Android and Web Service สำหรับ Web Service เป็นช่องทางหนึ่งที่นิยมใช้สำหรับการแลกเปลี่ยนข้อมูลระหว่าง Server กับ Client หรือ Server กับ Server เหตุผลที่ Web Service ได้รับความนิยมก็คือ การที่ Web Service มีมาตรฐานที่ได้ออกแบบไว้สำหรับการเชื่อมต่อผ่าน SOAP Interface ของ WSDL เพาะฉะนั้นไม่ว่าจะพัฒนาโปรแกรมด้วยภาษาอะไรก็ตาม เมื่อใช้ Web Service เป็นตัวกลางในการแลกเปลี่ยนข้อมูล ก็จะทำให้จัดการกับข้อมูลต่าง ๆ นั้นมีประสิทธิภาพและตรงกับความต้องการ
พื้นฐานของ Web Service จะใช้ XML เป็นตัวกลางในการแลกเปลี่ยนข้อมูล โดยอาจจะทำการส่งค่า String หรือ JSON มาพร้อมกับ XML ซึ่งปัจจุบัน JSON จะได้รับความนิยมในการรับส่งข้อมูลซะมากกว่า แต่ JSON เหล่านี้ยังคงถูกส่งภายใต้ XML เช่นเดียวกัน
Basic Web Service
AndroidManifest.xml
<uses-permission android:name="android.permission.INTERNET" />
ในการเขียน Android เพื่อติดต่อกับ Internet จะต้องกำหนด Permission ในส่วนนี้ด้วยทุกครั้ง
ทดสอบการสร้าง Web Service และเรียก Web Service ด้วย Android
WebServiceServer.php
<?php
require_once("lib/nusoap.php");
//Create a new soap server
$server = new soap_server();
//Define our namespace
$namespace = "https://www.thaicreate.com/android/WebServiceServer.php";
$server->wsdl->schemaTargetNamespace = $namespace;
//Configure our WSDL
$server->configureWSDL("HelloWorld");
// Register our method and argument parameters
$varname = array(
'strName' => "xsd:string",
'strEmail' => "xsd:string"
);
$server->register('HelloWorld',$varname, array('return' => 'xsd:string'));
function HelloWorld($strName,$strEmail)
{
return "Hello, Khun ($strName , Your email : $strEmail)";
}
// Get our posted data if the service is being consumed
// otherwise leave this data blank.
$POST_DATA = isset($GLOBALS['HTTP_RAW_POST_DATA']) ? $GLOBALS['HTTP_RAW_POST_DATA'] : '';
// pass our posted data (or nothing) to the soap service
$server->service($POST_DATA);
exit();
?>
สร้าง Web Service ด้วย PHP โดยใช้ NuSoap (สามารถอ่านได้จากบทความของ PHP Web Service)
คำอธิบาย
เป็นการสร้าง Web Service แบบง่าย ๆ โดยมี method ชื่อว่า HelloWorld และรับค่า strName และ strEmail จากนั้นจะ Return ค่า Hello, Khun ($strName , Your email : $strEmail) กลับไปยัง Client
