iOS/iPhone Search Bar and Scope Bar (Objective-C , iPhone) |
iOS/iPhone Search Bar and Scope Bar (Objective-C , iPhone)ใน Search Bar สามารถใส่ Button ต่าง ๆ เช่น Scope Search Bar ฟีเจอร์นี้เราจะเห็นได้จากโปรแกรม Mail ของ iPhone หรือ iPad โดยจะใช้เมื่อเราต้องการ Scope ข้อมูลที่ต้องการค้นหา เช่น ค้นหาจาก From , To , Subject หรือ All ทั้งหมด แต่เราสามารถ Apply ความสามารถของ Scope Bar ให้ใช้ได้กับ App ของเราได้หลากหลาย และในตัวอย่างนี้เราจะมาสร้าง Scope Bar บน Search Bar แบบง่าย ๆ เพื่อความเข้าใจและการทำไป Apply ใช้งานอย่างอื่น
iOS/iPhone Search Bar and Scope Bar
เพื่อความเข้าใจควบอ่านบทความที่เกี่ยวข้องก่อนหน้านี้ เช่น Search Bar (UISearchBar) และ Search and Bar Display Controller (UISearchDisplayController) ซึ่งทั้ง 2 บทความนี้จะเป็นพื้นฐานการใช้งาน Search Bar กับ Table View
iOS/iPhone Search Bar (UISearchBar) and Table View (UITableView)
iOS/iPhone Search and Bar Display Controller (UISearchDisplayController)
Example การสร้าง Search Bar และ Scope Bar แบบง่าย ๆ
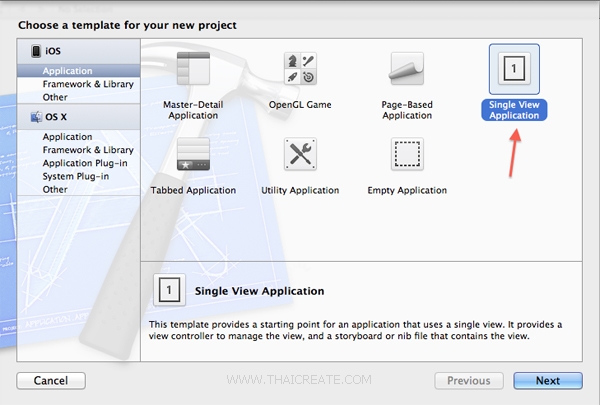
