|
jQery Cascading dropdown list / select menu (Dependent Dropdown list) |
jQuery cascading dropdown list "Dependent Dropdown list"
This code written by Nautilus.
README.md
Options
- url
A string containing the URL to which the request is sent.
Default: "json.php?val="
- parent
Parent drop down list.
Default: null
- child
Child drop down list.
Default: null
- initialText
Set initial text in option.
Deafault: "-- Please Select --"
- selectedOption
Set selected option of dropdown list.
Default: "-1"
- waitMsg
The message and indicator display before server sent respons.
Default: "Loading..."
- loadingImg
Set loading image.
Default: "images/loading4.gif"
- disabled
True -> set disable dropdownlist while dropdown list value is -1.
Default: true
How to Use.
Parte 1 - Setup
jquery cascading plugin uses the jquery JavaScript library, only. So, include just these two javascript files in your header.
<script type="text/javascript" src="js/jquery.min.js"></script>
<script type="text/javascript" src="js/jquery.cascading.min.js"></script>
Include the CSS file responsible to style the jquery cascading plugin.
<link rel="stylesheet" href="css/style.css" type="text/css">
Part 2 - Activate
See some examples:
$(function(){
$("#model").cascading( { parent:"#brand", child: ["#model_des", "#model_det", "#model_dett"] } );
$("#model_des").cascading( { parent:"#model", child: ["#model_det", "#model_dett"] } );
$("#model_det").cascading( { parent:"#model_des", child: "#model_dett" } );
$("#model_dett").cascading( { parent:"#model_det" } );
});
index.php
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset=utf-8>
<title>Cascading dropdown car model</title>
<link rel="stylesheet" href="css/style.css" type="text/css">
<script type="text/javascript" src="js/jquery.min.js"></script>
<script type="text/javascript" src="js/jquery.cascading.min.js"></script>
<script type="text/javascript">
// Specify a function to execute when the DOM is fully loaded.
$(function(){
$("#model").cascading( { parent:"#brand", child: ["#model_des", "#model_det", "#model_dett"] } );
$("#model_des").cascading( { parent:"#model", child: ["#model_det", "#model_dett"] } );
$("#model_det").cascading( { parent:"#model_des", child: "#model_dett" } );
$("#model_dett").cascading( { parent:"#model_det" } );
});
</script>
</head>
<body>
<div id="stylized" class="myform">
<form id="form" name="form" method="post" action="">
<h1>Cascading dropdown with jQuery</h1>
<p>This code wrote by Nautilus.</p>
<label>Brand
<span class="small">Select Brand</span>
</label>
<select id="brand" name="brand">
<option value="-1"> -- Please Select -- </option>
<?php
$brand = array(
'honda' => 'HONDA',
'toyota' => 'TOYOTA',
'mazda' => 'MAZDA',
'bmw' => 'BMW'
);
foreach ($brand as $k => $v ) {
echo '<option value="',$k, '">', $v, '</option>';
}
?>
</select>
<label>Model
<span class="small">Select Model</span>
</label>
<select id="model" name="model" disabled="disabled">
<option value="-1"> -- Please Select -- </option>
</select>
<label>Model Description
<span class="small">Select Model Description</span>
</label>
<select id="model_des" name="model_des" disabled="disabled">
<option value="-1"> -- Please Select -- </option>
</select>
<label>Model detail
<span class="small">Select Model detail</span>
</label>
<select id="model_det" name="model_det" disabled="disabled">
<option value="-1"> -- Please Select -- </option>
</select>
<label>Model detail tt
<span class="small">Select Model detail tt</span>
</label>
<select id="model_dett" name="model_dett" disabled="disabled">
<option value="-1"> -- Please Select -- </option>
</select>
<button type="submit" name="submit">Submit</button>
<div class="spacer"></div>
</form>
<?php
if(isset($_POST['submit'])) {
echo '<span>Brand: ', $_POST['brand'], '</span><br />';
echo '<span>Model: ', $_POST['model'], '</span><br />';
echo '<span>Model_des: ', $_POST['model_des'], '</span><br />';
echo '<span>Model_det: ', $_POST['model_det'], '</span><br />';
echo '<span>Model_dett: ', $_POST['model_dett'], '</span><br />';
}
?>
</div>
</body>
</html>
jquery.jqcascading.js
/**
* jQuery jqcascading Plugin
* Narong Rammanee
* [email protected]
*
* Latest Release: April 2012
*/
(function ($) {
// Cascading methods.
var cascading = {
init : function (options) {
return this.each(function () {
var self, settings;
self = $(this); // Self dropdown list.
settings = $.extend({
url : "json.php?val=", // Set URL.
parent : null, // Parent dropdown list.
child : null, // Set child.
initialText : "-- Please Select --", // Set initial text in option.
selectedOption : "-1", // Set selected option.
waitMsg : "Loading...", // Set wait message.
loadingImg : "images/loading4.gif", // Set loading image.
disabled : true // Set disabled dropdown list.