Web Service ถุกจัดเก็บไว้ที่
https://www.thaicreate.com/android/WebServiceServer.php
Android Project Web Service
ใน Android จะไม่มี Library ที่จะใช้สำหรับจัดการและติดต่อกับ Web Service ได้โดยตรง แต่จะมี Library ที่สามารถดาวน์โหลดมาใช้งานได้ฟรี โดยมีชื่อส่า KSOAP สามารถดาวน์โหลดได้จาก Link นี้
Download
http://code.google.com/p/ksoap2-android/wiki/HowToUse?tm=2
ไฟล์ที่ได้ตะอยู่ในรูปแบบของ Jar ไฟล์ โดยเราจะต้องนำมา Import เข้าใน Project
Android Import Jar Library
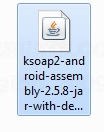
ไฟล์ jar library ของ Web Service
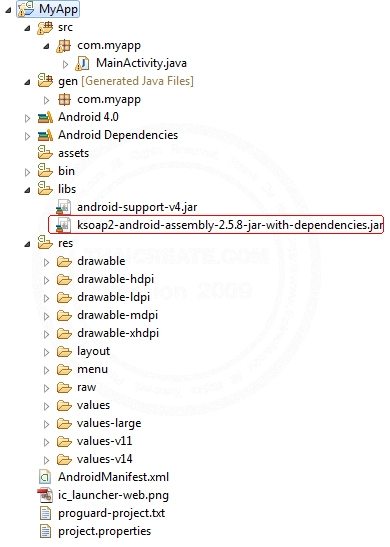
ให้ Copy ไฟล์ไปไว้ใน Android Project โดยจัดเก็บไว้ที่ /lib/
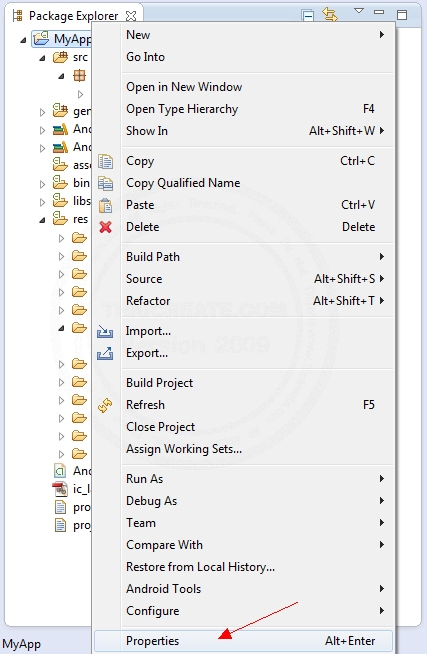
คลิกขวาที่ Project -> Properties
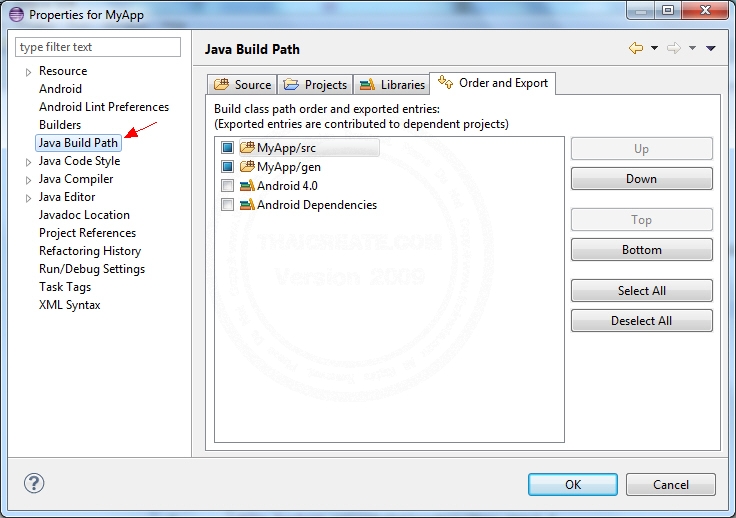
คลิกที่ Java Build Path -> Add JARs ดูรูปภาพประกอบ
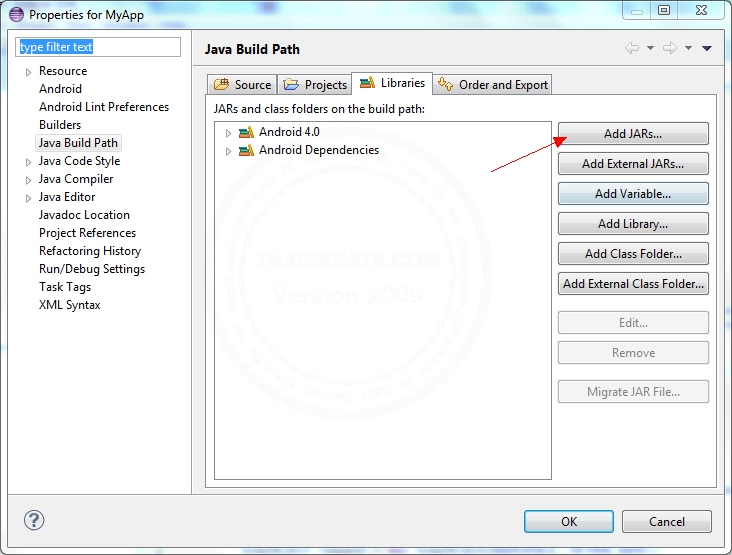
เลือกไฟล์ Library ที่อยู่ใน /lib/
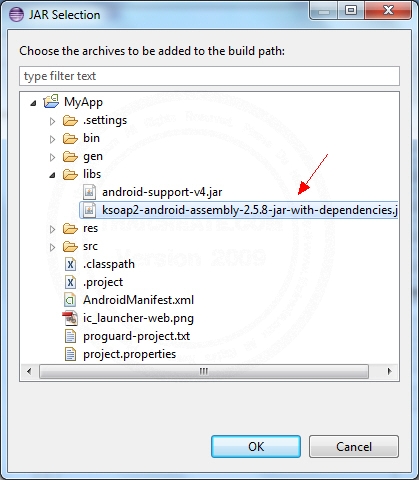
แสดงรายการ jar ไฟล์ที่ถูก Import เข้ามาใน Project
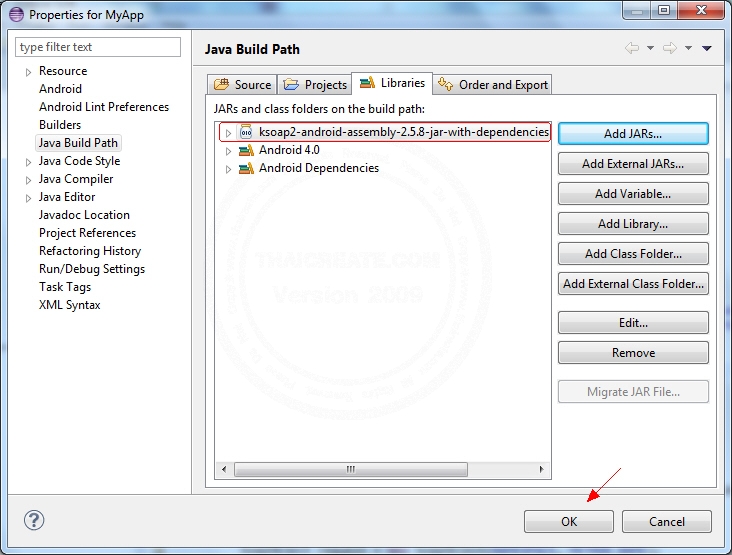
เลือก OK
โครงสร้างของไฟล์ประกอบด้วย 2 ไฟล์คือ MainActivity.java, activity_main.xml
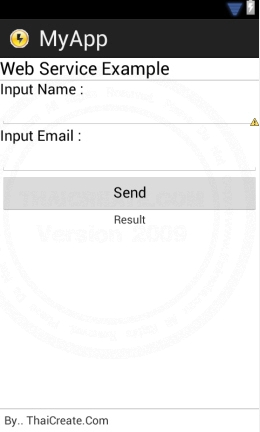
activity_main.xml
<TableLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/tableLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<TableRow
android:id="@+id/tableRow1"
android:layout_width="wrap_content"
android:layout_height="wrap_content" >
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:gravity="center"
android:text="Web Service Example "
android:layout_span="1"
android:textAppearance="?android:attr/textAppearanceLarge" />
</TableRow>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<TableLayout
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_weight="0.1"
android:orientation="horizontal">
<TableRow>
</TableRow>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Input Name :"
android:textAppearance="?android:attr/textAppearanceMedium" />
<EditText
android:id="@+id/txtName"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:ems="10" >
</EditText>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Input Email :"
