 |
iOS - Run UITableView แล้วไม่ขึ้นข้อมูลอะไรออกมาเลย ช่วยด้วยครับ TT |
|
 |
|
|
 |
 |
|
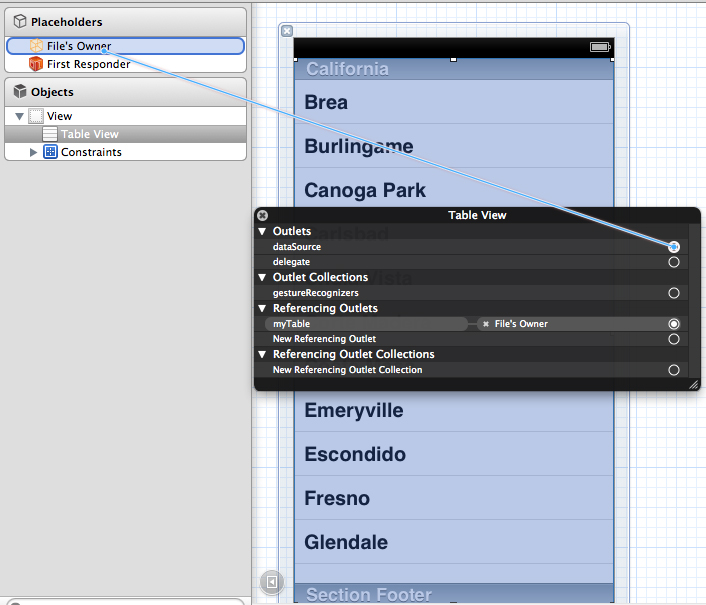
ให้เดา น่าจะไมไ่ด้เชื่อม Delegate ตัว Method หรือเปล่าครับ
|
 |
 |
 |
 |
Date :
2014-11-16 19:48:08 |
By :
mr.win |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ผมเชื่อม delegate กับ datasource แล้วคับ
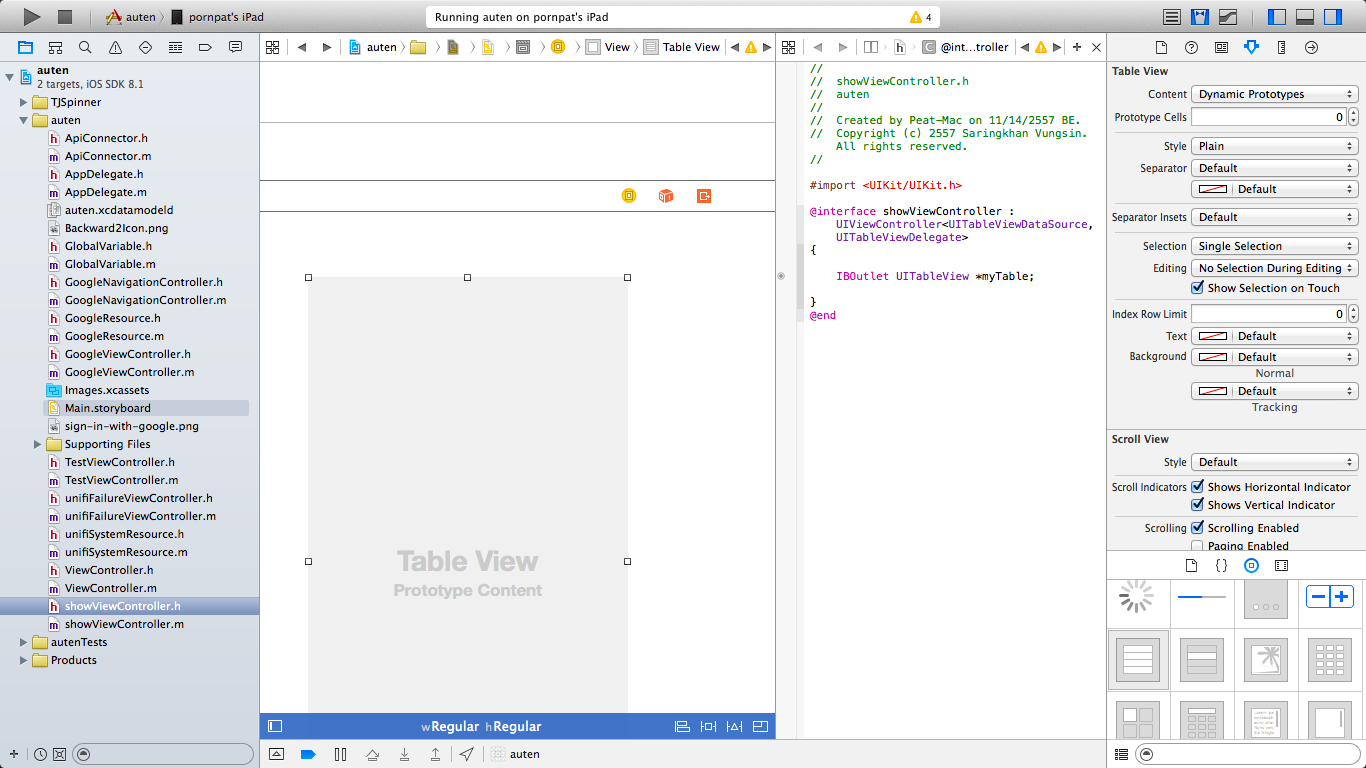
ไฟล์ .m
Code
//
// ViewController.m
// getDataFromServer
//
// Created by Weerachai on 12/10/55 BE.
// Copyright (c) 2555 Weerachai. All rights reserved.
//
#import "showViewController.h"
@interface showViewController ()
{
NSMutableArray *myObject;
// A dictionary object
NSDictionary *dict;
// Define keys
NSString *device_id;
NSString *ip_address;
NSString *mac_address;
NSMutableData *receivedData;
UIAlertView *loading;
}
@end
@implementation showViewController
- (void)viewDidLoad
{
[super viewDidLoad];
// Do any additional setup after loading the view, typically from a nib.
// Define keys
device_id = @"device_id";
ip_address = @"ip_address";
mac_address = @"mac_address";
// Create array to hold dictionaries
myObject = [[NSMutableArray alloc] init];
NSURLRequest *theRequest =
[NSURLRequest requestWithURL:[NSURL URLWithString:@"http://202.44.47.47/mac/show.php"]
cachePolicy:NSURLRequestReloadIgnoringLocalCacheData
timeoutInterval:10.0];
NSURLConnection *theConnection=[[NSURLConnection alloc] initWithRequest:theRequest delegate:self];
// Loading...
[UIApplication sharedApplication].networkActivityIndicatorVisible = YES;
loading = [[UIAlertView alloc] initWithTitle:@"" message:@"Please Wait..." delegate:nil cancelButtonTitle:nil otherButtonTitles:nil];
UIActivityIndicatorView *progress= [[UIActivityIndicatorView alloc] initWithFrame:CGRectMake(125, 50, 30, 30)];
