 |
|
code ตามนี้ครับ รบกวนผู้รู้ด้วยครับ
มือใหม่เพิ่งหัดทำนะครับ
Code (PHP)
<?php
session_start();
include "connection.php";
$id = $_GET["id"];
$sql = "SELECT * FROM product inner join type_product
on product.type_product_id = type_product.type_product_id
where product.product_id = '$id'
order by product.product_id Desc";
$objQuery = mysql_query($sql) or die ("Error Query [".$sql."]"); echo mysql_error();
$rs = mysql_fetch_array($objQuery)
?>
<link rel="stylesheet" media="screen, projection" href="./dist/drift-basic.css">
<style type="text/css">
body {
font-family: Helvetica Neue, Arial, sans;
margin-top: 2em;
background: #FAFAFA;
}
.wrapper {
margin: 0 auto;
width: 860px;
}
.drift-demo-trigger {
width: 40%;
float: left;
}
.detail {
position: relative;
width: 55%;
margin-left: 5%;
float: left;
}
h1 {
color: #013C4A;
margin-top: 1em;
margin-bottom: 1em;
}
p {
max-width: 32em;
margin-bottom: 1em;
color: #23637f;
line-height: 1.6em;
}
p:last-of-type {
margin-bottom: 2em;
}
a {
color: #00C0FA;
}
.ix-link {
display: block;
margin-bottom: 3em;
}
@media (max-width: 900px) {
.wrapper {
text-align: center;
width: auto;
}
.detail, .drift-demo-trigger {
float: none;
}
.drift-demo-trigger {
max-width: 100%;
width: auto;
margin: 0 auto;
}
.detail {
margin: 0;
width: auto;
}
p {
margin: 0 auto 1em;
}
.responsive-hint {
display: none;
}
}
</style>
<div class="wow flipInY" >
<div class="section-heading text-center">
<h4 class="h-bold">รายละเอียดสินค้า " <?=$rs[product_name]?> "</h4>
<div class="divider-header"></div>
</div>
</div>
<form action="cart_.php#work" method="post">
<div class="row">
<div class="wrapper">
<img class="drift-demo-trigger" data-zoom="admin/img/product/<?=$rs[product_image]?>" src="admin/img/product/<?=$rs[product_image]?>">
<div class="detail">
<div class="col-md-12">
<table width="100%" border="0">
<tr>
<td width="20%"><strong>รหัสสินค้า</strong></td>
<td><?=$rs[product_id]?></td>
</tr>
<tr>
<td><strong>หมวดสินค้า</strong></td>
<td><?=$rs[type_name]?></td>
</tr>
<tr>
<td><strong>ชื่อสินค้า</strong></td>
