 |
PHP+Ajax+SQLServer+Bootstrap+Modal เจอปัญหาค่าที่ส่งไป Modal ได้แค่ Column สุดท้ายครับ..Column คลิกแล้วไม่มีอะไรเกิดขึ้น |
|
 |
|
|
 |
 |
|
เจอปัญหาค่าที่ส่งไป Modal ไปแค่ Column สุดท้ายครับ
ตามภาพ...กดปุ่มเพิ่มการลา..Modal จะทำงานแค่ชื่อสุดท้ายครับ..คนอื่นคลิกแล้วไม่มีอะไรเกิดขึ้น
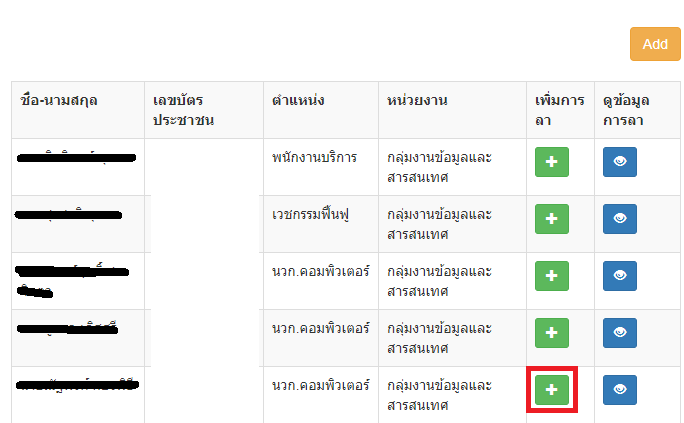
Code (PHP)
<?php
include "connection.php";
$query = "SELECT user_serial,user_bz,user_lname,user_depname,user_id FROM dt_user WHERE dep_no='0010401' and user_type='0'";
$result = sqlsrv_query($conn, $query);
?>
<!DOCTYPE html>
<html>
<head>
<title><?=$title;?></title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.2.0/jquery.min.js"></script>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.6/css/bootstrap.min.css" />
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.6/js/bootstrap.min.js"></script>
</head>
<body>
<br /><br />
<div class="container" style="width:700px;">
<br />
<div class="table-responsive">
<div align="right">
<button type="button" name="add" id="add" data-toggle="modal" data-target="#add_data_Modal" class="btn btn-warning">Add</button>
</div>
<br />
<div id="employee_table">
<table class="table table-bordered table-striped">
<tr>
<th >ชื่อ-นามสกุล</th>
<th >เลขบัตรประชาชน</th>
<th >ตำแหน่ง</th>
<th >หน่วยงาน</th>
<th >เพิ่มการลา</th>
<th >ดูข้อมูลการลา</th>
</tr>
<?php
while($row = sqlsrv_fetch_array($result))
{
?>
<tr>
<td><?php echo $row["user_lname"]; ?></td>
<td><?php echo $row["user_id"]; ?></td>
<td><?php echo $row["user_bz"]; ?></td>
<td><?php echo $row["user_depname"]; ?></td>
<td><button type="button" name="edit" id="<?php echo $row["user_serial"]; ?>" class="btn btn-success btn-sm edit_data" /><span class='glyphicon glyphicon-plus' aria-hidden='true'></span></button></td>
<td><button type="button" name="view" id="<?php echo $row["user_id"]; ?>" class="btn btn-primary btn-sm view_data" /><span class='glyphicon glyphicon-eye-open' aria-hidden='true'></span></button></td>
</tr>
<?php
}
?>
</table>
</div>
</div>
</div>
</body>
</html>
<div id="dataModal" class="modal fade">
<div class="modal-dialog">
<div class="modal-content">
<div class="modal-header">
<button type="button" class="close" data-dismiss="modal">×</button>
<h4 class="modal-title">รายละเอียดการลา</h4>
</div>
<div class="modal-body" id="employee_detail">
</div>
<div class="modal-footer">
<button type="button" class="btn btn-default" data-dismiss="modal">ปิด</button>
</div>
</div>
</div>
</div>
<div id="add_data_Modal" class="modal fade">
<div class="modal-dialog">
<div class="modal-content">
<div class="modal-header">
<button type="button" class="close" data-dismiss="modal">×</button>
<h4 class="modal-title">เพิ่ม</h4>
</div>
<div class="modal-body">
<form method="post" id="insert_form">
<label>Enter Employee Name</label>
<input type="text" name="name" id="name" class="form-control" />
<br />
<label>Enter Employee Address</label>
<textarea name="address" id="address" class="form-control"></textarea>
<br />
<label>Select Gender</label>
<select name="gender" id="gender" class="form-control">
<option value="Male">Male</option>
<option value="Female">Female</option>
</select>
<br />
<label>Enter Designation</label>
