 |
|
Code (PHP)
<?php
ini_set('display_errors', 1);
error_reporting(~0);
include("dbConnect.php");
$conn1 = bc();
$conn2 = wms();
$conn3 = bcinfo();
//session start
session_start();
$name = $_SESSION['name'];
$_SESSION['member_status'] = $_POST['member_status'];
if($_SESSION['member_status'] != "RETAIL")
//check name can't to be null value
if($_SESSION['name'] == ""){
header("location: index.php");
}
//if
//***********************************************************************//
//*********************************CART**********************************//
$message = '';
if(isset($_POST["add_to_cart"])){
if(isset($_COOKIE["shopping_cart"])){
$cookie_data = stripcslashes($_COOKIE['shopping_cart']);
$cart_data = json_decode($cookie_data, true);
}else{
$cart_data = array();
}
$item_id_list = array_column($cart_data, 'item_code');
if(in_array($_POST["hidden_code"], $item_id_list) && in_array($_POST["hidden_unit"], $item_id_list)){
foreach($cart_data as $keys => $values){
if($cart_data[$keys]["item_code"] == $_POST["hidden_code"] && $cart_data[$keys]["unit"] == $_POST["hidden_unit"]){
$cart_data[$keys]["qty"] = $cart_data[$keys]["qty"] + $_POST["qty"];
}//if
}//foreach
}else{//if
$item_array = array(
'item_code' => $_POST["hidden_code"],
'qty' => $_POST["qty"],
'unit' => $_POST["unit"],
'price' => $_POST["hidden_price"]
);
$cart_data[] = $item_array;
}//else
$item_data = json_encode($cart_data, JSON_UNESCAPED_UNICODE);
setcookie('shopping_cart', $item_data, time() + (86400 * 30));
header("location: sales_order.php?success=1");
}//if
//***********************************************************************//
//***********************************************************************//
//******************************BARCODE**********************************//
if(isset($_GET["search_item_barcode"])){
if(isset($_COOKIE["shopping_cart"])){
$cookie_data = stripcslashes($_COOKIE['shopping_cart']);
$cart_data = json_decode($cookie_data, true);
}else{//if
$cart_data = array();
}//else
$qty = "1";
$price = "100";
$item_id_list = array_column($cart_data, 'item_code');
if(in_array($_POST["item_no_barcode"], $item_id_list) && in_array($_POST["item_packing_barcode"], $item_id_list)){
//
}else{
$item_array = array(
'item_code' => $_POST["item_no_barcode"],
'unit' => $_POST["item_packing_barcode"]
);
$cart_data[] = $item_array;
}//else
$item_data = json_encode($cart_data, JSON_UNESCAPED_UNICODE);
setcookie('shopping_cart', $item_data, time() + (86400 * 30));
header("location: sales_order.php?success=1");
}//if
//***********************************************************************//
if(isset($_GET["action"])){
if($_GET["action"] == "delete"){
$cookie_data = stripslashes($_COOKIE['shopping_cart']);
$cart_data = json_decode($cookie_data, true);
foreach($cart_data as $keys => $values){
if($cart_data[$keys]['item_code'] == $_GET["code"] && $cart_data[$keys]['unit'] == $_GET["unit"]){
unset($cart_data[$keys]);
$item_data = json_encode($cart_data, JSON_UNESCAPED_UNICODE);
setcookie("shopping_cart", $item_data, time() + (86400 * 30));
header("location: sales_order.php");
}//if
}//foreach
}//if
