|
PHP กับ MySQLi การสร้าง JSON จาก MySQL Database ด้วยฟังก์ชั่น (function) ของ mysqli |
PHP กับ MySQLi การสร้าง JSON จาก MySQL Database ด้วยฟังก์ชั่น (function) ของ mysqli บทความนี้เป็นการอัพเดด Code ในการเข้ารหัส JSON จาก MySQL Database ด้วยฟังก์ชั่น (function) ของ mysqli ซึ่งเป็นชุดคำสั่งล่าสุดที่ทาง php แนะนำให้ใช้ในการติดต่อกับ Database ของ MySQL ส่วนคำสั่งในการเข้ารหัสเรายังคงใช้ json_encode() และกรณีจะ Decode ก็สามารถใช้ json_decode() ได้ แต่ถ้านำไปใช้งานกับ Application อื่น ๆ ด้วยการรับส่งผ่าน API ไปยัง Application ประเภท Android, iOS , Windows Phone ก็จะต้องใช้ชุดคำสั่งของภาษานั้น ๆ (Native) ในการที่จะ Decode ค่าตัวแปร JSON แล้วค่อยนำไปใช้งาน
PHP และ JSON กับ Web Service การรับส่งข้อมูลจาก MySQL ในรูปแบบของ JSON
jQuery Ajax กับ JSON ทำความเข้าใจ การรับส่งข้อมูล JSON ผ่าน jQuery กับ Ajax
ก่อนอ่านบทความนี้ควรอ่าน 3 บทความนี้ก่อน
head]ฐานข้อมูลฝั่ง Server[/head]
CREATE TABLE IF NOT EXISTS `customer` (
`CustomerID` varchar(4) NOT NULL,
`Name` varchar(50) NOT NULL,
`Email` varchar(50) NOT NULL,
`CountryCode` varchar(2) NOT NULL,
`Budget` decimal(18,2) NOT NULL,
`Used` decimal(18,2) NOT NULL
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
--
-- Dumping data for table `customer`
--
INSERT INTO `customer` (`CustomerID`, `Name`, `Email`, `CountryCode`, `Budget`, `Used`) VALUES
('C001', 'Win Weerachai', '[email protected]', 'TH', '1000000.00', '600000.00'),
('C002', 'John Smith', '[email protected]', 'UK', '2000000.00', '800000.00'),
('C003', 'Jame Born', '[email protected]', 'US', '3000000.00', '600000.00'),
('C004', 'Chalee Angel', '[email protected]', 'US', '4000000.00', '100000.00');
นำคำสั่ง SQL นี้ไปสร้าง Database ในฝั่งของ Web Service Server
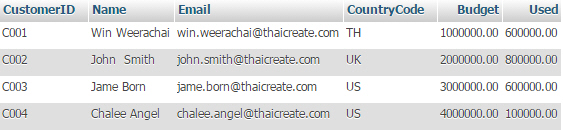
เมื่อสร้างเสร็จจะได้โครงสร้างและข้อมูลดังรูป
Example 1 การสร้าง JSON ในรูปแบบของข้อมูลหลาย Rows หรือ Array หลายมิติ
<?php
ini_set('display_errors', 1);
error_reporting(~0);
$serverName = "localhost";
$userName = "root";
$userPassword = "root";
$dbName = "mydatabase";
$conn = mysqli_connect($serverName,$userName,$userPassword,$dbName);
$sql = "SELECT * FROM customer";
$query = mysqli_query($conn,$sql);
if (!$query) {
printf("Error: %s\n", $conn->error);
exit();
}
$resultArray = array();
while($result = mysqli_fetch_array($query,MYSQLI_ASSOC))
{
array_push($resultArray,$result);
}
mysqli_close($conn);
echo json_encode($resultArray);
?>
Result
[
{"CustomerID":"C001","Name":"Win Weerachai","Email":"[email protected]","CountryCode":"TH","Budget":"1000000.00","Used":"600000.00"}
,{"CustomerID":"C002","Name":"John Smith","Email":"[email protected]","CountryCode":"UK","Budget":"2000000.00","Used":"800000.00"}
,{"CustomerID":"C003","Name":"Jame Born","Email":"[email protected]","CountryCode":"US","Budget":"3000000.00","Used":"600000.00"}
,{"CustomerID":"C004","Name":"Chalee Angel","Email":"[email protected]","CountryCode":"US","Budget":"4000000.00","Used":"100000.00"}
]
จาก Result ดังกล่าวในกรณีที่ฝั่งที่เรียกใช้สามารถใช้ json_decode() ได้ดังนี้
<?php
ini_set('display_errors', 1);
error_reporting(~0);
function createJSON()
{
$serverName = "localhost";
$userName = "root";
$userPassword = "root";
$dbName = "mydatabase";
$conn = mysqli_connect($serverName,$userName,$userPassword,$dbName);
$sql = "SELECT * FROM customer";
$query = mysqli_query($conn,$sql);
if (!$query) {
printf("Error: %s\n", $conn->error);
exit();
}
$resultArray = array();
while($result = mysqli_fetch_array($query,MYSQLI_ASSOC))
{
