 |
php pdo insert sql server แล้ว error เรื่องค่าไม่ตรงกับที่ตั้งในดาต้าเบสครับ |
|
 |
|
|
 |
 |
|
หน้าส่งค่าครับ
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>ProductRecipe</title>
<link rel = "icon" type = "image/png" href = "images/icon/favicon.ico">
<?php
$user_id = $_REQUEST["user_id"];
include('navbar.php');
$batch=$_REQUEST["batch"];
include("connectpdo1.php");
$sql = "SELECT SampleName,Batch,Quantity,Batch.Application_ID as ApplicationID,Application.Name as name
FROM [Application].[dbo].[Batch]
Inner join Application on Batch.Application_ID=Application.ID
where Batch='$batch'";
$stmt = $conn->prepare($sql);
$stmt->execute();
$result = $stmt->fetch(PDO::FETCH_ASSOC);
?>
<link rel="stylesheet" herf="css/bootstrap.min.css" >
<script src="js/bootstrap.min.js"></script>
<script src="js/jquery-3.6.0.min.js"></script>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/css/bootstrap.min.css">
<script src="http://code.jquery.com/jquery-latest.js"></script>
<script type="text/javascript">
$(document).ready(function(){
var rows = 1;
$("#createRows").click(function(){
var tr = "<tr>";
tr = tr + "<td><input type='text' name='ingredient"+rows+"' id='ingredient"+rows+"' ></td>";
tr = tr + "<td><input type='text' name='percent"+rows+"' id='percent"+rows+"' ></td>";
tr = tr + "</tr>";
$('#myTable > tbody:last').append(tr);
$('#hdnCount').val(rows);
rows = rows + 1;
});
$("#deleteRows").click(function(){
if ($("#myTable tr").length != 1) {
$("#myTable tr:last").remove();
}
});
$("#clearRows").click(function(){
rows = 1;
$('#hdnCount').val(rows);
$('#myTable > tbody:last').empty(); // remove all
});
$("#frmMain").submit(function() {
var bFlag = true;
$("table#myTable input[id*='ingredient']").each(function (index) {
var line = index + 1;
var ingredient = $("table#myTable input[id*='ingredient']").eq(index);
var percent = $("table#myTable input[id*='percent']").eq(index);
if($(ingredient).val() != "" || $(percent).val() != "")
{
if($(ingredient).val() == "")
{
alert('Please input Ingredient line...' + line);
bFlag = false;
}
if($(percent).val() == "")
{
alert('Please input % line...' + line);
bFlag = false;
}
}
});
if(!bFlag) { return false; }
return true;
});
});
</script>
</head>
<body>
<form id="frmMain" name="frmMain" method="post" action="SavePredust.php">
<div class="container">
<table class="table table-bordered" >
<thead class="thead-dark">
<tr>
<th>Sample Name</th>
<th>Batch Ref No.</th>
<th>Quantity</th>
<th>Application</th>
</tr>
</thead>
<tbody>
<tr>
<td><?php echo $result["SampleName"];?></td>
<td>
