Java GUI Show Master-Detail from Database |
Java GUI Show Master-Detail from Database หัวข้อนี้จะเป็นการเขียน Java GUI เพื่ออ่านข้อมูลจากฐานข้อมูล Database มาแสดงในรูปแบบของ Master-Detail ซึ่งจะถูกแบ่งออกเป็น 2 Frame คือ Frame แรกจะแสดงข้อมูลบน JTable และ Frame ที่สองจะแสดง Modal Dialog รายละเอียดของข้อมูลที่ได้จากการคลิกข้อมูลในแต่ล่ะ Cell
แสดงข้อมูลใน JTable สามารถคลิกเพื่อดูรายละเอียดในแต่ล่ะ Rows
Java GUI Show Master-Detail from Database
ในตัวอย่างนี้จะเลือกใช้ Database ของ MySQL แต่ในกรณีที่จะใช้ร่วมกับ Database อื่น ๆ ก็สามารถทำได้ง่าย ๆ เพียงแค่เปลี่ยน Connector และ Connection String เท่านั้น และการแสดงข้อมูลจะใช้ JTable ของ Java Swing สามารถอ่านเพิ่มเติมได้ที่บทความนี้
Java Connect to MySQL Database (JDBC)
Java Table (JTable) - Swing Example
Java GUI Retrieve List Data from Database
โครงสร้างของ MySQL และ Table
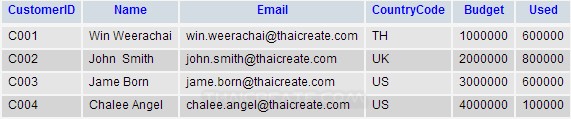
CREATE TABLE `customer` (
`CustomerID` varchar(4) NOT NULL,
`Name` varchar(50) NOT NULL,
`Email` varchar(50) NOT NULL,
`CountryCode` varchar(2) NOT NULL,
`Budget` double NOT NULL,
`Used` double NOT NULL,
PRIMARY KEY (`CustomerID`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
--
-- dump ตาราง `customer`
--
INSERT INTO `customer` VALUES ('C001', 'Win Weerachai', '[email protected]', 'TH', 1000000, 600000);
INSERT INTO `customer` VALUES ('C002', 'John Smith', '[email protected]', 'UK', 2000000, 800000);
INSERT INTO `customer` VALUES ('C003', 'Jame Born', '[email protected]', 'US', 3000000, 600000);
INSERT INTO `customer` VALUES ('C004', 'Chalee Angel', '[email protected]', 'US', 4000000, 100000);
คำสั่งของ MySQL สามารถนำไปรัน Query เพื่อสร้าง Table ได้ทันที
กลับมาที่ Java Project ซึ่งเราจะต้องสร้างไฟล์ Java ขึ้นมา 2 ไฟล์ โดยไฟล์แรกชื่อว่า MyForm.java ไว้แสดงข้อมูล
JTable table = new JTable();
table.addMouseListener(new MouseAdapter() {
@Override
public void mouseClicked(MouseEvent arg0) {
int index = table.getSelectedRow();
String CustomerID = table.getValueAt(index, 0).toString();
/*
String Name = table.getValueAt(index, 1).toString();
String Email = table.getValueAt(index, 2).toString();
String CountryCode = table.getValueAt(index, 3).toString();
String Budget = table.getValueAt(index, 4).toString();
String Used = table.getValueAt(index, 5).toString();
*/
MyDetail detail = new MyDetail(CustomerID);
detail.setModal(true);
detail.setVisible(true);
}
});
เป็นคำสั่งคลิกที่ Cell เพื่อส่งไปยัง Frame ที่สองที่เป็น Modal Dialog สามารถเขียน Code ทั้งหมดได้ดังนี้
MyForm.java
package com.java.myapp;
import java.awt.EventQueue;
import java.awt.event.MouseAdapter;
import java.awt.event.MouseEvent;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
import javax.swing.JOptionPane;
import javax.swing.JFrame;
import javax.swing.JTable;
import javax.swing.JScrollPane;
import javax.swing.table.DefaultTableModel;
public class MyForm extends JFrame {
/**
* Launch the application.
*/
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
MyForm frame = new MyForm();
frame.setVisible(true);
}
});
}
/**
* Create the frame.