array_push($resultArray,$result);
}
mysqli_close($conn);
return json_encode($resultArray);
}
// Read JSON Decode
$jsonCode = createJSON();
$jsonDecode = json_decode($jsonCode, true);
foreach ($jsonDecode as $result)
{
echo $result['CustomerID']." ";
echo $result['Name']." ";
echo $result['Email']." ";
echo $result['CountryCode']." ";
echo $result['Budget']." ";
echo $result['Used']." ";
echo "<br>";
}
?>
Result
Example 2 การสร้าง JSON ในรูปแบบของข้อมูล Rows เดียวหรือชุดเดียว
<?php
ini_set('display_errors', 1);
error_reporting(~0);
$serverName = "localhost";
$userName = "root";
$userPassword = "root";
$dbName = "mydatabase";
$strCustomerID = "C001";
$conn = mysqli_connect($serverName,$userName,$userPassword,$dbName);
$sql = "SELECT * FROM customer WHERE CustomerID = '$strCustomerID' ";
$query = mysqli_query($conn,$sql);
if (!$query) {
printf("Error: %s\n", $conn->error);
exit();
}
$result = mysqli_fetch_array($query,MYSQLI_ASSOC);
mysqli_close($conn);
echo json_encode($result);
?>
Result
{"CustomerID":"C001","Name":"Win Weerachai","Email":"[email protected]","CountryCode":"TH","Budget":"1000000.00","Used":"600000.00"}
จาก Result จะเห็นว่าจะไม่มีเครื่องหมาย [ ] ครอบชุดของ JSON และฝั่งที่เรียกใช้สามารถใช้ json_decode() ได้ดังนี้
<?php
ini_set('display_errors', 1);
error_reporting(~0);
function createJSON()
{
$serverName = "localhost";
$userName = "root";
$userPassword = "root";
$dbName = "mydatabase";
$strCustomerID = "C001";
$conn = mysqli_connect($serverName,$userName,$userPassword,$dbName);
$sql = "SELECT * FROM customer WHERE CustomerID = '$strCustomerID' ";
$query = mysqli_query($conn,$sql);
if (!$query) {
printf("Error: %s\n", $conn->error);
exit();
}
$result = mysqli_fetch_array($query,MYSQLI_ASSOC);
mysqli_close($conn);
return json_encode($result);
}
// Read JSON Decode
$jsonCode = createJSON();
$jsonDecode = json_decode($jsonCode, true);
echo $jsonDecode['CustomerID']."<br/>";
echo $jsonDecode['Name']."<br/>";
echo $jsonDecode['Email']."<br/>";
echo $jsonDecode['CountryCode']."<br/>";
echo $jsonDecode['Budget']."<br/>";
echo $jsonDecode['Used']."<br/>";
?>
Result
สรุป
ในตัวอย่างนี้จะเป็นเพียงการสร้าง JSON ด้วย PHP และเรียกใช้งานในหน้าเว็บเดียวกัน แต่ในกรณีที่ต้องการเรียกหรือรับ-ส่งค่าผ่าน API อื่น ๆ เช่น Ajax , Web Services หรือพัฒนาร่วมกับ Application ประเภท Smartphone (Android, iOS, Windows Phone) ก็จะสามารถนำ JSON นี้ไปใช้ได้เช่นเดียวกัน ส่วนวิธีการ Decode หรือ Parser นั้นก็ขึ้นอยู่กับ Application ในฝั่งที่รับข้อความ JSON แล้วเอาไปใช้งาน
บทความอื่น ๆ ที่เกี่ยวข้อง
Go to : jQuery Ajax กับ JSON ทำความเข้าใจ การรับส่งข้อมูล JSON ผ่าน jQuery กับ Ajax
Go to : วิธีการสร้าง PHP กับ Web Service และ Return Array ไปยัง Client ด้วย NuSoap
Go to : PHP - Web Service กับ MySQL Database รับส่งค่า Result ผ่านเว็บเซอร์วิส (NuSoap)
Go to : การทำ Login Form และ Update MySQL Data ผ่าน Web Service ด้วย PHP (NuSoap)
Go to : PHP Create - Call Web Service สร้างและเรียกเว็บเซอร์วิส ด้วย PHP (NuSoap and Soap)
Go to : PHP สร้าง Web Service และใช้ .NET เรียก Web Service ของ PHP (ASP.NET and Windows Form)
Go to : php กับ web service ขอคำปรึกษาเรื่องการ return array ใน webservice ครับ
Go to : สอบถาม jquery ในส่วนของการนำ json data ที่เป็นภาษาไทย ในการนำไปใช้งานครับ
|
|
|
|
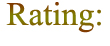 |
|
|
|
By : |
TC Admin
|
|
Article : |
บทความเป็นการเขียนโดยสมาชิก หากมีปัญหาเรื่องลิขสิทธิ์ กรุณาแจ้งให้ทาง webmaster ทราบด้วยครับ |
|
Score Rating : |
  |
|
Create Date : |
2015-11-19 |
|
Download : |
No files |
|
|