<?php echo $result["Batch"];?>
<input type ="hidden" name="batch" value="<?php echo $result["Batch"];?>">
</td>
<td><?php echo $result["Quantity"];?></td>
<td><?php echo $result["name"];?></td>
</tr>
</tbody>
</table>
<fieldset><legend>ProductRecipe</legend>
<table class="table table-bordered" >
<thead class="thead-dark" align="center">
<tr>
<th>NameProduct</th>
<th><input name="NameProduct" type="text"></th>
<th>Date</th>
<th><input name="date" type="date"></th>
</tr>
</thead>
<tbody>
<tr>
<td>flour name</td>
<td><input name="flourname" type="text"></td>
<td>Volume</td>
<td><input name=Volume type="text"></td>
</tr>
</tbody>
<tbody>
<tr>
<td>CustomerName</td>
<td><input name="customerName" typr="text"></td>
<td>Application</td>
<td>
<?php echo $result["name"];?>
<input type="hidden" name="Application_ID" value="<?php echo $result["ApplicationID"]?>">
</td>
</tr>
</tbody>
<tbody>
<tr>
<td>Note</td>
<td><textarea name="Note" rows="4" cols="40"></textarea></td>
</tr>
</tbody>
</table>
</fieldset>
<fieldset><legend>Predust</legend>
<table id="myTable" width="40%" cellspacing="1" cellpadding="1" align="center" border="1">
<thead>
<tr>
<td align="middle">ingredient</td>
<td align="middle">%</td>
</tr>
</thead>
<tbody>
<tr>
<input type="button" id="createRows" value="Add">
<input type="button" id="deleteRows" value="Del">
<input type="button" id="clearRows" value="Clear">
</tr>
</tbody>
</table>
</fieldset>
<input type="hidden" id="hdnCount" name="hdnCount">
<input type ="hidden" name="user_id" value="<?php echo $user_id; ?>">
<input type="submit" value="Submit">
</div>
</form>
</body>
</html>
พอรับค่ามาแล้วให้มัน Insert ลง SQL SERVER 2008 มันมีปัญหาเรื่องตัวแปรครับ
SaveProduct.pdf
<!DOCTYPE html>
<html>
<head>
<title>ThaiCreate.Com</title>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8">
<meta charset=utf-8 />
</head>
<body>
<?php
ini_set('display_errors', 1);
error_reporting(~0);
$nameproduct=$_REQUEST["NameProduct"];
$Batch=$_REQUEST["batch"];
$date=$_REQUEST["date"];
$flourname=$_REQUEST["flourname"];
$volume=floatval($_REQUEST["Volume"]);
$customer=$_REQUEST["customerName"];
$Application_ID=$_REQUEST["Application_ID"];
$Note=$_REQUEST["Note"];
$user_id=$_REQUEST["user_id"];
echo "batch : ".$Batch."<BR>";
echo "NameProduct : ".$nameproduct."<br>";
echo "Date : ".$date."<br>";
echo "Flourname : ".$flourname."<br>";
echo "Volume : ".$volume."<br>";
echo "CustomerName : ".$customer."<br>";
echo "Application ID : ".$Application_ID."<br>";
echo "Note : ".$Note."<br>";
echo "user_id : ".$user_id."<br>";
