 |
Android รบกวนช่วยดู Code เกี่ยวกับ Android Google Map API V2 MySQL |
|
 |
|
|
 |
 |
|
ผมต้องการ add marker บน map โดยดึงข้อมูลจาก database
ตอนนี้ Code ไม่มี Error แต่มีปัญหาตอน Run หรือ Debug
เลยคิดว่าน่าจะมีตรงไหนที่ผิด เลยอยากจะรบกวนผู้เชี่ยวชาญ
ช่วยชี้แนะส่วนที่ผิดพลาด และแนวทางการแก้ไขครับ ขอบคุณครับ
Code (Android-Java)
package cpc.shopbedocafe;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.util.ArrayList;
import java.util.HashMap;
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.StatusLine;
import org.apache.http.client.ClientProtocolException;
import org.apache.http.client.HttpClient;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.impl.client.DefaultHttpClient;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import com.google.android.gms.maps.CameraUpdateFactory;
import com.google.android.gms.maps.GoogleMap;
import com.google.android.gms.maps.SupportMapFragment;
import com.google.android.gms.maps.GoogleMap.OnInfoWindowClickListener;
import com.google.android.gms.maps.model.BitmapDescriptorFactory;
import com.google.android.gms.maps.model.LatLng;
import com.google.android.gms.maps.model.Marker;
import com.google.android.gms.maps.model.MarkerOptions;
import android.content.Context;
import android.content.Intent;
import android.location.Location;
import android.location.LocationListener;
import android.location.LocationManager;
import android.os.AsyncTask;
import android.os.Bundle;
import android.support.v4.app.FragmentActivity;
import android.support.v4.app.FragmentManager;
import android.util.Log;
import android.view.Menu;
public class MapActivity extends FragmentActivity implements OnInfoWindowClickListener {
private GoogleMap myMap;
private Double lat,lng;
private LocationManager locationManager;
private String userid;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_map);
showMarker();
FragmentManager myFragmentManager = getSupportFragmentManager();
SupportMapFragment mySupportMapFragment = (SupportMapFragment)myFragmentManager.findFragmentById(R.id.map_canvas);
myMap = mySupportMapFragment.getMap();
myMap.setOnInfoWindowClickListener(this);
myMap.setMyLocationEnabled(true);
myMap.animateCamera(CameraUpdateFactory.newLatLngZoom(new LatLng(lat,lng), 8));
LocationListener locationListener = new LocationListener(){
public void onLocationChanged(Location location) {
lat = location.getLatitude();
lng = location.getLongitude();
myMap.addMarker(new MarkerOptions()
.position(new LatLng(lat,lng))
.icon(BitmapDescriptorFactory.defaultMarker(BitmapDescriptorFactory.HUE_RED))
.title("You Here"));
}
public void onProviderDisabled(String provider) {
// TODO Auto-generated method stub
}
public void onProviderEnabled(String provider) {
// TODO Auto-generated method stub
}
public void onStatusChanged(String provider, int status,
Bundle extras) {
// TODO Auto-generated method stub
}
};
locationManager = (LocationManager) getSystemService(Context.LOCATION_SERVICE);
locationManager.requestLocationUpdates(LocationManager.NETWORK_PROVIDER, 0, 0, locationListener);
}
public void showMarker() {
new LoadContentFromServer().execute();
}
class LoadContentFromServer extends AsyncTask<Object, Integer, Object> {
@Override
protected Object doInBackground(Object... params) {
String url = "http://s.creation-it.com/php/showallshop.php";
try {
JSONArray data = new JSONArray(getJSONUrl(url));
final ArrayList<HashMap<String, String>> MyArrList = new ArrayList<HashMap<String, String>>();
HashMap<String, String> map;
for(int i = 0; i < data.length(); i++) {
JSONObject c = data.getJSONObject(i);
map = new HashMap<String, String>();
map.put("UserID", c.getString("UserID"));
map.put("Name", c.getString("Name"));
map.put("Address", c.getString("Address"));
map.put("Latitude", c.getString("Latitude"));
