Android and Picasso (Image Loader LIB:Library) |
Android and Picasso (Image Loader LIB:Library) สำหรับ Image Loader Library ตัวหนึ่งที่น่าสนใจคือ Picasso เป็น Library ที่เข้ามาช่วยเกี่ยกับการจัดการการแสดงผลรูปภาพแก้ไขปัยหา OutofMemory โดยความสามารถของ Picasso สามารถอ้างถึงแหล่งของ Image Resource จากแหล่งต่าง ๆ ได้เช่น Drawable, Asset, Storage (SD card) , Internet URL สามารถแปลงเป็น Bitmap แล้วค่อยนำไปใช้งานได้ มีรูปแบบการใช้งานง่ายมาก โดยความสามารถเด่น ๆ ของมันที่เกี่ยวข้องคือ การทำแคชรูปภาพทั้งบน Disk Storage และ Memory , การ Resize ลดขนาดของภาพ ลด memory ที่ใช้ การทำงานได้อย่างรวดเร็ว และความสามารถที่สามารถใช้งานบนพวก Adapter เมื่อใช้งานร่วมกับ ListView , GridView ได้อย่างมีประสิทธิภาพ เพราะมีการ Re-use ภาพที่ได้โหลดมาแล้วไม่ให้โหลดภาพเดิมซ้ำ ๆ
Android and Picasso
ในการใช้งาน Picasso Library เราอาจจะได้รู้จักกับ Glide Library ซึ่งทั้ง 2 ตัวนี้มีรูปแบบการใช้งานคล้ายคลึงกันมากกว่า 90% ส่วนข้อแตกต่างระหว่าง Glide กับ Picasso คือ Glide รองรับการทำงานทั้ง Context, Activity, Fragment และ FragmentActivity และสามารถโหลดรุปภาพได้เร็วมากกว่า Picasso แต่ระดับควาคมชัดตัว Picasso ยังสามารถทำได้ดีกว่า และสิ่งที่ Glide เหนือกว่า Picasso อีกข้อหนึ่งคือรองรับไฟล์ GIF ในรูปแบบของไฟล์เคลื่อนไหวได้
สำหรับตัวอย่างและ Code นี้รองรับการเขียนทั้งบนโปรแกรม Eclipse และ Android Studio
Download Picasso
Feature
- Handling ImageView recycling and download cancelation in an adapter.
- Complex image transformations with minimal memory use.
- Automatic memory and disk caching.
รุปแบบการเข้าถึง Resource จากแหล่งต่าง ๆ
Picasso.with(context).load(ImageURI).into(imageView);
ImageURI คือ Image Resource เช่น Drawable, Asset, Storage (SD card) , Internet URL
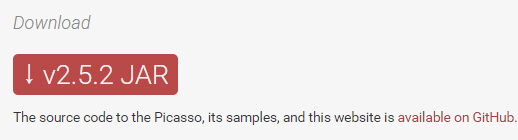
การติดตั้งสามารถดาวน์โหลดไฟล์ .JAR แล้วนำไป Import ลงใน Eclipse หรือ Android Studio
กรณีใช้บน Android Studio เพิ่ม build.gradle
compile 'com.squareup.picasso:picasso:2.5.2' อาจจะต้องตรวจสอบเวอร์ชั่นจากเว็บไซต์
ใน AndroidManifest.xml เพิ่ม Permission ดังนี้
<uses-permission android:name="android.permission.INTERNET" />
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
ขั้นตอนการเรียกใช้
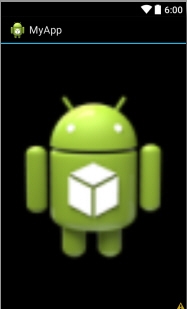
สร้าง Layout และ ImageView แบบง่าย ๆ
