Android Google Map : Marker Location (Latitude , Longitude) |
Android Google Map : Marker Location (Latitude, Longitude) ในหัวข้อนี้เราจะมารู้วิธีการ Marker (ปักหมุด) Location ลงใน Google Map ด้วย Android App จะเป็นวิธีการระบุตำแหน่งหรือพิกัด Location ที่เราต้องการะทำเป็นสัญลักษณ์ โดยตำแหน่งที่จะปักหมุด (Marker) นั้นเราจะอ้างจากค่า Latitude, Longitude นอกจากจะปักหมุดแล้วเรายังสามารถเพิ่ม Tooltip หรือคำอธิบายลงในแต่ล่ะหมุดได้ ส่วนจำนวนที่สามารถจะปักหมุดได้ลงนั้น ขึ้นอยู่กับ API ที่จะเรียกใช้งานว่าใน API Key นั้น ๆ รองรับได้กี่จุด
Android Google Map : Marker Location (Latitude, Longitude)
สำหรับตัวอย่างและ Code นี้รองรับการเขียนทั้งบนโปรแกรม Eclipse และ Android Studio
ใน AndroidManifest.xml เพิ่ม Permission ดังนี้
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" />
<uses-permission android:name="android.permission.INTERNET" />
<uses-permission android:name="com.google.android.providers.gsf.permission.READ_GSERVICES" />
<uses-permission android:name="android.permission.ACCESS_COARSE_LOCATION" />
<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" />
รูปแบบการ Marker Location บน Google Map
Marker Syntax
MarkerOptions marker = new MarkerOptions().position(new LatLng(Latitude, Longitude)).title(toolTip);
googleMap.addMarker(marker);
ในการ Maker Location บน Google Map สามารถใช้คำสั่ง addMarker(marker) ซึ่งรองรับการปักหมุดได้ในหลาย ๆ ตำแหน่ง (จำกัดอยู่ที่ API ว่าได้กี่ตำแหน่ง) อีกทั้งยังสามารถใส่ Tooltip ลงใน Marker ได้อีกด้วย
Example 1 : ตัวอย่างการเขียน Android App ปักหมุดหรือ Mark Location จาก Latitude และ Longitude
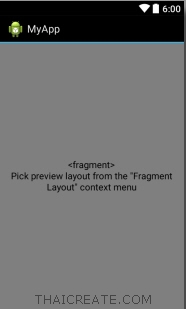
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<fragment xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/googleMap"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:name="com.google.android.gms.maps.SupportMapFragment"/>
MainActivity.java
package com.myapp;
import com.google.android.gms.maps.GoogleMap;
import com.google.android.gms.maps.SupportMapFragment;
import com.google.android.gms.maps.model.LatLng;
import com.google.android.gms.maps.model.MarkerOptions;
import android.os.Bundle;
import android.support.v4.app.FragmentActivity;
import com.google.android.gms.maps.CameraUpdateFactory;
public class MainActivity extends FragmentActivity {
