Android Login Form via Web Service |
Android Login Form via Web Service การทำ Android Login Form เพื่อตรวจสอบข้อมูล Username และ Password ที่อยู่ใน Server ผ่านช่องทางของ Web Service หลักการก็คือออกแบบ Web Service ที่ทำหน้าที่ตรวจสอบการ Login โดยรอรับค่า Username และ Password จาก Client เมื่อ Android Client ได้ส่ง Username และ Password มายัง Web Service ก็จะนำข้อมูลไปตรวจสอบใน Database ถ้าข้อมูลถุกต้องก็จะส่งสถานะการ Login กลับไปยัง Android Client
Android and Web Service
Screenshot Form Login ที่ทำงานอยู่บน Android กับ Web Service
AndroidManifest.xml
<uses-permission android:name="android.permission.INTERNET" />
ในการเขียน Android เพื่อติดต่อกับ Internet จะต้องกำหนด Permission ในส่วนนี้ด้วยทุกครั้ง
Web Service (PHP กับ MySQL)
member
CREATE TABLE `member` (
`MemberID` int(2) NOT NULL auto_increment,
`Username` varchar(50) NOT NULL,
`Password` varchar(50) NOT NULL,
`Name` varchar(50) NOT NULL,
`Tel` varchar(50) NOT NULL,
`Email` varchar(150) NOT NULL,
PRIMARY KEY (`MemberID`),
UNIQUE KEY `Username` (`Username`),
UNIQUE KEY `Email` (`Email`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8 AUTO_INCREMENT=4 ;
--
-- Dumping data for table `member`
--
INSERT INTO `member` VALUES (1, 'weerachai', 'weerachai@1', 'Weerachai Nukitram', '0819876107', '[email protected]');
INSERT INTO `member` VALUES (2, 'adisorn', 'adisorn@2', 'Adisorn Bunsong', '021978032', '[email protected]');
INSERT INTO `member` VALUES (3, 'surachai', 'surachai@3', 'Surachai Sirisart', '0876543210', '[email protected]');

chkLogin.php ใช้สำหรับตรวจสอบข้อมูลการ Login
<?php
ob_start();
require_once("lib/nusoap.php");
//Create a new soap server
$server = new soap_server();
//Define our namespace
$namespace = "https://www.thaicreate.com/android/chkLogin.php";
$server->wsdl->schemaTargetNamespace = $namespace;
//Configure our WSDL
$server->configureWSDL("loginMember");
// Register our method and argument parameters
$varname = array(
'strUsername' => "xsd:string",
'strPassword' => "xsd:string"
);
$server->register('loginMember',$varname, array('return' => 'xsd:string'));
function loginMember($strUsername,$strPassword)
{
$objConnect = mysql_connect("localhost","root","root");
$objDB = mysql_select_db("mydatabase");
$strSQL = "SELECT * FROM member WHERE 1
AND Username = '".$strUsername."'
AND Password = '".$strPassword."'
";
$objQuery = mysql_query($strSQL);
$objResult = mysql_fetch_array($objQuery);
$intNumRows = mysql_num_rows($objQuery);
if($intNumRows==0)
{
$arr['StatusID'] = "0";
$arr['MemberID'] = "0";
$arr['Error'] = "Incorrect Username and Password";
}
else
{
$arr['StatusID'] = "1";
$arr['MemberID'] = $objResult["MemberID"];
$arr['Error'] = "";
}
mysql_close($objConnect);
header('Content-type: application/json');
return json_encode($arr);
}
// Get our posted data if the service is being consumed
// otherwise leave this data blank.
$POST_DATA = isset($GLOBALS['HTTP_RAW_POST_DATA']) ? $GLOBALS['HTTP_RAW_POST_DATA'] : '';
// pass our posted data (or nothing) to the soap service
$server->service($POST_DATA);
exit();
?>
resultByMemberID.php ส่งข้อมูลรายละเอียดของ User กลับไปเมื่อ Login ถูกต้อง
<?php
ob_start();
require_once("lib/nusoap.php");
//Create a new soap server
$server = new soap_server();
//Define our namespace
$namespace = "https://www.thaicreate.com/android/resultByMemberID.php";
$server->wsdl->schemaTargetNamespace = $namespace;
//Configure our WSDL
$server->configureWSDL("resultMember");
// Register our method and argument parameters
$varname = array(
'strMemberID' => "xsd:string"
);
$server->register('resultMember',$varname, array('return' => 'xsd:string'));
function resultMember($strMemberID)
{
$objConnect = mysql_connect("localhost","root","root");
$objDB = mysql_select_db("mydatabase");
$strSQL = "SELECT * FROM member WHERE 1
AND MemberID = '".$strMemberID."'