include("connectpdo1.php");
$sql="INSERT INTO ProductRecipe (NameProduct,FlourName,CustomerName,Date,Volume,Detail,Batch_ID,Member) VALUES ('?','?','?','?','?','?','?','?')";
$params = array($nameproduct,$flourname,$customer,$date,$volume,$Note,$Batch,$user_id);
$stmt = $conn->prepare($sql);
$stmt->execute($params);
if( $stmt->rowCount()) {
echo "Record add successfully";
}
for ($i = 1; $i<= (int)$_POST["hdnCount"]; $i++){
if($_POST["ingredient$i"] != "" && $_POST["percent$i"] != "")
{
echo "<br>Line $i";
echo "<br>ingredient =".$_POST["ingredient$i"];
echo "<br>% =".$_POST["percent$i"];
echo "<hr>";
$sql="INSERT INTO Predust (Ingredient,[Value],Batch_ID,Member) VALUES ('?','?','?','?')";
$params = array($_POST["ingredient$i"],$_POST["percent$i"],$Batch,$user_id);
$stmt = $conn->prepare($sql);
$stmt->execute($params);
if( $stmt->rowCount() ) {
echo "Record add Predust successfully";
}
}
}
?>
</body>
</html>
ปัญหาคือผมไม่ค่อยเข้าใจการใช้ PDO เท่าไหร่ครับพอหาข้อมูลเรื่องตัวแปรก็ไม่เข้าใจเลยมาขอความรู้หน่อยครับ
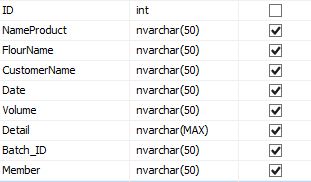
นี่คือดาต้าเบสที่จะเอาเข้าครับ

นี่คือเออเร่อครับ
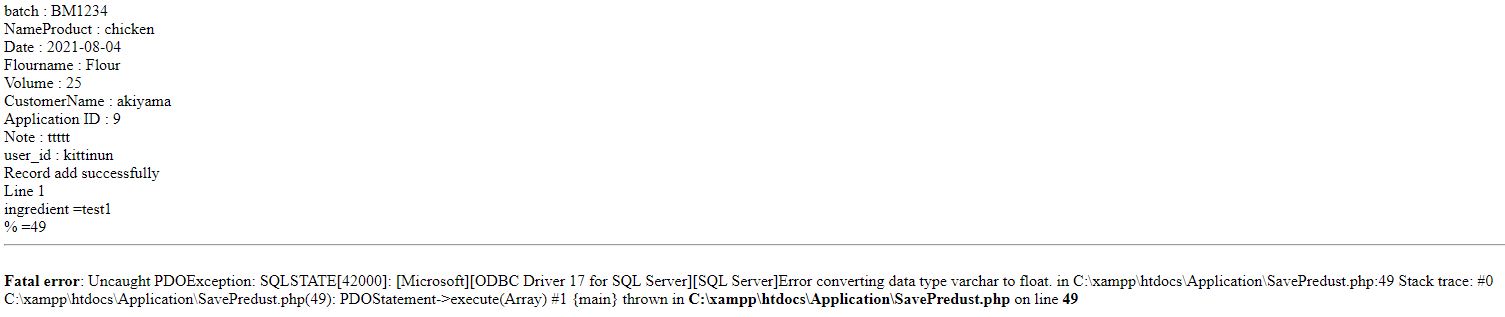
จะมีสองตัวแปรครับที่เออเร่อครับคือ date กับ flort จากเออเร่อมันบอกว่าตัวแปร Vachar มัน insert date กับ float ไม่ได้พอจะมีวิธีแก้ไหมครับ
Tag : PHP, Ms SQL Server 2008, HTML5, JavaScript, Apache, XAMPP
|
|
 |
 |
 |
 |
Date :
2021-08-04 15:59:55 |
By :
kittitnun |
View :
1303 |
Reply :
8 |
|
 |
 |
 |
 |
|
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ตอบความคิดเห็นที่ : 1 เขียนโดย : mr.v เมื่อวันที่ 2021-08-04 16:37:05
รายละเอียดของการตอบ ::
ลองแก้โค้ดใหม่ดูครับ
<!DOCTYPE html>
<html>
<head>
<title>ThaiCreate.Com</title>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8">
<meta charset=utf-8 />
</head>
<body>
<?php
ini_set('display_errors', 1);
error_reporting(~0);
$nameproduct=$_REQUEST["NameProduct"];
$Batch=$_REQUEST["batch"];
$date=$_REQUEST["date"];
$flourname=$_REQUEST["flourname"];
$volume=floatval($_REQUEST["Volume"]);
$customer=$_REQUEST["customerName"];
$Application_ID=$_REQUEST["Application_ID"];
$Note=$_REQUEST["Note"];
$user_id=$_REQUEST["user_id"];
echo "batch : ".$Batch."<BR>";
echo "NameProduct : ".$nameproduct."<br>";
echo "Date : ".$date."<br>";
echo "Flourname : ".$flourname."<br>";
echo "Volume : ".$volume."<br>";
echo "CustomerName : ".$customer."<br>";
echo "Application ID : ".$Application_ID."<br>";
echo "Note : ".$Note."<br>";
echo "user_id : ".$user_id."<br>";
include("connectpdo1.php");
try{
$sql="INSERT INTO ProductRecipe (NameProduct,FlourName,CustomerName,Date,Volume,Detail,Batch_ID,Member)
