(C#) ASP.NET CheckBoxList & DataBinding |
(C#) ASP.NET CheckBoxList & DataBinding เป็นการใช้ CheckBoxList เรียกข้อมูลจาก Database ผ่าน ADO.NET ตัวอย่างนี้ผมได้ยกตัวอย่างการใช้บน DataTable,TableRows,SortedList รวมทั้งการเพิ่ม Item เข้าไปใน Control
Language Code : VB.NET || C#
Framework : 1,2,3,4
AspNetCheckBoxListDataBind.aspx
<%@ Import Namespace="System.Data"%>
<%@ Import Namespace="System.Data.OleDb"%>
<%@ Page Language="C#" Debug="true" %>
<script runat="server">
void Page_Load(object sender, EventArgs e)
{
if(!Page.IsPostBack)
{
CheckBoxListDataTable();
CheckBoxListDataTableRows();
CheckBoxListSortedList();
CheckBoxListAddInsertItem();
}
}
//*** CheckBoxList & DataTable ***//
void CheckBoxListDataTable()
{
OleDbConnection objConn;
OleDbDataAdapter dtAdapter;
DataTable dt = new DataTable();
String strConnString;
strConnString = "Provider=Microsoft.Jet.OLEDB.4.0;Data Source="+
Server.MapPath("database/mydatabase.mdb")+"";
objConn = new OleDbConnection(strConnString);
objConn.Open();
String strSQL;
strSQL = "SELECT * FROM country";
dtAdapter = new OleDbDataAdapter(strSQL, objConn);
dtAdapter.Fill(dt);
dtAdapter = null;
objConn.Close();
objConn = null;
//*** CheckBoxList ***//
this.myCBoxList1.DataSource = dt;
this.myCBoxList1.DataTextField = "CountryName";
this.myCBoxList1.DataValueField = "CountryCode";
this.myCBoxList1.DataBind();
//*** Default Value ***//
myCBoxList1.SelectedIndex = myCBoxList1.Items.IndexOf(myCBoxList1.Items.FindByValue("TH")); //*** By DataValueField ***//
//myCBoxList1.SelectedIndex = myCBoxList1.Items.IndexOf(myCBoxList1.Items.FindByText("Thailand")); //*** By DataTextField ***//
}
//*** CheckBoxList & TableRows ***//
void CheckBoxListDataTableRows()
{
DataTable dt = new DataTable();
DataRow dr;
//*** Column ***//
dt.Columns.Add("Sex");
dt.Columns.Add("SexDesc");
//*** Rows ***//
dr = dt.NewRow();
dr["Sex"] = "M";
dr["SexDesc"] = "Man";
dt.Rows.Add(dr);
//*** Rows ***//
dr = dt.NewRow();
dr["Sex"] = "W";
dr["SexDesc"] = "Woman";
dt.Rows.Add(dr);
//*** CheckBoxList ***//
this.myCBoxList2.DataSource = dt;
this.myCBoxList2.DataTextField = "SexDesc";
this.myCBoxList2.DataValueField = "Sex";
this.myCBoxList2.DataBind();
//*** Default Value ***//
myCBoxList2.SelectedIndex = myCBoxList2.Items.IndexOf(myCBoxList2.Items.FindByValue("W")); //*** By DataValueField ***//
//myCBoxList2.SelectedIndex = myCBoxList2.Items.IndexOf(myCBoxList2.Items.FindByText("Woman")); //*** By DataTextField ***//
}
//*** CheckBoxList & SortedList ***//
void CheckBoxListSortedList()
{
SortedList mySortedList = new SortedList();
mySortedList.Add("M","Man");
mySortedList.Add("W","Woman");
//*** CheckBoxList ***//
this.myCBoxList3.DataSource = mySortedList;
this.myCBoxList3.DataTextField = "Value";
this.myCBoxList3.DataValueField = "Key";
this.myCBoxList3.DataBind();
//*** Default Value ***//
myCBoxList3.SelectedIndex = myCBoxList3.Items.IndexOf(myCBoxList3.Items.FindByValue("W")); //*** By DataValueField ***//
//myCBoxList3.SelectedIndex = myCBoxList3.Items.IndexOf(myCBoxList3.Items.FindByText("Woman")); //*** By DataTextField ***//
}
//*** Add/Insert Items ***//
void CheckBoxListAddInsertItem()
