 |
|
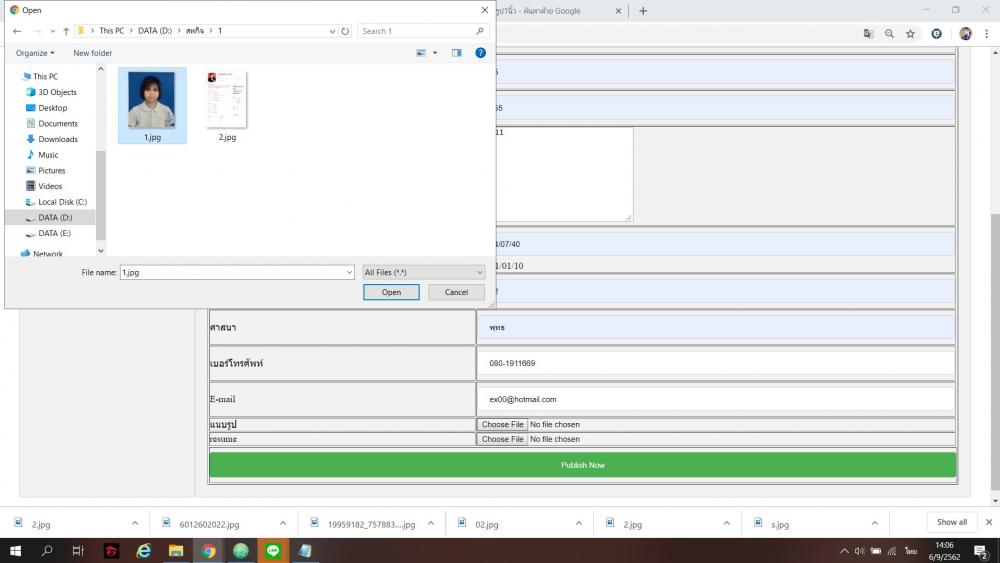

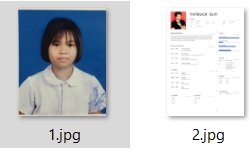
Code (PHP)
<?php
ini_set('post_max_size', '2000M');
ini_set('upload_max_filesize', '40M');
session_start();
require_once "connect.php";
if (isset($_POST['submit'])) {
//print_r($_POST);
//die();
$post_id = $_POST['id'];
$post_nameth = $_POST['nameth'];
$post_nameen = $_POST['nameen'];
$post_weight = $_POST['weight'];
$post_height = $_POST['height'];
$post_address = $_POST['address'];
$post_birthday = $_POST['birthday'];
$post_age = $_POST['age'];
$post_religion = $_POST['religion'];
$post_tel = $_POST['tel'];
$post_email = $_POST['email'];
$post_image = $_FILES['image']['name'];
$image_tmp = $_FILES['image']['tmp_name'];
$post_resume = $_FILES['image1']['name'];
$image_tmp = $_FILES['image1']['tmp_name'];
move_uploaded_file($image_tmp, "../img/$post_image");
move_uploaded_file($image_tmp, "../img/$post_resume");
$insert_query = "INSERT INTO job (post_personal_id, post_nameth, post_nameen, post_weight, post_height, post_address, post_birthday, post_age, post_religion, post_tel, post_email, post_image, post_resume )
VALUES ('$post_id', '$post_nameth', '$post_nameen', '$post_weight', '$post_height','$post_address','$post_birthday','$post_age','$post_religion', '$post_tel', '$post_email', '$post_image', '$post_resume')";
//die($insert_query);
if (mysqli_query($conn, $insert_query)) {
echo "<script>alert('Post published successfully');</script>";
header("location: viewjob.php");
} else {
echo "<script>alert('Something wrong!');</script>";
}
}
?>
<!DOCTYPE html>
<html lang="en" dir="ltr">
<head>
<meta charset="utf-8">
<title>Insert Page</title>
<link rel="stylesheet" href="css/stylemew.css">
</head>
<body>
<header align="center">
<div class="container">
<h1>ยินดีต้อนรับเข้าสู่เว็บไซต์บริษัท</h1>
</div>
</header>
<style>
input[type=text], select {
width: 100%;
padding: 12px 20px;
margin: 8px 0;
display: inline-block;
border: 1px solid #ccc;
border-radius: 4px;
box-sizing: border-box;
}
input[type=submit] {
width: 100%;
background-color: #4CAF50;
color: white;
padding: 14px 20px;
margin: 8px 0;
border: none;
border-radius: 4px;
cursor: pointer;
}
input[type=submit]:hover {
background-color: #45a049;
}
div.sidebar{
border-radius: 5px;
background-color: #f2f2f2;
padding: 20px;
}
div.showinfo{
border-radius: 5px;
background-color: #f2f2f2;
padding: 20px;
}
</style>
<section class="content">
<div class="content_grid">
<div class="sidebar">
<h1>Welcome : </h1>
<h3><a href="index.php">Home</a></h3>
<h3><a href="viewjob.php">View</a></h3>
<h3><a href="insertjob.php">แบบฟอร์มสมัครงาน</a></h3>
<h3><a href="logout.php">Logout</a></h3>
</div>
<div class="showinfo">
<h1>แบบฟอร์มสมัครงาน</h1>
<form action="insertjob.php" method="post" enctype="multipart/form-data">
<table width="100%" align="center" border="1">
<tr>
<td align="center" colspan="6"><h1>Insert</h1></td>
</tr>
<tr>
<td>เลขประจำตัวประชาชน</td>
<td><input type="text" name="id" size="25"></td>
</tr>
<tr>
<td>ชื่อ-นามสกุล (ภาษาไทย)</td>
<td><input type="text" name="nameth" size="25"></td>
</tr>
<tr>
<td>ชื่อ-นามสกุล (ภาษาอังกฤษ)</td>
<td><input type="text" name="nameen" size="25"></td>
</tr>
<tr>
<td>น้ำหนัก</td>
<td><input type="text" name="weight" size="25"></td>
</tr>
<tr>
<td>ส่วนสูง</td>
<td><input type="text" name="height" size="25"></td>
</tr>
<tr>
<td>ที่อยู่</td>
<td><textarea name="address" cols="35" rows="10"></textarea></td>
</tr>
<tr>
<td>วันเกิด</td>
<td><input type="text" name="birthday" size="25">Ex.01/01/10</td>
</tr>
<tr>
<td>อายุ</td>
<td><input type="text" name="age" size="25"></td>
</tr>
<tr>
<td>ศาสนา</td>
<td><input type="text" name="religion" size="25"></td>
</tr>
<tr>
<td>เบอร์โทรศัพท์</td>
<td><input type="text" name="tel" size="25"></td>
</tr>
<tr>
<td>E-mail</td>
<td><input type="text" name="email" size="25"></td>
</tr>
<tr>
<td>แนบรูป</td>
<td><input type="file" name="image" size="50"></td>
</tr>
<tr>
<td>resume</td>
<td><input type="file" name="image1" size="50"></td>
</tr>
<tr>
<td align="center" colspan="6"><input type="submit" name="submit" value="Publish Now"></td>
</tr>
</table>
</form>
</div>
</div>
</section>
</body>
</html>
Tag : PHP
|
|
 |
 |
 |
 |
Date :
2019-09-06 14:10:34 |
By :
2379645052123537 |
View :
600 |
Reply :
2 |
|
 |
 |
 |
 |
|
|
|
 |