android:textAppearance="?android:attr/textAppearanceMedium" />
<EditText
android:id="@+id/txtEmail"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:inputType="textEmailAddress"
android:ems="10" >
</EditText>
<Button
android:id="@+id/btnSend"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Send" />
<TextView
android:id="@+id/txtResult"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:gravity="center"
android:textAppearance="?android:attr/textAppearanceSmall"
android:text="Result" />
</TableLayout >
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<LinearLayout
android:id="@+id/LinearLayout1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="5dip" >
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="By.. ThaiCreate.Com" />
</LinearLayout>
</TableLayout>
MainActivity.java
package com.myapp;
import java.io.IOException;
import org.ksoap2.SoapEnvelope;
import org.ksoap2.serialization.SoapObject;
import org.ksoap2.serialization.SoapSerializationEnvelope;
import org.ksoap2.transport.HttpTransportSE;
import org.xmlpull.v1.XmlPullParserException;
import android.os.Bundle;
import android.os.StrictMode;
import android.view.Menu;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.TextView;
import android.widget.Toast;
import android.annotation.SuppressLint;
import android.app.Activity;
public class MainActivity extends Activity {
private final String NAMESPACE = "https://www.thaicreate.com/android/WebServiceServer.php";
private final String URL = "https://www.thaicreate.com/android/WebServiceServer.php?wsdl"; // WSDL URL
private final String SOAP_ACTION = "https://www.thaicreate.com/android/WebServiceServer.php/HelloWorld";
private final String METHOD_NAME = "HelloWorld"; // Method on web service
@SuppressLint("NewApi")
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Permission StrictMode
if (android.os.Build.VERSION.SDK_INT > 9) {
StrictMode.ThreadPolicy policy = new StrictMode.ThreadPolicy.Builder()
.permitAll().build();
StrictMode.setThreadPolicy(policy);
}
// btnSend
Button btnSend = (Button) this.findViewById(R.id.btnSend);