<input type="text" name="designation" id="designation" class="form-control" />
<br />
<label>Enter Age</label>
<input type="text" name="age" id="age" class="form-control" />
<br />
<input type="hidden" name="employee_id" id="employee_id" />
<input type="submit" name="insert" id="insert" value="Insert" class="btn btn-success" />
</form>
</div>
<div class="modal-footer">
<button type="button" class="btn btn-default" data-dismiss="modal">Close</button>
</div>
</div>
</div>
</div>
<script>
$(document).ready(function(){
$('#add').click(function(){
$('#insert').val("Insert");
$('#insert_form')[0].reset();
});
$(document).on('click', '.edit_data', function(){
var user_serial = $(this).attr("id");
$.ajax({
url:"fetch.php",
method:"POST",
data:{user_serial:user_serial},
dataType:"json",
success:function(data){
//alert(data);
$('#name').val(data.user_lname);
$('#address').val(data.user_id);
$('#gender').val(data.gender);
$('#designation').val(data.user_depname);
$('#age').val(data.user_bz);
$('#employee_id').val(data.user_serial);
$('#insert').val("เพิ่มการลา");
$('#add_data_Modal').modal('show');
}
});
});
$('#insert_form').on("submit", function(event){
event.preventDefault();
if($('#name').val() == "")
{
alert("Name is required");
}
else if($('#address').val() == '')
{
alert("Address is required");
}
else if($('#designation').val() == '')
{
alert("Designation is required");
}
else if($('#age').val() == '')
{
alert("Age is required");
}
else
{
$.ajax({
url:"insert.php",
method:"POST",
data:$('#insert_form').serialize(),
beforeSend:function(){
$('#insert').val("Inserting");
},
success:function(data){
$('#insert_form')[0].reset();
$('#add_data_Modal').modal('hide');
$('#employee_table').html(data);
}
});
}
});
$(document).on('click', '.view_data', function(){
var user_id = $(this).attr("id");
if(user_id != '')
{
$.ajax({
url:"select.php",
method:"POST",
data:{user_id:user_id},
success:function(data){
$('#employee_detail').html(data);
$('#dataModal').modal('show');
}
});
}
});
});
</script>
Code (PHP)
<?php
//fetch.php
include "connection.php";
if(isset($_POST["user_serial"]))
{
$query = "SELECT * FROM dt_user WHERE user_serial = '".$_POST["user_serial"]."'";
$result = sqlsrv_query($conn, $query);
$row = sqlsrv_fetch_array($result);
echo json_encode($row);
}
?>
Tag : PHP, Ms SQL Server 2008, Ajax, Appserv
|
ประวัติการแก้ไข 2017-10-17 17:20:38
|
 |
 |
 |
 |
Date :
2017-10-17 15:23:45 |
By :
binzaa_cs |
View :
4928 |
Reply :
4 |
|
 |
 |
 |
 |
|
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ส่งข้อมูลออกมาใส่ array แล้วส่งโดย json ก่อนไหม
while(//ตรงนี้ผมไม่รู้ว่า sqlv while ค่าแบบไหน){
$json_data[]=array(
"id"=>$row->id,
"title"=>$row->name,
"start"=>$row->timego,
"end"=>$row->timeback,
);
}
$json= json_encode($json_data);
ซึ่งตรง sqlv ผมไม่รู้ว่าสั่งให้มันส่งค่าแบบไหนครับ
หาอ่านมา คงต้อง while ค่าออกมาก่อนครับ
while( $row = sqlsrv_fetch_array( $stmt, SQLSRV_FETCH_ASSOC) ) {
$json_data[]=array(
"LastName"= $row['LastName'],
"FirstName" =$row['FirstName'],
);
}
$json= json_encode($json_data);
|
ประวัติการแก้ไข 2017-10-18 16:13:34 2017-10-18 16:19:16
 |
 |
 |
 |
Date :
2017-10-18 16:07:16 |
By :
apisitp |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
|
|