activity_main.xml
<TableLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent" >
<ImageView
android:id="@+id/imageView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="0.39"
android:src="@drawable/ic_launcher" />
</TableLayout>
การเรียกรูปภาพจาก Resource
//*** Display Images
Picasso.with(this).load(R.drawable.picture).into(imageView); // URIs from drawable
Code เต็ม ๆ
package com.myapp;
import android.os.Bundle;
import android.os.StrictMode;
import com.squareup.picasso.Picasso;
import android.app.Activity;
import android.view.Menu;
import android.widget.ImageView;
public class MainActivity extends Activity {
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Permission StrictMode
if (android.os.Build.VERSION.SDK_INT > 9) {
StrictMode.ThreadPolicy policy = new StrictMode.ThreadPolicy.Builder().permitAll().build();
StrictMode.setThreadPolicy(policy);
}
// *** ImageView
ImageView imageView = (ImageView) findViewById(R.id.imageView);
//*** Display Images
Picasso.with(this).load(R.drawable.picture).into(imageView); // URIs from drawable
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.main, menu);
return true;
}
}
Example 1 : การเรียก Image Resource จาก drawable
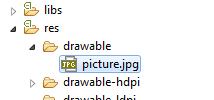
// *** ImageView
ImageView imageView = (ImageView) findViewById(R.id.imageView);
//*** Display Images
Picasso.with(this).load(R.drawable.picture).into(imageView); // URIs from drawable
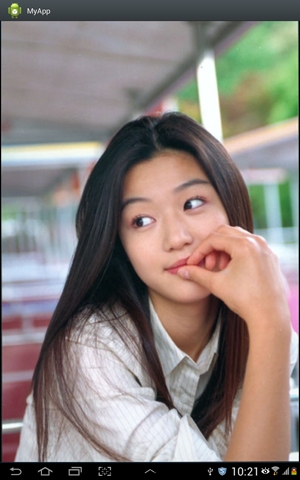
Example 2 : การเรียก Image Resource จาก Asset
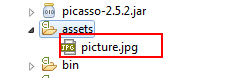
// *** ImageView
ImageView imageView = (ImageView) findViewById(R.id.imageView);
//*** Display Images
Picasso.with(this).load("file:///android_asset/picture.jpg").into(imageView); // URIs from asset
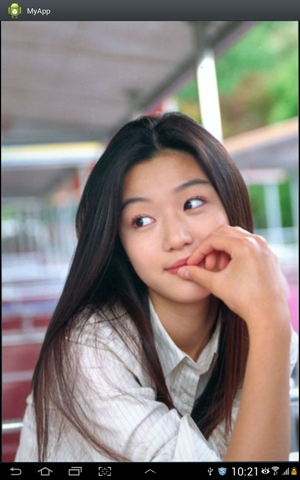
Example 3 : การเรียก Image Resource จาก SD Card
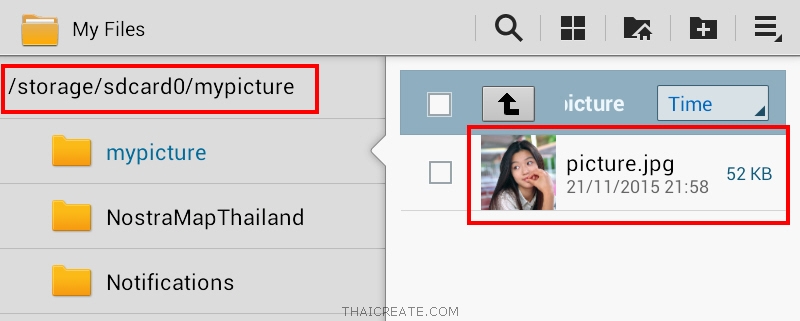
// *** ImageView
ImageView imageView = (ImageView) findViewById(R.id.imageView);
//*** Display Images
String SDPath = "/storage/sdcard0/mypicture/picture.jpg"; // URIs from SD card
Picasso.with(this).load(new File(SDPath)).into(imageView);
หรือ
// *** ImageView
