 |
|
เลือกข้อมมูล datagridviewcheckbox ไห้ไปแสดงบน Datagridview ต่างฟอร์ม |
|
 |
|
|
 |
 |
|
ประกาศตัวแปรเป็น Shared ก่อนครับ แล้วก็เก็บข้อมูล พอเปิดฟอร์มใหม่ก็เรียกผ่านตัวแปรที่ได้ทำการ Shared ไว้ครับ
|
 |
 |
 |
 |
Date :
2015-09-09 21:52:42 |
By :
Balll2iFFer |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
แล้วส่วนของโค๊ด ของ datagridviewcheckboxcolumn หละครับต้องทำอย่างไรบ้าง คืออยากให้มันเชคว่าตัวที่ติกตัวไหนไปบ้าง ถ้าติกข้อมูลก็จะย้ายไปอะครับ ถ้าไม่ติกคืออยู่ที่เดิม แล้ว การประกาศตัวแปร Shared พอจะมีตัวอย่างไหมครับ ขอบคุณครับ
|
 |
 |
 |
 |
Date :
2015-09-10 09:13:46 |
By :
phuriwat |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ต้องถามว่า ใช้อะไรเก็บข้อมูลครับ Data Table หรือ List
|
 |
 |
 |
 |
Date :
2015-09-10 09:27:11 |
By :
Freedom |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
นี่โค๊ดของผมครับ
Code (C#)
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data.SqlClient;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace test2
{
public partial class add_receive : Form
{
public add_receive()
{
InitializeComponent();
}
DataSet ds = new DataSet();
string cn = "Data Source=ADMIN;Initial Catalog=BTEC_MANAGEMENT;User ID=Programmer;Password=1234";
private void add_receive_Load(object sender, EventArgs e)
{
string sql = "select private_code, product_code, serial,name, unit_name, description, remark, add_date FROM products";
SqlDataAdapter da = new SqlDataAdapter(sql, cn);
da.Fill(ds, "products");
dataGridView1.DataSource = ds.Tables["products"];
dataGridView1.Columns.Add(new DataGridViewCheckBoxColumn {Name = "Status", HeaderText = "" });
}
private void simpleButton2_Click(object sender, EventArgs e)
{
this.Close();
}
private void dataGridView1_CellContentClick_1(object sender, DataGridViewCellEventArgs e)
{
if (e.RowIndex == -1)
return;
else
{
dataGridView1.Rows[e.RowIndex].Selected = true;
}
}
private void ok_receive_Click(object sender, EventArgs e)
{
}
}
}
|
 |
 |
 |
 |
Date :
2015-09-10 09:41:21 |
By :
phuriwat |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ถ้าจะให้ง่ายๆ เลยครับ เพิ่ม Status เข้าไปใน Data Table อีกตัวครับ เอาไว้เก็บสถานะการเซ็ค จาก Check box
พอเพิ่มแล้วเวลาใช้งาน ก็วนลูป for ปกติครับ แล้วเลือกเอาเฉพาะ Status = true เท่านั้นมาใช้งานครับ
|
 |
 |
 |
 |
Date :
2015-09-10 09:59:56 |
By :
Freedom |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ตอนนี้เชคคลิก ได้แล้วครับแต่ยังย้ายไม่ได้ พอจะมีโค๊ดตัวอย่างไหมครับ งงมากครับ อันนี้คือโค๊ดที่ได้ตอนนี้ครับ ไม่รู้ว่าโค๊ดถูกจุดไหมนะครับCode (C#)
private void ok_receive_Click(object sender, EventArgs e)
{
foreach (DataGridViewRow dr in dataGridView1.Rows)
{
if (dr.Cells[8].Value != null)
{
if(bool.Parse(dr.Cells[8].Value.ToString()))
{
//คำสั่งย้ายไป ฟอร์ม2
}
}
}
}
|
 |
 |
 |
 |
Date :
2015-09-10 10:52:42 |
By :
phuriwat |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ผมชอบใช้ยุสองแบบครับ
1.ผ่าน datagrid เลย