if($_GET["action"] == "clear"){
$cookie_data = stripslashes($_COOKIE['shopping_cart']);
$cart_data = json_decode($cookie_data, true);
if(is_array($cart_data) || is_object($cart_data)){
foreach($cart_data as $keys => $values){
setcookie("shopping_cart", "", "Thu, 01 Jan 1970 00:00:00 GMT");
header("location: sales_order.php");
}//foreach
}//if
}//if
}//if
?>
<!DOCTYPE html>
<html lang="th">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link href="https://maxcdn.bootstrapcdn.com/bootstrap/4.5.0/css/bootstrap.min.css" rel="stylesheet">
<link href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/4.7.0/css/font-awesome.min.css" rel="stylesheet">
<link href="lib/jquery-ui.min.css?v=<?php echo filemtime('lib/jquery-ui.min.css'); ?>" type="text/css" rel="stylesheet">
<link href="lib/style.css?v=<?php echo filemtime('lib/style.css'); ?>" rel="stylesheet">
<link href="lib/autocomplete.css?v=<?php echo filemtime('lib/autocomplete.css'); ?>" rel="stylesheet">
<link href="icon/favicon.ico" rel="shortcut icon">
<link rel="stylesheet" href="https://use.fontawesome.com/releases/v5.7.1/css/all.css">
<script src="lib/jquery-1.9.1.min.js?v=<?php echo filemtime('lib/jquery-1.9.1.min.js'); ?>" type="text/javascript"></script>
<script src="lib/jquery-ui.min.js?v=<?php echo filemtime('lib/jquery-ui.min.js'); ?>" type="text/javascript"></script>
<script src="lib/jquery.ui.autocomplete.scroll.min.js?v=<?php echo filemtime('lib/jquery.ui.autocomplete.scroll.min.js'); ?>" type="text/javascript"></script>
<script src="lib/jquery.ui.autocomplete.scroll.js?v=<?php echo filemtime('lib/jquery.ui.autocomplete.scroll.js'); ?>" type="text/javascript"></script>
<script>
//Check null
function CheckItem(item) {
if (item.name.value == "") {
return false;
}
}
function CheckMember() {
let member_status = "WHOLESALE";
let member = document.getElementById("member").value;
document.getElementById("member_status").value = member_status;
}
//Click to display alert
$(document).ready(function(){
$("#purchase").click(function() {
alert("บันทึกข้อมูลเรียบร้อยแล้ว");
});
});
$(document).ready(function(){
$("#cancel").click(function() {
alert("แจ้งยกเลิกเรียบร้อยแล้ว");
});
});
//Count Stock
function CountStock() {
let stock = prompt("กรุณาป้อนสต๊อกที่นับได้", "0");
const code = document.getElementById("code").value;
const description = document.getElementById("hidden_description").value;
localStorage.setItem("CountStock", stock);
location.replace("add_to_purchase_2_sales_order.php?code="+ code + "&name=" + description + "&stock=" + stock);
}
//Event After Print
window.onafterprint = function() {
var c = document.cookie.split("; ");
for (i in c)
document.cookie =/^[^=]+/.exec(c[i])[0]+"=;expires=Thu, 01 Jan 1970 00:00:00 GMT";
location.replace("sales_order.php");
}
</script>
<title>ค้นหาสินค้า</title>
</head>
<body class="order" style="width: auto; max-height: 10rem;">
<!-- Menu -->
<ul id="menu" class="noprint">
<li><a href="order_purch.php">หน้าหลัก</a></li>
<li><a href="order_to_purchase.php">รายการรอสั่งซื้อ</a></li>
<li><a href="order_to_delete.php">รายการสั่งยกเลิกแล้ว</a></li>
<li><a href="#">ใบสั่งขาย</a></li>
