 |
ช่วยดูการนำข้อมูลตารางที่เชื่อมกันมาแสดงทีครับ บันทึกเป็นไอดี เเต่จะให้เเสดงเป็นชื่อ |
|
 |
|
|
 |
 |
|
ตารางผู้ใช้ user
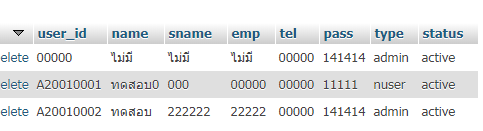
ตารางแจ้งซ่อม informit

ตารางเเจ้งซ่อมจะมี user_id(ผู้แจ้ง) , wker(ผู้รับงาน) , wkerc(ผู้ปิดงาน) เชื่อมมาจากตาราง user โดยใช้ user_id (ผู้เเจ้ง ผู้รับงาน ผู้ปิดงาน ระบุโดยใช้ user_idจากตาราง user)
ติดตรงที่เวลาเอามาเเสดง ผู้เเจ้งสามารถแสดงชื่อได้ ทั้งที่บันทึกเป็น user_id เเต่ว่า ผู้รับ ผู้ปิด เเสดงเป็น user_id ตามที่บันทึกไว้ อยากให้ออกมาเป็นชื่อทั้งหมดเลยครับ ผมน่าจะพลาดตรงโค๊ด SQL ไม่เเม่น ช่วยดูหน่อยนะครับ ขอบคุณครับ

Code (PHP)
<!DOCTYPE html>
<html>
<head>
<?php
include "css/css.php";
include "css/menu.php";
include 'db/connect.php';
?>
</head>
<body class="w3-animate-opacity bg">
<div class="container">
<?php
$perpage = 10;
if (isset($_GET['page'])) {
$page = $_GET['page'];
} else {
$page = 1;
}
$start = ($page - 1) * $perpage;
$sql = "select * from informit inner join user on user.user_id = informit.user_id ORDER BY id DESC limit {$start} , {$perpage} ";
$query = mysqli_query($connect, $sql);
?>
<center> <h3>รายการแจ้งซ่อมไอที</h3> </center>
<br>
<div class="table-responsive">
<table class="table table-striped">
<thead>
<tr>
<th>หมายเลข</th>
<th>วันที่/เวลา</th>
<th>ผู้แจ้ง</th>
<th>ผู้รับงาน</th>
<th>ผู้ปิดงาน</th>
<th>สถานะ</th>
<th>รายละเอียด</th>
</tr>
</thead>
<tbody>
<?php while ($row = mysqli_fetch_assoc($query)) { ?>
<tr>
<td scope="row"><?php echo $row['id'];?></td>
<td><?php echo $row['datet'];?></td>
<td><?php echo $row['name'];?></td>
<td><?php echo $row['wker'];?></td>
<td><?php echo $row['wkerc'];?></td>
<td><?php echo $row['statusit'];?></td>
<td><a href="vinformit.php?id=<?php echo $row["id"];?>" target="_blank"><button type="button" class="btn btn-info btn-sm">เปิด</button></a></td>
</tr>
<?php } ?>
</tbody>
</table>
<!--Table-->
<?php
$sql2 = "select * from informit";
$query2 = mysqli_query($connect, $sql2);
$total_record = mysqli_num_rows($query2);
$total_page = ceil($total_record / $perpage);
?>
</div>
<div class="btn-group">
<button type="button" class="btn btn-primary dropdown-toggle" data-toggle="dropdown" aria-haspopup="true" aria-expanded="false">
หน้า <?php echo $page; ?>
</button>
<div class="dropdown-menu">
<?php for($i=1;$i<=$total_page;$i++){ ?>
<a class="dropdown-item" href="main.php?page=<?php echo $i; ?>"><?php echo $i; ?></a>
<?php } ?>
</div>
</div>
</div>
</body>
</html>
Tag : PHP, HTML, CSS, HTML5, CakePHP, FuelPHP
|
ประวัติการแก้ไข 2020-04-14 00:31:45
|
 |
 |
 |
 |
Date :
2020-04-14 00:29:12 |
By :
1607845825935583 |
View :
652 |
Reply :
2 |
|
 |
 |
 |
 |
|
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
Code (SQL)
SELECT `informit`.*,
`user`.`name`,
`userwker`.`name` AS `userwker_name`,
`userwkerc`.`name` AS `userwkerc_name`,
FROM `informit`
JOIN `user` ON user.user_id = informit.user_id
JOIN `user` AS `userwker` ON `userwker`.`user_id` = informit.wker
JOIN `user` AS `userwkerc` ON `userwkerc`.`user_id` = informit.wkerc
ORDER BY `id` DESC
LIMIT {$start} , {$perpage}
เวลาแสดงชื่อก็ใช้ echo ที่คอลัมน์ userwker_name กับ userwkerc_name.
อ่านโค้ดให้เข้าใจก่อนด้วย
ยังไม่ได้ทดลอง แต่เปรียบเทียบเอาเองกับของผมที่ทดลองแล้วทำงานได้
Code (SQL)
SELECT `test`.*,
`rdb_users`.`user_id`,
`rdb_users`.`user_login` AS `u_poster`,
`usercommenter`.`user_login` AS `u_commenter`
FROM `test`
LEFT JOIN `rdb_users` ON `rdb_users`.`user_id` = `test`.`poster`
LEFT JOIN `rdb_users` AS `usercommenter` ON `usercommenter`.`user_id` = `test`.`commenter`
อ้างอิง
https://stackoverflow.com/questions/48082502/mysql-multiple-relations-between-one-field-of-a-table-and-two-fields-from-anoth
|
ประวัติการแก้ไข 2020-04-14 03:24:36 2020-04-14 03:25:31
 |
 |
 |
 |
Date :
2020-04-14 03:23:01 |
By :
mr.v |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
จะลองดูครับ ขอบคุณมากนะครับ
|
 |
 |
 |
 |
Date :
2020-04-14 09:43:05 |
By :
1607845825935583 |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
|
|