ImageView imageView = (ImageView) findViewById(R.id.imageView);
//*** Display Images
String SDPath = Environment.getExternalStorageDirectory() + "/mypicture/picture.jpg"; // URIs from SD card
Picasso.with(this).load(new File(SDPath)).into(imageView);
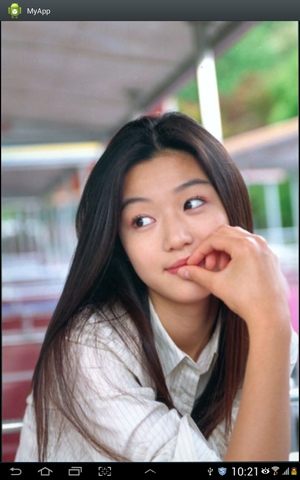
Example 4 : การเรียก Image Resource จาก HTTP (Internet)
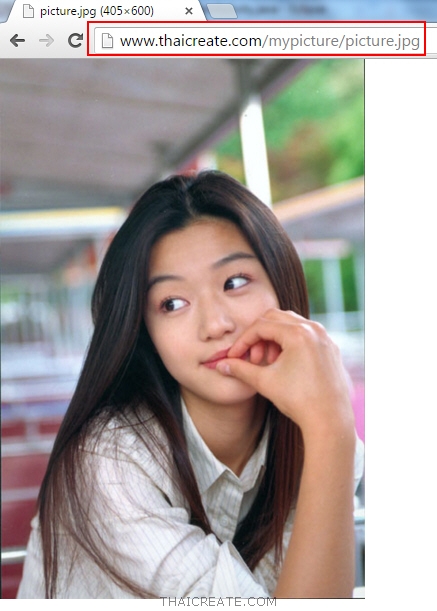
// *** ImageView
ImageView imageView = (ImageView) findViewById(R.id.imageView);
//*** Display Images
Picasso.with(this).load("https://www.thaicreate.com/mypicture/picture.jpg").into(imageView); // URIs from URL
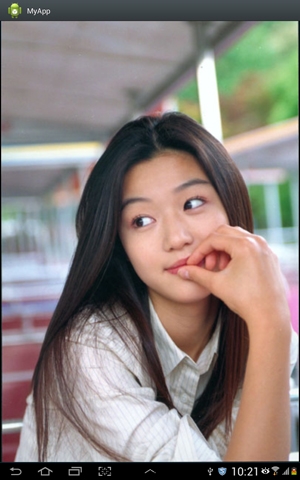
Example 5 : การ Resize ลดขนาดของภาพให้มีขนาดตามต้องการ
// *** ImageView
ImageView imageView = (ImageView) findViewById(R.id.imageView);
//*** Display Images
Picasso.with(this)
.load("https://www.thaicreate.com/mypicture/picture.jpg")
.resize(50, 50)
.centerCrop()
.into(imageView);
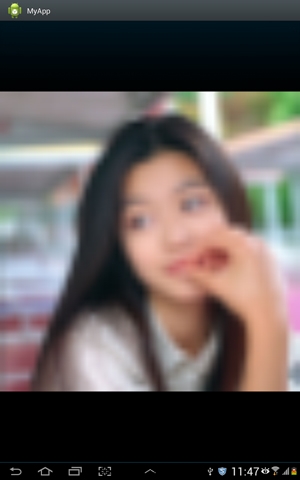
Example 6 : การกำหนด placeholder และ error ในช่วงระหว่างรอโหลดและกรณีที่เกิดข้อผิดพลาด
// *** ImageView
ImageView imageView = (ImageView) findViewById(R.id.imageView);
//*** Display Images
Picasso.with(this)
.load("https://www.thaicreate.com/mypicture/picture.jpg")
.placeholder(R.drawable.user_placeholder)
.error(R.drawable.user_placeholder_error)
.into(imageView);
Example 7 : การใช่ร่วมกับ Adapter ในส่วนของ getView เช่น ListView , GridView
// Adapter re-use is automatically detected and the previous download canceled.
@Override public void getView(int position, View convertView, ViewGroup parent) {
SquaredImageView view = (SquaredImageView) convertView;
if (view == null) {
view = new SquaredImageView(context);
}
String url = getItem(position);
Picasso.with(context).load(url).into(view);
}
จากตัวอย่างจะเห็นว่าการใช้งาน Picasso ช่วยให้การจัดการกับ Image นั้นง่ายและสะดวกมาก อีกทั้งยังได้มาพร้อมกับประสิทธิภาพของภาพทั้งความคมชัด ประหยัด memory ได้ความรวดเร็วกในการโหลดข้อมูล
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
   |
|
|
Create/Update Date : |
2015-11-22 12:58:45 /
2017-03-26 21:27:24 |
|
Download : |
No files |
|
Sponsored Links / Related |
|
|
|
|
|
|