map.put("Longitude", c.getString("Longitude"));
map.put("Picture", c.getString("Picture"));
MyArrList.add(map);
userid = map.get("UserID");
String name = map.get("Latitude");
String address = map.get("Address");
String latitude = map.get("Latitude");
String longitude = map.get("Longitude");
myMap.addMarker(new MarkerOptions()
.position(new LatLng(Double.parseDouble(latitude),Double.parseDouble(longitude)))
.icon(BitmapDescriptorFactory.defaultMarker(BitmapDescriptorFactory.HUE_ORANGE))
.title(name)
.snippet(address));
}
} catch (JSONException e) {
e.printStackTrace();
}
return null;
}
}
@Override
public void onInfoWindowClick(Marker marker) {
marker.hideInfoWindow();
Intent it = new Intent(MapActivity.this,ShopProfileActivity.class);
it.putExtra("UserID", userid);
startActivity(it);
}
public String getJSONUrl(String url) {
StringBuilder str = new StringBuilder();
HttpClient client = new DefaultHttpClient();
HttpGet httpGet = new HttpGet(url);
try {
HttpResponse response = client.execute(httpGet);
StatusLine statusLine = response.getStatusLine();
int statusCode = statusLine.getStatusCode();
if (statusCode == 200) {
HttpEntity entity = response.getEntity();
InputStream content = entity.getContent();
BufferedReader reader = new BufferedReader(new InputStreamReader(content));
String line;
while ((line = reader.readLine()) != null) {
str.append(line);
}
} else {
Log.e("Log", "Failed to download file..");
}
} catch (ClientProtocolException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
return str.toString();
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.activity_map, menu);
return true;
}
}
Tag : Mobile, MySQL, Android
|
|
 |
 |
 |
 |
Date :
2013-01-27 16:55:34 |
By :
Lay |
View :
3366 |
Reply :
8 |
|
 |
 |
 |
 |
|
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ใช้การ Debug ดูค่านี้ครับ getJSONUrl(url)
|
 |
 |
 |
 |
Date :
2013-01-28 20:20:43 |
By :
mr.win |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ขอบคุณครับ ให้รุ่นพี่ช่วยดู Code เจอปัญหาแล้วว่าไม่มี onPostExecute ครับ กับการวางตำแน่งของคำสั่งต่าง ๆ นิดหน่อย ซึ่งแก้ไขแล้วสามารถใช้งานได้ตามปกติแล้วครับ
Code (Android-Java)
package cpc.shopbedocafe;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.util.ArrayList;
import java.util.HashMap;
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.StatusLine;
import org.apache.http.client.ClientProtocolException;
import org.apache.http.client.HttpClient;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.impl.client.DefaultHttpClient;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import com.google.android.gms.common.ConnectionResult;
import com.google.android.gms.common.GooglePlayServicesUtil;
import com.google.android.gms.maps.CameraUpdateFactory;
import com.google.android.gms.maps.GoogleMap;
import com.google.android.gms.maps.SupportMapFragment;
import com.google.android.gms.maps.GoogleMap.OnInfoWindowClickListener;
import com.google.android.gms.maps.model.BitmapDescriptorFactory;
import com.google.android.gms.maps.model.LatLng;
import com.google.android.gms.maps.model.Marker;
import com.google.android.gms.maps.model.MarkerOptions;
import android.content.Context;
import android.content.Intent;
import android.location.Location;
import android.location.LocationListener;
import android.location.LocationManager;
import android.os.AsyncTask;
import android.os.Bundle;
import android.support.v4.app.FragmentActivity;
import android.support.v4.app.FragmentManager;
import android.util.Log;
import android.view.Menu;
import android.widget.Toast;
public class MapActivity extends FragmentActivity implements OnInfoWindowClickListener {
final int RQS_GooglePlayServices = 1;
private GoogleMap myMap;
private LocationManager locationManager;
private String userid;