<li class="right"><a href="check_logout.php">ออกจากระบบ</a></li>
<li class="right"><a><?php echo "[ ".$name." ]"; ?></a></li>
<a href="#" onclick="window.print();" class="right" style="width: 30px;">
<i class="fas fa-print" style="padding-top: 18px;"><span id="span"></span></i>
</a>
</ul>
<!-- Content -->
<div id="grad" style="max-height: 100vh">
<div id="content" style="margin-left: 2.5%;">
<div id="grid">
<form name="search_member" method="post">
<div class="search-member" style="margin-top: 55px; margin-left: 30%;">
<input type="text" name="member" id="member" class="search_item" placeholder="ชื่อลูกค้า" autocomplete="off" onkeydown="CheckMember()">
<input type="text" name="member_status" id="member_status" class="search_item" value="Retail">
</div>
<input type="submit" style="display: none;">
</form>
<form name="search_item_barcode" class="form-search" method="post" action="sales_order_cart.php">
<div class="search-text noprint" style="margin-top: 10px; margin-left: 40%; float: left;">
<input type="text" id="search_item_barcode" name="Barcode" class="search_item" placeholder="จำนวน*บาร์โค้ด" autocomplete="off" autofocus>
</div>
<input type="submit" name="search_item_barcode" style="display: none;">
</form>
<!-- SEARCH ITEM -->
<form class="form-search" method="post" onsubmit="return CheckItem(this)">
<div class="search-item noprint">
<div class="search-text">
<input type="text" id="search_item" name="Code" class="search_item" placeholder="ชื่อสินค้า | รหัสสินค้า | บาร์โค้ด" autocomplete="off">
</div>
<div class="search-button" style="margin-left: 0px;">
<input style="display: none;" type="submit" class="submit" value="ค้นหา">
</div>
</div>
</form>
</div>
<!-- INITIAL VAR -->
<?php
$strKeyword = null;
if(isset($_POST["Code"])){
$strKeyword = $_POST["Code"];
?>
<!-- CONTENT ITEM -->
<form method="post" class="noprint">
<table width="90%" border="1" class="table">
<tr align="center">
<th width="10%">รหัสสินค้า</th>
<th width="32%">ชื่อสินค้า</th>
<th width="8%">สต๊อก</th>
<th width="13%">แจ้งสั่งล่าสุด</th>
<th width="6%">สั่งโดย</th>
<th width="8%">แจ้งสั่งซื้อ</th>
<th width="6%">จำนวน</th>
<th width="8%">หน่วย</th>
<th width="5%">เพิ่ม</th>
</tr>
<?php
if(strpos($strKeyword, "|")){
$strKeyword = explode("|", $strKeyword);
$strKeyword = $strKeyword[1];
}//if
$qq = explode(" ", $strKeyword);
$sql8 = "SELECT TOP(1) item_no, uom_barcode, description, item_uom FROM product_uom WITH (NOLOCK) WHERE uom_barcode != '' AND item_no LIKE '%".$strKeyword."%' OR uom_barcode LIKE '%".$strKeyword."%' OR";
$i = 0;
foreach($qq as $aa){
if($i != 0){
$sql8 .= " AND ";
}//if
$sql8 .= " description LIKE '%".$aa."%'";
$i++;
}//foreach
$view1 = sqlsrv_query($conn2, $sql8);
while($data1 = sqlsrv_fetch_array($view1)){
$code = $data1["item_no"];
$name = $data1["description"];
?>
<tr>
<input type="hidden" id="code" value="<?php echo $data1["item_no"]; ?>">
<input type="hidden" id="hidden_description" value="<?php echo $data1["description"]; ?>">
<td align="center"><?php echo $data1["item_no"]; ?></td>
<td><?php echo $data1["description"];?></td>
<td align="center" id="show-stock" onmouseover="showForm();" onmouseout="hideForm();"><?php