btnSend.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
// txtResult
TextView txtResult = (TextView) findViewById(R.id.txtResult);
// txtName
EditText txtName = (EditText) findViewById(R.id.txtName);
// txtEmail
EditText txtEmail = (EditText) findViewById(R.id.txtEmail);
SoapObject request = new SoapObject(NAMESPACE, METHOD_NAME);
request.addProperty("strName", txtName.getText().toString());
request.addProperty("strEmail", txtEmail.getText().toString());
SoapSerializationEnvelope envelope = new SoapSerializationEnvelope(
SoapEnvelope.VER11);
envelope.setOutputSoapObject(request);
HttpTransportSE androidHttpTransport = new HttpTransportSE(URL);
try {
androidHttpTransport.call(SOAP_ACTION, envelope);
SoapObject result = (SoapObject) envelope.bodyIn;
if (result != null) {
txtResult.setText(result.getProperty(0).toString());
} else {
Toast.makeText(getApplicationContext(),
"Web Service not Response!", Toast.LENGTH_LONG)
.show();
}
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (XmlPullParserException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
});
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.activity_main, menu);
return true;
}
}
คำอธิบาย
private final String NAMESPACE = "https://www.thaicreate.com/android/WebServiceServer.php";
เป็น NameSpace ของ Web Service สามารถปล่อยให้ว่างได้
private final String URL = "https://www.thaicreate.com/android/WebServiceServer.php?wsdl"; // WSDL URL
เป็น URL ของ WSDL ที่จะเรียกใช้งาน Web Service
private final String SOAP_ACTION = "https://www.thaicreate.com/android/WebServiceServer.php/HelloWorld";
เป็น Soap Action ปกติจะเป็น URL ตามด้วย Method
private final String METHOD_NAME = "HelloWorld"; // Method on web service
ชื่อ Method ของ Web Service
Screenshot
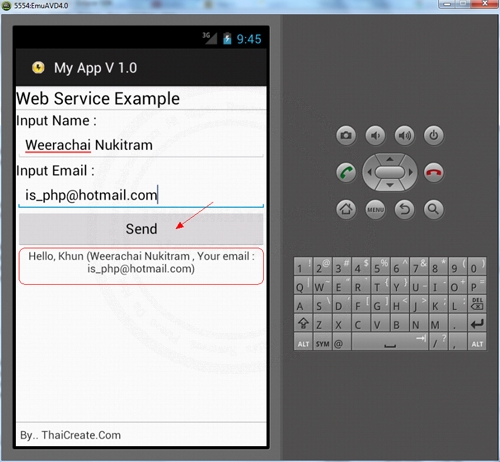
ทดสอบการ Request ไปยัง Web Service และการแสดงค่าที่ถูกส่งกลับมาจาก Web Service
Property & Method (Others Related) |
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
   |
|
|
Create/Update Date : |
2012-08-11 15:14:45 /
2017-03-26 21:48:21 |
|
Download : |
No files |
|
Sponsored Links / Related |
|
|
|
|
|
|