}, options);
$(settings.parent).bind('change', function () {
// Call method chage when dropdown list is changed.
cascading.change(self, settings);
});
});
},
change : function (self, settings) {
var parent, url, defaultOption, selectedOption, child, waitMsg,
disabled, loadingImg, parentValue, wait, loading;
parent = $(settings.parent);
if (parent) {
url = settings.url;
selectedOption = settings.selectedOption;
child = settings.child;
waitMsg = settings.waitMsg;
initialText = settings.initialText
disabled = settings.disabled;
loadingImg = settings.loadingImg;
parentValue = parent.val(); // Get parent value.
wait = $("<em />"); // Create em element contain loading.
loading = $("<img />").attr({ src: loadingImg, alt: waitMsg }); //Set loading image.
newOption = $("<option></option>"); // Create new option
defaultOption = newOption.val("-1").text(initialText); // Set default option.
$.ajax({
type: "GET",
dataType: "json",
url: url + parentValue + '&self=' + self.attr('id'),
beforeSend: function () {
// Show loading image & message
wait.remove()
.html(loading)
.append(waitMsg)
.appendTo("h1");
},
success: function (json) {
// Set default option & disabled dropdown list.
self.html(defaultOption.clone())
.removeAttr("disabled", "disabled");
if (json) {
// Loop json object.
$.each(json, function (value, text) {
// Append option to dropdown list.
self.append(newOption.clone().val(value).text(text));
});
}
// Remove waiting image & message
wait.empty();
// Set selected option
if (selectedOption != "-1") {
self.val(selectedOption);
}
// Disabled self dropdown list.
if (disabled && parentValue == "-1") {
self.attr("disabled", "disabled");
}
// Reset chain
cascading.resetChain(defaultOption, child, disabled);
}
});
}
},
resetChain : function (defaultOption, child, disabled) {
// If child is null.
if (!child) {
return;
}
// If child is object.
if (typeof child === "object") {
// Loop disabled child dropdown list.
$.each(child, function (index, value) {
$(value).html(defaultOption.clone());
if (disabled) {
$(value).attr("disabled", "disabled");
}
});
} else {
$(child).html(defaultOption.clone());
if (disabled) {
$(child).attr("disabled", "disabled");
}
}
}
};
$.fn.cascading = function () {
return cascading.init.apply(this, arguments);
};
}(jQuery));
json.php
<?php
/*!
* Json cascading Plugin
* Narong Rammanee
* [email protected]
*
* Latest Release: April 2012
*/
// Self dropdown list.
$self = isset($_GET['self']) ? $_GET['self'] : '';
$data = array();
switch($self) {
case 'model':
$brand = isset($_GET['val']) ? $_GET['val'] : '';
$model = array(
'honda' => array('accord' => 'ACCORD', 'civic' => 'CVIC'),
'mazda' => array('mazda_2' => 'mazda 2', 'mazda_3' => 'mazda 3'),
'toyota' => array('camry' => 'CAMRY', 'altis' => 'ALTIS')
);
$data = $model[$brand];
break;
case 'model_des':
$model = isset($_GET['val']) ? $_GET['val'] : '';
$model_des = array(
'accord' => array(
'2.0 I-VTEC E', '2.0 I-VTEC E White', '2.0 I-VTEC EL', '2.0 I-VTEC EL E White',
'2.0 I-VTEC EL NAVI', '2.0 I-VTEC EL NAVI', '2.4 I-VTEC EL', '2.4 I-VTEC EL White',
'2.4 I-VTEC EL NAVI', '2.4 I-VTEC EL NVI White', '3.6 I-VTEC V6', '3.6 I-VTEC V6 White'
),
'civic' => array(
'1.8 I-VTEC E AT (AS)', '1.8 I-VTEC S MT', '1.8 I-VTEC S AT',
'1.8 I-VTEC S AT (AS)', '1.8 I-VTEC E AT (AS)', '1.8 I-VTEC E AT',
'1.8 I-VTEC (AS) NAVI', '2.0 I-VTEC EL AT(AS)', '2.0 I-VTEC EL AT(AS)',
'2.0 I-VTEC NAVI'
),
'mazda_2' => array('Mazda 2 Elegance', ' Mazda 2 Sports'),
'mazda_3' => array('Mazda 3'),
'camry' => array('2.5 HV Navigator', '2.5 HV DVD', '2.5 HV CD', '2.5G', '2.0G'),
'altis' => array(
'2.0V Navi A/T', '2.0V A/T', '2.0G A/T', '1.8G A/T', '1.8E A/T',
'1.8E 50th Anniversary', '1.6G A/T', '1.6E CNG A/T', '1.6E A/T',
'1.6J A/T', '1.6J M/T', '1.6 CNG M/T'
)
);
$data = $model_des[$model];
break;
case 'model_det':
$model_des = isset($_GET['val']) ? $_GET['val'] : '';
$model_det = array(array('det_aa','det_bb'),array('det_yy', 'det_zz'));
$data = $model_det[$model_des];
break;
case 'model_dett':
$model_det = isset($_GET['val']) ? $_GET['val'] : '';
$model_dett = array(array('dett_aa','dett_bb'),array('dett_yy', 'dat_zz'));
$data = $model_dett[$model_det];
break;
}
// Print the JSON representation of a value
echo json_encode($data);
|
|
|
|
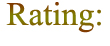 |
|