progress.activityIndicatorViewStyle = UIActivityIndicatorViewStyleWhiteLarge;
[loading addSubview:progress];
[progress startAnimating];
[loading show];
if (theConnection) {
receivedData = nil;
} else {
UIAlertView *connectFailMessage = [[UIAlertView alloc] initWithTitle:@"NSURLConnection " message:@"Failed in viewDidLoad" delegate: self cancelButtonTitle:@"Ok" otherButtonTitles: nil];
[connectFailMessage show];
}
}
- (void)connection:(NSURLConnection *)connection didReceiveResponse:(NSURLResponse *)response
{
receivedData = [[NSMutableData alloc] init];
}
- (void)connection:(NSURLConnection *)connection didReceiveData:(NSData *)data
{
sleep(2);
[receivedData appendData:data];
}
- (void)connection:(NSURLConnection *)connection didFailWithError:(NSError *)error
{
// inform the user
UIAlertView *didFailWithErrorMessage = [[UIAlertView alloc] initWithTitle: @"NSURLConnection " message: @"didFailWithError" delegate: self cancelButtonTitle: @"Ok" otherButtonTitles: nil];
[didFailWithErrorMessage show];
//inform the user
NSLog(@"Connection failed! Error - %@", [error localizedDescription]);
}
- (void)connectionDidFinishLoading:(NSURLConnection *)connection
{
[UIApplication sharedApplication].networkActivityIndicatorVisible = NO;
[loading dismissWithClickedButtonIndex:0 animated:YES];
if(receivedData)
{
//NSLog(@"%@",receivedData);
//NSString *dataString = [[NSString alloc] initWithData:receivedData encoding:NSASCIIStringEncoding];
//NSLog(@" abc = %@",dataString);
id jsonObjects = [NSJSONSerialization JSONObjectWithData:receivedData options:NSJSONReadingMutableContainers error:nil];
// values in foreach loop
for (NSDictionary *dataDict in jsonObjects) {
NSString *strdevice_id = [dataDict objectForKey:@"Device_id"];
NSString *strip_address = [dataDict objectForKey:@"IP_Address"];