VALUES (':nameProduct',':flourName',':customerName',':Date',':volume',':detail',':batch_ID',':member')";
$stmt = $conn->prepare($sql);
$stmt->bindValue(':nameProduct',$nameproduct,\PDO::PARAM_STR);
$stmt->bindValue(':flourName',$flourname,\PDO::PARAM_STR);
$stmt->bindValue(':customerName',$customer,\PDO::PARAM_STR);
$stmt->bindValue(':Date',$date,\PDO::PARAM_STR);
$stmt->bindValue(':volume',$volume,\PDO::PARAM_BOOL);
$stmt->bindValue(':detail',$Note,\PDO::PARAM_STR);
$stmt->bindValue(':batch_ID',$Batch,\PDO::PARAM_STR);
$stmt->bindValue(':member',$user_id,\PDO::PARAM_STR);
$result=$stmt->execute();
if($result){
echo "<script>alert('เพิ่มข้อมูลสำเร็จ');</script>";
}
else{
echo "<script>alert('มีข้อผิดพลาด');</script>";
}
}
catch(\Exception $ex){
echo $ex->getMessage().'<br>'."\n";
$stmt->debugDumpParams();
}
for ($i = 1; $i<= (int)$_POST["hdnCount"]; $i++){
if($_POST["ingredient$i"] != "" && $_POST["percent$i"] != "")
{
echo "<br>Line $i";
echo "<br>ingredient =".$_POST["ingredient$i"];
echo "<br>% =".$_POST["percent$i"];
echo "<hr>";
$sql="INSERT INTO Predust (Ingredient,[Value],Batch_ID,Member)
VALUES (':Ingredient',':Value',':Batch_ID',':Member')";
$stmt = $conn->prepare($sql);
$stmt->bindParam(':Ingredient',$nameproduct,\PDO::PARAM_STR);
$stmt->bindParam(':Value',$flourname,\PDO::PARAM_BOOL);
$stmt->bindParam(':Batch_ID',$customer,\PDO::PARAM_STR);
$stmt->bindParam(':Member',$user_id,\PDO::PARAM_STR);
$result=$stmt->execute();
if($result){
echo "บันทึกสำเร็จ";
}
}
}
?>
</body>
</html>
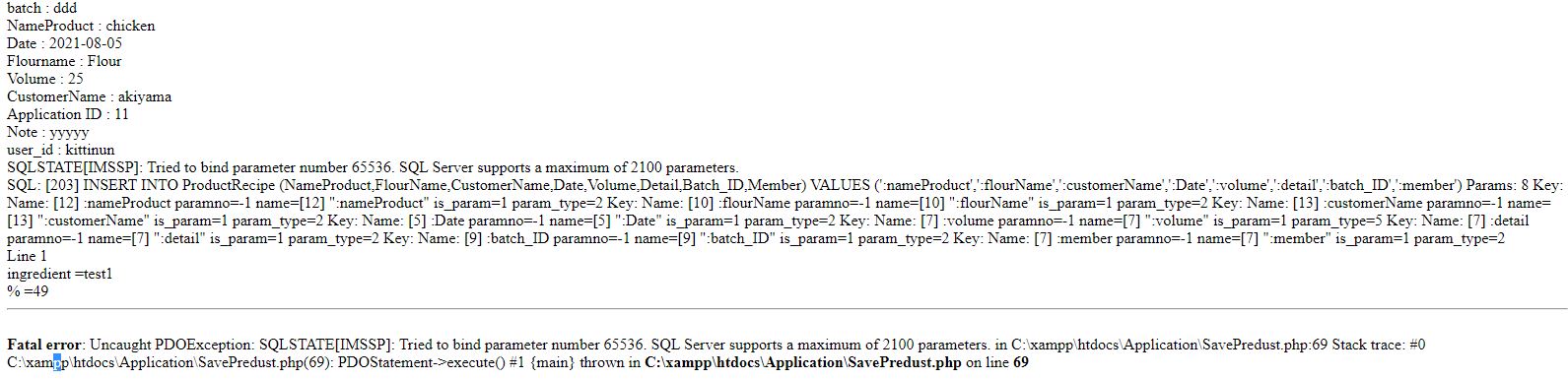
ได้เออเร่อใหม่มาแทนครับ
|
 |
 |
 |
 |
Date :
2021-08-05 12:54:12 |
By :
kittitnun |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ตอบความคิดเห็นที่ : 3 เขียนโดย : kittitnun เมื่อวันที่ 2021-08-05 12:54:12
รายละเอียดของการตอบ ::
แก้ได้ล่ะครับ
$sql="INSERT INTO ProductRecipe (NameProduct,FlourName,CustomerName,Date,Volume,Detail,Batch_ID,Member)
VALUES (':nameProduct',':flourName',':customerName',':Date',':volume',':detail',':batch_ID',':member')";
แก้เป็น
Code (PHP)
$sql="INSERT INTO ProductRecipe (NameProduct,FlourName,CustomerName,Date,Volume,Detail,Batch_ID,Member)
VALUES (:nameProduct,:flourName,:customerName,:Date,:volume,:detail,:batch_ID,:member)";
ก็หายแล้วครับ
|
ประวัติการแก้ไข 2021-08-05 13:14:10
 |
 |
 |
 |
Date :
2021-08-05 13:13:33 |
By :
kittitnun |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
เพิ่งเห็น
แนะนำอีกเรื่อง
การใช้ bindValue กับ bindParam ต่างกันมาก เลือกใช้ให้ดี ไม่ใช่ว่ามันเหมือนกันนะ ถ้าไม่เลือกไม่ดูให้ดี พัง!