";
$objQuery = mysql_query($strSQL);
$obResult = mysql_fetch_array($objQuery);
if($obResult)
{
$arr["MemberID"] = $obResult["MemberID"];
$arr["Username"] = $obResult["Username"];
$arr["Password"] = $obResult["Password"];
$arr["Name"] = $obResult["Name"];
$arr["Email"] = $obResult["Email"];
$arr["Tel"] = $obResult["Tel"];
}
mysql_close($objConnect);
header('Content-type: application/json');
return json_encode($arr);
}
// Get our posted data if the service is being consumed
// otherwise leave this data blank.
$POST_DATA = isset($GLOBALS['HTTP_RAW_POST_DATA']) ? $GLOBALS['HTTP_RAW_POST_DATA'] : '';
// pass our posted data (or nothing) to the soap service
$server->service($POST_DATA);
exit();
?>
Android Project
โครงสร้างของไฟล์ประกอบด้วย 4 ไฟล์คือ MainActivity.java, activity_main.xml และ DetailActivity.java, activity_detail.xml
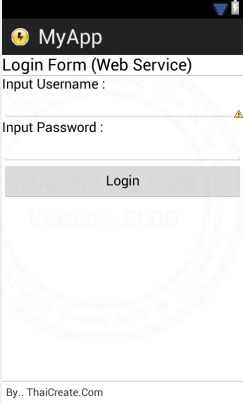
activity_main.xml
<TableLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/tableLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<TableRow
android:id="@+id/tableRow1"
android:layout_width="wrap_content"
android:layout_height="wrap_content" >
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:gravity="center"
android:text="Login Form (Web Service) "
android:layout_span="1"
android:textAppearance="?android:attr/textAppearanceLarge" />
</TableRow>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<TableLayout
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_weight="0.1"
android:orientation="horizontal">
<TextView
android:id="@+id/textView3"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Input Username :"
android:textAppearance="?android:attr/textAppearanceMedium" />
<EditText
android:id="@+id/txtUsername"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:ems="10" >
</EditText>
<TextView
android:id="@+id/textView4"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Input Password :"
android:textAppearance="?android:attr/textAppearanceMedium" />
<EditText
android:id="@+id/txtPassword"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:ems="10"
android:inputType="textPassword" >
</EditText>
<Button
android:id="@+id/btnLogin"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Login" />
</TableLayout >
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<LinearLayout
android:id="@+id/LinearLayout1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="5dip" >
<TextView
android:id="@+id/textView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="By.. ThaiCreate.Com" />
</LinearLayout>
</TableLayout>
MainActivity.java
package com.myapp;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.util.List;
import android.app.AlertDialog;
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.NameValuePair;
import org.apache.http.StatusLine;
import org.apache.http.client.ClientProtocolException;
import org.apache.http.client.HttpClient;
import org.apache.http.client.entity.UrlEncodedFormEntity;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.impl.client.DefaultHttpClient;
import org.json.JSONException;
import org.json.JSONObject;
import org.ksoap2.SoapEnvelope;
import org.ksoap2.serialization.SoapObject;
import org.ksoap2.serialization.SoapSerializationEnvelope;
import org.ksoap2.transport.HttpTransportSE;
import org.xmlpull.v1.XmlPullParserException;
import android.os.Bundle;
import android.os.StrictMode;
import android.annotation.SuppressLint;
import android.app.Activity;
import android.content.Intent;
import android.util.Log;
import android.view.View;
import android.view.Menu;
import android.widget.Button;
import android.widget.EditText;
import android.widget.Toast;
public class MainActivity extends Activity {