{
SortedList mySortedList = new SortedList();
mySortedList.Add("M","Man");
mySortedList.Add("W","Woman");
//*** CheckBoxList ***//
this.myCBoxList4.DataSource = mySortedList;
this.myCBoxList4.DataTextField = "Value";
this.myCBoxList4.DataValueField = "Key";
this.myCBoxList4.DataBind();
//*** Add & Insert New Item ***//
String strText,strValue;
//*** Insert Item ***//
strText = "";
strValue = "";
ListItem InsertItem = new ListItem(strText, strValue);
myCBoxList4.Items.Insert(0, InsertItem);
//*** Add Items ***//
strText = "Guy";
strValue = "G";
ListItem AddItem = new ListItem(strText, strValue);
myCBoxList4.Items.Add(AddItem);
//*** Default Value ***//
myCBoxList4.SelectedIndex = myCBoxList4.Items.IndexOf(myCBoxList4.Items.FindByValue("W")); //*** By DataValueField ***//
//myCBoxList4.SelectedIndex = myCBoxList4.Items.IndexOf(myCBoxList4.Items.FindByText("Woman")); //*** By DataTextField ***//
}
void Button1_OnClick(object sender, EventArgs e)
{
int i;
this.lblText1.Text = "";
for(i = 0 ; i <= this.myCBoxList1.Items.Count - 1; i ++)
{
if(this.myCBoxList1.Items[i].Selected==true)
{
this.lblText1.Text = this.lblText1.Text + "," + this.myCBoxList1.Items[i].Value; //*** Or this.myCBoxList1.Items[i].Text ***//
}
}
this.lblText2.Text = "";
for(i = 0 ; i <= this.myCBoxList2.Items.Count - 1; i ++)
{
if(this.myCBoxList2.Items[i].Selected==true)
{
this.lblText2.Text = this.lblText2.Text + "," + this.myCBoxList2.Items[i].Value; //*** Or this.myCBoxList2.Items[i].Text ***//
}
}
this.lblText3.Text = "";
for(i = 0 ; i <= this.myCBoxList3.Items.Count - 1; i ++)
{
if(this.myCBoxList3.Items[i].Selected==true)
{
this.lblText3.Text = this.lblText3.Text + "," + this.myCBoxList3.Items[i].Value; //*** Or this.myCBoxList3.Items[i].Text ***//
}
}
this.lblText4.Text = "";
for(i = 0 ; i <= this.myCBoxList4.Items.Count - 1; i ++)
{
if(this.myCBoxList4.Items[i].Selected==true)
{
this.lblText4.Text = this.lblText4.Text + "," + this.myCBoxList4.Items[i].Value; //*** Or this.myCBoxList4.Items[i].Text ***//
}
}
}
</script>
<html>
<head>
<title>ThaiCreate.Com ASP.NET - CheckBoxList & DataBind</title>
</head>
<body>
<form id="form1" runat="server">
<asp:CheckBoxList id="myCBoxList1" runat="server"></asp:CheckBoxList><hr />
<asp:CheckBoxList id="myCBoxList2" runat="server"></asp:CheckBoxList><hr />
<asp:CheckBoxList id="myCBoxList3" runat="server"></asp:CheckBoxList><hr />
<asp:CheckBoxList id="myCBoxList4" runat="server"></asp:CheckBoxList>
<asp:Button id="Button1" onclick="Button1_OnClick" runat="server" Text="Button"></asp:Button>
<hr />
<asp:Label id="lblText1" runat="server"></asp:Label><br /><br />
<asp:Label id="lblText2" runat="server"></asp:Label><br /><br />
<asp:Label id="lblText3" runat="server"></asp:Label><br /><br />
<asp:Label id="lblText4" runat="server"></asp:Label><br /><br />
</form>
</body>
</html>
Screenshot
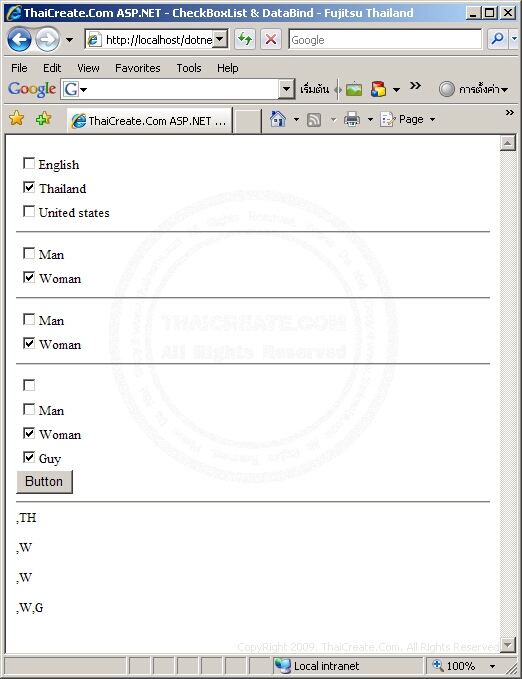
ASP.NET & AccessDataSource and CheckBoxList
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
  |
|
|
Create/Update Date : |
2008-11-22 09:25:52 /
2017-03-28 20:47:55 |
|
Download : |
|
|
Sponsored Links / Related |
|
|
|
|
|
|