 |
|
คือผมอยากตัดส่วนที่มันเเสดงออกมาในรายงานที่ผมวงสีแดงไว้ ถ้าไม่อยากให้มันเเสดงต้องแก้โค้ดยังไงครับ
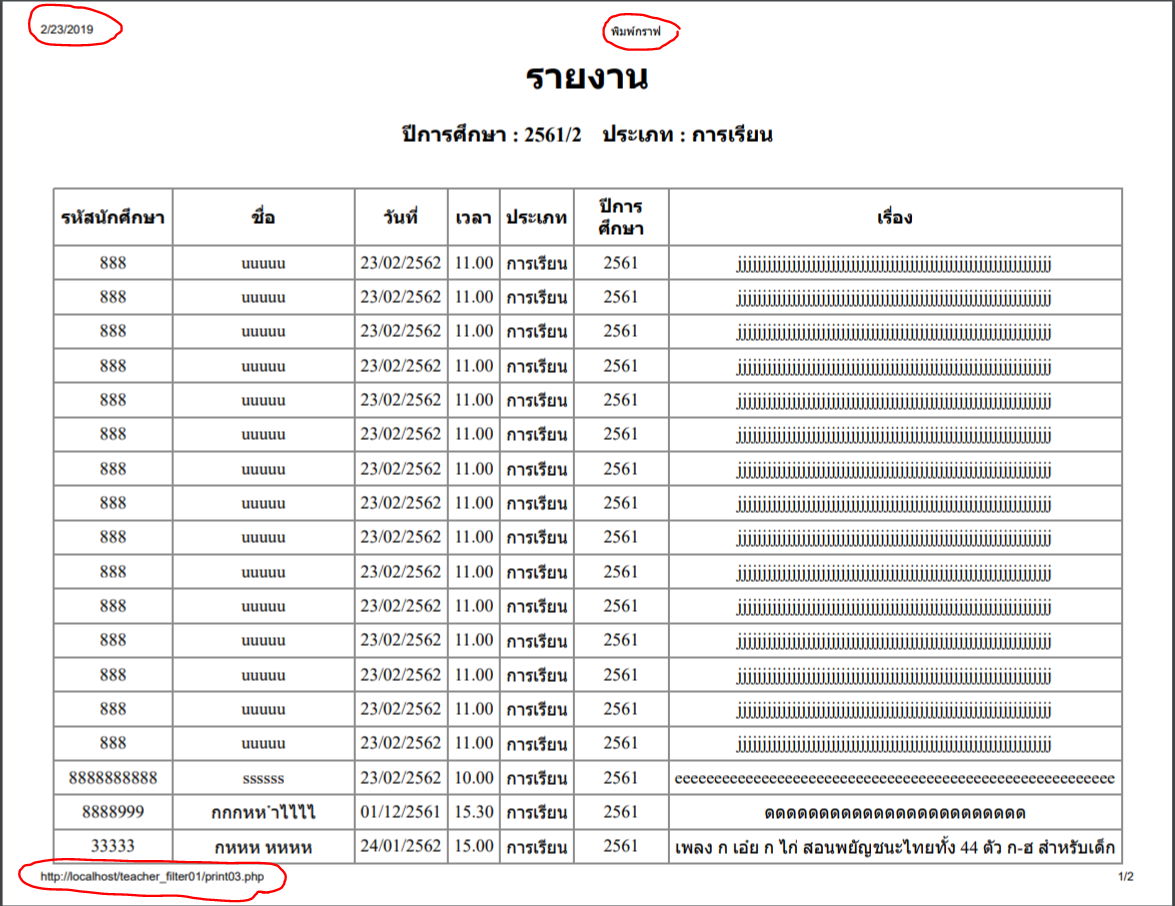
Code (PHP)
<?php
include('database_connection.php');
list($year, $term) = explode("/",$_POST["filter_year"]);
function show_date($tmp) {
if($tmp!="0000-00-00") {
list($date,$time) = explode(" ",$tmp);
list($y,$m,$d) = explode("-",$date);
$y += 543;
return $d."/".$m."/".$y;
}
}
?>
<html>
<head>
<title>พิมพ์กราฟ</title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.2.0/jquery.min.js"></script>
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.6/js/bootstrap.min.js"></script>
<script src="js/Chart.bundle.js"></script>
<style type="text/css" media="print">
@page { size: landscape; }
@media print
{
.no-print, .no-print *
{
display: none !important;
}
}
</style>
</head>
<body>
<div class=" box">
<h1 align="center">สรุปรายงานนัดหมายอาจารย์ที่ปรึกษา</h1>
<h3 align="center">ปีการศึกษา : <?php print ($term)?>/<?php print ($year+543)?> ประเภท : <?php print $_POST["filter_category"]?></h3>
<br />
<?php if($_POST["print"]!="") { ?>
<style>
table {
border-collapse: collapse;
}
table,th,tr,td {
border: 1px solid #8b8b8b;
}
</style>
<div class="table-responsive">
<table border="1" cellpadding="5" width="100%">
<thead>
<tr align="center">
<th>รหัสนักศึกษา</th>
<th>ชื่อ</th>
<th>วันที่</th>
<th>เวลา</th>
<th>ประเภท</th>
<th>ปีการศึกษา</th>
<th>เรื่อง</th>
</tr>
</thead>
<tbody>
<?php
$column = array('student_number', 'student_name', 'app_date', 'time_id', 'category_id', 'app_year', 'app_message');
$query = "SELECT * FROM appointment ";
if(isset($_POST['filter_year'], $_POST['filter_category']) && $_POST['filter_year'] != '' && $_POST['filter_category'] != '')
{
list($year, $term) = explode("/",$_POST['filter_year']);
if($term=="1") {
$fyear = " app_date between '".$year."-06-15' and '".$year."-10-24' ";
} elseif($term=="2") {
$fyear = " app_date between '".$year."-11-19' and '".($year+1)."-03-17' ";
} else {
$fyear = " app_date between '".($year+1)."-04-08' and '".($year+1)."-06-09' ";
}
# $query .= ' WHERE app_year = "'.$_POST['filter_year'].'" AND category_id = "'.$_POST['filter_category'].'" ';
$query .= ' WHERE '.$fyear.' AND category_id = "'.$_POST['filter_category'].'" ';
}
if(isset($_POST['order']))
{
$query .= 'ORDER BY '.$column[$_POST['order']['0']['column']].' '.$_POST['order']['0']['dir'].' ';
}
else
{
$query .= 'ORDER BY appstudent_id DESC ';
}
$statement = $connect->prepare($query);