private final String NAMESPACE = "https://www.thaicreate.com/android/chkLogin.php";
private final String URL = "https://www.thaicreate.com/android/chkLogin.php?wsdl"; // WSDL URL
private final String SOAP_ACTION = "https://www.thaicreate.com/android/chkLogin.php/loginMember";
private final String METHOD_NAME = "loginMember"; // Method on web service
@SuppressLint("NewApi")
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Permission StrictMode
if (android.os.Build.VERSION.SDK_INT > 9) {
StrictMode.ThreadPolicy policy = new StrictMode.ThreadPolicy.Builder().permitAll().build();
StrictMode.setThreadPolicy(policy);
}
final AlertDialog.Builder ad = new AlertDialog.Builder(this);
// txtUsername & txtPassword
final EditText txtUser = (EditText)findViewById(R.id.txtUsername);
final EditText txtPass = (EditText)findViewById(R.id.txtPassword);
// btnLogin
final Button btnLogin = (Button) findViewById(R.id.btnLogin);
// Perform action on click
btnLogin.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
SoapObject request = new SoapObject(NAMESPACE, METHOD_NAME);
request.addProperty("strUsername", txtUser.getText().toString());
request.addProperty("strPassword", txtPass.getText().toString());
SoapSerializationEnvelope envelope = new SoapSerializationEnvelope(
SoapEnvelope.VER11);
envelope.setOutputSoapObject(request);
HttpTransportSE androidHttpTransport = new HttpTransportSE(URL);
String resultServer = null;
try {
androidHttpTransport.call(SOAP_ACTION, envelope);
SoapObject result = (SoapObject) envelope.bodyIn;
resultServer = result.getProperty(0).toString();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (XmlPullParserException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
/** Get result from Server (Return the JSON Code)
* StatusID = ? [0=Failed,1=Complete]
* MemberID = ? [Eg : 1]
* Error = ? [On case error return custom error message]
*
* Eg Login Failed = {"StatusID":"0","MemberID":"0","Error":"Incorrect Username and Password"}
* Eg Login Complete = {"StatusID":"1","MemberID":"2","Error":""}
*/
/*** Default Value ***/
String strStatusID = "0";
String strMemberID = "0";
String strError = "Unknow Status!";
JSONObject c;
try {
c = new JSONObject(resultServer);
strStatusID = c.getString("StatusID");
strMemberID = c.getString("MemberID");
strError = c.getString("Error");
} catch (JSONException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
// Prepare Login
if(strStatusID.equals("0"))
{
// Dialog
ad.setTitle("Error! ");
ad.setIcon(android.R.drawable.btn_star_big_on);
ad.setPositiveButton("Close", null);
ad.setMessage(strError);
ad.show();
txtUser.setText("");
txtPass.setText("");
}
else
{
Toast.makeText(MainActivity.this, "Login OK", Toast.LENGTH_SHORT).show();
Intent newActivity = new Intent(MainActivity.this,DetailActivity.class);
newActivity.putExtra("MemberID", strMemberID);
startActivity(newActivity);
}
}
});
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.activity_main, menu);
return true;
}
}
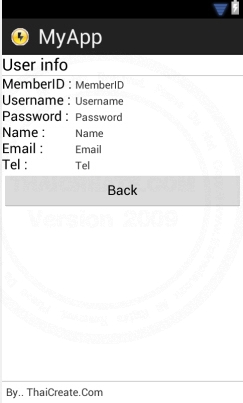
activity_detail.xml
<TableLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/tableLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<TableRow
android:id="@+id/tableRow1"
android:layout_width="wrap_content"
android:layout_height="wrap_content" >
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:gravity="center"
android:text="User info "
android:layout_span="1"
android:textAppearance="?android:attr/textAppearanceLarge" />
</TableRow>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<TableLayout
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_weight="0.1"
android:orientation="horizontal">
<TableRow