NSString *strmac_address = [dataDict objectForKey:@"MAC_Address"];
dict = [NSDictionary dictionaryWithObjectsAndKeys:
strdevice_id, device_id,
strip_address, ip_address,
strmac_address, mac_address,
nil];
[myObject addObject:dict];
}
[myTable reloadData];
}
// release the connection, and the data object
}
- (NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section
{
int nbCount = [myObject count];
if (nbCount == 0)
return 1;
else
return [myObject count];
}
- (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath
{
static NSString *CellIdentifier = @"Cell";
UITableViewCell *cell = [tableView dequeueReusableCellWithIdentifier:CellIdentifier];
if (cell == nil) {
// Use the default cell style.
cell = [[UITableViewCell alloc] initWithStyle : UITableViewCellStyleSubtitle
reuseIdentifier : CellIdentifier];
}
int nbCount = [myObject count];
if (nbCount ==0)
cell.textLabel.text = @"Loading Data";
else
{
NSDictionary *tmpDict = [myObject objectAtIndex:indexPath.row];
// MemberID
NSMutableString *text;
text = [NSMutableString stringWithFormat:@"Device ID : %@",[tmpDict objectForKey:device_id]];
// Name & Tel
NSMutableString *detail;
detail = [NSMutableString stringWithFormat:@"MAC : %@ , IP : %@"
,[tmpDict objectForKey:mac_address]
,[tmpDict objectForKey:ip_address]];
cell.textLabel.text = text;
cell.detailTextLabel.text= detail;
//[tmpDict objectForKey:memberid]
//[tmpDict objectForKey:name]
//[tmpDict objectForKey:tel]
}
return cell;
}
- (void)didReceiveMemoryWarning
{
[super didReceiveMemoryWarning];
// Dispose of any resources that can be recreated.
}
@end
ไฟล.h
Code
//
// showViewController.h
// auten
//
// Created by Peat-Mac on 11/14/2557 BE.
// Copyright (c) 2557 Saringkhan Vungsin. All rights reserved.