Code (C#)
private void dgvCri_CellMouseDoubleClick(object sender, DataGridViewCellMouseEventArgs e)
{
MessageBox.Show(dgvCri[0, e.RowIndex].Value.ToString());
//ส่งค่าออกไป
}
private void dgvCri_CellContentClick(object sender, DataGridViewCellEventArgs e)
{
MessageBox.Show(dgvCri[0, e.RowIndex].Value.ToString());
//ส่งค่าออกไป
}
2. เพิ่ม คอลัมอีกตัวเปน checkbok กับ ปุ่มกด
เลือกที่เราต้องการแล้วกดทีเดียว
Code (VB.NET)
private void ok_receive_Click(object sender, EventArgs e)
{
foreach (DataGridViewRow dr in dataGridView1.Rows)
{
if (dr.Cells[8].Value != null)
{
if(bool.Parse(dr.Cells[8].Value.ToString()))
{
//คำสั่งย้ายไป ฟอร์ม2
}
}
}
}
ขึ้นอยู่กับความสะดวก งานแต่ละงาน ครับ
|
 |
 |
 |
 |
Date :
2015-09-10 11:35:06 |
By :
lamaka.tor |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ขอโทษครับ ขอรบกวนอีกที คือผมยังทำไม่ได้เลยครับ ผมลองเปลี่ยนมาเป็น datatable แล้ว แต่ผมไม่รู้โค๊ดที่ต้อง ทำไห้ไปอีกฟอร์มนึงอะครับ
จาก การเลือกของ checkboxของdatagrid ไห้ข้อมูลที่ติกแล้วกด ok ไป แสดง อีกdatagrid ของคนละฟรอร์มครับ แล้วถ้า อีกฟอร์มcancle ข้อมูลจะกลับมาหน้า datagrid เดิมครับ
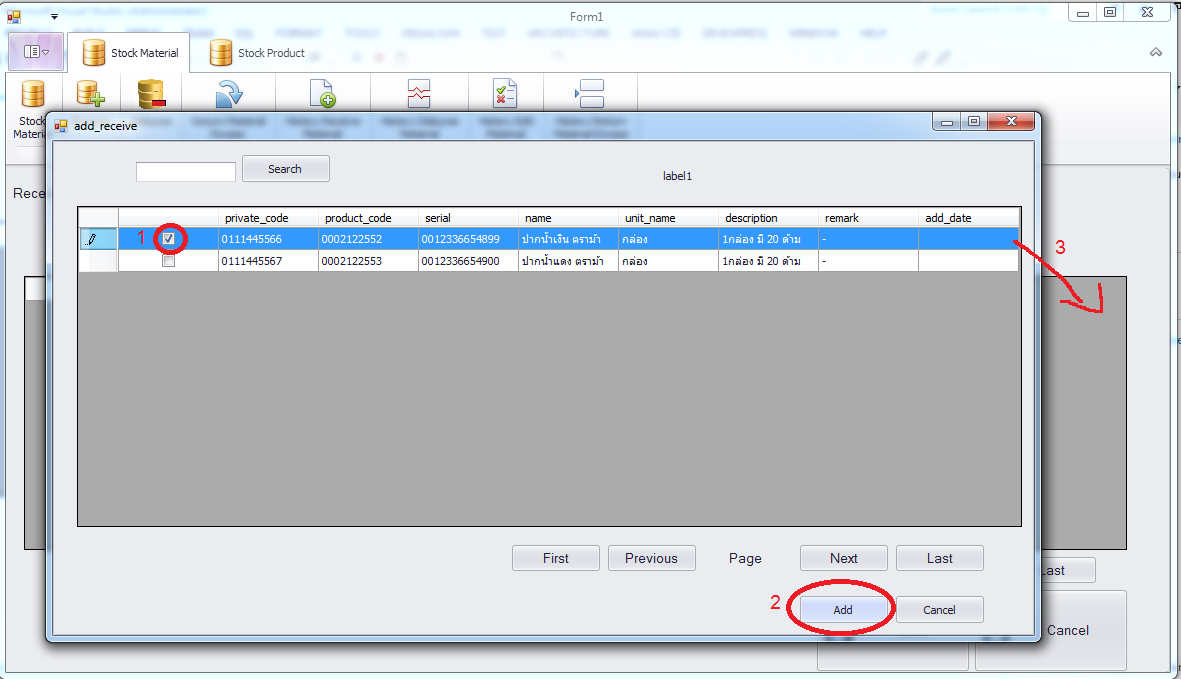
นี่คือโค๊ดตอนนี้ครับคือหน้าตามรูปCode (C#)
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data.SqlClient;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace test2
{
public partial class add_receive : Form
{
public add_receive()
{
InitializeComponent();
}
/*
DataSet ds = new DataSet();
string cn = "Data Source=ADMIN;Initial Catalog=BTEC_MANAGEMENT;User ID=Programmer;Password=1234";
*/
private void add_receive_Load(object sender, EventArgs e)
{ /*
string sql = "select private_code, product_code, serial,name, unit_name, description, remark, add_date FROM products";
SqlDataAdapter da = new SqlDataAdapter(sql, cn);
da.Fill(ds, "products");
dataGridView1.DataSource = ds.Tables["products"];
*/
SqlConnection conn = new SqlConnection("Data Source=ADMIN;Initial Catalog=BTEC_MANAGEMENT;User ID=Programmer;Password=1234");
SqlDataAdapter da = new SqlDataAdapter("select private_code, product_code, serial,name, unit_name, description, remark, add_date FROM products", conn);
DataTable dt = new DataTable();
da.Fill(dt);
foreach (DataRow item in dt.Rows)
{
int n = dataGridView1.Rows.Add();
dataGridView1.Rows[n].Cells[1].Value = item["private_code"].ToString();
dataGridView1.Rows[n].Cells[2].Value = item["product_code"].ToString();
dataGridView1.Rows[n].Cells[3].Value = item["serial"].ToString();
dataGridView1.Rows[n].Cells[4].Value = item["name"].ToString();
dataGridView1.Rows[n].Cells[5].Value = item["unit_name"].ToString();
dataGridView1.Rows[n].Cells[6].Value = item["description"].ToString();
dataGridView1.Rows[n].Cells[7].Value = item["remark"].ToString();
dataGridView1.Rows[n].Cells[8].Value = item["add_date"].ToString();
dataGridView1.Rows[n].Cells[0].Value = false;
}
}
private void simpleButton2_Click(object sender, EventArgs e)
