 |
ข้อมูลไม่ขึ้น Update ให้ค่ะ ตัวโค้ดผิดตรงไหนหรือป่าวค่ะรบกวนช่วยตรวจสอบด้วยค่ะ |
|
 |
|
|
 |
 |
|
Code (PHP)
<?php require_once('../Connections/MyConnec.php'); ?>
<?php
if (!function_exists("GetSQLValueString")) {
function GetSQLValueString($theValue, $theType, $theDefinedValue = "", $theNotDefinedValue = "")
{
if (PHP_VERSION < 6) {
$theValue = get_magic_quotes_gpc() ? stripslashes($theValue) : $theValue;
}
$theValue = function_exists("mysql_real_escape_string") ? mysql_real_escape_string($theValue) : mysql_escape_string($theValue);
switch ($theType) {
case "text":
$theValue = ($theValue != "") ? "'" . $theValue . "'" : "NULL";
break;
case "long":
case "int":
$theValue = ($theValue != "") ? intval($theValue) : "NULL";
break;
case "double":
$theValue = ($theValue != "") ? doubleval($theValue) : "NULL";
break;
case "date":
$theValue = ($theValue != "") ? "'" . $theValue . "'" : "NULL";
break;
case "defined":
$theValue = ($theValue != "") ? $theDefinedValue : $theNotDefinedValue;
break;
}
return $theValue;
}
}
$editFormAction = $_SERVER['PHP_SELF'];
if (isset($_SERVER['QUERY_STRING'])) {
$editFormAction .= "?" . htmlentities($_SERVER['QUERY_STRING']);
}
if ((isset($_POST["MM_update"])) && ($_POST["MM_update"] == "form2")) {
$updateSQL = sprintf("UPDATE subject SET Sub_id=%s, Sub_name=%s, group_id=%s",
GetSQLValueString($_POST['Sub_id'], "text"),
GetSQLValueString($_POST['Sub_name'], "text"),
GetSQLValueString($_POST['group_id'], "text"));
mysql_select_db($database_MyConnec, $MyConnec);
$Result1 = mysql_query($updateSQL, $MyConnec) or die(mysql_error());
$updateGoTo = "subject.php";
if (isset($_SERVER['QUERY_STRING'])) {
$updateGoTo .= (strpos($updateGoTo, '?')) ? "&" : "?";
$updateGoTo .= $_SERVER['QUERY_STRING'];
}
header(sprintf("Location: %s", $updateGoTo));
}
if ((isset($_POST["MM_update"])) && ($_POST["MM_update"] == "form2")) {
$updateSQL = sprintf("UPDATE subject SET Sub_id=%s, St_User=%s, Sub_name=%s, group_id=%s",
GetSQLValueString($_POST['Sub_id'], "text"),
GetSQLValueString($_POST['St_User'], "text"),
GetSQLValueString($_POST['Sub_name'], "text"));
mysql_select_db($database_MyConnec, $MyConnec);
$Result1 = mysql_query($updateSQL, $MyConnec) or die(mysql_error());