//
#import <UIKit/UIKit.h>
@interface showViewController : UIViewController<UITableViewDataSource,UITableViewDelegate>
{
IBOutlet UITableView *myTable;
}
@end
|
 |
 |
 |
 |
Date :
2014-11-17 12:51:19 |
By :
peatza130 |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
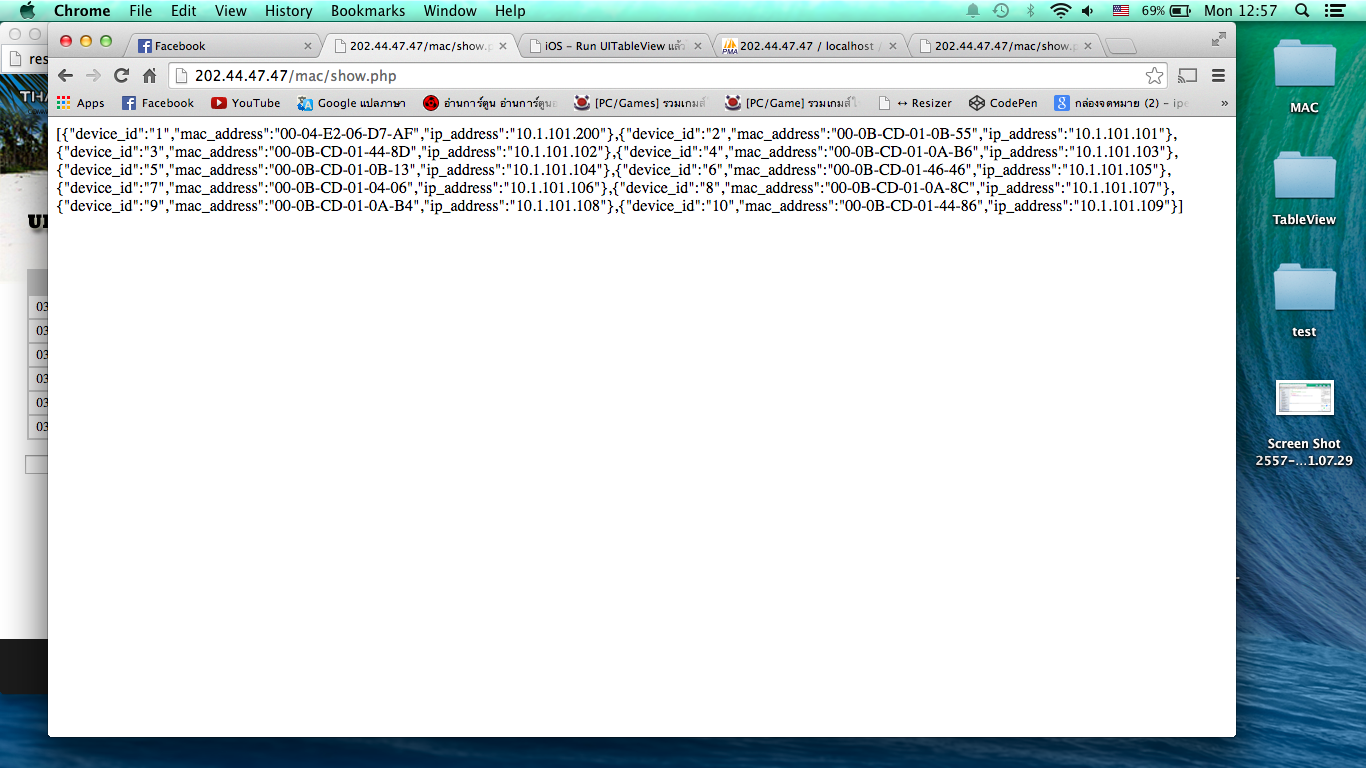
ข้อมูลจาก DB คับ
|
 |
 |
 |
 |
Date :
2014-11-17 12:59:03 |
By :
peatza130 |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ผม nslog ออกมาดูค่าในแต่ละฟังชัน ฟังชัน
- (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath
เหมือนมันไม่ถุกเรียกใช่งานเลยอะคับ เพราะ ใช่คำสั่ง
NSLog(@"receivedData: %d",nbCount);
มันไม่มีอะไรออกมาเลยแม้แต่ receivedData
|
 |
 |
 |
 |
Date :
2014-11-17 14:00:51 |
By :
peatza130 |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
มันไม่ถูกเรียกเพราะคุณยังไม่ได้เชื่อม Delegate ตัว Method น่ะครับ
|
 |
 |
 |
 |
Date :
2014-11-18 20:13:49 |
By :
mr.win |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
|
|