{
this.Close();
}
private void ok_receive_Click(object sender, EventArgs e)
{
foreach (DataGridViewRow dr in dataGridView1.Rows)
{
if ((bool)dr.Cells[0].Value == true)
{
label1.Text = dr.Cells[0].RowIndex.ToString();
}
/* if (dr.Cells[0].Value != null)
{
if(bool.Parse(dr.Cells[0].Value.ToString()))
{
//คำสั่งย้ายไป ฟอร์ม2 ยังไม่ได้
}
}*/
}
}
private void dataGridView1_CellContentClick_1(object sender, DataGridViewCellEventArgs e)
{
if (e.RowIndex == -1)
return;
else
{
dataGridView1.Rows[e.RowIndex].Selected = true;
}
}
}
}
|
 |
 |
 |
 |
Date :
2015-09-11 10:56:57 |
By :
phuriwat |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
// Form1 (C#)
public partial class Form1 : Form
{
List<TestEntity> TestEntityList = new List<TestEntity>();
public Form1()
{
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e)
{
TestEntity TestEntity = new TestEntity();
TestEntity.Id = 1;
TestEntity.Name = "Test1";
TestEntity.Status = false;
TestEntityList.Add(TestEntity);
TestEntity = new TestEntity();
TestEntity.Id = 2;
TestEntity.Name = "Test2";
TestEntity.Status = false;
TestEntityList.Add(TestEntity);
TestEntity = new TestEntity();
TestEntity.Id = 3;
TestEntity.Name = "Test3";
TestEntity.Status = false;
TestEntityList.Add(TestEntity);
dataGridView1.DataSource = TestEntityList;
}
private void button1_Click(object sender, EventArgs e)
{
Form2 Form2 = new Form2();
Form2.testEntityList = TestEntityList.FindAll(x => x.Status == true);
Form2.ShowDialog();
}
}
public class TestEntity
{
public int Id { get; set; }
public string Name { get; set; }
public bool Status { get; set; }
}
// Form2(C#)
public partial class Form2 : Form
{
List<TestEntity> TestEntityList = new List<TestEntity>();
public List<TestEntity> testEntityList
{
get
{
return this.TestEntityList;
}
set
{
this.TestEntityList = value;
}
}
public Form2()
{
InitializeComponent();
}
private void Form2_Load(object sender, EventArgs e)
{
dataGridView1.DataSource = TestEntityList;
}
}
ลองเอาตัวอย่างด้านบนไปดูครับ ไม่มีไรยากเลย ใช้ Get Set ส่งค่าไปปกติครับ
|
 |
 |
 |
 |
Date :
2015-09-11 11:21:39 |
By :
Freedom |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ทำไม่ได้เลยครับ งง ครับ ไม่รู้จะต้องเริ่มตรงไหน ก่อน    
|
 |
 |
 |
 |
Date :
2015-09-11 14:06:14 |
By :
phuriwat |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ไม่ยากเลยครับ ไม่ได้มีไรเลย Copy โค้ด ไปแปะ Form 1 กับ Form 2 ก็ใ้ชงานได้แล้วอะ
|
 |
 |
 |
 |
Date :
2015-09-11 14:10:44 |
By :
Freedom |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
แปะตรงส่วนไหนบ้างครับ ฟอร์ม1 นี่คือส่วนของฟอร์มที่จะไปโชว์หรือป่าวครับ(ฟอร์มข้างล่างหรือป่าวครับ)
|
 |
 |
 |
 |
Date :
2015-09-11 14:32:08 |
By :
phuriwat |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
 |
|
|
 |
 |
|
ได้แล้วครับขอบคุณทุกคนมากครับ
|
 |
 |
 |
 |
Date :
2015-09-17 09:37:09 |
By :
phuriwat |
|
 |
 |
 |
 |
|
|
 |
 |
|
 |
 |
|
|