$statement->execute();
$result = $statement->fetchAll();
$data = array();
foreach($result as $row)
{
?>
<tr align="center">
<td><?php print $row["student_number"]?></td>
<td><?php print $row["student_name"]?></td>
<td><?php print show_date($row['app_date'])?></td>
<td><?php print $row["time_id"]?></td>
<td><?php print $row["category_id"]?></td>
<td><?php print $row["app_year"]?></td>
<td><?php print $row["app_message"]?></td>
</tr>
<?php } ?>
<tbody>
</table>
<br />
<br />
<br />
</div>
<?php } else { ?>
<?php
$query = "SELECT month(app_date) as app_month, year(app_date) as app_year, count( appstudent_id) as qty FROM appointment ";
if($term=="1") {
$fyear = " app_date between '".$year."-06-15' and '".$year."-10-24' ";
} elseif($term=="2") {
$fyear = " app_date between '".$year."-11-19' and '".($year+1)."-03-17' ";
} else {
$fyear = " app_date between '".($year+1)."-04-08' and '".($year+1)."-06-09' ";
}
$query .= ' WHERE '.$fyear.' AND category_id = "'.$_POST['filter_category'].'" ';
$query .= ' group by app_month order by app_date';
$statement = $connect->prepare($query);
$statement->execute();
$result = $statement->fetchAll();
foreach($result as $row) {
$data .= ($data!="")?",":"";
$data .= $row['qty'];
$labels .= ($labels!="")?",":"";
$labels .= '"'.$row['app_month'].'/'.($row['app_year']+543).'"';
}
?>
<canvas id="myChart" style="width: 75%; height: 50%;"></canvas>
<?php } ?>
<button type="submit" name="graph" id="graph" class="no-print" value="graph" onclick="window.print();">พิมพ์กราฟ</button>
</div>
</body>
</html>
<?php if($_POST["print"]!="") { ?>
<script type="text/javascript" language="javascript" >
window.print();
</script>
<?php } else { ?>
<script>
var ctx = document.getElementById("myChart").getContext('2d');
var myChart = new Chart(ctx, {
type: 'bar',
data: {
labels: [<?php print $labels?>],
datasets: [{
label: '# จำนวน',
data: [<?php print $data?>],
backgroundColor: [
'rgba(255, 99, 132, 0.2)',
'rgba(54, 162, 235, 0.2)',
'rgba(255, 206, 86, 0.2)',
'rgba(75, 192, 192, 0.2)',
'rgba(153, 102, 255, 0.2)',
'rgba(255, 159, 64, 0.2)'
],
borderColor: [
'rgba(255,99,132,1)',
'rgba(54, 162, 235, 1)',
'rgba(255, 206, 86, 1)',
'rgba(75, 192, 192, 1)',
'rgba(153, 102, 255, 1)',
'rgba(255, 159, 64, 1)'
],
borderWidth: 1
}]
},
options: {
scales: {
yAxes: [{
ticks: {
beginAtZero: true
}
}]
}
}
});
</script>
<?php } ?>
Tag : PHP, MySQL
|
|
 |
 |
 |
 |
Date :
2019-02-23 17:06:53 |
By :
max |
View :
671 |
Reply :
2 |
|
 |
 |
 |
 |
|
|
|
 |