$updateGoTo = "subject.php";
if (isset($_SERVER['QUERY_STRING'])) {
$updateGoTo .= (strpos($updateGoTo, '?')) ? "&" : "?";
$updateGoTo .= $_SERVER['QUERY_STRING'];
}
header(sprintf("Location: %s", $updateGoTo));
}
$colname_Recordset1 = "-1";
if (isset($_GET['Sub_id'])) {
$colname_Recordset1 = $_GET['Sub_id'];
}
mysql_select_db($database_MyConnec, $MyConnec);
$query_Recordset1 = sprintf("SELECT * FROM subject WHERE Sub_id = %s", GetSQLValueString($colname_Recordset1, "int"));
$Recordset1 = mysql_query($query_Recordset1, $MyConnec) or die(mysql_error());
$row_Recordset1 = mysql_fetch_assoc($Recordset1);
$totalRows_Recordset1 = mysql_num_rows($Recordset1);
?>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<title>Untitled Document</title>
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.1.1/jquery.min.js"></script>
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/js/bootstrap.min.js"></script>
</head>
<body>
<nav class="navbar navbar-inverse">
<div class="container-fluid">
<div class="navbar-header">
<a class="navbar-brand" href="for_admin.php">รายชื่อวิชา</a>
</div>
<ul class="nav navbar-nav">
<li class="active"><a href="for_admin.php">Home</a></li>
<li><a href="Student_Information.php">ผู้ใช้งาน</a></li>
<li><a href="subject.php">รายวิชา</a></li>
<li><a href="report.php">ออกรายงาน</a></li>
</ul>
<ul class="nav navbar-nav navbar-right">
<li><a href="index.php"><span class="glyphicon glyphicon-log-in"></span> Logout</a></li>
</ul>
</div>
</nav>
<p> </p>
<div class="row">
<div class="col-sm-4"></div>
<div class="row">
<div class="col-xs-4">
</div>
</div>
</div>
<form action="<?php echo $editFormAction; ?>" method="post" name="form2" id="form2">
<table align="center">
<tr valign="baseline">
<td nowrap="nowrap" align="right">ลำดับ ::</td>
<td><input type="text" name="Sub_id" class="form-control" value="<?php echo htmlentities($row_Recordset1['Sub_id'], ENT_COMPAT, 'utf-8'); ?>" size="32" /></td>
</tr>
<tr valign="baseline">
<td nowrap="nowrap" align="right">ชื่อวิชา ::</td>
<td><input type="text" name="Sub_name" class="form-control" value="<?php echo htmlentities($row_Recordset1['Sub_name'], ENT_COMPAT, 'utf-8'); ?>" size="32" /></td>