<td><?=$rs[product_name]?></td>
</tr>
<tr>
<td><strong>ราคา</strong></td>
<td><?=$rs[product_price]?> บาท</td>
</tr>
<tr>
<td><strong>น้ำหนัก</strong></td>
<td><?=$rs[product_weight]?> กรัม </td>
</tr>
<tr>
<td valign="top"><strong>รายละเอียด</strong></td>
<td><?=$rs[product_detail]?> </td>
</tr>
<tr>
<td valign="top"> </td>
<td><? if($rs[product_stock] <= 0)
{
echo"<font color='red'>สินค้าหมดชั่วคราว</font>";
}
?>
<br>
</td>
</tr>
<tr>
<td valign="top"> </td>
<td> </td>
</tr>
</table>
<div class="row" >
<div class="col-md-3" align="center">
<div id="fb-root"></div>
<script>(function(d, s, id) {
var js, fjs = d.getElementsByTagName(s)[0];
if (d.getElementById(id)) return;
js = d.createElement(s); js.id = id;
js.src = "//connect.facebook.net/th_TH/sdk.js#xfbml=1&version=v2.0";
fjs.parentNode.insertBefore(js, fjs);
}(document, 'script', 'facebook-jssdk'));</script>
<div class="fb-share-button" data-href="http://www.scomputer.in.th/?file=product_detail.php&id=<?=$id?>" data-layout="button_count" ></div>
</div>
<div class="col-md-3" align="right" >
<input type=button onClick='window.history.back()' value='ย้อนกลับ' class="btn btn-red">
</div>
<div class="col-md-3" >
<? if($rs[product_stock] > 0)
{ ?>
<input type="submit" name="add_to_cart" class="btn btn-info" value="เพิ่มสินค้าลงตระกร้า" />
<? }?>
</div></div>
</div>
</div>
<input type="hidden" name="h_product_id" value="<?=$rs['product_id']?>">
<input type="hidden" name="h_product_price" value="<?=$rs['product_price']?>">
<input type="hidden" name="h_weight" value="<?=$rs['product_weight']?>">
<input type="hidden" name="h_product_name" value="<?=$rs['product_name']?>">
</div>
</div>
<script src="dist/Drift.js"></script>
<script>
new Drift(document.querySelector('.drift-demo-trigger'), {
paneContainer: document.querySelector('.detail'),
inlinePane: 400,
inlineOffsetY: -85,
containInline: true
});
</script>
</form>
<div style="padding:7%"></div>
<div class="col-xs-12">
<div class="service-box">
<div class="accordion" id="accordionArea">
<div class="accordion-group">
