Windows Store App and XML Parser (C#) |
Windows Store App and XML Parser (C#) สำหรับ XML เป็นรูปแบบ Format ไฟล์หนึ่งที่ยังได้รับความนิยม และได้ใช้มานานนับ สิบปี และปัจุบันหลาย ๆ Interface ก็ยังมีการใช้งานอยู่จนถึงปัจจุบัน เหตุผลหลัก็คือ XML เป็นรูปแบบการจัดเก็บข้อมูลที่ง่าย เป็นมาตรฐานสากล รองรับการทำงานหลาย ๆ Platform และหลาย ๆ Application ก็มี Class Library ที่จะจัดการกับไฟล์ของ XML อาทิ การสร้างไฟล์ XML หรือ การอ่าน XML ไฟล์ เพื่อที่จะนำข้อมูลที่ได้ไปใช้กับโปรแกรมในอ่านต่าง ๆ
และบน Windows Store Apps การอ่านเขียน XML ไฟล์ก็มีรูปแบบการใช้งานที่เหมือนกับการเขียนบน .NET Application ในรูปแบบอื่น ๆ คือสามารถใช้ NameSpace ในชุด Library ของ System.Xml เข้ามาจัดการได้ในทันที
myXML.xml ตัวอย่างไฟล์ XML
<?xml version="1.0" encoding="utf-8"?>
<customers>
<customer>
<CustomerID>C001</CustomerID>
<Name>Win Weerachai</Name>
<Email>[email protected]</Email>
<CountryCode>TH</CountryCode>
<Budget>1000000</Budget>
<Used>600000</Used>
</customer>
<customer>
<CustomerID>C002</CustomerID>
<Name>John Smith</Name>
<Email>[email protected]</Email>
<CountryCode>UK</CountryCode>
<Budget>2000000</Budget>
<Used>800000</Used>
</customer>
<customer>
<CustomerID>C003</CustomerID>
<Name>Jame Born</Name>
<Email>[email protected]</Email>
<CountryCode>US</CountryCode>
<Budget>3000000</Budget>
<Used>600000</Used>
</customer>
<customer>
<CustomerID>C004</CustomerID>
<Name>Chalee Angel</Name>
<Email>[email protected]</Email>
<CountryCode>US</CountryCode>
<Budget>4000000</Budget>
<Used>100000</Used>
</customer>
</customers>
การอ่าน XML บน Windows Store Apps (C#)
สร้าง Class สำหรับ Mapping Column
public class myCustomer
{
public string CustomerID { get; set; }
public string Name { get; set; }
public string Email { get; set; }
public string CountryCode { get; set; }
public string Budget { get; set; }
public string Used { get; set; }
}
สร้างชุดคำสั่งสำหรับการอ่าน XML และ Mapping กับ Column ในรูปแบบของ List Object
List<myCustomer> myCustomer = new List<myCustomer>();
XDocument loadData = XDocument.Load("XML/myXML.xml");
var data = from query in loadData.Descendants("customer")
select new myCustomer()
{
CustomerID = (string)query.Element("CustomerID"),
Name = (string)query.Element("Name"),
Email = (string)query.Element("Email"),
CountryCode = (string)query.Element("CountryCode"),
Budget = (string)query.Element("Budget"),
Used = (string)query.Element("Used")
};
ลองมาดูตัวอย่างแบบเต็ม ๆ
Example การเขียน Windows Store Apps อ่านข้อมูลที่อยู่ในรูปแบบของ XML ไฟล์
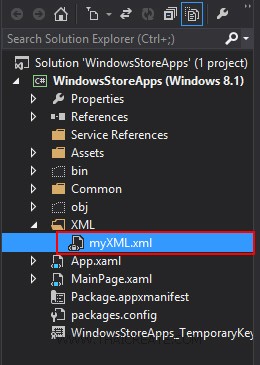
ไฟล์ XML ที่อยู่บน Project
MainPage.xaml
<Page
x:Class="WindowsStoreApps.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:WindowsStoreApps"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d">
<Grid Background="{ThemeResource ApplicationPageBackgroundThemeBrush}">
<TextBlock x:Name="lblResult" HorizontalAlignment="Left" Margin="66,83,0,0" TextWrapping="Wrap" VerticalAlignment="Top" FontSize="20" Text="lblResult"/>
</Grid>
</Page>
MainPage.xaml.cs
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using Windows.Devices.Geolocation;
using Windows.Foundation;
using Windows.Foundation.Collections;
using Windows.UI.Core;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml.Controls.Primitives;
using Windows.UI.Xaml.Data;
using Windows.UI.Xaml.Input;
using Windows.UI.Xaml.Media;
using Windows.UI.Xaml.Navigation;
using System.Xml.Linq;
// The Blank Page item template is documented at http://go.microsoft.com/fwlink/?LinkId=234238
namespace WindowsStoreApps
{
/// <summary>
/// An empty page that can be used on its own or navigated to within a Frame.
