 |
|
ตอนรันบนเครื่องก็ไม่พบปัญหาอะไร ภาษาไทยก็แสดงผลได้ แต่พออัพขึ้น host จริงกับหาฟอนต์ไม่เจอ ทั้งทีฟอนต์ก็อัพขึ้นตามกระทู้ที่ให้เอาใส่ใน folder fonts ให้อยู่ในระดับเดียวกันกับ เพจแสดงกราฟ ก็ยังไม่ได้
เขียนโค้ดแบบนี้
<?php
define('TTF_DIR',dirname(__FILE__).'/fonts/');
include("config.php");
require_once("../jpgraph/jpgraph.php");
require_once("../jpgraph/jpgraph_bar.php");
echo TTF_DIR;
$m = $_GET['month'];
$y = $_GET['year'];
$arr = array("มกราคม-ธันวาคม","มกราคม","กุมภาพันธ์","มีนาคม","เมษายน","พฤษภาคม","มิถุนายน","กรกฎาคม","สิงหาคม","กันยายน","ตุลาคม",
"พฤศจิกายน","ธันวาคม");
// ติดต่อ ฐานข้อมูล เลือกข้อมูลขึ้นมาเพื่อแสดงกราฟ
if($m==0)
{
$strSQL = "SELECT o.ot_code as ot_code, Count(t.ot_id) as count_ot_id FROM patient AS t INNER JOIN ot AS o ON o.ot_id = t.ot_id
WHERE MONTH(pat_date) = $m OR YEAR(pat_date) = $y GROUP BY o.ot_code";
}else{
$strSQL = "SELECT o.ot_code as ot_code, Count(t.ot_id) as count_ot_id FROM patient AS t INNER JOIN ot AS o ON o.ot_id = t.ot_id
WHERE MONTH(pat_date) = $m AND YEAR(pat_date) = $y GROUP BY o.ot_code";
}
$objQuery = mysql_query($strSQL) or die ("Error Query [".$strSQL."]");
// เตรียมข้อมุลที่จะแสดง เพื่อสร้างกราฟ
//$datay=array();
while($objResult = mysql_fetch_array($objQuery))
{
$datay[] = $objResult["count_ot_id"];
$datax[] = $objResult["ot_code"];
}
// Create the graph.
$graph = new Graph(600,400,'auto');
$graph->SetScale("textlin");
$graph->SetMarginColor('navy:1.9');
$graph->SetBox();
$graph->SetMargin(60,50,0,80);
// Create and add a new text
$txt=new Text('เดือน '.$arr[$m].' ปี '.((int)$y+543));
$txt->SetPos(220,360);
$txt->SetColor('#000000');
$txt->SetFont(FF_ANGSA,FS_BOLD,15);
$txt->SetBox('yellow','navy','#[email protected]');
$graph->AddText($txt);
// Setup the titles
$graph->title->Set('กราฟแสดงจำนวนผู้ป่วย');
$graph->xaxis->title->Set('OT');
$graph->yaxis->title->Set('จำนวนผู้ป่วย');
$graph->ygrid->SetFill(true,'#[email protected]','#[email protected]');
$graph->title->SetFont(FF_ANGSA,FS_BOLD,16);
$graph->yaxis->title->SetFont(FF_ANGSA,FS_BOLD,16);
$graph->xaxis->title->SetFont(FF_ANGSA,FS_BOLD,16);
$graph->title->Set('กราฟแสดงจำนวน');
$graph->title->SetFont(FF_ANGSA,FS_BOLD,18);
$graph->SetTitleBackground('lightblue:1.3',TITLEBKG_STYLE2,TITLEBKG_FRAME_BEVEL);
$graph->SetTitleBackgroundFillStyle(TITLEBKG_FILLSTYLE_HSTRIPED,'lightblue','blue');
$graph->xaxis->SetTickLabels($datax);
// Create a bar pot
$bplot = new BarPlot($datay);
$bplot->SetFillColor('#ff9900');
$bplot->SetWidth(0.6);
$bplot->SetPattern(PATTERN_CROSS1,'navy');
$graph->Add($bplot);
$graph->Stroke();
?>
ก็ฟ้องแบบนี้
/home/zp5800/domains/zp5800.tld/public_html/webpages/fonts/JpGraph Error: 25049 Font file "/home/zp5800/domains/zp5800.tld/public_html/webpages/fonts/ANGSAB.ttf" is not readable or does not exist.
แต่ถ้าไม่ใส่โค้ด
//define('TTF_DIR',dirname(__FILE__).'/fonts/');
include("config.php");
require_once("../jpgraph/jpgraph.php");
require_once("../jpgraph/jpgraph_bar.php");
//echo TTF_DIR;
ก็จะเป็นแบบนี้
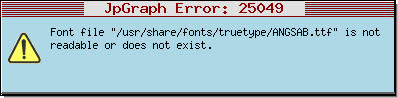
ใครแก้ปัญหานี้ได้ช่วยบอกทีครับ
กระทู้ที่ได้เข้าไปดูแล้ว แต่ก็ยังแก้ไม่ได้ครับ
https://www.thaicreate.com/php/forum/042664.html
https://www.thaicreate.com/php/forum/061798.html
https://www.thaicreate.com/php/forum/041909.html
Tag : PHP
|
|
 |
 |
 |
 |
Date :
2012-06-15 12:23:55 |
By :
บู้ |
View :
1700 |
Reply :
4 |
|
 |
 |
 |
 |
|
|
|
 |