เริ่มต้นด้วยการสร้าง Project บน Xcode แบบง่าย ๆ ด้วย Single View Application
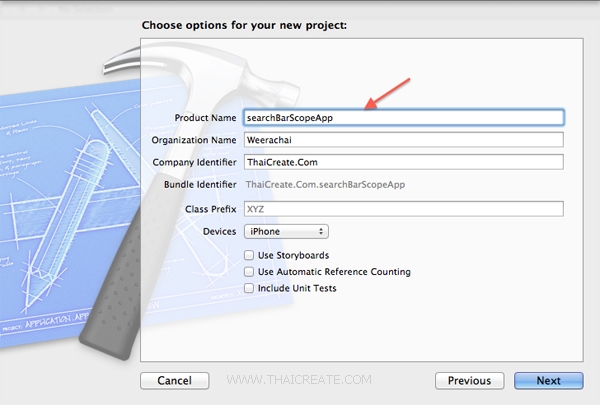
เลือกและไม่เลือกรายการดังรูป จากนั้นเลือกที่ Create
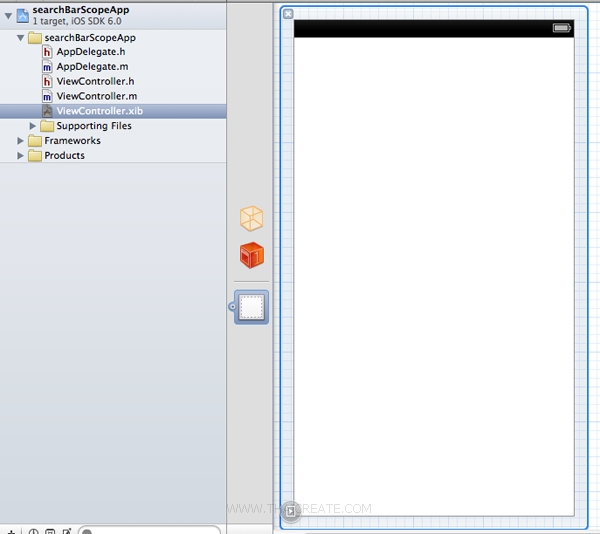
ได้โปรเจคบน Xcode ตอนนี้หน้าจอ View ของเรายังว่าง ๆ
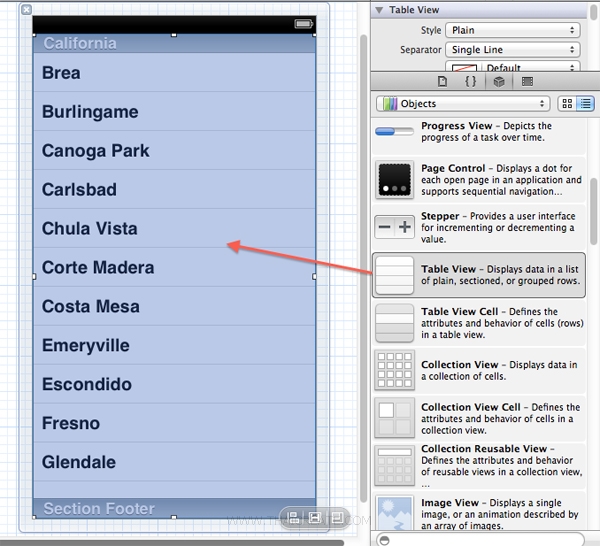
ลาก Object ของ Table View มาวางไว้บนหน้าจอ Design ของ View
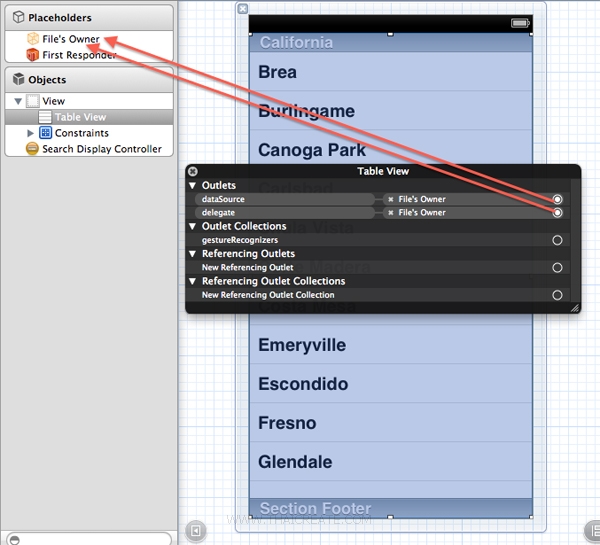
คลิกควาที่ Table View ทำการเชื่อม delegate และ dataSource กับ File's Owner

ใน Class ของ .h ประกาศค่าต่าง ๆ ดังรูป
@interface ViewController : UIViewController <UITableViewDataSource, UISearchBarDelegate,UISearchDisplayDelegate>
@property (nonatomic, retain) UISearchDisplayController *searchDisplayController;
@end
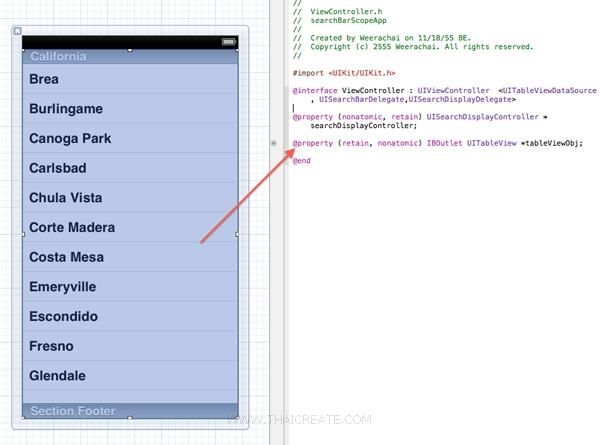
ให้เชื่อม TableView กับ IBOutlet จากนั้นจะเป็นส่วนของ Code ดังนี้
Code ทั้งหมดของ .h และ .m ในภาษา Objective-C
ViewController.h
//
// ViewController.h
// searchBarScopeApp
//
// Created by Weerachai on 11/18/55 BE.
// Copyright (c) 2555 Weerachai. All rights reserved.