*/
public MyForm() {
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setBounds(100, 100, 580, 278);
setTitle("ThaiCreate.Com Java GUI Tutorial");
getContentPane().setLayout(null);
// ScrollPane
JScrollPane scrollPane = new JScrollPane();
scrollPane.setBounds(64, 65, 440, 89);
getContentPane().add(scrollPane);
// Table
final JTable table = new JTable();
table.addMouseListener(new MouseAdapter() {
@Override
public void mouseClicked(MouseEvent arg0) {
int index = table.getSelectedRow();
String CustomerID = table.getValueAt(index, 0).toString();
/*
String Name = table.getValueAt(index, 1).toString();
String Email = table.getValueAt(index, 2).toString();
String CountryCode = table.getValueAt(index, 3).toString();
String Budget = table.getValueAt(index, 4).toString();
String Used = table.getValueAt(index, 5).toString();
*/
MyDetail detail = new MyDetail(CustomerID);
detail.setModal(true);
detail.setVisible(true);
}
});
scrollPane.setViewportView(table);
// Model for Table
DefaultTableModel model = (DefaultTableModel)table.getModel();
model.addColumn("CustomerID");
model.addColumn("Name");
model.addColumn("Email");
model.addColumn("CountryCode");
model.addColumn("Budget");
model.addColumn("Used");
Connection connect = null;
Statement s = null;
try {
Class.forName("com.mysql.jdbc.Driver");
connect = DriverManager.getConnection("jdbc:mysql://localhost/mydatabase" +
"?user=root&password=root");
s = connect.createStatement();
String sql = "SELECT * FROM customer ORDER BY CustomerID ASC";
ResultSet rec = s.executeQuery(sql);
int row = 0;
while((rec!=null) && (rec.next()))
{
model.addRow(new Object[0]);
model.setValueAt(rec.getString("CustomerID"), row, 0);
model.setValueAt(rec.getString("Name"), row, 1);
model.setValueAt(rec.getString("Email"), row, 2);
model.setValueAt(rec.getString("CountryCode"), row, 3);
model.setValueAt(rec.getFloat("Budget"), row, 4);
model.setValueAt(rec.getFloat("Used"), row, 5);
row++;
}
} catch (Exception e) {
// TODO Auto-generated catch block
JOptionPane.showMessageDialog(null, e.getMessage());
e.printStackTrace();
}
try {
if(s != null) {
s.close();
connect.close();
}
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
สร้างไฟล์ MyDetail.java สำหรับเป็น Dialog ไว้แสดงรายละเอียด
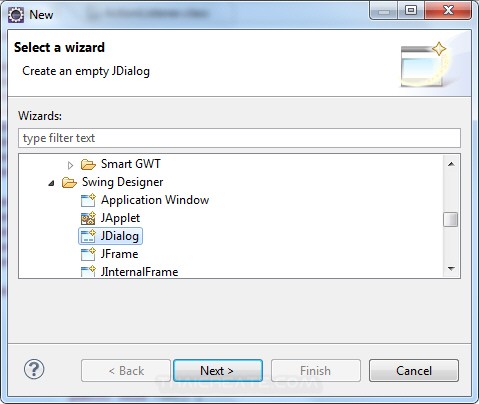
เลือก JDialog
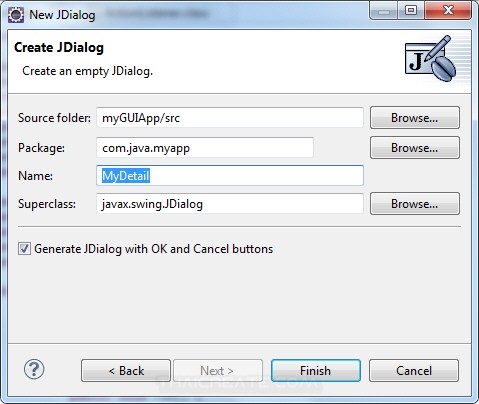
ตั้งชื่อเป็น MyDetail.java
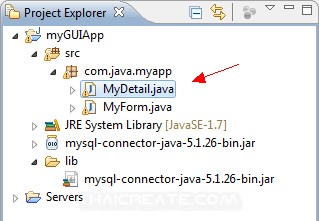
ได้ไฟล์ MyDetail.java ขึ้นมาเรียบร้อยแล้ว
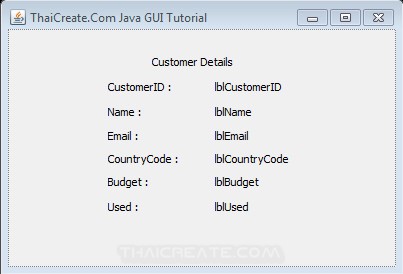
ตัวอย่างหน้า Design หรือจะเขียน Code ดังนี้
MyDetail.java
package com.java.myapp;
import javax.swing.JDialog;
import javax.swing.JLabel;
import javax.swing.JButton;
import java.awt.event.ActionListener;
import java.awt.event.ActionEvent;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
import javax.swing.JOptionPane;
public class MyDetail extends JDialog {
/**
* Create the dialog.
