 |
ต้องการโชว์ข้อมูลที่กรอกลงไปบนหน้าเว็บนึง เพื่อไปโชว์อีกหน้าเว็บนึง mysql php |
|
 |
|
|
 |
 |
|
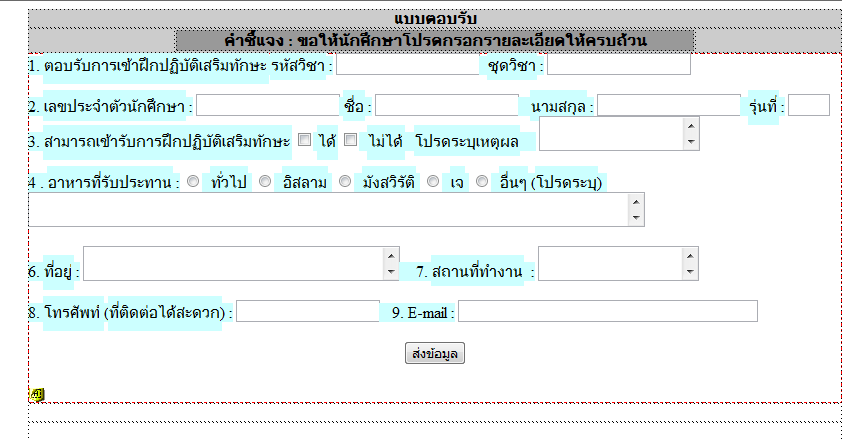
คือต้องการให้ข้อมูลที่กรอกลงไปมาใชว์อีกหน้าเว็บนึงอ่ะคะ
Code (PHP)
<?php require_once('Connections/conn.php'); ?>
<?php
if (!function_exists("GetSQLValueString")) {
function GetSQLValueString($theValue, $theType, $theDefinedValue = "", $theNotDefinedValue = "")
{
if (PHP_VERSION < 6) {
$theValue = get_magic_quotes_gpc() ? stripslashes($theValue) : $theValue;
}
$theValue = function_exists("mysql_real_escape_string") ? mysql_real_escape_string($theValue) : mysql_escape_string($theValue);
switch ($theType) {
case "text":
$theValue = ($theValue != "") ? "'" . $theValue . "'" : "NULL";
break;
case "long":
case "int":
$theValue = ($theValue != "") ? intval($theValue) : "NULL";
break;
case "double":
$theValue = ($theValue != "") ? doubleval($theValue) : "NULL";
break;
case "date":
$theValue = ($theValue != "") ? "'" . $theValue . "'" : "NULL";
break;
case "defined":
$theValue = ($theValue != "") ? $theDefinedValue : $theNotDefinedValue;
break;
}
return $theValue;
}
}
$editFormAction = $_SERVER['PHP_SELF'];
if (isset($_SERVER['QUERY_STRING'])) {
$editFormAction .= "?" . htmlentities($_SERVER['QUERY_STRING']);
}
if ((isset($_POST["MM_insert"])) && ($_POST["MM_insert"] == "form1")) {
$insertSQL = sprintf("INSERT INTO tb_accept_human (student_id, name, lastname, code, list, version, accept, accept_other, food_other, address, officer, phone, email) VALUES (%s, %s, %s, %s, %s, %s, %s, %s, %s, %s, %s, %s, %s)",
GetSQLValueString($_POST['student_id'], "text"),
GetSQLValueString($_POST['name'], "text"),
GetSQLValueString($_POST['lastname'], "text"),
GetSQLValueString($_POST['code'], "text"),
GetSQLValueString($_POST['list'], "text"),
GetSQLValueString($_POST['version'], "text"),
GetSQLValueString(isset($_POST['accept']) ? "true" : "", "defined","'Y'","'N'"),
GetSQLValueString($_POST['accept_other'], "text"),
GetSQLValueString($_POST['food_other'], "text"),
GetSQLValueString($_POST['address'], "text"),
GetSQLValueString($_POST['officer'], "text"),
GetSQLValueString($_POST['phone'], "text"),
GetSQLValueString($_POST['email'], "text"));
mysql_select_db($database_conn, $conn);
$Result1 = mysql_query($insertSQL, $conn) or die(mysql_error());
$insertGoTo = "check_accept_human.php";
if (isset($_SERVER['QUERY_STRING'])) {
$insertGoTo .= (strpos($insertGoTo, '?')) ? "&" : "?";
$insertGoTo .= $_SERVER['QUERY_STRING'];
}
header(sprintf("Location: %s", $insertGoTo));
}
?>
<!doctype html>
<html>
<head>
<meta charset="utf-8">
<title>แบบกรอกรายละเอียด</title>
</head>
<body>
<table width="814" border="0" align="center" cellpadding="0" cellspacing="0">
<tr>
<th width="1819" bgcolor="#CCCCCC" scope="col">แบบตอบรับ</th>
</tr>
<tr>
<td bgcolor="#CCCCCC"><table width="522" border=0" align="center">
<tr bgcolor="#999999">
<th align="center" valign="middle" scope="col" colortxt="red">คำชี้แจง : ขอให้นักศึกษาโปรดกรอกรายละเอียดให้ครบถ้วน</th>
</tr>
</table></td>
</tr>
<tr align="left">
<td><form name="form1" method="POST" action="<?php echo $editFormAction; ?>">
<p>1. ตอบรับการเข้าฝึกปฏิบัติเสริมทักษะ รหัสวิชา :
<label for="code"></label>
<input type="text" name="code" id="code">
ชุดวิชา :
<label for="list"></label>
<input type="text" name="list" id="list">
</p>
<p>2. เลขประจำตัวนักศึกษา :
<label for="phone"></label>
<input type="text" name="student_id" id="textfield4">
ชื่อ :
<label for="lastname"></label>
<input type="text" name="name" id="textfield2">
นามสกุล
:
<label for="student_id"></label>