//
#import <UIKit/UIKit.h>
@interface ViewController : UIViewController <UITableViewDataSource, UISearchBarDelegate,UISearchDisplayDelegate>
@property (nonatomic, retain) UISearchDisplayController *searchDisplayController;
@property (retain, nonatomic) IBOutlet UITableView *tableViewObj;
@end
ViewController.m
//
// ViewController.m
// searchBarScopeApp
//
// Created by Weerachai on 11/18/55 BE.
// Copyright (c) 2555 Weerachai. All rights reserved.
//
#import "ViewController.h"
@interface ViewController ()
{
NSMutableArray *allObject;
NSMutableArray *displayObject;
int Scope;
}
@end
@implementation ViewController
@synthesize searchDisplayController;
- (void)viewDidLoad
{
[super viewDidLoad];
// Do any additional setup after loading the view, typically from a nib.
allObject = [[NSMutableArray alloc] init];
[allObject addObject:@"1. One"];
[allObject addObject:@"2. Two"];
[allObject addObject:@"3. Three"];
[allObject addObject:@"4. Four"];
[allObject addObject:@"5. Five"];
[allObject addObject:@"6. Six"];
[allObject addObject:@"7. Seven"];
[allObject addObject:@"8. Eight"];
[allObject addObject:@"9. Nine"];
[allObject addObject:@"10. Ten"];
displayObject =[[NSMutableArray alloc] initWithArray:allObject];
/*
set up the searchDisplayController programically
*/
UISearchBar *mySearchBar = [[UISearchBar alloc] init];
[mySearchBar setScopeButtonTitles:[NSArray arrayWithObjects:@"All",@"1 To 5",@"6 To 10",nil]];
mySearchBar.delegate = self;
[mySearchBar setAutocapitalizationType:UITextAutocapitalizationTypeNone];
[mySearchBar sizeToFit];
self.tableViewObj.tableHeaderView = mySearchBar;
searchDisplayController = [[UISearchDisplayController alloc] initWithSearchBar:mySearchBar contentsController:self];
[self setSearchDisplayController:searchDisplayController];
[searchDisplayController setDelegate:self];
[searchDisplayController setSearchResultsDataSource:self];
[mySearchBar release];
}
- (NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section
{
return displayObject.count;
}
- (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath
{
static NSString *CellIdentifier = @"Cell";
UITableViewCell *cell = [tableView dequeueReusableCellWithIdentifier:CellIdentifier];
if (cell == nil) {
// Use the default cell style.
cell = [[[UITableViewCell alloc] initWithStyle : UITableViewCellStyleSubtitle
reuseIdentifier : CellIdentifier] autorelease];
}
NSString *cellValue = [displayObject objectAtIndex:indexPath.row];
cell.textLabel.text = cellValue;
return cell;
}
- (BOOL)searchDisplayController:(UISearchDisplayController *)controller shouldReloadTableForSearchString:(NSString *)searchString
{
if([searchString length] == 0)
{
[displayObject removeAllObjects];
[displayObject addObjectsFromArray:allObject];
}
else
{
int i;
i = 0;
[displayObject removeAllObjects];
switch (Scope)
{
case 0 : // All
for(NSString * string in allObject)
{
NSRange r = [string rangeOfString:searchString options:NSCaseInsensitiveSearch];
if(r.location != NSNotFound)
{
[displayObject addObject:string];
}
}
break;
case 1 : // 1 To 5
for(NSString * string in allObject)
{
i = i + 1;
if(i>=1 && i<=6)
{
NSRange r = [string rangeOfString:searchString options:NSCaseInsensitiveSearch];
if(r.location != NSNotFound)
{
[displayObject addObject:string];
}
}
}
break;
case 2 : // 6 To 10
for(NSString * string in allObject)
{
i = i + 1;
if(i>=6 && i<=10)
{
NSRange r = [string rangeOfString:searchString options:NSCaseInsensitiveSearch];
if(r.location != NSNotFound)
{
[displayObject addObject:string];
}
}
}
break;
}
}
return YES;
}
- (BOOL)searchDisplayController:(UISearchDisplayController *)controller shouldReloadTableForSearchScope:(NSInteger)searchOption{
Scope = searchOption;
[displayObject removeAllObjects];
return YES;
}
- (void)didReceiveMemoryWarning
{
[super didReceiveMemoryWarning];
// Dispose of any resources that can be recreated.
