocation.Add(New GoogleMapLocations() With {.PK = "TTC10", .PKName = "บ้านท่าตะคร้อ", .Lat = 13.116442, .Lng = 99.73253, .Title = "", .Description = "", .MarkerColor = "red"})
lstLocation.Add(New GoogleMapLocations() With {.PK = "TTC11", .PKName = "บ้านห้วยกวางจริง", .Lat = 13.005129, .Lng = 99.74265, .Title = "", .Description = "", .MarkerColor = "red"})
lstLocation.Add(New GoogleMapLocations() With {.PK = "TTC12", .PKName = "เขื่อนเพชร (เหนือน้ำ)", .Lat = 12.91296, .Lng = 99.85525, .Title = "", .Description = "", .MarkerColor = "red"})
lstLocation.Add(New GoogleMapLocations() With {.PK = "TTC13", .PKName = "เขื่อนเพชร (ท้ายน้ำ)", .Lat = 12.914403, .Lng = 99.85451, .Title = "", .Description = "", .MarkerColor = "red"})
lstLocation.Add(New GoogleMapLocations() With {.PK = "TTC14", .PKName = "ท่ายาง", .Lat = 12.973196, .Lng = 99.88275, .Title = "", .Description = "", .MarkerColor = "red"})
lstLocation.Add(New GoogleMapLocations() With {.PK = "TTC15", .PKName = "บ้านลาด", .Lat = 13.048535, .Lng = 99.91988, .Title = "", .Description = "", .MarkerColor = "red"})
lstLocation.Add(New GoogleMapLocations() With {.PK = "TTC16", .PKName = "เมืองเพชร", .Lat = 13.121885, .Lng = 99.95282, .Title = "", .Description = "", .MarkerColor = "red"})
lstLocation.Add(New GoogleMapLocations() With {.PK = "TTC17", .PKName = "บ้านแหลม", .Lat = 13.2124, .Lng = 99.98281, .Title = "", .Description = "", .MarkerColor = "red"})