*/
public MyDetail(String sCustomerID) {
setTitle("ThaiCreate.Com Java GUI Tutorial");
setDefaultCloseOperation(JDialog.DISPOSE_ON_CLOSE);
setBounds(100, 100, 385, 266);
getContentPane().setLayout(null);
setResizable(false);
// Header Customer Detail
JLabel hCustomerDetail = new JLabel("Customer Details");
hCustomerDetail.setBounds(144, 21, 132, 14);
getContentPane().add(hCustomerDetail);
//*** Header ***//
JLabel hCustomerID = new JLabel("CustomerID :");
hCustomerID.setBounds(100, 51, 89, 14);
getContentPane().add(hCustomerID);
JLabel hName = new JLabel("Name :");
hName.setBounds(100, 76, 89, 14);
getContentPane().add(hName);
JLabel hEmail = new JLabel("Email :");
hEmail.setBounds(100, 100, 89, 14);
getContentPane().add(hEmail);
JLabel hCountryCode = new JLabel("CountryCode :");
hCountryCode.setBounds(100, 123, 89, 14);
getContentPane().add(hCountryCode);
JLabel hBudget = new JLabel("Budget :");
hBudget.setBounds(100, 146, 89, 14);
getContentPane().add(hBudget);
JLabel hUsed = new JLabel("Used :");
hUsed.setBounds(100, 171, 89, 14);
getContentPane().add(hUsed);
//*** Detail ***//
JLabel lblCustomerID = new JLabel("lblCustomerID");
lblCustomerID.setBounds(207, 51, 89, 14);
getContentPane().add(lblCustomerID);
JLabel lblName = new JLabel("lblName");
lblName.setBounds(207, 76, 89, 14);
getContentPane().add(lblName);
JLabel lblEmail = new JLabel("lblEmail");
lblEmail.setBounds(207, 100, 162, 14);
getContentPane().add(lblEmail);
JLabel lblCountryCode = new JLabel("lblCountryCode");
lblCountryCode.setBounds(207, 123, 89, 14);
getContentPane().add(lblCountryCode);
JLabel lblBudget = new JLabel("lblBudget");
lblBudget.setBounds(207, 146, 89, 14);
getContentPane().add(lblBudget);
JLabel lblUsed = new JLabel("lblUsed");
lblUsed.setBounds(207, 171, 89, 14);
getContentPane().add(lblUsed);
//*** Bind Data ***//
Connection connect = null;
Statement s = null;
try {
Class.forName("com.mysql.jdbc.Driver");
connect = DriverManager.getConnection("jdbc:mysql://localhost/mydatabase" +
"?user=root&password=root");
s = connect.createStatement();
String sql = "SELECT * FROM customer " +
"WHERE CustomerID = '" + sCustomerID + "' ";
ResultSet rec = s.executeQuery(sql);
if(rec != null) {
rec.next();
lblCustomerID.setText(rec.getString("CustomerID"));
lblName.setText(rec.getString("Name"));
lblEmail.setText(rec.getString("Email"));
lblCountryCode.setText(rec.getString("CountryCode"));
lblBudget.setText(rec.getString("Budget"));
lblUsed.setText(rec.getString("Used"));
}
rec.close();
} catch (Exception e) {
// TODO Auto-generated catch block
JOptionPane.showMessageDialog(null, e.getMessage());
e.printStackTrace();
}
try {
if(s != null) {
s.close();
connect.close();
}
} catch (SQLException e) {
// TODO Auto-generated catch block
System.out.println(e.getMessage());
e.printStackTrace();
}
// Close Button
JButton btnClose = new JButton("Close");
btnClose.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
dispose();
}
});
btnClose.setBounds(161, 204, 69, 23);
getContentPane().add(btnClose);
}
}
Output
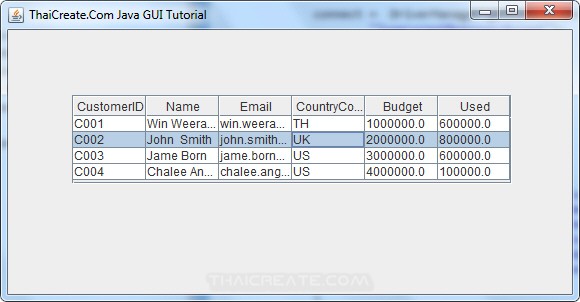
แสดงข้อมูลใน Frame แรกบน JTable สามารถคลิกที่ Rows เพื่อสดงรายละเอียด
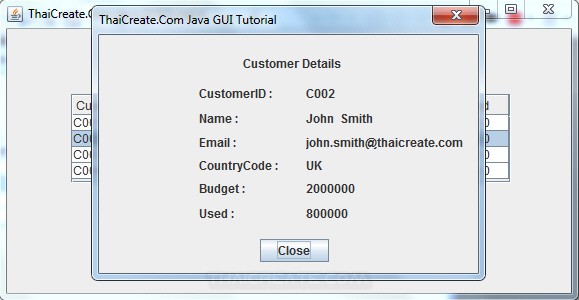
แสดงรายละเอียดใน Frame ที่สองในรูปแบบของ Modal Dialog
กรณีที่ใช้ร่วมกับ Database อื่น ๆ สามารถดูวิธีการใช้ Connector และ Connection String ได้ที่นี่
Property & Method (Others Related) |
|