|
การจัดการไฟล์ NCN Karaoke แบบบ้านๆ |
การจัดการไฟล์ NCN Karaoke แบบบ้านๆ
จากบทความเรื่อง
โค้ดสำหรับโหลดไฟล์จากเว็บ one2up แบบบ้านๆ
https://www.thaicreate.com/community/load-one2up-ban-ban/view.html
ซึ่งทำให้ผมได้ NCN Karaoke มาเป็นที่เรียบร้อย
ก็มาถึงคราวที่จะนำ NCN Karaoke มาจัดเรียงใหม่ครับ
ครั้นจะก๊อบวางเฉย ๆ ก็แน่อยู่แล้วว่าต้องเละแน่ครับ
NCN มีไฟล์ที่เกี่ยวข้องด้วยกันอยู่ 3 ไฟล์ คือ
.LYR ในโฟลเดอร์ Lyrics
.CUR ในโฟลเดอร์ Cursor
และ .MID ในโฟลเดอร์ Song

ก๊อบวางเฉย ๆ ก็อาจจะทำให้ไม่สมบุรณ์ได้ อย่างเพลงมีแค่ .LYR กับ .CUR แต่ไม่มี .MID
มันก็จะทำให้รกเครื่องปล่าวๆ ครับ
เอาละครับหลักการบ้านๆที่ผมทำคือ
เอามาจัดเรียงใหม่โดยเลือกเพลงที่ครบที่ 3 ไฟล์เท่านั้น
1. List ไฟล์ ออกมาเก็บไว้ก่อนในที่นี้ผมจะ List เป็น .LYR ใครจะใช้ .CUR หรือ .MID ก็ได้นะครับ
2. สร้างโฟลเดอร์ขึ้นมาเก็บไฟล์ต่างๆ
3. วน loop เพื่อจัดการไฟล์ต่างๆ
ออกแบบหน้าตาประมาณนี้ครับ บ้านๆดี
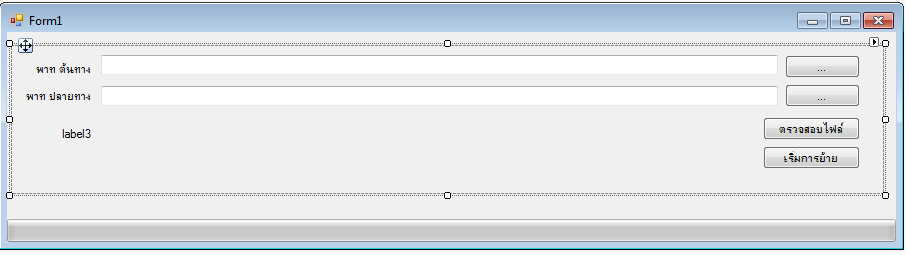
ส่วนโค้ดก็ประมาณนี้ครับ
Code (C#)
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace NCN_Mng
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
List<string> lstLYR;
//Symbol สำหรับใช้ ตั้งชื่อ โฟลเดอร์
string[] Symbol = new string[] { "0", "1", "2", "3", "4", "5", "6", "7", "8", "9", "A", "B", "C", "D", "E", "F", "G", "H", "I", "J", "K", "L", "M", "N", "O", "P", "Q", "R", "S", "T", "U", "V", "W", "X", "Y", "Z" };
private void button1_Click(object sender, EventArgs e)
{
FolderBrowserDialog fol = new FolderBrowserDialog();
fol.ShowDialog();
textBox1.Text = fol.SelectedPath;
}
private void button2_Click(object sender, EventArgs e)
{
FolderBrowserDialog fol = new FolderBrowserDialog();
fol.ShowDialog();
textBox2.Text = fol.SelectedPath;
}
private void button3_Click(object sender, EventArgs e)
{
lstLYR = System.IO.Directory.GetFiles(textBox1.Text, "*.LYR", System.IO.SearchOption.AllDirectories).ToList<string>();
label3.Text = "file LYR ทั้งหมด " + (lstLYR.Count-1) + " ไฟล์.";
progressBar1.Maximum = lstLYR.Count - 1;
}
private void button4_Click(object sender, EventArgs e)
{
new System.Threading.Thread(SetSongNCN).Start();
}
void SetSongNCN()
{
//สร้าง folder Cursor,Lyrics,Song ใน folder ปลายทาง
int iSymbol = 0,nsong = 0;
foreach (string s in Symbol)
{
System.IO.Directory.CreateDirectory(textBox2.Text + "\\Cursor\\" + s);