$sql4 = "SELECT SUM(qty_avail) AS Qty FROM pallet_location P WITH (NOLOCK) WHERE item_no = '".$code."' GROUP BY warehouse ";
$view4 = sqlsrv_query($conn2, $sql4);
$sql5 = "SELECT uom FROM pallet_location WHERE item_no = '".$code."'";
$view5 = sqlsrv_query($conn2, $sql5);
$total = 0;
$uom = "";
$sql9 = "SELECT MAX(item_qty) AS Max FROM product_uom WITH (NOLOCK) WHERE item_no ='".$code."' AND uom_barcode != ''";
$view9 = sqlsrv_query($conn2, $sql9);
if($data9 = sqlsrv_fetch_array($view9)){
$sql10 = "SELECT item_packing FROM product_uom WITH (NOLOCK) WHERE item_no = '".$code."' AND item_qty = '".$data9["Max"]."' AND uom_barcode != ''";
$view10 = sqlsrv_query($conn2, $sql10);
if($data5 = sqlsrv_fetch_array($view5)){
while($data4 = sqlsrv_fetch_array($view4)){
$total += $data4["Qty"];
}//while
if($data10 = sqlsrv_fetch_array($view10)){
if(fmod($total, $data9["Max"]) == 0){
echo number_format($total / $data9["Max"])." ".$data5["uom"];
}else{
echo number_format($total / $data9["Max"], 0, "", ",").".".number_format(fmod($total, $data9["Max"]), 0, "", "")." ".$data10["item_packing"];
}//if
}//if
}//if
}//if
?></td>
<td><?php
$sql2 = "SELECT date, name FROM order_purchase WITH (NOLOCK) WHERE itemcode = '".$code."'";
$view2 = sqlsrv_query($conn3, $sql2);
$name_query = "";
if($data2 = sqlsrv_fetch_array($view2)){
echo $data2["date"]->format('d-m-Y H:i')." น.";
$name_query = $data2["name"];
}//if
?></td>
<td align="center"><?php echo $name_query; ?></td>
<td align="center">
<a id="purchase" class="btn btn-info" onclick="CountStock()">แจ้งสั่งซื้อ</a>
</td>
<td>
<input type="number" min="1" value="1" name="qty" id="qty" style="width: 100%; height: 30px; float: right;">
</td>
<td align="center" id="show-price" onmouseover="showForm();" onmouseout="hideForm();">
<select name="unit" id="unit" style="width: 100%; height: 30px;">
<?php
$sql12 = "SELECT DISTINCT item_packing, item_qty FROM product_uom WITH (NOLOCK) WHERE client = '001' AND item_packing != 'PALLET' AND item_no = '".$code."'";
$view12 = sqlsrv_query($conn2, $sql12);
while($data12 = sqlsrv_fetch_array($view12)){
$unit = $data12["item_packing"];
?>
<option value="<?php echo $data12['item_packing']; ?>"><?php echo $data12["item_packing"]; ?></option>
<?php
}
?>
</select>
</td>
<td align="center">
<input type="submit" name="add_to_cart" class="btn btn-success" value="เพิ่ม">
</td>
<input type="hidden" name="hidden_code" value="<?php echo $code; ?>">
<input type="hidden" name="hidden_unit" value="<?php echo $unit; ?>">
</tr>
<?php
}//while
?>
</table>
</form>
<div id="show-detail-stock" style="left: 44%; position: fixed; top: auto; color: white;">
<?php
$sql6 = "SELECT warehouse, SUM(qty_avail) AS QtyAvailable FROM pallet_location WITH (NOLOCK) WHERE item_no = '".$code."' GROUP BY warehouse";
$sql7 = "SELECT uom FROM pallet_location WITH (NOLOCK) WHERE item_no = '".$code."'";
$view6 = sqlsrv_query($conn2, $sql6);
$view7 = sqlsrv_query($conn2, $sql7);
while($data7 = sqlsrv_fetch_array($view7)){
while($data6 = sqlsrv_fetch_array($view6)){
?>
<div class="column">