private String name, address, latitude, longitude;
Double lat;
Double lng;
final ArrayList<HashMap<String, String>> MyArrList = new ArrayList<HashMap<String, String>>();
HashMap<String, String> map;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_map);
FragmentManager myFragmentManager = getSupportFragmentManager();
SupportMapFragment mySupportMapFragment = (SupportMapFragment) myFragmentManager
.findFragmentById(R.id.map_canvas);
myMap = mySupportMapFragment.getMap();
myMap.setOnInfoWindowClickListener(this);
myMap.setMyLocationEnabled(true);
LocationListener locationListener = new LocationListener() {
public void onLocationChanged(Location location) {
LatLng latlng = new LatLng(location.getLatitude(),
location.getLongitude());
myMap.animateCamera(CameraUpdateFactory.newLatLngZoom((latlng),
10));
}
public void onProviderDisabled(String provider) {
// TODO Auto-generated method stub
}
public void onProviderEnabled(String provider) {
// TODO Auto-generated method stub
}
public void onStatusChanged(String provider, int status,
Bundle extras) {
// TODO Auto-generated method stub
}
};
locationManager = (LocationManager) getSystemService(Context.LOCATION_SERVICE);
locationManager.requestLocationUpdates(
LocationManager.NETWORK_PROVIDER, 20, 20, locationListener);
showMarker();
}
@Override
protected void onResume() {
super.onResume();
int resultCode = GooglePlayServicesUtil
.isGooglePlayServicesAvailable(getApplicationContext());
if (resultCode == ConnectionResult.SUCCESS) {
Toast.makeText(getApplicationContext(),
"GooglePlayServices is Available", Toast.LENGTH_LONG)
.show();
} else {
GooglePlayServicesUtil.getErrorDialog(resultCode, this,
RQS_GooglePlayServices);
}
}
@Override
public void onInfoWindowClick(Marker marker) {
marker.hideInfoWindow();
Intent it = new Intent(MapActivity.this,ShopProfileActivity.class);
it.putExtra("UserID", userid);
startActivity(it);
}
public void addMarker() {
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.activity_map, menu);
return true;
}
public void showMarker() {
new LoadContentFromServer().execute();
}
class LoadContentFromServer extends AsyncTask<String, Integer, String> {
@Override
protected String doInBackground(String... params) {
String url = "http://s.creation-it.com/php/showallshop.php";
return getJSONUrl(url);
}
public String getJSONUrl(String url) {
StringBuilder str = new StringBuilder();
HttpClient client = new DefaultHttpClient();
HttpGet httpGet = new HttpGet(url);
try {
HttpResponse response = client.execute(httpGet);
StatusLine statusLine = response.getStatusLine();
int statusCode = statusLine.getStatusCode();
if (statusCode == 200) {
HttpEntity entity = response.getEntity();
InputStream content = entity.getContent();
BufferedReader reader = new BufferedReader(
new InputStreamReader(content));
String line;
while ((line = reader.readLine()) != null) {
str.append(line);
}
} else {
Log.e("Log", "Failed to download file..");
}
} catch (ClientProtocolException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
return str.toString();
}
@Override
protected void onPostExecute(String result) {
try {
JSONArray data = new JSONArray(result);
for (int i = 0; i < data.length(); i++) {
JSONObject c = data.getJSONObject(i);
map = new HashMap<String, String>();
map.put("UserID", c.getString("UserID"));
map.put("Name", c.getString("Name"));
map.put("Address", c.getString("Address"));
map.put("Latitude", c.getString("Latitude"));
map.put("Longitude", c.getString("Longitude"));
map.put("Picture", c.getString("Picture"));
MyArrList.add(map);
userid = map.get("UserID");
name = map.get("Name");
address = map.get("Address");
latitude = map.get("Latitude");
longitude = map.get("Longitude");
lat = Double.parseDouble(latitude);
lng = Double.parseDouble(longitude);
try {