System.IO.Directory.CreateDirectory(textBox2.Text + "\\Lyrics\\" + s);
System.IO.Directory.CreateDirectory(textBox2.Text + "\\Song\\" + s);
}
string s_S = "", t_S = "", s_L = "", t_L = "", s_C = "", t_C = "";//ไฟล์ ต่างๆ ที่ต้องใช้งาน
//วน loop เพื่อจัดการไฟล์ ใน lstLYR
for (int i = 0; i < lstLYR.Count - 1; i++)
{
//ตั้งค่าไฟล์ต่างๆในต้นทาง
s_L = lstLYR[i].ToLower();
s_S = s_L.Replace("\\lyrics\\", "\\song\\").Replace(".lyr", ".mid");
s_C = s_L.Replace("\\lyrics\\", "\\cursor\\").Replace(".lyr", ".cur");
//ตั้งค่าไฟล์ต่างๆในปลายทางหากมีแล้วก็ให้ข้าม ID นั้น
if (System.IO.File.Exists(s_C) && System.IO.File.Exists(s_S))
{
do
{
if (nsong > 20000) { iSymbol++; nsong = 0; }//ถ้าใน folder นั้นมีเพลงเกิน 20000 ก็ให้เปลี่ยนไปใช้ folder ถัดไป
t_S = textBox2.Text + "\\Song\\" + Symbol[iSymbol] + "\\" + Symbol[iSymbol] + string.Format("{0:00000}", nsong) + ".mid";
t_C = textBox2.Text + "\\Cursor\\" + Symbol[iSymbol] + "\\" + Symbol[iSymbol] + string.Format("{0:00000}", nsong) + ".cur";
t_L = textBox2.Text + "\\Lyrics\\" + Symbol[iSymbol] + "\\" + Symbol[iSymbol] + string.Format("{0:00000}", nsong) + ".lyr";
nsong++;
} while (System.IO.File.Exists(t_L) || System.IO.File.Exists(t_C) || System.IO.File.Exists(t_S));
//ย้ายไฟล์ s_S s_L s_C ไปยัง t_S t_L t_C
//บางครั้งการย้ายอาจจะมีปัญหาก็อย่าไปสนใจมัน 5555
try { System.IO.File.Move(s_S, t_S); System.IO.File.Move(s_C, t_C); System.IO.File.Move(s_L, t_L); }
catch { }
}
try
{
// ตั้งค่า ให้รับค่าปัจจุบัน
this.Invoke(new Action(() => { this.Text = "Check File " + System.IO.Path.GetFileName(s_L); }));
label3.Invoke(new Action(() => { label3.Text = "Check File " + s_L; }));
progressBar1.Invoke(new Action(() => { progressBar1.Value =i; }));
}
catch { }
System.Threading.Thread.Sleep(50);
}
}
}
}
ว่าแล้วก็มาลองดูผลงานดีกว่าครับ
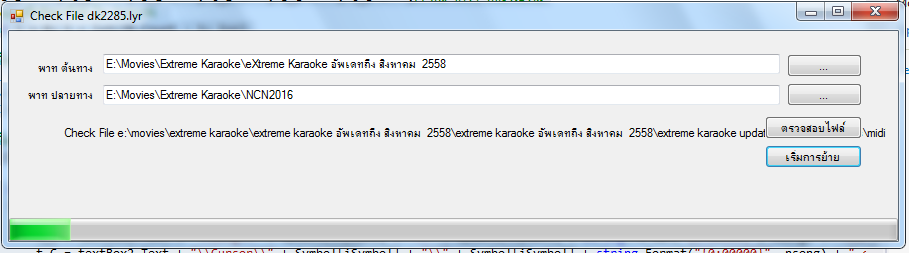
หน้าตาบ้านๆแต่ก็พอใช้งานได้ครับ
ส่วนไฟล์ .EMK ก็ใช้หลักการเดียวกันได้ครับ
ว่าแล้วก็ลงมือตกแต่งเลยครับ
ได้โค้ดบ้านๆแบบนี้ครับ
Code (C#)
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace NCN_Mng
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
List<string> lstLYR;
List<string> lstEMK;
//Symbol สำหรับใช้ ตั้งชื่อ โฟลเดอร์
