 |
สอบถามถามการ sum ยอดสินค้าที่ขายออกหน่อยครับ ขอรบกวนด้วยครับ |
|
 |
|
|
 |
 |
|
ศึ่กษาคำสั่ง sum , group by ของ sql statement
|
 |
 |
 |
 |
Date :
2019-02-14 08:00:45 |
By :
Chaidhanan |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ตอบความคิดเห็นที่ : 1 เขียนโดย : Chaidhanan เมื่อวันที่ 2019-02-14 08:00:45
รายละเอียดของการตอบ ::
ผมลองทดสอบดูแล้วคับ แต่เนื่องจากโจทย์ของผมคือแยกราคาตามลูกค้า ผมจึงกำหนด Type เพิ่มว่าตอนบันทึกว่ามี Type อะไรบ้าง
ผลออกมาก็ได้ตามต้องการ
แต่ก็ยังมิวายมีปัญหาเกิดขึ้นอีกคับ คือว่า Type ที่ไว้เช็คค่า เวลาผมบันทึกเสร็จก็จะขายสินค้าใหม่แต่ครั้งนี้ค่า Type ไม่บันทึกให้คับยกเว้นผมจะ login เข้ามาแล้วขายใหม่ Type จึงจะบันทึก ผมปริ้นเช็ดก่อนบันทึกทุกรอบค่าก็ส่งถูกส่งมาแต่มันไม่บันทึก ให้คับ
ตอนส่งค่าค่า
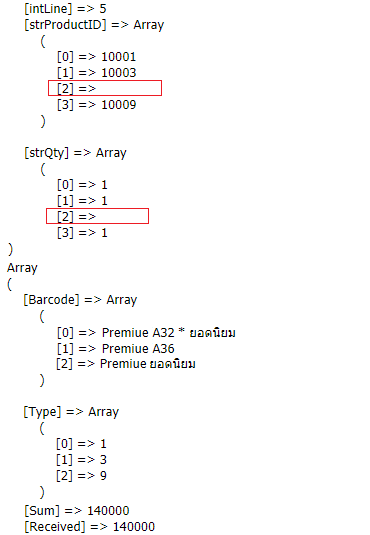
หน้ารับคับ
Code (PHP)
<?php
session_start();
$serverName = "localhost";
$userName = "root";
$userPassword = "12345678";
$dbName = "mydatabase";
$conn = mysqli_connect($serverName,$userName,$userPassword,$dbName);
if (!$conn) {
echo $conn->connect_error;
exit();
}
/*
echo "<pre>";
print_r($_SESSION);
echo "</pre>";
echo "<br/>";
echo "<pre>";
print_r($_POST);
echo "</pre>";
exit();
*/
$Subtotal = $_POST["Subtotal"];
$Balance = $_POST["SumTotal"];
$Received = $_POST["Received"];
$Refund = $_POST["Refund"];
$Credit = $_POST["Credit"];
$Save = $_POST["m_name"];
$Type = $_POST["Type"];
$strSQL = "
INSERT INTO orders (OrderDate,Balance,Received,Refund,Credit,Save)
VALUES
('".date("Y-m-d H:i:s")."','".$_POST["Balance"]."' ,'".$_POST["Received"]."','".$_POST["Refund"]."','".$_POST["Credit"]."','".$_POST["Save"]."' )
";
$objQuery = mysqli_query($conn,$strSQL);
if(!$objQuery)
{
echo $conn->error;
exit();
}
$strOrderID = mysqli_insert_id($conn);
for($i=0;$i<=(int)$_SESSION["intLine"];$i++)
{
if($_SESSION["strProductID"][$i] != "")
{
$strSQL = "
INSERT INTO orders_detail (OrderID,ProductID,Barcode,Qty,Type)
VALUES
('".$strOrderID."','".$_SESSION["strProductID"][$i]."','".$_SESSION["Barcode"][$i]."','".$_SESSION["strQty"][$i]."','".$_POST["Type"][$i]."')";
$result = mysqli_query($conn,$strSQL);
if(!$result)
{
echo $conn->error;
exit();
}
else
{
$update = "UPDATE producttestqty SET Qty = Qty-'".$_SESSION["strQty"][$i]."' WHERE Barcode = '".$_SESSION["Barcode"][$i]."' ";
mysqli_query($conn,$update) or die(mysqli_error($conn));
if(!$update){
echo $conn->error;
exit();
}
}
}
}
mysqli_close($conn);
unset($_SESSION['strProductID']);
unset($_SESSION['strQty']);
header("location:cusshoworder.php?OrderID=".$strOrderID);
?>
|
 |
 |
 |
 |
Date :
2019-02-14 12:18:08 |
By :
HLEW |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
แล้วอีกปัญหาที่เจอคือ เวลาลบสินค้าก็ยังมีค่าว่างส่งมาด้วย ทำให้การค่า Type บางรายการบันทึกข้าเป็น 0