/// </summary>
///
public sealed partial class MainPage : Page
{
public MainPage()
{
this.InitializeComponent();
}
public class myCustomer
{
public string CustomerID { get; set; }
public string Name { get; set; }
public string Email { get; set; }
public string CountryCode { get; set; }
public string Budget { get; set; }
public string Used { get; set; }
}
protected override void OnNavigatedTo(NavigationEventArgs e)
{
List<myCustomer> myCustomer = new List<myCustomer>();
XDocument loadData = XDocument.Load("XML/myXML.xml");
var data = from query in loadData.Descendants("customer")
select new myCustomer()
{
CustomerID = (string)query.Element("CustomerID"),
Name = (string)query.Element("Name"),
Email = (string)query.Element("Email"),
CountryCode = (string)query.Element("CountryCode"),
Budget = (string)query.Element("Budget"),
Used = (string)query.Element("Used")
};
this.lblResult.Text = string.Empty;
foreach (myCustomer customer in data)
{
//customer.CustomerID.ToString();
//customer.Name.ToString();
//customer.Email.ToString();
//customer.CountryCode.ToString();
//customer.Budget.ToString();
//customer.Used.ToString();
this.lblResult.Text = this.lblResult.Text + "CustomerID : " + customer.CustomerID.ToString();
this.lblResult.Text = this.lblResult.Text + Environment.NewLine;
this.lblResult.Text = this.lblResult.Text + "Name : " + customer.Name.ToString();
this.lblResult.Text = this.lblResult.Text + Environment.NewLine;
this.lblResult.Text = this.lblResult.Text + "Email : " + customer.Email.ToString();
this.lblResult.Text = this.lblResult.Text + Environment.NewLine;
this.lblResult.Text = this.lblResult.Text + "CountryCode : " + customer.CountryCode.ToString();
this.lblResult.Text = this.lblResult.Text + Environment.NewLine;
this.lblResult.Text = this.lblResult.Text + "Budget : " + customer.Budget.ToString();
this.lblResult.Text = this.lblResult.Text + Environment.NewLine;
this.lblResult.Text = this.lblResult.Text + "Used : " + customer.Used.ToString();
this.lblResult.Text = this.lblResult.Text + Environment.NewLine;
this.lblResult.Text = this.lblResult.Text + "======================";
this.lblResult.Text = this.lblResult.Text + Environment.NewLine;
}
}
}
}
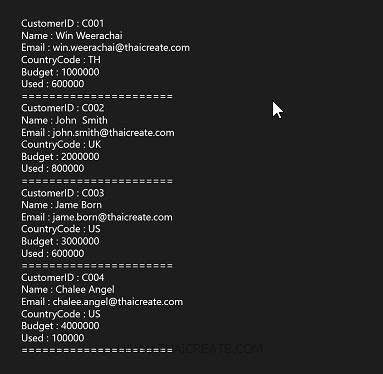
แสดงข้อมูลจาก XML บน Windows Store Apps ซึ่ง Loop แสดงผลบน TextBlock แบบง่าย ๆ
.
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
 |
|
|
Create/Update Date : |
2014-05-27 11:55:19 /
2017-03-19 15:13:33 |
|
Download : |
No files |
|
Sponsored Links / Related |
|
|
|
|
|
|