android:layout_width="fill_parent"
android:layout_height="wrap_content">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="MemberID : "
android:textAppearance="?android:attr/textAppearanceMedium" />
<TextView
android:id="@+id/txtMemberID"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="MemberID" />
</TableRow>
<TableRow
android:layout_width="fill_parent"
android:layout_height="wrap_content">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Username : "
android:textAppearance="?android:attr/textAppearanceMedium" />
<TextView
android:id="@+id/txtUsername"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Username" />
</TableRow>
<TableRow
android:layout_width="fill_parent"
android:layout_height="wrap_content">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Password : "
android:textAppearance="?android:attr/textAppearanceMedium" />
<TextView
android:id="@+id/txtPassword"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Password" />
</TableRow>
<TableRow
android:layout_width="fill_parent"
android:layout_height="wrap_content">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Name : "
android:textAppearance="?android:attr/textAppearanceMedium" />
<TextView
android:id="@+id/txtName"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Name" />
</TableRow>
<TableRow
android:layout_width="fill_parent"
android:layout_height="wrap_content">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Email : "
android:textAppearance="?android:attr/textAppearanceMedium" />
<TextView
android:id="@+id/txtEmail"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Email" />
</TableRow>
<TableRow
android:layout_width="fill_parent"
android:layout_height="wrap_content">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Tel : "
android:textAppearance="?android:attr/textAppearanceMedium" />
<TextView
android:id="@+id/txtTel"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Tel" />
</TableRow>
<Button
android:id="@+id/btnBack"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Back" />
</TableLayout >
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<LinearLayout
android:id="@+id/LinearLayout1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="5dip" >
<TextView
android:id="@+id/textView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="By.. ThaiCreate.Com" />
</LinearLayout>
</TableLayout>
DetailActivity.java
package com.myapp;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.util.List;
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.NameValuePair;
import org.apache.http.StatusLine;
import org.apache.http.client.ClientProtocolException;
import org.apache.http.client.HttpClient;
import org.apache.http.client.entity.UrlEncodedFormEntity;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.impl.client.DefaultHttpClient;
import org.json.JSONException;
import org.json.JSONObject;
import org.ksoap2.SoapEnvelope;
import org.ksoap2.serialization.SoapObject;
import org.ksoap2.serialization.SoapSerializationEnvelope;
import org.ksoap2.transport.HttpTransportSE;
import org.xmlpull.v1.XmlPullParserException;
import android.os.Bundle;
import android.os.StrictMode;
import android.annotation.SuppressLint;
import android.app.Activity;
import android.content.Intent;
import android.util.Log;
import android.view.View;
import android.view.Menu;
import android.widget.Button;
import android.widget.TextView;
public class DetailActivity extends Activity {
private final String NAMESPACE = "https://www.thaicreate.com/android/resultByMemberID.php";
private final String URL = "https://www.thaicreate.com/android/resultByMemberID.php?wsdl"; // WSDL URL