<?php echo $data6["warehouse"]." / ".number_format($data6["QtyAvailable"], 2, '.', ',')." ".$data7["uom"]."<br>";?>
</div>
<?php
}//while
}//while
?>
</div>
<div id="show-detail-price" style="left: 85%; position: fixed; top: auto;">
<?php
$sql11 = "SELECT DISTINCT order_no, item_no, uom, unit_price
FROM sale_pricelist_detail WITH (NOLOCK)
WHERE client = '001' AND order_no = 'RETAIL' AND item_no = '".$code."' OR order_no = 'WHOLESALE' AND item_no = '".$code."'";
$view11 = sqlsrv_query($conn2, $sql11);
while($data11 = sqlsrv_fetch_array($view11)){
$unitprice = $data11["unit_price"];
echo ($data11["order_no"] == "RETAIL" ? "ราคาปลีก " : "ราคาส่ง ")."".$data11["uom"]."ละ ".number_format($data11["unit_price"], 2, '.', ',')." บาท"."<br>";
}//while
?>
<input type="hidden" name="hidden_price" value="<?php echo $unitprice; ?>">
</div>
<?php
}//if
//*********************************************************************BARCODE*********************************************************************//
$uom_barcode = "";
$item_no_barcode = "";
$item_packing_barcode = "";
$item_qty_barcode = "";
if(isset($_POST["Barcode"])){
$barcode = $_POST["Barcode"];
$sql14 = "SELECT DISTINCT uom_barcode, item_no, item_packing, item_qty FROM product_uom WITH (NOLOCK)
WHERE client = '001' AND item_packing != 'PALLET' AND uom_barcode = '".$barcode."'";
$view14 = sqlsrv_query($conn2, $sql14);
if($data14 = sqlsrv_fetch_array($view14)){
$uom_barcode = $data14["uom_barcode"];
$item_no_barcode = $data14["item_no"];
$item_packing_barcode = $data14["item_packing"];
$item_qty_barcode = $data14["item_qty"];
?>
<input type="text" name="uom_barcode" value="<?php echo $uom_barcode; ?>">
<input type="text" name="item_no_barcode" value="<?php echo $item_no_barcode; ?>">
<input type="text" name="item_packing_barcode" value="<?php echo $item_packing_barcode; ?>">
<input type="text" name="item_qty_barcode" value="<?php echo $item_qty_barcode; ?>">
<?php
}//if
}//if
//**************************************************************************************************************************************************//
?>
<!--//*********************************************************************CART*********************************************************************//-->
<div style="clear: both"></div><br>
<h2 align="center">รายการสั่งสินค้า</h2>
<div class="table-responsive" style="margin-bottom: 0%;"
<?php echo $message; ?>
<div align="right">
<a href="sales_order.php?action=clear" class="noprint">
<b>ล้างตระกร้า</b>
</a>
</div>
<table id="count" class="table table-bordered">
<tr align="center">
<th width="15%">รหัส</th>
<th width="45%">ชื่อ</th>
<th width="5%">จำนวน</th>
<th width="10%">หน่วย</th>
<th width="10%">ราคาต่อหน่วย</th>
<th width="10%">ราคารวม</th>
<th width="5%" class="noprint">ลบ</th>
</tr>
<?php
if(isset($_COOKIE["shopping_cart"])){
$total = 0;
$member_status = "RETAIL";
// $member_status = "WHOLESALE";
$cookie_data = stripslashes($_COOKIE['shopping_cart']);
$cart_data = json_decode($cookie_data, true);
if(is_array($cart_data) || is_object($cart_data)){
foreach($cart_data as $keys => $values){
$sql13 = "SELECT DISTINCT SPD.unit_price, PM.description FROM sale_pricelist_detail AS SPD WITH (NOLOCK)