string[] Symbol = new string[] { "0", "1", "2", "3", "4", "5", "6", "7", "8", "9", "A", "B", "C", "D", "E", "F", "G", "H", "I", "J", "K", "L", "M", "N", "O", "P", "Q", "R", "S", "T", "U", "V", "W", "X", "Y", "Z" };
private void button1_Click(object sender, EventArgs e)
{
FolderBrowserDialog fol = new FolderBrowserDialog();
fol.ShowDialog();
textBox1.Text = fol.SelectedPath;
}
private void button2_Click(object sender, EventArgs e)
{
FolderBrowserDialog fol = new FolderBrowserDialog();
fol.ShowDialog();
textBox2.Text = fol.SelectedPath;
}
private void button3_Click(object sender, EventArgs e)
{
lstLYR = System.IO.Directory.GetFiles(textBox1.Text, "*.LYR", System.IO.SearchOption.AllDirectories).ToList<string>();
lstEMK = System.IO.Directory.GetFiles(textBox1.Text, "*.EMK", System.IO.SearchOption.AllDirectories).ToList<string>();
label3.Text = "file LYR ทั้งหมด " + (lstLYR.Count - 1) + " ไฟล์." + Environment.NewLine + "file EMK ทั้งหมด " + (lstEMK.Count - 1) + " ไฟล์.";
progressBar1.Maximum = lstLYR.Count - 1;
progressBar2.Maximum = lstEMK.Count - 1;
}
private void button4_Click(object sender, EventArgs e)
{
new System.Threading.Thread(SetSongNCN).Start();
new System.Threading.Thread(SetSongEMK).Start();
}
void SetSongNCN()
{
//สร้าง folder Cursor,Lyrics,Song ใน folder ปลายทาง
int iSymbol = 0, nsong = 0;
foreach (string s in Symbol)
{
System.IO.Directory.CreateDirectory(textBox2.Text + "\\Cursor\\" + s);
System.IO.Directory.CreateDirectory(textBox2.Text + "\\Lyrics\\" + s);
System.IO.Directory.CreateDirectory(textBox2.Text + "\\Song\\" + s);
}
string s_S = "", t_S = "", s_L = "", t_L = "", s_C = "", t_C = "";//ไฟล์ ต่างๆ ที่ต้องใช้งาน
//วน loop เพื่อจัดการไฟล์ ใน lstLYR
for (int i = 0; i < lstLYR.Count - 1; i++)
{
//ตั้งค่าไฟล์ต่างๆในต้นทาง
s_L = lstLYR[i].ToLower();
s_S = s_L.Replace("\\lyrics\\", "\\song\\").Replace(".lyr", ".mid");
s_C = s_L.Replace("\\lyrics\\", "\\cursor\\").Replace(".lyr", ".cur");
//ตั้งค่าไฟล์ต่างๆในปลายทางหากมีแล้วก็ให้ข้าม ID นั้น
if (System.IO.File.Exists(s_C) && System.IO.File.Exists(s_S))
{
do
{
if (nsong > 20000) { iSymbol++; nsong = 0; }//ถ้าใน folder นั้นมีเพลงเกิน 20000 ก็ให้เปลี่ยนไปใช้ folder ถัดไป
t_S = textBox2.Text + "\\Song\\" + Symbol[iSymbol] + "\\" + Symbol[iSymbol] + string.Format("{0:00000}", nsong) + ".mid";
t_C = textBox2.Text + "\\Cursor\\" + Symbol[iSymbol] + "\\" + Symbol[iSymbol] + string.Format("{0:00000}", nsong) + ".cur";
