ASP.NET DataGrid Control - VS 2005,2008,2010 (FX 2.0,3.5,4.0) |
ASP.NET DataGrid Control - Visual Studio 2005,2008,2010 (Framework 2.0,3.5,4.0) แม้ว่าใน Framework 2.0,3.5,4.0 จะเปลี่ยนชื่อ DataGrid เป็น GridView แต่ก็ยังสามารถใช้งาน DataGrid ได้เช่นเดิมทุกประการ ไม่ว่าจะเป็น Event Handles ของ DataGrid จะยังคงไว้และสามารถเรียกใช้งานได้เช่นเดิมครับ
Code นี้จะเป็นการ Add,Edit,Update และ Delete ข้อมูลในฐานข้อมูล
Language Code : VB.NET || C#
Framework : 2,3,4
DataGrid1.aspx
<%@ Page Language="VB" AutoEventWireup="false" CodeFile="DataGrid1.aspx.vb" Inherits="DataGrid1" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml" >
<head runat="server">
<title>ThaiCreate.Com ASP.NET - DataGrid</title>
</head>
<body>
<form id="form1" runat="server">
<asp:DataGrid id="myDataGrid" runat="server" AutoGenerateColumns="False"
ShowFooter="True"
DataKeyField="CustomerID">
<Columns>
<asp:TemplateColumn HeaderText="CustomerID">
<ItemTemplate>
<asp:Label id="lblCustomerID" runat="server" Text='<%# DataBinder.Eval(Container, "DataItem.CustomerID") %>'></asp:Label>
</ItemTemplate>
<EditItemTemplate>
<asp:TextBox id="txtEditCustomerID" size="5" runat="server" Text='<%# DataBinder.Eval(Container, "DataItem.CustomerID") %>'></asp:TextBox>
</EditItemTemplate>
<FooterTemplate>
<asp:TextBox id="txtAddCustomerID" size="5" runat="server"></asp:TextBox>
</FooterTemplate>
</asp:TemplateColumn>
<asp:TemplateColumn HeaderText="Name">
<ItemTemplate>
<asp:Label id="lblName" runat="server" Text='<%# DataBinder.Eval(Container, "DataItem.Name") %>'></asp:Label>
</ItemTemplate>
<EditItemTemplate>
<asp:TextBox id="txtEditName" size="10" runat="server" Text='<%# DataBinder.Eval(Container, "DataItem.Name") %>'></asp:TextBox>
</EditItemTemplate>
<FooterTemplate>
<asp:TextBox id="txtAddName" size="10" runat="server"></asp:TextBox>
</FooterTemplate>
</asp:TemplateColumn>
<asp:TemplateColumn HeaderText="Email">
<ItemTemplate>
<asp:Label id="lblEmail" runat="server" Text='<%# DataBinder.Eval(Container, "DataItem.Email") %>'></asp:Label>
</ItemTemplate>
<EditItemTemplate>
<asp:TextBox id="txtEditEmail" size="20" runat="server" Text='<%# DataBinder.Eval(Container, "DataItem.Email") %>'></asp:TextBox>
</EditItemTemplate>
<FooterTemplate>
<asp:TextBox id="txtAddEmail" size="20" runat="server"></asp:TextBox>
</FooterTemplate>
</asp:TemplateColumn>
<asp:TemplateColumn HeaderText="CountryCode">
<ItemTemplate>
<asp:Label id="lblCountryCode" runat="server" Text='<%# DataBinder.Eval(Container, "DataItem.CountryCode") %>'></asp:Label>
</ItemTemplate>
<EditItemTemplate>
<asp:TextBox id="txtEditCountryCode" size="2" runat="server" Text='<%# DataBinder.Eval(Container, "DataItem.CountryCode") %>'></asp:TextBox>
</EditItemTemplate>
<FooterTemplate>
<asp:TextBox id="txtAddCountryCode" size="2" runat="server"></asp:TextBox>
</FooterTemplate>