<div class="accordion-heading accordionize">
<a class="accordion-toggle" data-toggle="collapse" data-parent="#accordionArea" href="#oneArea">
รีวิวสินค้า
<i class="fa fa-chevron-down"></i>
</a>
</div>
<div id="oneArea" class="accordion-body collapse">
<? if(isset($_SESSION['member_id']))
{
$member_id = $_SESSION['member_id'];
$strSQL = "SELECT * FROM member WHERE member_id = '$member_id' ";
$objQuery = mysql_query($strSQL);
$objResult = mysql_fetch_array($objQuery);
?>
<form action="comment_product.php" method="post">
<label>ความคิดเห็นที่มีต่อสินค้า</label> <textarea name="comment_detail" type="text" class="form-control" rows="3" ></textarea>
<label>ชื่อจริง</label><input name="member_id" type="text" class="form-control" placeholder="ชื่อผู้ร่วมรีวิว" value="<?=$objResult["username"];?> " readonly="readonly" >
<br>
<button type="submit" class="btn btn-info">แสดงความคิดเห็น</button>
<button type="reset" class="btn btn-danger">ยกเลิก </button>
<input type="hidden" name="product_id" value="<?=$rs[product_id]?>">
</form> <? } ?>
<div style="padding:30px"></div>
<h3>ความคิดเห็นสินค้า</h3>
<?
$product_id = $rs['product_id'];
$i = 1 ;
$q="SELECT * FROM comment INNER JOIN member ON member.member_id = comment.member_id WHERE comment.product_id = '$product_id' ORDER BY comment.comment_id DESC ";
$qr=mysql_query($q);
$Num_Rows=mysql_num_rows($qr);
if($Num_Rows==0){
echo "ยังไม่มีความคิดเห็นต่อสินค้านี้"; }
while($rs=mysql_fetch_array($qr)){
?>
ความคิดเห็นที่ <?=$i?>
<p/><?=$rs['comment_detail']?>
<p/>ผู้แสดงความคิดเห็น คุณ : <?=$rs['username']?>
<hr>
<? $i++; } ?>
</div>
</div>
</div>
</div>
</div>
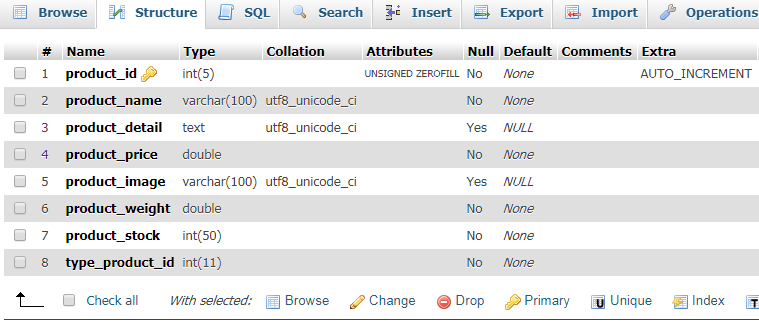
นี้ทดลองจาก localhost ครับ ได้ปกติ
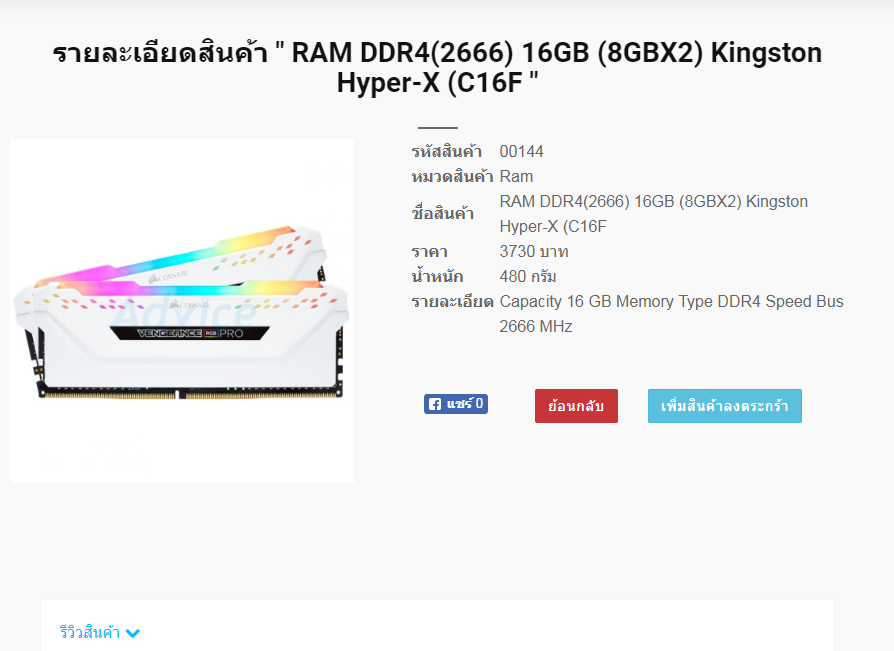
[color=red]อันนี้อัพขึ้นโฮสครับ
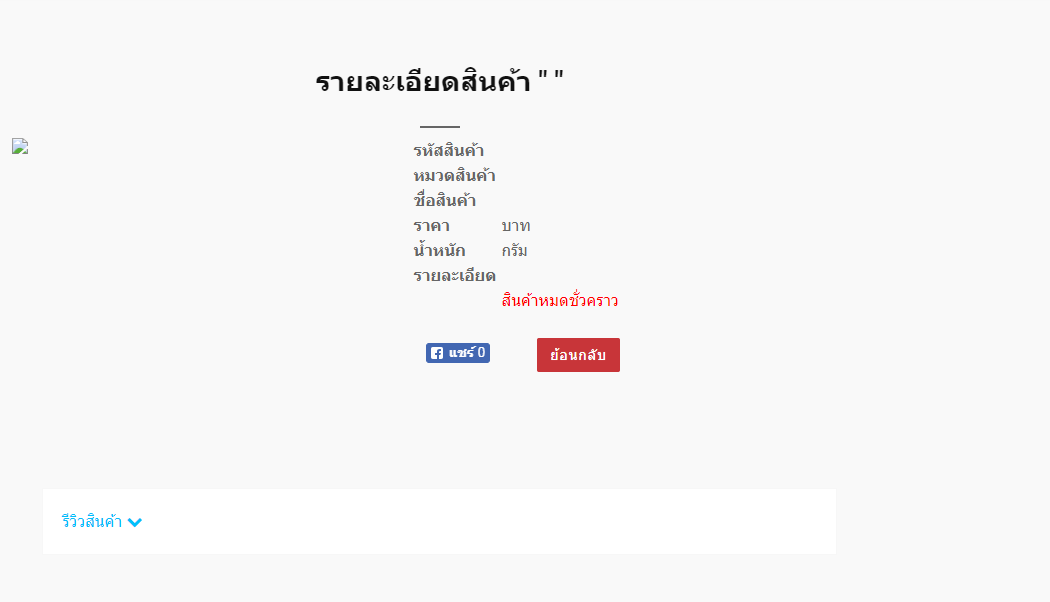
Tag : PHP, MySQL
|
ประวัติการแก้ไข 2019-03-27 20:01:18 2019-03-27 20:02:36 2019-03-27 20:03:14 2019-03-27 20:05:44
|
 |
 |
 |
 |
Date :
2019-03-27 19:59:51 |
By :
takul206 |
View :
806 |
Reply :
2 |
|
 |
 |
 |
 |
|
|
|
 |