INNER JOIN product_master AS PM WITH (NOLOCK) ON PM.item_no = SPD.item_no
WHERE SPD.client = '001' AND SPD.order_no = '".$member_status."' AND SPD.item_no = '".$values["item_code"]."' AND SPD.uom = '".$values["unit"]."'";
$query13 = sqlsrv_query($conn2, $sql13);
if($data13 = sqlsrv_fetch_array($query13)){
?>
<tr id="product">
<td><?php echo $values["item_code"]; ?></td>
<td><?php echo $data13["description"]; ?></td>
<td align="center"><?php echo number_format($values["qty"], 0, ".", ","); ?></td>
<td align="center"><?php echo $values["unit"]; ?></td>
<td align="center"><?php echo number_format($data13["unit_price"], 0, ".", ",")." บาท"; ?></td>
<td align="right"><?php echo number_format($values["qty"] * $data13["unit_price"], 0, ".", ",")." บาท"; ?></td>
<td class="noprint" align="center">
<a href="sales_order.php?action=delete&code=<?php echo $values["item_code"]; ?>&unit=<?php echo $values["unit"]; ?>">
<span class="text-danger">ลบ</span>
</a>
</td>
</tr>
<?php
$total = $total + ($values["qty"] * $data13["unit_price"]);
}//if
}//for
}//if
?>
<tr>
<td colspan="5" align="right">ยอดสุทธิ</td>
<td align="right"><?php echo number_format($total, 2, ".", ",")." บาท"; ?></td>
</tr>
<?php
}else{
echo '<tr><td colspan="5" align="center">ไม่มีสินค้าในตะกร้า</td></tr>';
}
//**************************************************************************************************************************************************//
?>
</table>
<div style="margin-top: 0px; align: center;">
<font name="remark" id="remark">หมายเหตุ :</font>
<textarea name="textarea" id="textarea" cols="100%" rows="1" style="border: none; overflow: hidden;"></textarea>
</div>
</div>
</div>
</div>
</body>
</html>
<script type="text/javascript">
//Auto Complete Textbox
$(function() {
$("#search_item").autocomplete({
source: "search_item.php",
minLength: 2,
select: function(event, ui) {
//assign value back to the form element
if(ui.item){
$(event.target).val(ui.item.value);
}
//submit from the selected
$(event.target.form).submit();
},
maxShowItems: 15
});
});
//Auto Complete Member
$(function() {
$("#member").autocomplete({
source: "search_member.php",
minLength: 2,
select: function(event, ui) {
if(ui.item){
$(event.target).val(ui.item.value);
}
//$(event.target.form).submit();
},
maxShowItems: 10
});
});
//Show-Hide stock
$("#show-stock").hover(function() {
$("#show-detail-stock").css({"display":"block"});
}, function() {
$("#show-detail-stock").css({"display":"none"});
});
//Show-Hide price
$("#show-price").hover(function() {
$("#show-detail-price").css({"display":"block"});
}, function() {
$("#show-detail-price").css({"display":"none"});
});
//Count Row
$(document).ready(function () {
var TableRowCount = null;
TableRowCount = $("#count tr:not(:first-child):not(:last-child)").length;
document.getElementById("span").innerHTML = TableRowCount;
});
</script>
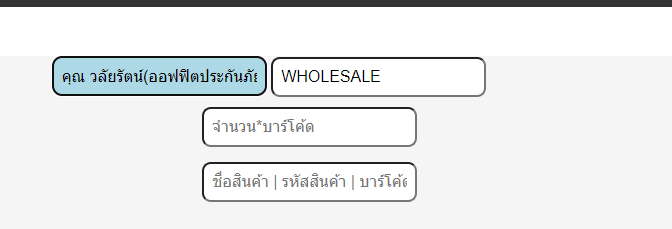
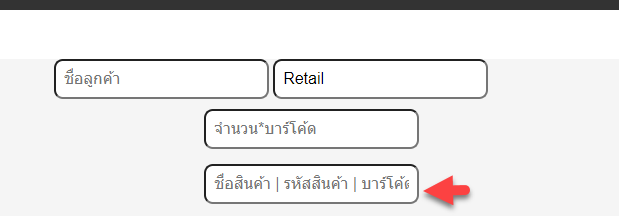
Tag : PHP
|
ประวัติการแก้ไข 2022-07-04 09:03:58
|
 |
 |
 |
 |
Date :
2022-07-04 08:45:06 |
By :
chayut095092 |
View :
579 |
Reply :
1 |
|
 |
 |
 |
 |
|
|
|
 |