</asp:TemplateColumn>
<asp:TemplateColumn HeaderText="Budget">
<ItemTemplate>
<asp:Label id="lblBudget" runat="server" Text='<%# DataBinder.Eval(Container, "DataItem.Budget") %>'></asp:Label>
</ItemTemplate>
<EditItemTemplate>
<asp:TextBox id="txtEditBudget" size="6" runat="server" Text='<%# DataBinder.Eval(Container, "DataItem.Budget") %>'></asp:TextBox>
</EditItemTemplate>
<FooterTemplate>
<asp:TextBox id="txtAddBudget" size="6" runat="server"></asp:TextBox>
</FooterTemplate>
</asp:TemplateColumn>
<asp:TemplateColumn HeaderText="Used">
<ItemTemplate>
<asp:Label id="lblUsed" runat="server" Text='<%# DataBinder.Eval(Container, "DataItem.Used") %>'></asp:Label>
</ItemTemplate>
<EditItemTemplate>
<asp:TextBox id="txtEditUsed" size="6" runat="server" Text='<%# DataBinder.Eval(Container, "DataItem.Used") %>'></asp:TextBox>
</EditItemTemplate>
<FooterTemplate>
<asp:TextBox id="txtAddUsed" size="6" runat="server"></asp:TextBox>
<asp:Button id="btnAdd" runat="server" Text="Add" CommandName="Add"></asp:Button>
</FooterTemplate>
</asp:TemplateColumn>
<asp:EditCommandColumn ButtonType="LinkButton" UpdateText="Update" CancelText="Cancel" EditText="Edit" HeaderText="Modify" ></asp:EditCommandColumn>
<asp:ButtonColumn Text="Delete" CommandName="Delete" HeaderText="Delete"></asp:ButtonColumn>
</Columns>
</asp:DataGrid>
</form>
</body>
</html>
DataGrid1.aspx.vb
Imports System.Data
Imports System.Data.OleDb
Partial Class DataGrid1
Inherits System.Web.UI.Page
Dim objConn As OleDbConnection
Dim objCmd As OleDbCommand
Dim strSQL As String
Protected Sub Page_Load(ByVal sender As Object, ByVal e As System.EventArgs) Handles Me.Load
Dim strConnString As String
strConnString = "Provider=Microsoft.Jet.OLEDB.4.0;Data Source=" & Server.MapPath("database/mydatabase.mdb") & ";"
objConn = New OleDbConnection(strConnString)
objConn.Open()
If Not Page.IsPostBack() Then
BindData()
End If
End Sub
Protected Sub BindData()
strSQL = "SELECT * FROM customer"
Dim dtReader As OleDbDataReader
objCmd = New OleDbCommand(strSQL, objConn)
dtReader = objCmd.ExecuteReader()
'*** BindData to DataGrid ***'
myDataGrid.DataSource = dtReader
myDataGrid.DataBind()
dtReader.Close()
dtReader = Nothing
End Sub
Protected Sub Page_Unload(ByVal sender As Object, ByVal e As System.EventArgs) Handles Me.Unload
objConn.Close()
objConn = Nothing
End Sub
Protected Sub myDataGrid_CancelCommand(ByVal source As Object, ByVal e As System.Web.UI.WebControls.DataGridCommandEventArgs) Handles myDataGrid.CancelCommand
myDataGrid.EditItemIndex = -1
myDataGrid.ShowFooter = True
BindData()
End Sub
Protected Sub myDataGrid_DeleteCommand(ByVal source As Object, ByVal e As System.Web.UI.WebControls.DataGridCommandEventArgs) Handles myDataGrid.DeleteCommand
strSQL = "DELETE FROM customer WHERE CustomerID = '" & myDataGrid.DataKeys.Item(e.Item.ItemIndex) & "'"