</tr>
<tr valign="baseline">
<td nowrap="nowrap" align="right">ระดับสมรรถนะ ::</td>
<td><input type="text" name="group_id" class="form-control" value="<?php echo htmlentities($row_Recordset1['group_id'], ENT_COMPAT, 'utf-8'); ?>" size="32" /></td>
</tr>
<tr valign="baseline">
<td nowrap="nowrap" align="right"> </td>
<td><br><div align="center">
<input type="submit" class="btn btn-success" value="ตกลง" />
</div></td>
</tr>
</table>
<input type="hidden" name="MM_update" value="form2" />
<input type="hidden" name="Sub_id" value="<?php echo $row_Recordset1['Sub_id']; ?>" />
</form>
<p> </p>
</body>
</html>
<?php
mysql_free_result($Recordset1);
?>
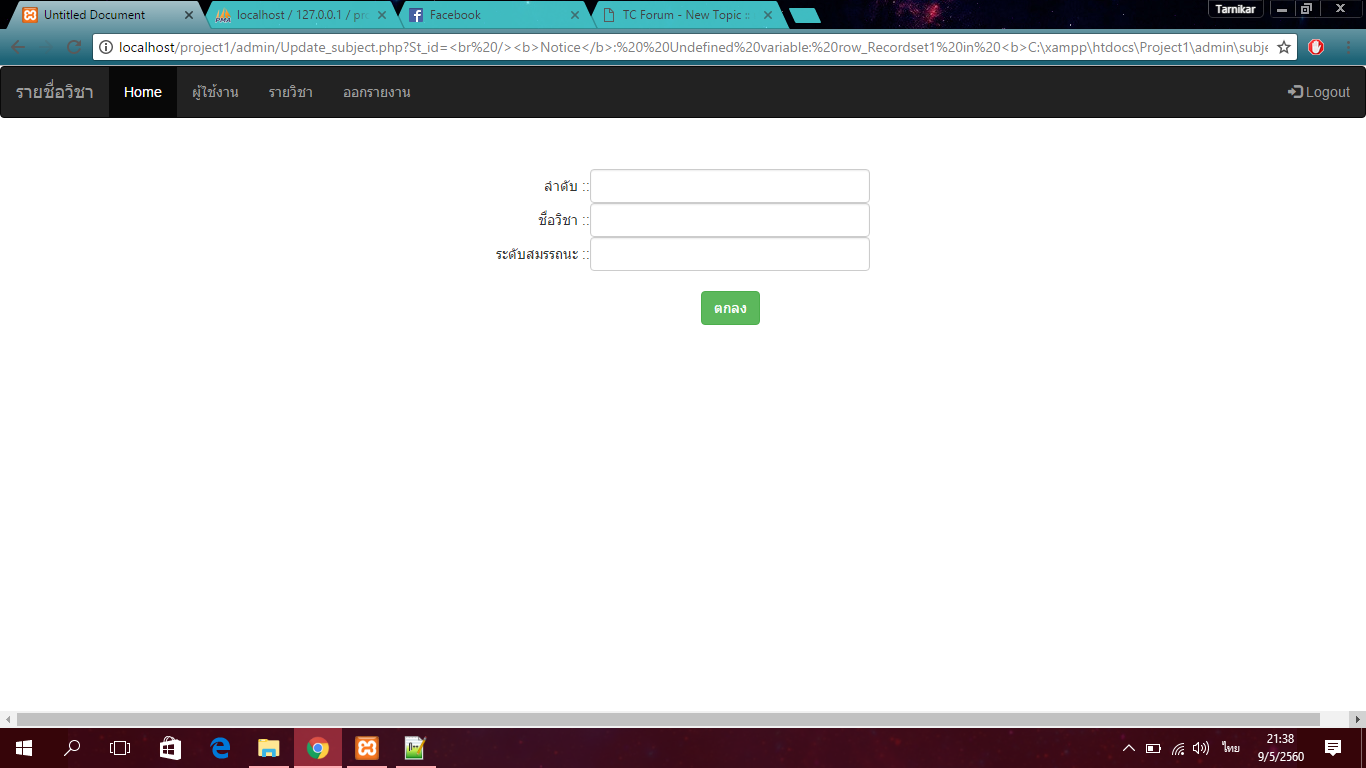
Tag : PHP, MySQL
|
|
 |
 |
 |
 |
Date :
2017-05-09 21:42:12 |
By :
Tarnikar |
View :
782 |
Reply :
3 |
|
 |
 |
 |
 |
|
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
Code (PHP)
$updateSQL = sprintf("UPDATE subject SET Sub_id=%s, St_User=%s, Sub_name=%s, group_id=%s",
GetSQLValueString($_POST['Sub_id'], "text"),
GetSQLValueString($_POST['St_User'], "text"),
GetSQLValueString($_POST['Sub_name'], "text"));
%s มี 4 ตัวแต่ parameter มีแค่ 3 ครับ
|
 |
 |
 |
 |
Date :
2017-05-09 21:54:43 |
By :
num |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|

|
 |
 |
 |
 |
Date :
2017-05-11 09:42:11 |
By :
mr.win |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
แก้ไขแล้วค่ะ แต่ก็ข้อมูลก็ยังไม่ขึ้นให้แก้ไขอีกค่ะ
Code (PHP)
<?php require_once('../Connections/MyConnec.php'); ?>
<?php
if (!function_exists("GetSQLValueString")) {
function GetSQLValueString($theValue, $theType, $theDefinedValue = "", $theNotDefinedValue = "")
{
if (PHP_VERSION < 6) {
$theValue = get_magic_quotes_gpc() ? stripslashes($theValue) : $theValue;
}
$theValue = function_exists("mysql_real_escape_string") ? mysql_real_escape_string($theValue) : mysql_escape_string($theValue);
switch ($theType) {
case "text":
$theValue = ($theValue != "") ? "'" . $theValue . "'" : "NULL";
break;
case "long":
case "int":
$theValue = ($theValue != "") ? intval($theValue) : "NULL";
break;
case "double":
$theValue = ($theValue != "") ? doubleval($theValue) : "NULL";
break;
case "date":