private final String SOAP_ACTION = "https://www.thaicreate.com/android/resultByMemberID.php/resultMember";
private final String METHOD_NAME = "resultMember"; // Method on web service
@SuppressLint("NewApi")
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_detail);
// Permission StrictMode
if (android.os.Build.VERSION.SDK_INT > 9) {
StrictMode.ThreadPolicy policy = new StrictMode.ThreadPolicy.Builder().permitAll().build();
StrictMode.setThreadPolicy(policy);
}
showInfo();
// btnBack
final Button btnBack = (Button) findViewById(R.id.btnBack);
// Perform action on click
btnBack.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
Intent newActivity = new Intent(DetailActivity.this,MainActivity.class);
startActivity(newActivity);
}
});
}
public void showInfo()
{
// txtMemberID,txtMemberID,txtUsername,txtPassword,txtName,txtEmail,txtTel
final TextView tMemberID = (TextView)findViewById(R.id.txtMemberID);
final TextView tUsername = (TextView)findViewById(R.id.txtUsername);
final TextView tPassword = (TextView)findViewById(R.id.txtPassword);
final TextView tName = (TextView)findViewById(R.id.txtName);
final TextView tEmail = (TextView)findViewById(R.id.txtEmail);
final TextView tTel = (TextView)findViewById(R.id.txtTel);
Intent intent= getIntent();
final String MemberID = intent.getStringExtra("MemberID");
SoapObject request = new SoapObject(NAMESPACE, METHOD_NAME);
request.addProperty("strMemberID", MemberID);
SoapSerializationEnvelope envelope = new SoapSerializationEnvelope(
SoapEnvelope.VER11);
envelope.setOutputSoapObject(request);
HttpTransportSE androidHttpTransport = new HttpTransportSE(URL);
String resultServer = null;
try {
androidHttpTransport.call(SOAP_ACTION, envelope);
SoapObject result = (SoapObject) envelope.bodyIn;
resultServer = result.getProperty(0).toString();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (XmlPullParserException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
/** Get result from Server (Return the JSON Code)
*
* {"MemberID":"2","Username":"adisorn","Password":"adisorn@2","Name":"Adisorn Bunsong","Tel":"021978032","Email":"[email protected]"}
*/
String strMemberID = "";
String strUsername = "";
String strPassword = "";
String strName = "";
String strEmail = "";
String strTel = "";
JSONObject c;
try {
c = new JSONObject(resultServer);
strMemberID = c.getString("MemberID");
strUsername = c.getString("Username");
strPassword = c.getString("Password");
strName = c.getString("Name");
strEmail = c.getString("Email");
strTel = c.getString("Tel");
if(!strMemberID.equals(""))
{
tMemberID.setText(strMemberID);
tUsername.setText(strUsername);
tPassword.setText(strPassword);
tName.setText(strName);
tEmail.setText(strEmail);
tTel.setText(strTel);
}
else
{
tMemberID.setText("-");
tUsername.setText("-");
tPassword.setText("-");
tName.setText("-");
tEmail.setText("-");
tTel.setText("-");
}
} catch (JSONException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.activity_main, menu);
return true;
}
}
AndroidManifest.xml
<activity
android:name="DetailActivity"
android:theme="@style/AppTheme"
android:screenOrientation="portrait"
android:label="@string/title_activity_main" />
เพิ่ม DetailActivity ในไฟล์ AndroidManifest.xml
Screenshot
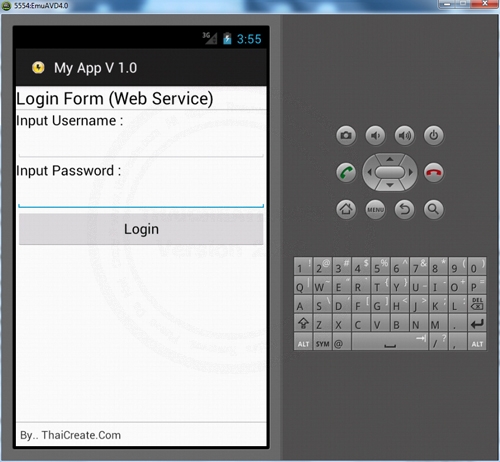
แสดงหน้าจอสำหรับการ Login
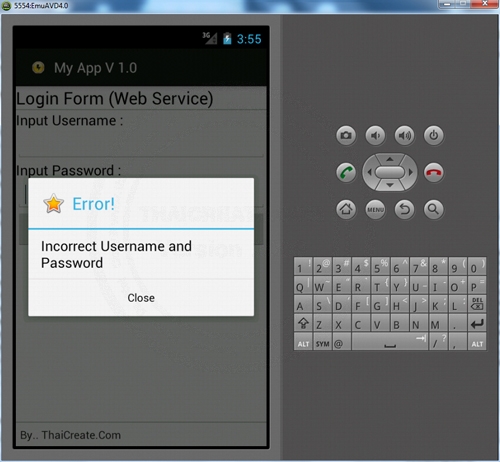
ในกรณีที่ Login ไม่ถุกต้อง
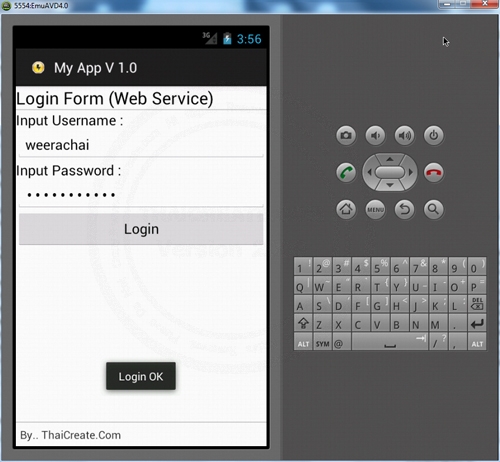
ในกรณีที่ Login ถูกต้อง
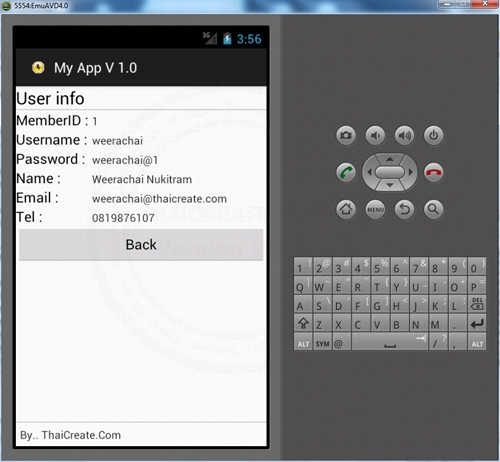
จะทำการ Intent ไปยังหน้า DetailActivity.java
.
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
  |
|
|
Create/Update Date : |
2012-08-11 15:23:49 /
2017-03-26 22:53:49 |
|
Download : |
No files |
|
Sponsored Links / Related |
|
|
|
|
|
|