 |
|
พอดีผมเพิ่งหัดเรียนเขียน App เมื่อเขียนเสร็จแล้วมันแสดงค่าแยกเป็น 2 หน้า โดยแต่ละหน้ามีข้อมูล 1 บรรทัด ผมต้องแก้ยังไงให้มันแสดงผลเป็น 1 หน้าแต่มีข้อมูล 2 บรรทัดครับ
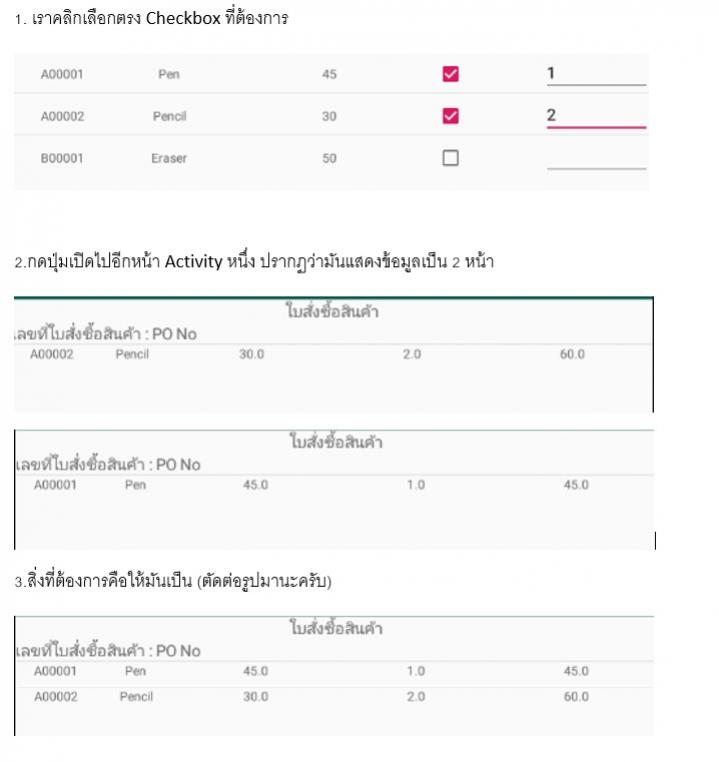
Code หน้าเลือก Checkbox
Code (Android-Java)
Button btnGetItem = (Button) findViewById(R.id.btnGetItem);
btnGetItem.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
String txtID = null,txtName=null;
double a=0, b=0, val=0, total = 0;
int Count = 0;
int count = lisView1.getAdapter().getCount();
for (int i = 0; i < count; i++) {
LinearLayout itemLayout = (LinearLayout) lisView1.getChildAt(i); // Find by under LinearLayout
CheckBox checkbox = (CheckBox) itemLayout.findViewById(R.id.ColChk);
if (checkbox.isChecked()) {
TextView txtPrice = (TextView) itemLayout.findViewById(ColPrice);
EditText txtNum = (EditText) itemLayout.findViewById(R.id.ColNum);
TextView ID = (TextView) itemLayout.findViewById(ColProductID);
TextView Name = (TextView) itemLayout.findViewById(ColName);
txtID = ID.getText().toString();
txtName = Name.getText().toString();
a = Double.parseDouble(txtPrice.getText().toString());
b = Double.parseDouble(txtNum.getText().toString());
val = a * b;
total = total + val;
Count = Count + 1;
Intent PO_Page = new Intent(SelectProductActivity.this, PurchaseOrderActivity.class);
PO_Page.putExtra("ProductID", txtID);
PO_Page.putExtra("Name", txtName);
PO_Page.putExtra("Price", a);
PO_Page.putExtra("Num", b);
PO_Page.putExtra("Val", val);
PO_Page.putExtra("Count", Count);
startActivity(PO_Page);
}
}
}
});
Code หน้าแสดงผล
Code (Android-Java)
public class PurchaseOrderActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
getSupportActionBar().hide();
setContentView(R.layout.activity_purchase_order);
Bundle bundle = getIntent().getExtras();
String txtProductID = bundle.getString("ProductID");
String txtName = bundle.getString("Name");
double Price = bundle.getDouble("Price");
double Num = bundle.getDouble("Num");
double Val = bundle.getDouble("Val");
Integer count=bundle.getInt("Count");
ArrayList<HashMap<String, String>> MyArrList = new ArrayList<HashMap<String, String>>();
HashMap<String, String> map;
map = new HashMap<>();
map.put("ProductID", txtProductID);
map.put("Name", txtName);
map.put("Price", Double.toString(Price));
map.put("Num", Double.toString(Num));
map.put("Val", Double.toString(Val));
MyArrList.add(map);
final ListView listView1 = (ListView) findViewById(R.id.listView1);
SimpleAdapter sAdap;
sAdap = new SimpleAdapter(PurchaseOrderActivity.this, MyArrList, R.layout.activity_column_po,
new String[] {"ProductID", "Name","Price","Num","Val"}, new int[]{R.id.ColProductID, R.id.ColName, R.id.ColPrice, R.id.ColNum, R.id.ColVal});
listView1.setAdapter(sAdap);
Button btnCancel = (Button)findViewById(R.id.btnCancel);
btnCancel.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
finish();
}
});
}
}
Tag : Mobile, Android
|
|
 |
 |
 |
 |
Date :
2020-01-17 15:12:32 |
By :
Pong_Virgin |
View :
1065 |
Reply :
1 |
|
 |
 |
 |
 |
|
|
|
 |