จึงเช็คค่า Type เป็นสินค้าตัวไหน
Code (PHP)
//ส่งค่า
<a href="customerdelete.php?Line=<?=$i;?>">del</a>
//รับค่ามาลบ
<?php
ob_start();
session_start();
if(isset($_GET["Line"]))
{
$Line = $_GET["Line"];
$_SESSION["strProductID"][$Line] = "";
$_SESSION["strQty"][$Line] = "";
}
header("location:customerconfirm.php");
?>
|
 |
 |
 |
 |
Date :
2019-02-14 12:26:11 |
By :
HLEW |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ตรวจสอบค่า $_POST มาถูกต้องไหม ตอนส่งมาใช้ตัวแปรถูกหรือไม่
การเขียนโค๊ดคิวรี่ ให้คำนึงถีง sql injection ไว้ด้วย ใช้คำสัง prepare ดีกว่า
|
 |
 |
 |
 |
Date :
2019-02-14 12:29:59 |
By :
Chaidhanan |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ตรงไหนคือคำสั่งเก็บค่า type ครับ
|
 |
 |
 |
 |
Date :
2019-02-14 13:19:42 |
By :
Chaidhanan |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ตอบความคิดเห็นที่ : 6 เขียนโดย : Chaidhanan เมื่อวันที่ 2019-02-14 13:19:42
รายละเอียดของการตอบ ::
ส่งมาจากหน้านี้คับ
Code (PHP)
<?php
session_start();
if(!isset($_SESSION["intLine"]))
{
echo "ยังไม่มีรายการสั่งซื้อ";
exit();
}
$serverName = "localhost";
$userName = "root";
$userPassword = "12345678";
$dbName = "mydatabase";
$objCon = mysqli_connect($serverName,$userName,$userPassword,$dbName);
if (!$objCon) {
echo $objCon->connect_error;
exit();
}
?>
<?php
$ProductID = '';
if( isset( $_GET['strProductID'])) {
$ProductID = $_GET['strProductID'];
}
$i = 0;
$Credit = 0;
$Total = 0;
$SumTotal = 0;
for($i=0;$i<=(int)$_SESSION["intLine"];$i++)
{
if($_SESSION["strProductID"][$i] != "")
{
$strSQL = "SELECT * FROM product_type WHERE ProductID = '".$_SESSION["strProductID"][$i]."' ";
$objQuery = mysqli_query($objCon,$strSQL);
$objResult = $objResult = mysqli_fetch_array($objQuery,MYSQLI_ASSOC);
$Total = $_SESSION["strQty"][$i] * $objResult["Price"];
$SumTotal = $SumTotal + $Total;
$Credit = $Credit + $Total;
?>
<tr>
<td><?=$objResult["Barcode"];?><input type="hidden" name="Barcode[]" id="Barcode" value="<?=$objResult["ProductName"];?>"></td>
<td><?=$objResult["ProductName"];?><input type="hidden" name="Type[]" id="Type" value="<?=$objResult["Type"];?>"></td>
<td><?=$objResult["Price"];?></td>
<td><?=$_SESSION["strQty"][$i];?></td>
<td><?=number_format($Total,2);?></td>
</tr>
</tbody>
<?php
}
}
?>
หน้านี้หน้ารับครับ
Code (PHP)
<?php
session_start();
$serverName = "localhost";
$userName = "root";
$userPassword = "12345678";
$dbName = "mydatabase";
$conn = mysqli_connect($serverName,$userName,$userPassword,$dbName);
if (!$conn) {
echo $conn->connect_error;
exit();
}
/*
echo "<pre>";
print_r($_SESSION);
echo "</pre>";
echo "<br/>";
echo "<pre>";
print_r($_POST);
echo "</pre>";
exit();
*/
$Subtotal = $_POST["Subtotal"];