}
- (void)dealloc {
[_tableViewObj release];
[super dealloc];
}
@end
คำอธิบาย จาก Code เราจะเห็น method ที่จะต้องดูอยู่ 2 ส่วนคือ
- (BOOL)searchDisplayController:(UISearchDisplayController *)controller shouldReloadTableForSearchScope:(NSInteger)searchOption{
Scope = searchOption;
[displayObject removeAllObjects];
return YES;
}
เป็น method เมื่อมีการคลิกที่ Scope โดนเราจะอ่านได้จาตำแหน่งของ index ของ Scope Bar เริ่มจาก 0...1...2 และเก็บไว้ในตัวแปร Scope
- (BOOL)searchDisplayController:(UISearchDisplayController *)controller shouldReloadTableForSearchString:(NSString *)searchString
{
case 1 : // 1 To 5
for(NSString * string in allObject)
{
i = i + 1;
if(i>=1 && i<=6)
{
NSRange r = [string rangeOfString:searchString options:NSCaseInsensitiveSearch];
if(r.location != NSNotFound)
{
[displayObject addObject:string];
}
}
}
และเมื่อได้ตัวแปร Scope แล้ว เราสามารถนำมาเป็นเงื่อนไขสำหรับการ Filter ข้อมูลต่าง ๆ ได้
Screenshot
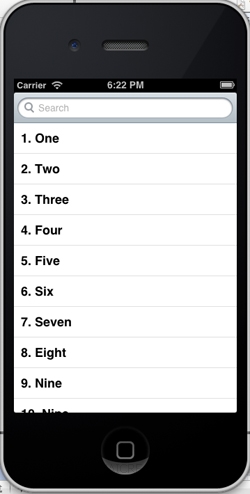
แสดงหน้าจอของ App
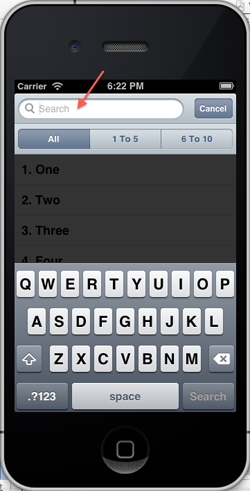
คลิกเพื่อค้นหา จะมี Scope Bar ขึ้นดังรูป โดยในตัวอย่างนี้จะแข่ง 1 To 5 และ 6 To 10 เพื่อ Scope ข้อมูลใน Array
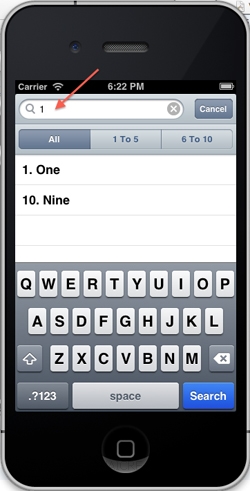
ค้นหา 1 จาก All ซึ่งจะแสดง 1 และ 10
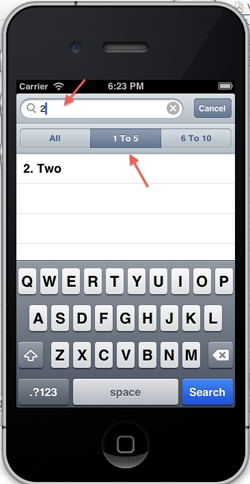
คลิกที่ 1 to 5 และค้นหา 2 จะยังมี 2. Two
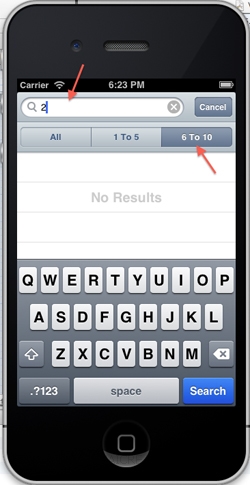
แต่เมื่อคลิกที่ 6 To 10 และค้นหา 2 จะไม่มี 2. Two เพราะใน Code เรากำหนดให้ Filter ข้อมูลใน 6 To 10 เท่านั้น
นอกจากนี้[b] Scope Bar ยังสามารถ Apply กับหลาย ๆ ประเภทเช่น[/b]
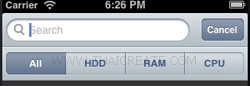
ค้นหาแยกตามประเภท
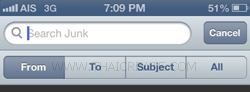
อันนี้เป็นตัวอย่างบน App ของ Mail
.
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
  |
|
|
Create/Update Date : |
2012-11-20 10:03:57 /
2017-03-26 09:10:50 |
|
Download : |
|
|
Sponsored Links / Related |
|
|
|
|
|
|