// Google Map
private GoogleMap googleMap;
// Latitude & Longitude
private Double Latitude = 0.00;
private Double Longitude = 0.00;
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Latitude = 13.844205;
Longitude = 100.598856;
if(Latitude > 0 && Longitude > 0)
{
//*** Display Google Map
googleMap = ((SupportMapFragment)getSupportFragmentManager()
.findFragmentById(R.id.googleMap)).getMap();
//*** Focus & Zoom
LatLng coordinate = new LatLng(Latitude, Longitude);
googleMap.setMapType(com.google.android.gms.maps.GoogleMap.MAP_TYPE_HYBRID);
googleMap.animateCamera(CameraUpdateFactory.newLatLngZoom(coordinate, 17));
//*** Marker
String toolTip = String.format("Your Location Lat=%s, Lon=%s", Latitude,Longitude);
MarkerOptions marker = new MarkerOptions().position(new LatLng(Latitude, Longitude)).title(toolTip);
googleMap.addMarker(marker);
}
}
}

Screenshot
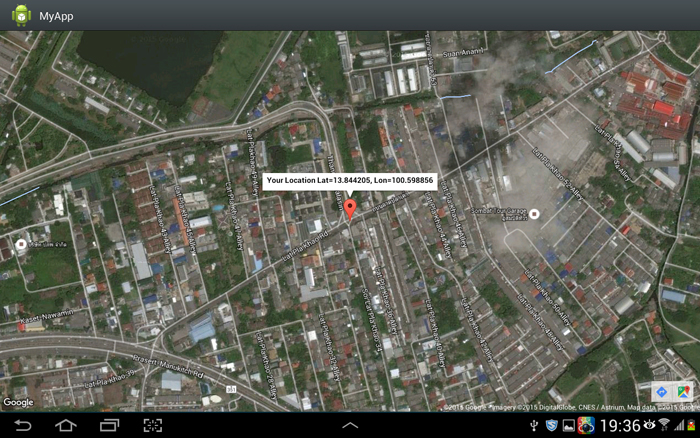
แสดงตำแหน่งที่ Marker (ปักหมุด)
กรณีต้องการเปลี่ยนสีของหมุด
String toolTip = String.format("Your Location Lat=%s, Lon=%s", Latitude,Longitude);
MarkerOptions marker = new MarkerOptions().position(new LatLng(Latitude, Longitude)).title(toolTip);
marker.icon(BitmapDescriptorFactory.defaultMarker(BitmapDescriptorFactory.HUE_BLUE));
googleMap.addMarker(marker);
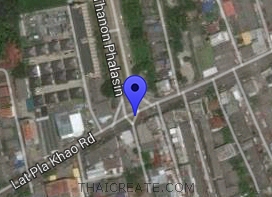
ตัวอย่างการเปลี่ยนสีของหมุดเป็นน้ำเงิน
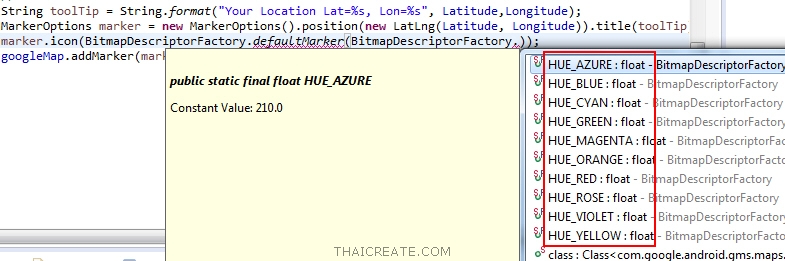
นอกจากนี้ยังสามารถเปลี่ยนเป็นสีอื่น ๆ ได้อีกมากมาย
Example 2 : การเปลี่ยนรูปภาพ (Image) ของหมุด (Marker) ที่จะปักลงบน Map
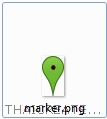
รูปภาพของหมุดแนะนำให้เป็น .png
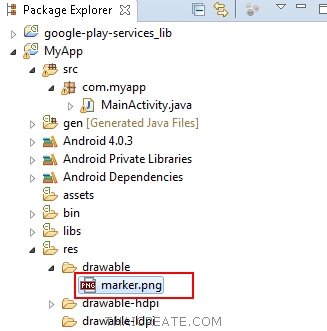
จัดเก็บลงใน drawable
Syntax
//*** Marker
String toolTip = String.format("Your Location Lat=%s, Lon=%s", Latitude,Longitude);
MarkerOptions marker = new MarkerOptions().position(new LatLng(Latitude, Longitude)).title(toolTip);
marker.icon(BitmapDescriptorFactory.fromResource(R.drawable.marker));
googleMap.addMarker(marker);
Screenshot
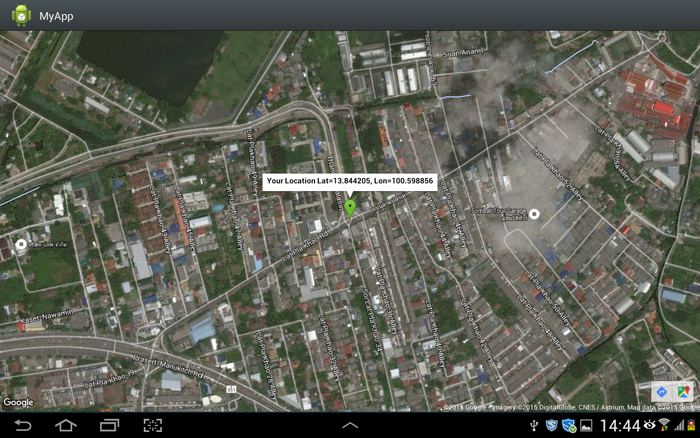
หมุด (Marker) ใหม่ที่ได้
.
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
   |
|
|
Create/Update Date : |
2015-11-21 22:50:44 /
2017-03-26 21:15:40 |
|
Download : |
No files |
|
Sponsored Links / Related |
|
|
|
|
|
|
|