$theValue = ($theValue != "") ? "'" . $theValue . "'" : "NULL";
break;
case "defined":
$theValue = ($theValue != "") ? $theDefinedValue : $theNotDefinedValue;
break;
}
return $theValue;
}
}
$editFormAction = $_SERVER['PHP_SELF'];
if (isset($_SERVER['QUERY_STRING'])) {
$editFormAction .= "?" . htmlentities($_SERVER['QUERY_STRING']);
}
if ((isset($_POST["MM_update"])) && ($_POST["MM_update"] == "form2")) {
$updateSQL = sprintf("UPDATE subject SET Sub_id=%s, Sub_name=%s, group_id=%s",
GetSQLValueString($_POST['Sub_id'], "text"),
GetSQLValueString($_POST['Sub_name'], "text"),
GetSQLValueString($_POST['group_id'], "text"));
mysql_select_db($database_MyConnec, $MyConnec);
$Result1 = mysql_query($updateSQL, $MyConnec) or die(mysql_error());
$updateGoTo = "subject.php";
if (isset($_SERVER['QUERY_STRING'])) {
$updateGoTo .= (strpos($updateGoTo, '?')) ? "&" : "?";
$updateGoTo .= $_SERVER['QUERY_STRING'];
}
header(sprintf("Location: %s", $updateGoTo));
}
if ((isset($_POST["MM_update"])) && ($_POST["MM_update"] == "form2")) {
$updateSQL = sprintf("UPDATE subject SET Sub_id=%s, Sub_name=%s, group_id=%s",
GetSQLValueString($_POST['Sub_id'], "text"),
GetSQLValueString($_POST['Sub_name'], "text"),
GetSQLValueString($_POST['group_id'], "text"));
mysql_select_db($database_MyConnec, $MyConnec);
$Result1 = mysql_query($updateSQL, $MyConnec) or die(mysql_error());
$updateGoTo = "subject.php";
if (isset($_SERVER['QUERY_STRING'])) {
$updateGoTo .= (strpos($updateGoTo, '?')) ? "&" : "?";
$updateGoTo .= $_SERVER['QUERY_STRING'];
}
header(sprintf("Location: %s", $updateGoTo));
}
$colname_Recordset1 = "-1";
if (isset($_GET['Sub_id'])) {
$colname_Recordset1 = $_GET['Sub_id'];
}
mysql_select_db($database_MyConnec, $MyConnec);
$query_Recordset1 = sprintf("SELECT * FROM subject WHERE Sub_id = %s", GetSQLValueString($colname_Recordset1, "int"));
$Recordset1 = mysql_query($query_Recordset1, $MyConnec) or die(mysql_error());
$row_Recordset1 = mysql_fetch_assoc($Recordset1);
$totalRows_Recordset1 = mysql_num_rows($Recordset1);
?>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<title>Untitled Document</title>
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.1.1/jquery.min.js"></script>