$Balance = $_POST["SumTotal"];
$Received = $_POST["Received"];
$Refund = $_POST["Refund"];
$Credit = $_POST["Credit"];
$Save = $_POST["m_name"];
$Type = $_POST["Type"];
$strSQL = "
INSERT INTO orders (OrderDate,Balance,Received,Refund,Credit,Save)
VALUES
('".date("Y-m-d H:i:s")."','".$_POST["Balance"]."' ,'".$_POST["Received"]."','".$_POST["Refund"]."','".$_POST["Credit"]."','".$_POST["Save"]."' )
";
$objQuery = mysqli_query($conn,$strSQL);
if(!$objQuery)
{
echo $conn->error;
exit();
}
$strOrderID = mysqli_insert_id($conn);
for($i=0;$i<=(int)$_SESSION["intLine"];$i++)
{
if($_SESSION["strProductID"][$i] != "")
{
$strSQL = "
INSERT INTO orders_detail (OrderID,ProductID,Barcode,Qty,Type)
VALUES
('".$strOrderID."','".$_SESSION["strProductID"][$i]."','".$_SESSION["Barcode"][$i]."','".$_SESSION["strQty"][$i]."','".$_POST["Type"][$i]."')";
$result = mysqli_query($conn,$strSQL);
if(!$result)
{
echo $conn->error;
exit();
}
else
{
$update = "UPDATE producttestqty SET Qty = Qty-'".$_SESSION["strQty"][$i]."' WHERE Barcode = '".$_SESSION["Barcode"][$i]."' ";
mysqli_query($conn,$update) or die(mysqli_error($conn));
if(!$update){
echo $conn->error;
exit();
}
}
}
}
mysqli_close($conn);
unset($_SESSION['strProductID']);
unset($_SESSION['strQty']);
header("location:cusshoworder.php?OrderID=".$strOrderID);
?>
อันนี้รูปที่บันทึกแต่ละครั้งครับ
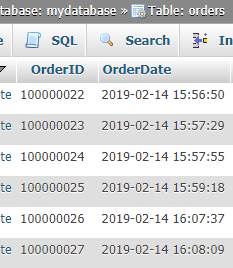
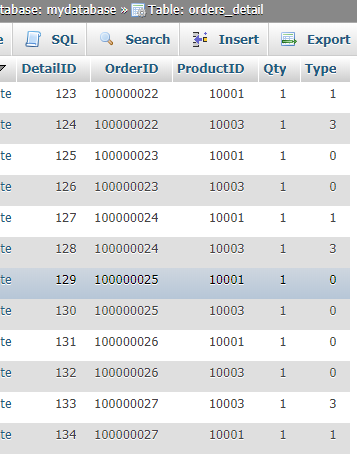
|
 |
 |
 |
 |
Date :
2019-02-14 16:11:28 |
By :
HLEW |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ในหน้ารับตั้งแต่บรรทัดที่ 45 - 54 คับวนลูปตามจำนวนที่ส่งมา
ผมลืมบอกคับตารางสินค้าผมเก็บข้อมูลแบบนี้คับ

|
ประวัติการแก้ไข 2019-02-14 16:28:03
 |
 |
 |
 |
Date :
2019-02-14 16:22:13 |
By :
HLEW |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
$Type = $_POST["Type"]; // ตอนรับค่า
// ตอน insert
$strSQL = "INSERT INTO orders_detail (OrderID,ProductID,Barcode,Qty,Type) VALUES
('".$strOrderID."','".$_SESSION["strProductID"][$i]."','".$_SESSION["Barcode"][$i]."','".$_SESSION["strQty"][$i]."','".$_POST["Type"][$i]."')";
แน่จะนะครับว่า ตอน รับค่า type มาเป็น array
|
 |
 |
 |
 |
Date :
2019-02-14 17:06:58 |
By :
Chaidhanan |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
บันทัดที่ 31 ตัวรับ
$Type = $_POST["Type"];
print_r($Type); รองตรวจสอบดูหน่อยครับ จากโค๊ดก็ไม่น่าจะผิด ควรได้รับค่าแล้ว
|
 |
 |
 |
 |
Date :
2019-02-15 09:53:10 |
By :
Chaidhanan |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ลองวิเคราะห์ index key ซักหน่อยครับ product_id มันเป็น 8 9 10 11
แต่ barcode กับ type มันเป็น 0 1 2 3 มันคงไม่แมทกัน หรือเปล่า
|
 |
 |
 |
 |
Date :
2019-02-16 08:39:38 |
By :
Chaidhanan |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
|
|