<input type="text" name="lastname" id="textfield3">
รุ่นที่
<label for="version"></label>
:
<input name="version" type="text" id="version" size="7">
3. สามารถเข้ารับการฝึกปฏิบัติเสริมทักษะ
<input name="accept" type="checkbox" id="accept">
<label for="accept"></label>
ได้
<input type="checkbox" name="decline" id="decline">
<label for="decline"></label>
ไม่ได้ โปรดระบุเหตุผล
<textarea name="accept_other" id="accept_other"></textarea>
</p>
<p>
<label for="accept_other"></label>
4
. อาหารที่รับประทาน :
<input type="radio" name="food_general" id="radio" value="food_general">
<label for="food_general"></label>
ทั่วไป
<input type="radio" name="food_halal" id="radio2" value="radio2">
<label for="food_halal"></label>
อิสลาม
<input type="radio" name="food_veget" id="radio3" value="radio3">
<label for="food_veget"></label>
มังสวิรัติ
<input type="radio" name="food_j" id="radio4" value="radio4">
<label for="food_j"></label>
เจ
<input type="radio" name="other" id="radio5" value="radio5">
<label for="other"></label>
อื่นๆ (โปรดระบุ)
<label for="food_other"></label>
<textarea name="food_other" cols="100" id="food_other"></textarea>
</p>
<p>6. ที่อยู่
:
<label for="address"></label>
<textarea name="address" cols="50" rows="2" id="address"></textarea>
7. สถานที่ทำงาน :
<label for="officer"></label>
<textarea name="officer" id="officer"></textarea>
</p>
<p>8. โทรศัพท์ (ที่ติดต่อได้สะดวก) :
<label for="textfield5"></label>
<input type="text" name="phone" id="textfield5">
9. E-mail :
<label for="email"></label>
<input name="email" type="text" id="email" size="50">
</p>
<p>
<center><input type="submit" name="confirm" id="confirm" value="ส่งข้อมูล"></center>
</p>
<input type="hidden" name="MM_insert" value="form1">
</form></td>
</tr>
<tr>
<td> </td>
</tr>
<tr>
<td> </td>
</tr>
</table>
</body>
</html>
Tag : PHP, MySQL
|
|
 |
 |
 |
 |
Date :
2015-06-15 15:54:03 |
By :
emopink009 |
View :
3499 |
Reply :
3 |
|
 |
 |
 |
 |
|
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ถ้าเว็บเดียวกันก็ Insert แลเงดึงมาว์ได้เลยครับ
PHP MySQL Add/Insert Record
|
 |
 |
 |
 |
Date :
2015-06-15 17:11:53 |
By :
mr.win |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ผิดพลาดตรงไหนหรอค่ะ ทำไมไม่โชว์ข้อมูล รบกวนด้วยคะ

Code (PHP)
<html>
<head>
<title>เช็ดรายละเอียด</title>
</head>
<body>
<?php
$objConnect = mysql_connect("localhost","root","1234") or die("Error Connect to Database");
$objDB = mysql_select_db("db_stou");
$strSQL = "INSERT INTO tb_accept_human";
$strSQL .="(code,list,student_id,name,lastname,version,accept,accept_other,food,other,food_other,address,officer,phone,email) ";
$strSQL .="VALUES ";
$strSQL .="('".$_POST["code"]."','".$_POST["list"]."','".$_POST["student_id"]."' ";
$strSQL .=",'".$_POST["name"]."','".$_POST["lastname"]."','".$_POST["version"]."') ";
$strSQL .="('".$_POST["accept"]."','".$_POST["accept_other"]."','".$_POST["food"]."' ";
$strSQL .="('".$_POST["food_other"]."','".$_POST["address"]."','".$_POST["officer"]."' ";
$strSQL .=",'".$_POST["phone"]."','".$_POST["email"]."') ";
$objQuery = mysql_query($strSQL);
if($objQuery)
{
echo "Save Done.";
}
else
{
echo "Error Save [".$strSQL."]";
}
mysql_close($objConnect);
?>
</body>
</html>
<?php
mysql_query("SET character_set_results=utf8");
mysql_query("SET character_set_client=utf8");
mysql_query("SET character_set_connection=utf8");
?>
|
 |
 |
 |
 |
Date :
2015-06-16 09:17:07 |
By :
emopink009 |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
Code (PHP)
|
 |
 |
 |
 |
Date :
2022-01-21 12:40:30 |
By :
กดอ |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
|
|