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/js/bootstrap.min.js"></script>
</head>
<body>
<nav class="navbar navbar-inverse">
<div class="container-fluid">
<div class="navbar-header">
<a class="navbar-brand" href="for_admin.php">รายชื่อวิชา</a>
</div>
<ul class="nav navbar-nav">
<li class="active"><a href="for_admin.php">Home</a></li>
<li><a href="Student_Information.php">ผู้ใช้งาน</a></li>
<li><a href="subject.php">รายวิชา</a></li>
<li><a href="report.php">ออกรายงาน</a></li>
</ul>
<ul class="nav navbar-nav navbar-right">
<li><a href="index.php"><span class="glyphicon glyphicon-log-in"></span> Logout</a></li>
</ul>
</div>
</nav>
<p> </p>
<div class="row">
<div class="col-sm-4"></div>
<div class="row">
<div class="col-xs-4">
</div>
</div>
</div>
<form action="<?php echo $editFormAction; ?>" method="post" name="form2" id="form2">
<table align="center">
<tr valign="baseline">
<td nowrap="nowrap" align="right">ลำดับ ::</td>
<td><input type="text" name="Sub_id" class="form-control" value="<?php echo htmlentities($row_Recordset1['Sub_id'], ENT_COMPAT, 'utf-8'); ?>" size="32" /></td>
</tr>
<tr valign="baseline">
<td nowrap="nowrap" align="right">ชื่อวิชา ::</td>
<td><input type="text" name="Sub_name" class="form-control" value="<?php echo htmlentities($row_Recordset1['Sub_name'], ENT_COMPAT, 'utf-8'); ?>" size="32" /></td>
</tr>
<tr valign="baseline">
<td nowrap="nowrap" align="right">ระดับสมรรถนะ ::</td>
<td><input type="text" name="group_id" class="form-control" value="<?php echo htmlentities($row_Recordset1['group_id'], ENT_COMPAT, 'utf-8'); ?>" size="32" /></td>
</tr>
<tr valign="baseline">
<td nowrap="nowrap" align="right"> </td>
<td><br><div align="center">
<input type="submit" class="btn btn-success" value="ตกลง" />
</div></td>
</tr>
</table>
<input type="hidden" name="MM_update" value="form2" />
<input type="hidden" name="Sub_id" value="<?php echo $row_Recordset1['Sub_id']; ?>" />
</form>
<p> </p>
</body>
</html>
<?php
mysql_free_result($Recordset1);
?>
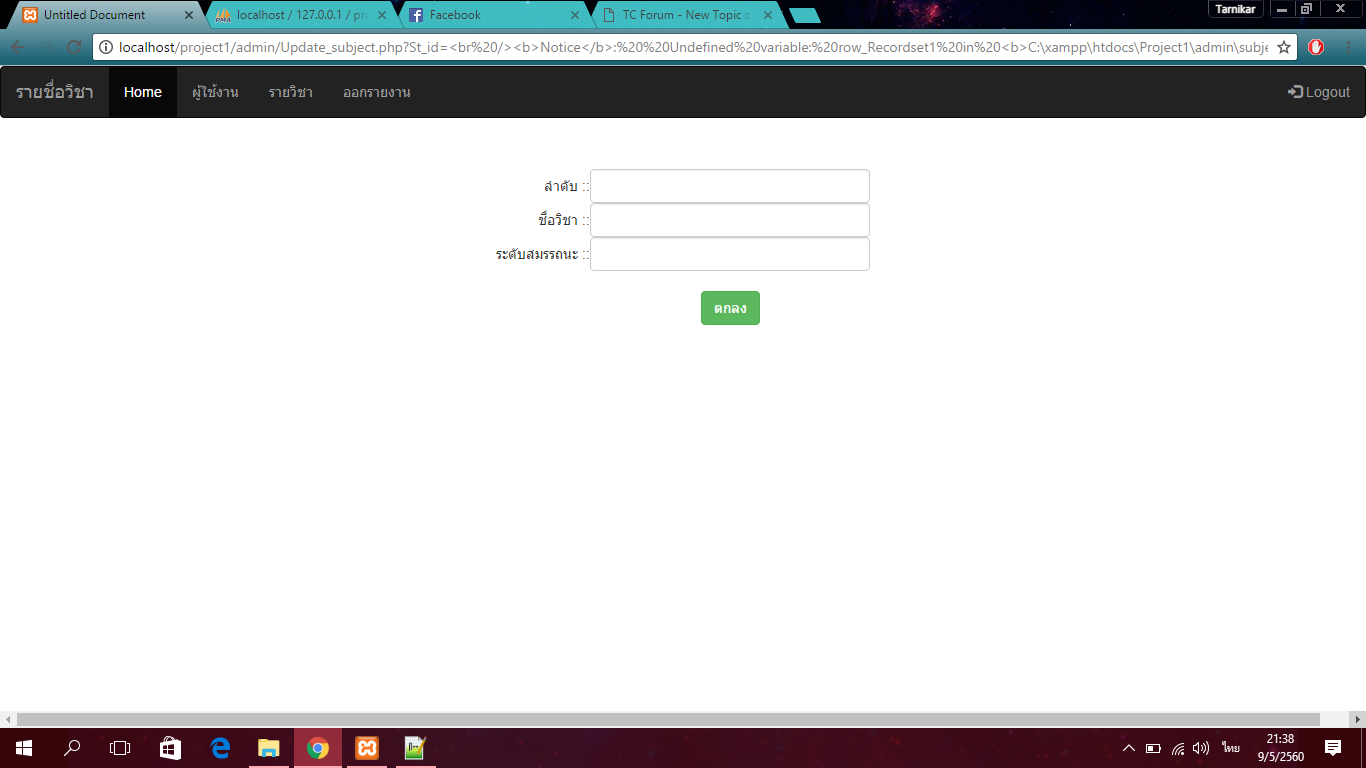
|
 |
 |
 |
 |
Date :
2017-05-16 09:24:39 |
By :
Tarnikar |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
|
|