objCmd = New OleDbCommand(strSQL, objConn)
objCmd.ExecuteNonQuery()
myDataGrid.EditItemIndex = -1
BindData()
End Sub
Protected Sub myDataGrid_EditCommand(ByVal source As Object, ByVal e As System.Web.UI.WebControls.DataGridCommandEventArgs) Handles myDataGrid.EditCommand
myDataGrid.EditItemIndex = e.Item.ItemIndex
myDataGrid.ShowFooter = False
BindData()
End Sub
Protected Sub myDataGrid_ItemCommand(ByVal source As Object, ByVal e As System.Web.UI.WebControls.DataGridCommandEventArgs) Handles myDataGrid.ItemCommand
If e.CommandName = "Add" Then
'*** CustomerID ***'
Dim txtCustomerID As TextBox = CType(e.Item.FindControl("txtAddCustomerID"), TextBox)
'*** Email ***'
Dim txtName As TextBox = CType(e.Item.FindControl("txtAddName"), TextBox)
'*** Name ***'
Dim txtEmail As TextBox = CType(e.Item.FindControl("txtAddEmail"), TextBox)
'*** CountryCode ***'
Dim txtCountryCode As TextBox = CType(e.Item.FindControl("txtAddCountryCode"), TextBox)
'*** Budget ***'
Dim txtBudget As TextBox = CType(e.Item.FindControl("txtAddBudget"), TextBox)
'*** Used ***'
Dim txtUsed As TextBox = CType(e.Item.FindControl("txtAddUsed"), TextBox)
strSQL = "INSERT INTO customer (CustomerID,Name,Email,CountryCode,Budget,Used) " & _
" VALUES ('" & txtCustomerID.Text & "','" & txtName.Text & "','" & txtEmail.Text & "' " & _
" ,'" & txtCountryCode.Text & "','" & txtBudget.Text & "','" & txtUsed.Text & "') "
objCmd = New OleDbCommand(strSQL, objConn)
objCmd.ExecuteNonQuery()
BindData()
End If
End Sub
Protected Sub myDataGrid_UpdateCommand(ByVal source As Object, ByVal e As System.Web.UI.WebControls.DataGridCommandEventArgs) Handles myDataGrid.UpdateCommand
'*** CustomerID ***'
Dim txtCustomerID As TextBox = CType(e.Item.FindControl("txtEditCustomerID"), TextBox)
'*** Email ***'
Dim txtName As TextBox = CType(e.Item.FindControl("txtEditName"), TextBox)
'*** Name ***'
Dim txtEmail As TextBox = CType(e.Item.FindControl("txtEditEmail"), TextBox)
'*** CountryCode ***'
Dim txtCountryCode As TextBox = CType(e.Item.FindControl("txtEditCountryCode"), TextBox)
'*** Budget ***'
Dim txtBudget As TextBox = CType(e.Item.FindControl("txtEditBudget"), TextBox)
'*** Used ***'
Dim txtUsed As TextBox = CType(e.Item.FindControl("txtEditUsed"), TextBox)
strSQL = "UPDATE customer SET CustomerID = '" & txtCustomerID.Text & "' " & _
" ,Name = '" & txtName.Text & "' " & _
" ,Email = '" & txtEmail.Text & "' " & _
" ,CountryCode = '" & txtCountryCode.Text & "' " & _
" ,Budget = '" & txtBudget.Text & "' " & _
" ,Used = '" & txtUsed.Text & "' " & _
" WHERE CustomerID = '" & myDataGrid.DataKeys.Item(e.Item.ItemIndex) & "'"
objCmd = New OleDbCommand(strSQL, objConn)
objCmd.ExecuteNonQuery()
myDataGrid.EditItemIndex = -1
myDataGrid.ShowFooter = True
BindData()
End Sub
End Class
Screenshot
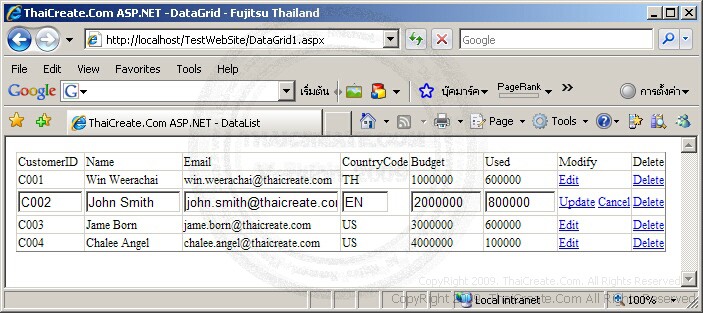
|