Java Excel and Create Excel file (jExcelAPI) |
Java Excel and Create Excel file (jExcelAPI) ในการสร้างไฟล์ Excel ด้วยภาษา Java นั้น อยากจะแนะนำให้ใช้ Library ของ jExcelAP ซึ่งเป็น Library ที่สามารถใช้งานได้ฟรี มีรูปแบบใช้งานง่าย ได้รับความนิยมและมีคู่มือวิธีการใช้งานอย่างละเอียด สามารถสร้างไฟล์ Excel ได้ง่ายมาก อีกทั้งยัมความสามารถเกี่ยวกับการอ่าน Excel ไฟล์ได้อีกด้วย
Java Excel and Create Excel file
ก่อนการใช้งานเราจะต้องทำการ Download ตัว Library ของ jExcelAPI มาติดตั้งใน Project ของเราก่อน โดย Library ตัวนี้สามารถ Download ใช้งานได้ฟรี ได้รับความนิยม และมีรูปแบบการใช้งาน
Download jExcelAPI Library
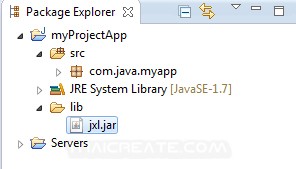
หลังจากที่ Download Library ไฟล์ jar ได้แล้วให้ Copy ไว้ในโฟเดอร์ lib
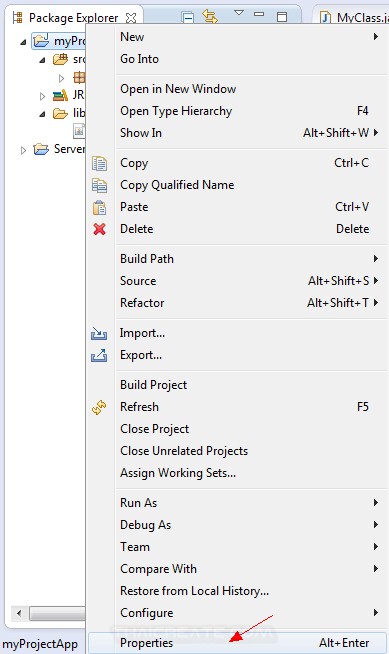
คลิกขวาที่ Project เลือก Properties
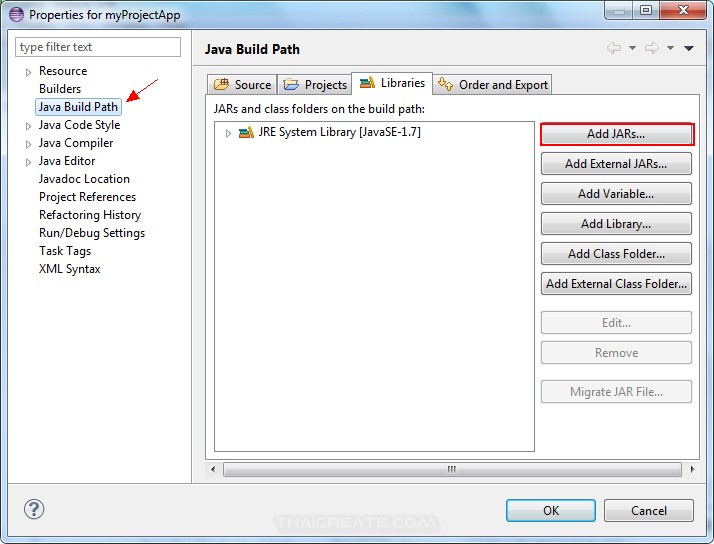
ในส่วนของ Build Path ให้เลือก Add JARs...
