 |
|
ผมให้ mainfrom โหลดขึ้นมาพร้อมแสดงข้อมูลใน datagridview ได้แล้ว
แต่ผมยังบันทึกข้อมูลไปยัง database ไม่ได้ครับ
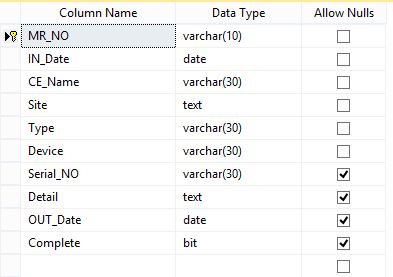
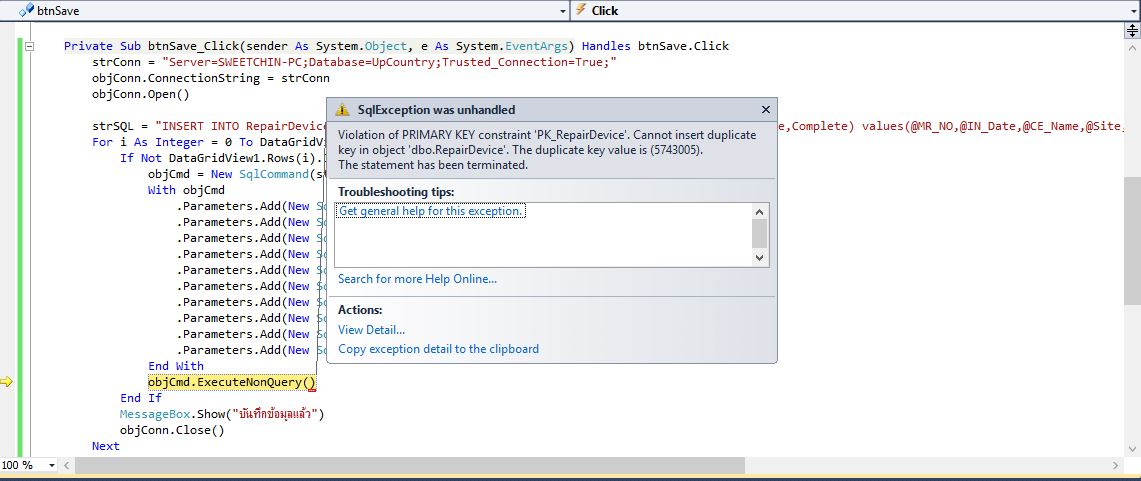
Code (VB.NET)
Public Class MainForm
Dim objConn As New SqlConnection
Dim objCmd As New SqlCommand
Dim strConn, strSQL As String
Dim da As New SqlDataAdapter
Dim dt As New DataTable
Private Sub MainForm_Load(sender As System.Object, e As System.EventArgs) Handles MyBase.Load
strConn = "Server=SWEETCHIN-PC;Database=UpCountry;Trusted_Connection=True;"
objConn.ConnectionString = strConn
objConn.Open()
strSQL = "SELECT * FROM RepairDevice"
objCmd = New SqlCommand(strSQL, objConn)
da.SelectCommand = objCmd
da.Fill(dt)
DataGridView1.DataSource = dt
da.Update(dt)
objConn.Close()
End Sub
Private Sub btnSave_Click(sender As System.Object, e As System.EventArgs) Handles btnSave.Click
strConn = "Server=SWEETCHIN-PC;Database=UpCountry;Trusted_Connection=True;"
objConn.ConnectionString = strConn
objConn.Open()
strSQL = "INSERT INTO RepairDevice(MR_NO,IN_Date,CE_Name,Site,Type,Device,Serial_NO,Detail,OUT_Date,Complete) values(@MR_NO,@IN_Date,@CE_Name,@Site,@Type,@Device,@Serial_NO,@Detail,@OUT_Date,@Complete)"
For i As Integer = 0 To DataGridView1.RowCount - 1
If Not DataGridView1.Rows(i).IsNewRow Then
objCmd = New SqlCommand(strSQL, objConn)
With objCmd
.Parameters.Add(New SqlParameter("@MR_NO", DataGridView1.Rows(i).Cells(0).Value))
.Parameters.Add(New SqlParameter("@IN_Date", DataGridView1.Rows(i).Cells(1).Value))
.Parameters.Add(New SqlParameter("@CE_Name", DataGridView1.Rows(i).Cells(2).Value))
.Parameters.Add(New SqlParameter("@Site", DataGridView1.Rows(i).Cells(3).Value))
.Parameters.Add(New SqlParameter("@Type", DataGridView1.Rows(i).Cells(4).Value))
.Parameters.Add(New SqlParameter("@Device", DataGridView1.Rows(i).Cells(5).Value))
.Parameters.Add(New SqlParameter("@Serial_NO", DataGridView1.Rows(i).Cells(6).Value))
.Parameters.Add(New SqlParameter("@Detail", DataGridView1.Rows(i).Cells(7).Value))
.Parameters.Add(New SqlParameter("@OUT_Date", DataGridView1.Rows(i).Cells(8).Value))
.Parameters.Add(New SqlParameter("@Complete", DataGridView1.Rows(i).Cells(9).Value))
End With
objCmd.ExecuteNonQuery()
End If
MessageBox.Show("บันทึกข้อมูลแล้ว")
objConn.Close()
Next
End Sub
End Class
Tag : .NET, Ms SQL Server 2012, VB.NET, VS 2010 (.NET 4.x)
|
|
 |
 |
 |
 |
Date :
2015-02-12 23:35:42 |
By :
sweetschin |
View :
1933 |
Reply :
6 |
|
 |
 |
 |
 |
|
|
|
 |