t_L = textBox2.Text + "\\Lyrics\\" + Symbol[iSymbol] + "\\" + Symbol[iSymbol] + string.Format("{0:00000}", nsong) + ".lyr";
nsong++;
} while (System.IO.File.Exists(t_L) || System.IO.File.Exists(t_C) || System.IO.File.Exists(t_S));
//ย้ายไฟล์ s_S s_L s_C ไปยัง t_S t_L t_C
//บางครั้งการย้ายอาจจะมีปัญหาก็อย่าไปสนใจมัน 5555
try { System.IO.File.Move(s_S, t_S); System.IO.File.Move(s_C, t_C); System.IO.File.Move(s_L, t_L); }
catch { }
}
try
{
// ตั้งค่า ให้รับค่าปัจจุบัน
label4.Invoke(new Action(() => { label4.Text = "Move" + s_L + Environment.NewLine + t_L; }));
progressBar1.Invoke(new Action(() => { progressBar1.Value = i; }));
}
catch { }
System.Threading.Thread.Sleep(50);
}
}
void SetSongEMK()
{
//สร้าง folder EMK ใน folder ปลายทาง
int iSymbol = 0,nsong = 0;
foreach (string s in Symbol)
{
System.IO.Directory.CreateDirectory(textBox2.Text + "\\EMK\\" + s);
}
string s_E = "", t_E = "";//ไฟล์ ต่างๆ ที่ต้องใช้งาน
//วน loop เพื่อจัดการไฟล์ ใน lstEMK
for (int i = 0; i < lstEMK.Count - 1; i++)
{
//ตั้งค่าไฟล์ต่างๆในต้นทาง
s_E = lstEMK[i].ToLower();
//ตั้งค่าไฟล์ต่างๆในปลายทางหากมีแล้วก็ให้ข้าม ID นั้น
do
{
if (nsong > 20000) { iSymbol++; nsong = 0; }//ถ้าใน folder นั้นมีเพลงเกิน 20000 ก็ให้เปลี่ยนไปใช้ folder ถัดไป
t_E = textBox2.Text + "\\EMK\\" + Symbol[iSymbol] + "\\" + Symbol[iSymbol] + string.Format("{0:00000}", nsong) + ".EMK";
nsong++;
} while ( System.IO.File.Exists(t_E));
//ย้ายไฟล์ s_E ไปยัง t_E
//บางครั้งการย้ายอาจจะมีปัญหาก็อย่าไปสนใจมัน 5555
try { System.IO.File.Move(s_E, t_E); }
catch { }
try
{
// ตั้งค่า ให้รับค่าปัจจุบัน
label5.Invoke(new Action(() => { label5.Text = "Move" + s_E + Environment.NewLine + t_E; }));
progressBar2.Invoke(new Action(() => { progressBar2.Value =i; }));
}
catch { }
System.Threading.Thread.Sleep(50);
}
}
}
}
หน้าตาก็แบบนี้ครับ
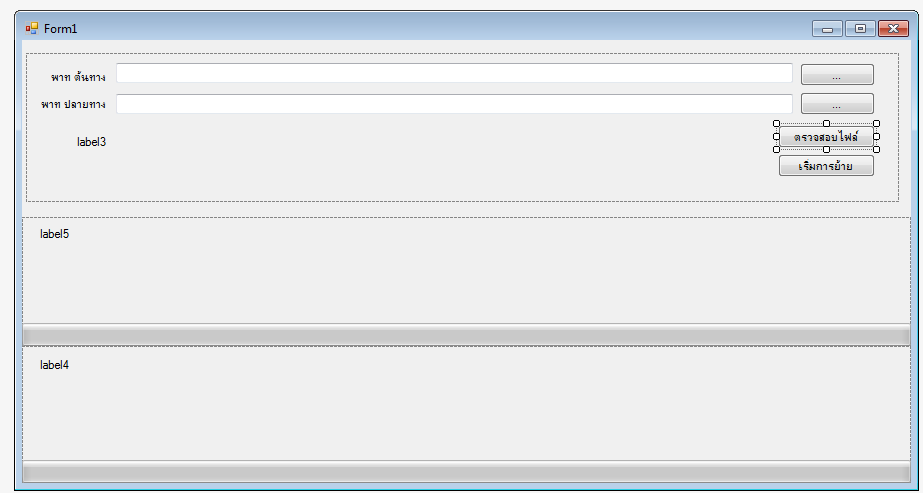
พอรันออกมาก็เป็นแบบนี้ครับ
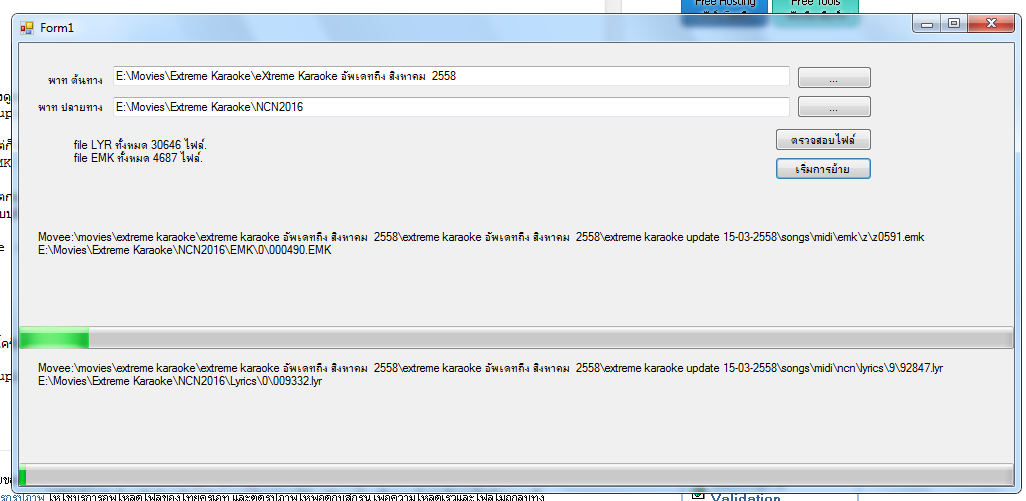
ที่นี้จะรอช้าอยู่ทำไมละครับท่านใดที่มี Extreme Karaoke อยู่ในคอมอย่าปล่ายให้มันรกพื้นที่อยู่ครับ
เอามันออกมายำได้แล้ว
แต่ก่อนอื่นแนะนำให้ image เป็น .iso ไว้ก่อนนะครับ จะได้ไม่เสียดายหากทำแล้วมันยิ่งเละไปกันใหญ่
ปล. ต่อไปก็เป็นการตัดไฟล์ซ้ำกันนะครับ
|
|
|
|
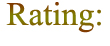 |
|