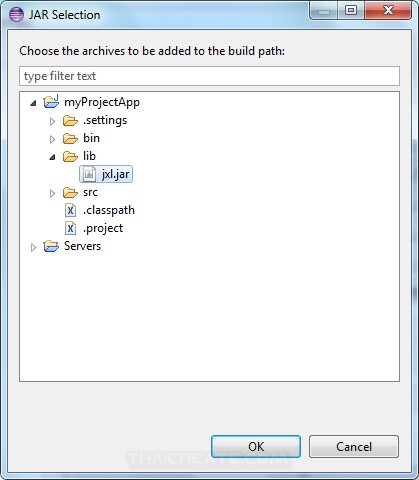
เลือกไฟล์ jar
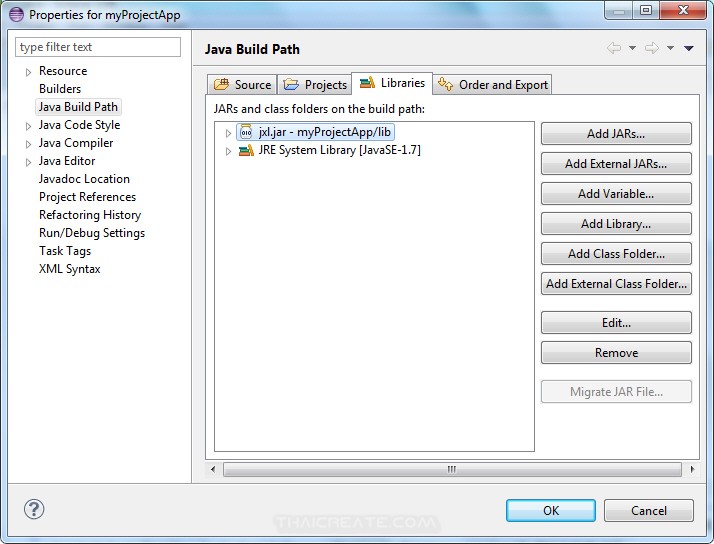
Library ถูก Add เข้ามาเรียบร้อยแล้ว
Example ตัวอย่างการสร้าง Excelไฟล์ ด้วย Java โดยใช้ Library ของ jExcelAPI
MyClass.java
package com.java.myapp;
import java.io.File;
import jxl.Workbook;
import jxl.write.Label;
import jxl.write.WritableSheet;
import jxl.write.WritableWorkbook;
public class MyClass {
public static void main(String[] args) {
try{
String fileName = "C:\\java\\myExcel.xls";
WritableWorkbook workbook = Workbook.createWorkbook(new File(fileName));
/*** Sheet 1 ***/
WritableSheet ws1 = workbook.createSheet("mySheet1", 0);
ws1.addCell(new Label(0,0,"Data 1"));
ws1.addCell(new Label(0,1,"Data 2"));
ws1.addCell(new Label(0,2,"Data 3"));
/*** Sheet 2 ***/
WritableSheet ws2 = workbook.createSheet("mySheet2", 1);
ws2.addCell(new Label(0,0,"Data 4"));
ws2.addCell(new Label(0,1,"Data 5"));
ws2.addCell(new Label(0,2,"Data 6"));
workbook.write();
workbook.close();
System.out.println("Excel file created.");
}
catch (Exception e) {
e.printStackTrace();
}
}
}
ในตัวอย่างนี้ทดสอบสร้างไฟล์ Excel ขึ้นมา 2 Sheet

Output
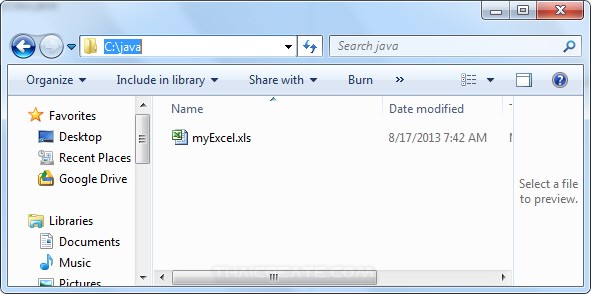
ไฟล์ Excel ถุกสร้างเรียบร้อยแล้ว
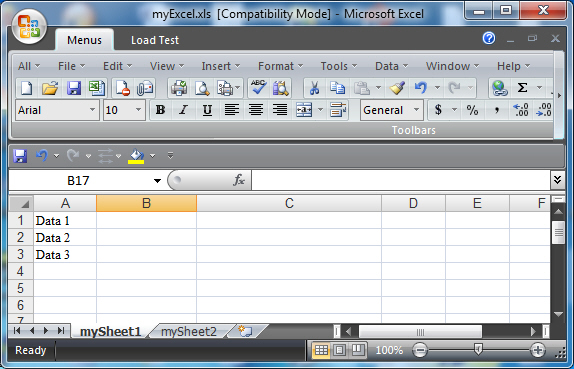
ผลลัพธ์ที่ได้ใน Sheet 1
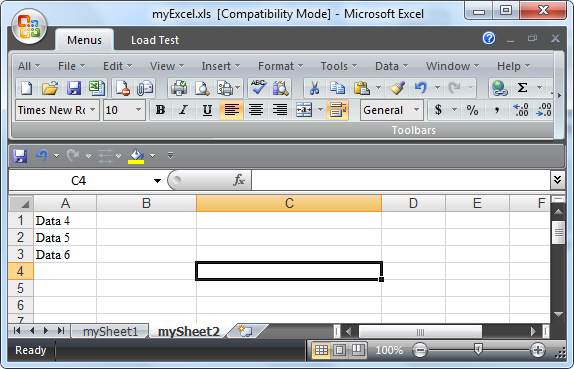
ผลลัพธ์ที่ได้ใน Sheet 2
คู่มือการใช้งาน jExcelAPI
http://jexcelapi.sourceforge.net/resources/faq/
|