myMap.addMarker(new MarkerOptions()
.position(new LatLng(lat, lng))
.icon(BitmapDescriptorFactory
.defaultMarker(BitmapDescriptorFactory.HUE_ORANGE))
.title(name).snippet(address));
} catch (Exception e) {
e.printStackTrace();
}
}
} catch (JSONException e) {
e.printStackTrace();
}
}
}
}
|
 |
 |
 |
 |
Date :
2013-01-30 02:29:08 |
By :
Two |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
จัดไปครับ
|
 |
 |
 |
 |
Date :
2013-01-30 05:49:22 |
By :
mr.win |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
สวัสดีครับพี่ ผมอยากทราบว่าพี่พอจะทำบทความเพื่อเผยแพร่ให้ได้รึเปล่าครับ ในเรื่องนี้อ่ะครับ
|
 |
 |
 |
 |
Date :
2013-12-26 10:27:44 |
By :
สมพงษ์ |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ไม่ทราบว่าคุณพอจะจำลิงก์ได้รึเปล่าครับ ผมเข้าไปดูมีแต่ของ php ไม่เห็นมีของ android เลยครับ รบกวนช่วยหาหน่อยได้ไหมครับ
|
 |
 |
 |
 |
Date :
2013-12-27 13:44:54 |
By :
สมพงษ์ |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ลองศึกษาเพิ่มเติมบทความ Android กับ Google Map ครับ
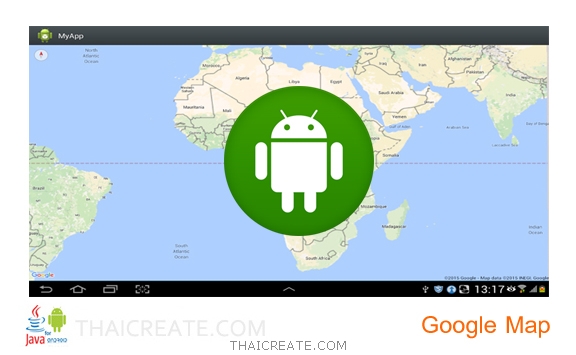
Android Google Map (Step by Step)
|
 |
 |
 |
 |
Date :
2015-11-18 14:13:02 |
By :
mr.win |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
จากบล็อกของ admin เขียนมาจาก https://www.thaicreate.com/mobile/android-google-map-marker-location.html ผมได้ลองทำดูแล้วมันแดงที่ getmap(); อ่ะครับ ไม่ทราบว่าเป็นเพราะอะไรครับ จากโค้ดด้านล่างที่ตัวอักษรสีแดงครับ ขอบคุณครับ
Code
package com.myapp;
import android.os.Bundle;
import android.support.v4.app.FragmentActivity;
import com.google.android.gms.maps.CameraUpdateFactory;
import com.google.android.gms.maps.GoogleMap;
import com.google.android.gms.maps.SupportMapFragment;
import com.google.android.gms.maps.model.BitmapDescriptorFactory;
import com.google.android.gms.maps.model.LatLng;
import com.google.android.gms.maps.model.MarkerOptions;
import android.content.Context;
import android.location.Location;
import android.location.LocationListener;
import android.location.LocationManager;
public class MainActivity extends FragmentActivity implements LocationListener {
protected LocationManager locationManager;
protected LocationListener locationListener;
// Google Map
private GoogleMap googleMap;
// Latitude & Longitude
private Double Latitude = 0.00;
private Double Longitude = 0.00;
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
//*** Location
locationManager = (LocationManager) getSystemService(Context.LOCATION_SERVICE);
locationManager.requestLocationUpdates(LocationManager.GPS_PROVIDER, 0, 0, this);
//*** Display Google Map
googleMap = ((SupportMapFragment)getSupportFragmentManager()
.findFragmentById(R.id.googleMap)).getMap();
}
@Override
public void onLocationChanged(Location location) {
if (location == null) { return; }
//** Get Latitude & Longitude
Latitude = location.getLatitude();
Longitude= location.getLongitude();
googleMap.clear();
//*** Focus & Zoom
LatLng coordinate = new LatLng(Latitude, Longitude);
googleMap.setMapType(com.google.android.gms.maps.GoogleMap.MAP_TYPE_HYBRID);
googleMap.animateCamera(CameraUpdateFactory.newLatLngZoom(coordinate, 17));
//*** Marker
MarkerOptions marker = new MarkerOptions()
.position(new LatLng(Latitude, Longitude)).title("Your current location");
marker.icon(BitmapDescriptorFactory.fromResource(R.drawable.marker));
googleMap.addMarker(marker);
}
@Override
public void onProviderDisabled(String provider) {
// Log.d("Latitude","disable");
}
@Override
public void onProviderEnabled(String provider) {
// Log.d("Latitude","enable");
}
@Override
public void onStatusChanged(String provider, int status, Bundle extras) {
// Log.d("Latitude","status");
}
}
|
ประวัติการแก้ไข 2016-10-13 12:42:35
 |
 |
 |
 |
Date :
2016-10-13 12:39:53 |
By :
arthit |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
|
|