Code (PHP)
$name = 'John';
$stmt->bindValue(':name', $name);
$name = 'Wick';
$stmt->execute();// $name จะบันทึกเป็น John
Code (PHP)
$name = 'John';
$stmt->bindParam(':name', $name);
$name = 'Wick';
$stmt->execute();// $name จะบันทึกเป็น Wick
|
 |
 |
 |
 |
Date :
2021-08-05 16:05:12 |
By :
mr.v |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ตอบความคิดเห็นที่ : 5 เขียนโดย : mr.v เมื่อวันที่ 2021-08-05 16:02:56
รายละเอียดของการตอบ ::
ปัญหาหัวกระทู้แก้ได้แล้วครับ
Code (PHP)
<!DOCTYPE html>
<html>
<head>
<title>ThaiCreate.Com</title>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8">
<meta charset=utf-8 />
</head>
<body>
<?php
ini_set('display_errors', 1);
error_reporting(~0);
$nameproduct=$_REQUEST["NameProduct"];
$Batch=$_REQUEST["batch"];
$date=$_REQUEST["date"];
$flourname=$_REQUEST["flourname"];
$volume=$_REQUEST["Volume"];
$customer=$_REQUEST["customerName"];
$Application_ID=$_REQUEST["Application_ID"];
$Note=$_REQUEST["Note"];
$user_id=$_REQUEST["user_id"];
echo "batch : ".$Batch."<BR>";
echo "NameProduct : ".$nameproduct."<br>";
echo "Date : ".$date."<br>";
echo "Flourname : ".$flourname."<br>";
echo "Volume : ".$volume."<br>";
echo "CustomerName : ".$customer."<br>";
echo "Application ID : ".$Application_ID."<br>";
echo "Note : ".$Note."<br>";
echo "user_id : ".$user_id."<br>";
include("connectpdo1.php");
try{
$sql="INSERT INTO ProductRecipe (NameProduct,FlourName,CustomerName,Date,Volume,Detail,Batch_ID,Member)
VALUES (:nameProduct,:flourName,:customerName,:Date,:volume,:detail,:batch_ID,:member)";
$stmt = $conn->prepare($sql);
$stmt->bindValue(':nameProduct',$nameproduct,\PDO::PARAM_STR);
$stmt->bindValue(':flourName',$flourname,\PDO::PARAM_STR);
$stmt->bindValue(':customerName',$customer,\PDO::PARAM_STR);
$stmt->bindValue(':Date',$date,\PDO::PARAM_STR);
$stmt->bindValue(':volume',$volume,\PDO::PARAM_BOOL);
$stmt->bindValue(':detail',$Note,\PDO::PARAM_STR);
$stmt->bindValue(':batch_ID',$Batch,\PDO::PARAM_STR);
$stmt->bindValue(':member',$user_id,\PDO::PARAM_STR);
$result=$stmt->execute();
if($result){
echo "<script>alert('เพิ่มข้อมูลสำเร็จ');</script>";
}
else{
echo "<script>alert('มีข้อผิดพลาด');</script>";
}
}
catch(\Exception $ex){
echo $ex->getMessage().'<br>'."\n";
$stmt->debugDumpParams();
}
for ($i = 1; $i<= (int)$_POST["hdnCount"]; $i++){
if($_POST["ingredient$i"] != "" && $_POST["percent$i"] != "")
{
echo "<br>Line $i";
echo "<br>ingredient =".$_POST["ingredient$i"];
echo "<br>% =".$_POST["percent$i"];
echo "<hr>";
try{
$sql="INSERT INTO Predust (Ingredient,[Value],Batch_ID,Member)
VALUES (:Ingredient,:Value,:Batch_ID,:Member)";
$stmt = $conn->prepare($sql);
$stmt->bindValue(':Ingredient',$_POST["ingredient$i"],\PDO::PARAM_STR);
$stmt->bindValue(':Value',$_POST["percent$i"],\PDO::PARAM_BOOL);
$stmt->bindValue(':Batch_ID',$Batch,\PDO::PARAM_STR);
$stmt->bindValue(':Member',$user_id,\PDO::PARAM_STR);
$result=$stmt->execute();
if($result){
echo "record susses";
}
}
catch(\Exception $ex){
echo $ex->getMessage().'<br>'."\n";
$stmt->debugDumpParams();
}
}
}
?>
</body>
</html>
แก้เป็นพวก bindValue แล้วเซ็ตค่าให้มันตรงกับที่ insert แล้วหายเลยครับ
|
 |
 |
 |
 |
Date :
2021-08-09 14:43:39 |
By :
kittitnun |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
|
|