lstLocation.Add(New GoogleMapLocations() With {.PK = "TTC17", .PKName = "บ้านชอบเลียหอย", .Lat = 69.2124, .Lng = 96.98281, .Title = "", .Description = "", .MarkerColor = "red"})
Await Task.Delay(0)
Return Ok(lstLocation.ToList())
End Function
|
 |
 |
 |
 |
Date :
2019-06-10 20:30:03 |
By :
หน้าฮี |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
เห็นไหมว่าการเล่นกับวันที่และเวลา มันไม่เคยจบสิ้น
(ให้เด็กฯทำ 100 คน ร้อยปีก็ไม่เสร็จ)
อันนี้ของจริงเลยนะ (ยิงมาทุก 1/10000 วินาที) ในรัศมี 50 กิโลเมตร กลางป่าเขา สีทนได้
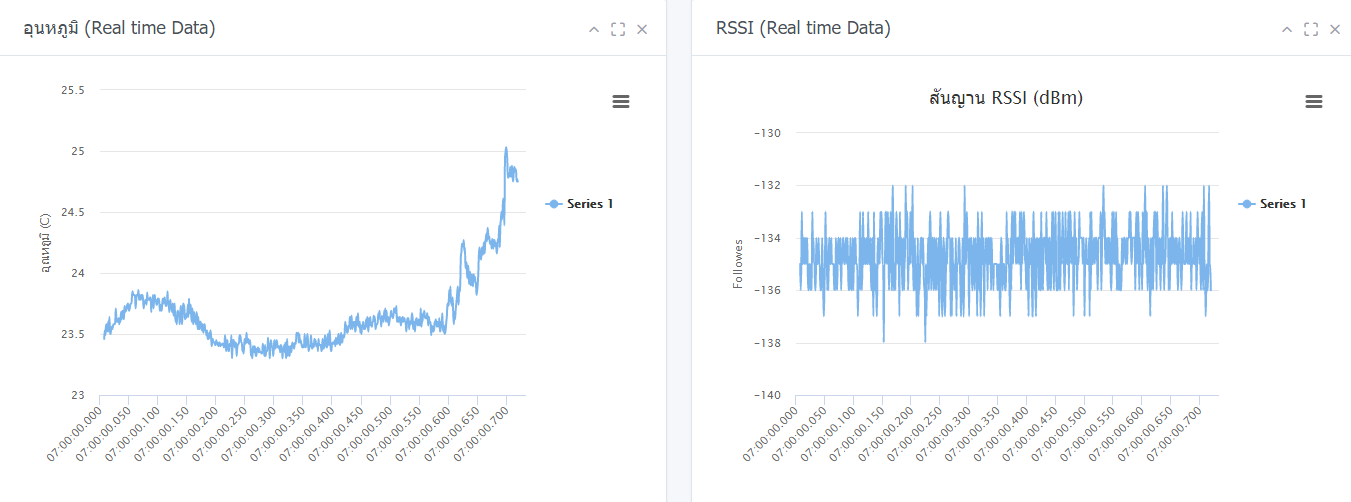
อยากดู Source Code หรือเปล่า (ของจริงเลยนะ) ไม่ใช่เด็กอมหมอยเมียร่วงหล่น เขียน
|
 |
 |
 |
 |
Date :
2019-06-10 20:43:57 |
By :
หน้าฮี |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ตัวเรียกเป็น C# (C#90%/VB.NET10%)
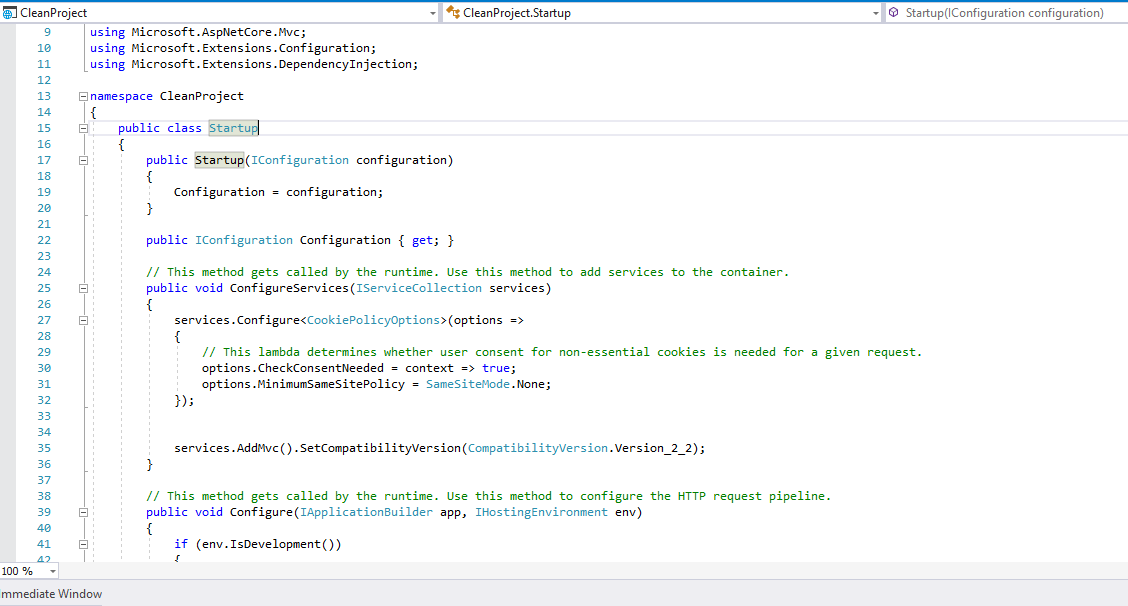
ผมมั่วไปหมด นึกอยากจะเขียนภาษาอะไรก็เขียน บางครั้งก็ภาษา Go with me/Python/etc... ห่าเหวอะไรก็ว่ากันไป
ปล. ลืมบอกไปว่า ผมใช้ Time Series Database (จริงฯแล้วตรูใช้เป็นหมดแหละ)
|
 |
 |
 |
 |
Date :
2019-06-10 20:56:19 |
By :
หน้าฮี |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
พังเพยคำโบราณ ผมเจอในผนังห้องน้ำในเมืองไทยนี่แหละ
-- พี่ทุกสถาบัน
-- พี่ไม่ต้องน้องคร่อมเอง
--- อยู่ในบ้านเก่งแต่ผมควยผัว อยู่ข้างนอก เก่งไปทุกอย่าง
--- อยู่ในบ้านของตัวเองเป็นเสือ ออกมาข้างนอก เป็นลูกหมา
...
...
...
ปล. ผมไม่ได้คิดเอง ผมเห็นเลยเอามาเล่าให้ฟังเฉยฯฯฯฯฯฯฯฯฯ
|
 |
 |
 |
 |
Date :
2019-06-10 22:42:50 |
By :
หน้าฮี |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
|
|